**C#**
namespace XSface
{
public partial class CSQLiteHelper : System.Web.UI.Page
{
private string _dbName = "";
private SQLiteConnection _SQLiteConn = null; //连接对象
private SQLiteTransaction _SQLiteTrans = null; //事务对象
private bool _IsRunTrans = false; //事务运行标识
private string _SQLiteConnString = null; //连接字符串
private bool _AutoCommit = false; //事务自动提交标识
public string SQLiteConnString
{
set { this._SQLiteConnString = value; }
get { return this._SQLiteConnString; }
}
public CSQLiteHelper(string dbPath)
{
this._dbName = dbPath;
this._SQLiteConnString = "Data Source=" + dbPath;
}
///
/// 新建数据库文件
///
/// 数据库文件路径及名称
/// 新建成功,返回true,否则返回false
static public Boolean NewDbFile(string dbPath)
{
try
{
SQLiteConnection.CreateFile(dbPath);
return true;
}
catch (Exception ex)
{
throw new Exception("新建数据库文件" + dbPath + "失败:" + ex.Message);
}
}
///
/// 创建表
///
/// 指定数据库文件
/// 表名称
static public void NewTable(string dbPath, string tableName)
{
SQLiteConnection sqliteConn = new SQLiteConnection("data source=" + dbPath);
if (sqliteConn.State != System.Data.ConnectionState.Open)
{
sqliteConn.Open();
SQLiteCommand cmd = new SQLiteCommand();
cmd.Connection = sqliteConn;
//cmd.CommandText = "CREATE TABLE " + tableName + "(Name varchar,Team varchar, Number varchar)";
cmd.CommandText = "CREATE TABLE " + tableName + "(name varchar,image varbinary, feature varbinary)";
cmd.ExecuteNonQuery();
}
sqliteConn.Close();
}
///
/// 打开当前数据库的连接
///
///
public Boolean OpenDb()
{
try
{
this._SQLiteConn = new SQLiteConnection(this._SQLiteConnString);
this._SQLiteConn.Open();
return true;
}
catch (Exception ex)
{
throw new Exception("打开数据库:" + _dbName + "的连接失败:" + ex.Message);
}
}
///
/// 打开指定数据库的连接
///
/// 数据库路径
///
public Boolean OpenDb(string dbPath)
{
try
{
string sqliteConnString = "Data Source=" + dbPath;
this._SQLiteConn = new SQLiteConnection(sqliteConnString);
this._dbName = dbPath;
this._SQLiteConnString = sqliteConnString;
this._SQLiteConn.Open();
return true;
}
catch (Exception ex)
{
throw new Exception("打开数据库:" + dbPath + "的连接失败:" + ex.Message);
}
}
///
/// 关闭数据库连接
///
public void CloseDb()
{
if (this._SQLiteConn != null && this._SQLiteConn.State != ConnectionState.Closed)
{
if (this._IsRunTrans && this._AutoCommit)
{
this.Commit();
}
this._SQLiteConn.Close();
this._SQLiteConn = null;
}
}
///
/// 开始数据库事务
///
public void BeginTransaction()
{
this._SQLiteConn.BeginTransaction();
this._IsRunTrans = true;
}
///
/// 开始数据库事务
///
/// 事务锁级别
public void BeginTransaction(IsolationLevel isoLevel)
{
this._SQLiteConn.BeginTransaction(isoLevel);
this._IsRunTrans = true;
}
///
/// 提交当前挂起的事务
///
public void Commit()
{
if (this._IsRunTrans)
{
this._SQLiteTrans.Commit();
this._IsRunTrans = false;
}
}
}
}
namespace XSface
{
public partial class testDB : System.Web.UI.Page
{
private static string dbPath = @"d:\face.db";
protected void Page_Load(object sender, EventArgs e){}
protected void button_Click(object sender, EventArgs e)
{
//string sConn = "Data Source=198.168.1.162;Initial Catalog=XSface;Integrated Security=True;User Id = sa;Password = xiangsheng;";
//using (SQLiteConnection cn = new SQLiteConnection("Data Source=D://test.db;Pooling=true;FailIfMissing=false"))
//string sConn = ConfigurationManager.AppSettings["ConnectionString"].ToString();
string sConn = "Data Source=198.168.1.162;Initial Catalog=XSface;Integrated Security=True;User Id = sa;Password = xiangsheng;";
string sConn2 = "Data Source=D://test3.db;Pooling=true;FailIfMissing=false;Initial Catalog=XSface;Integrated Security=True;User Id = sa;Password = xiangsheng;";
int i = 0;
SqlConnection objConn = new SqlConnection(sConn);
objConn.Open();
string sql = "select * from faceid";
SqlCommand cmd = new SqlCommand(sql, objConn);
SqlDataReader dr = cmd.ExecuteReader();
//创建一个数据库db文件
CSQLiteHelper.NewDbFile(dbPath);
//创建一个表
//string tableName = "Stars";
string tableName = "faceid";
CSQLiteHelper.NewTable(dbPath, tableName);
while (dr.Read())
{
if ( i < 2001 )
{
string name = dr["name"].ToString();
byte[] image = (byte[])dr["image"];
byte[] feature = (byte[])dr["feature"];
AddStars(name,image,feature,tableName);
i++;
}
if (i == 2001) {
break;
}
}
Response.Write("导入成功");
}
private void AddStars(string name,byte[] image,byte[] feature,string tableName)
{
SQLiteConnection sqliteConn = new SQLiteConnection("data source=" + dbPath);
//string commandStr = "insert into Stars(Name,Team,Number) values(@name,@team,@number)";
string commandStr = "insert into " + tableName + "(name,image,feature) values(@name,@image,@feature)";
if (sqliteConn.State != System.Data.ConnectionState.Open)
{
sqliteConn.Open();
SQLiteCommand cmd = new SQLiteCommand();
cmd.Connection = sqliteConn;
cmd.CommandText = commandStr;
cmd.CommandType = CommandType.Text;
cmd.Parameters.Add("@name", DbType.String);
cmd.Parameters["@name"].Value = name;
cmd.Parameters.Add("@image", DbType.Object);
cmd.Parameters["@image"].Value = image;
cmd.Parameters.Add("@feature", DbType.Object);
cmd.Parameters["@feature"].Value = feature;
cmd.ExecuteNonQuery();
cmd.Dispose();
sqliteConn.Close();
}
sqliteConn.Close();
}
}
}
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="testDB.aspx.cs" Inherits="XSface.testDB" %>
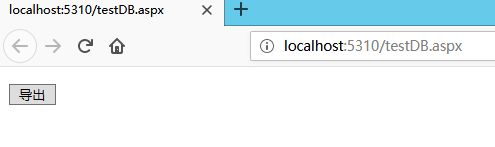
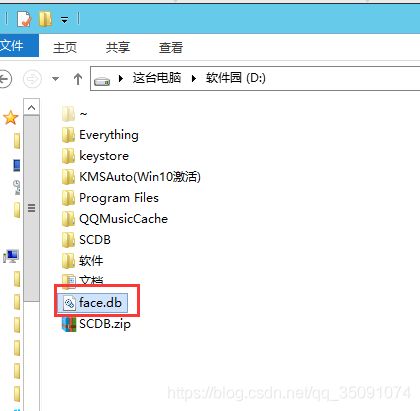
android
package com.xiangsheng.xsface.sql;
import android.content.Context;
import android.content.res.AssetManager;
import android.database.sqlite.SQLiteDatabase;
import android.util.Log;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* Created by Administrator on 2019/3/13.
*/
public class SQLdm
{
//数据库存储路径
// String filePath = "data/data/com.datab.cn/face.db";
String filePath = "data/data/com.xiangsheng.xsface/face.db";
//数据库存放的文件夹 data/data/com.main.jh 下面
// String pathStr = "data/data/com.datab.cn";
String pathStr = "data/data/com.xiangsheng.xsface";
SQLiteDatabase database;
public SQLiteDatabase openDatabase(Context context)
{
System.out.println("filePath:"+filePath);
File jhPath=new File(filePath);
//查看数据库文件是否存在
if(jhPath.exists())
{
Log.i("test", "存在数据库");
//存在则直接返回打开的数据库
return SQLiteDatabase.openOrCreateDatabase(jhPath, null);
}else{
//不存在先创建文件夹
File path=new File(pathStr);
Log.i("test", "pathStr="+path);
if (path.mkdir()){
Log.i("test", "创建成功");
}else{
Log.i("test", "创建失败");
};
try {
//得到资源
AssetManager am= context.getAssets();
//得到数据库的输入流
InputStream is=am.open("face.db");
Log.i("test", is+"");
//用输出流写到SDcard上面
FileOutputStream fos=new FileOutputStream(jhPath);
Log.i("test", "fos="+fos);
Log.i("test", "jhPath="+jhPath);
//创建byte数组 用于1KB写一次
byte[] buffer=new byte[1024];
int count = 0;
while((count = is.read(buffer))>0)
{
// Log.i("test", "得到");
fos.write(buffer,0,count);
}
//最后关闭就可以了
fos.flush();
fos.close();
is.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return null;
}
//如果没有这个数据库 我们已经把他写到SD卡上了,然后在执行一次这个方法 就可以返回数据库了
return openDatabase(context);
}
}
}
if (mResgist.size() == 0)
{
//******//
// 打开数据库输出流
SQLdm s = new SQLdm();
SQLiteDatabase db = s.openDatabase(getApplicationContext());
//查询数据库中testid=1的数据
// Cursor cursor = db.rawQuery("select * from faceid where _id=?", new String[]{editText.getText().toString()});
cursor = db.rawQuery("select * from faceid", null);
String name = null;
//cursor : 光标
byte[] imagebyte = null;
byte[] featurebyte = null;
int i = 1;
final AFR_FSDKFace face = new AFR_FSDKFace();
Bitmap imagebitmap = null;
if (cursor != null)
{
while (cursor.moveToNext())
{
name = cursor.getString(cursor.getColumnIndex("name"));
imagebyte = cursor.getBlob(cursor.getColumnIndex("image"));
featurebyte = cursor.getBlob(cursor.getColumnIndex("feature"));
face.setFeatureData(featurebyte);
imagebitmap = BitmapFactory.decodeByteArray(imagebyte, 0, imagebyte.length);
((Application) RecordActivity.this.getApplicationContext()).mFaceDB.addFace(name, face, imagebitmap);
}
// 在进度条走完时删除Dialog
// mProgressDialog.dismiss();
}
db.close();
cursor.close();
}
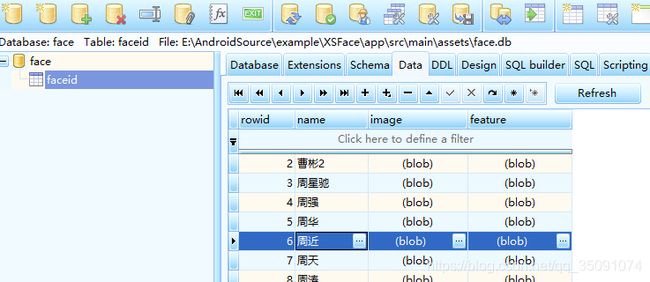