支付宝捐赠
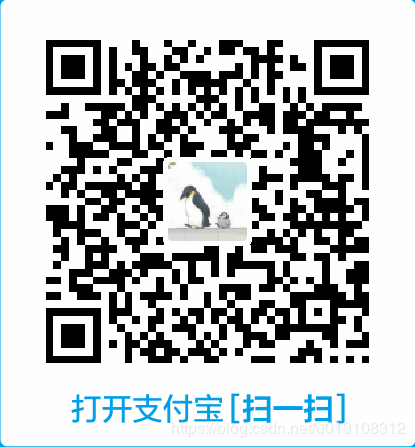
- 注释很详细,具体使用方法看代码注释
var timerid = TimerHeap.AddTimer(0, 500, () =>
{
});
TimerHeap.AddTimer(1000, 0, () =>
{
TimerHeap.DelTimer(timerid);
});
TimerHeap.AddTimer(2000, 0, () =>
{
Debug.LogError("Hello World");
});
void Awake()
{
InvokeRepeating("Tick", 1, 0.02f);
}
void Tick()
{
TimerHeap.Tick();
FrameTimerHeap.Tick();
}
- TimerData.cs
internal abstract class AbsTimerData
{
private uint m_nTimerId;
public uint NTimerId
{
get { return m_nTimerId; }
set { m_nTimerId = value; }
}
private int m_nInterval;
public int NInterval
{
get { return m_nInterval; }
set { m_nInterval = value; }
}
private ulong m_unNextTick;
public ulong UnNextTick
{
get { return m_unNextTick; }
set { m_unNextTick = value; }
}
public abstract Delegate Action
{
get;
set;
}
public abstract void DoAction();
}
internal class TimerData : AbsTimerData
{
private Action m_action;
public override Delegate Action
{
get { return m_action; }
set { m_action = value as Action; }
}
public override void DoAction()
{
m_action();
}
}
internal class TimerData<T> : AbsTimerData
{
private Action<T> m_action;
public override Delegate Action
{
get { return m_action; }
set { m_action = value as Action<T>; }
}
private T m_arg1;
public T Arg1
{
get { return m_arg1; }
set { m_arg1 = value; }
}
public override void DoAction()
{
m_action(m_arg1);
}
}
internal class TimerData<T, U> : AbsTimerData
{
private Action<T, U> m_action;
public override Delegate Action
{
get { return m_action; }
set { m_action = value as Action<T, U>; }
}
private T m_arg1;
public T Arg1
{
get { return m_arg1; }
set { m_arg1 = value; }
}
private U m_arg2;
public U Arg2
{
get { return m_arg2; }
set { m_arg2 = value; }
}
public override void DoAction()
{
m_action(m_arg1, m_arg2);
}
}
internal class TimerData<T, U, V> : AbsTimerData
{
private Action<T, U, V> m_action;
public override Delegate Action
{
get { return m_action; }
set { m_action = value as Action<T, U, V>; }
}
private T m_arg1;
public T Arg1
{
get { return m_arg1; }
set { m_arg1 = value; }
}
private U m_arg2;
public U Arg2
{
get { return m_arg2; }
set { m_arg2 = value; }
}
private V m_arg3;
public V Arg3
{
get { return m_arg3; }
set { m_arg3 = value; }
}
public override void DoAction()
{
m_action(m_arg1, m_arg2, m_arg3);
}
}
- TimerHeap.cs
public class TimerHeap
{
private static uint m_nNextTimerId;
private static uint m_unTick;
private static KeyedPriorityQueue<uint, AbsTimerData, ulong> m_queue;
private static Stopwatch m_stopWatch;
private static readonly object m_queueLock = new object();
private TimerHeap() { }
static TimerHeap()
{
m_queue = new KeyedPriorityQueue<uint, AbsTimerData, ulong>();
m_stopWatch = new Stopwatch();
}
public static uint AddTimer(uint start, int interval, Action handler)
{
var p = GetTimerData(new TimerData(), start, interval);
p.Action = handler;
return AddTimer(p);
}
public static uint AddTimer<T>(uint start, int interval, Action<T> handler, T arg1)
{
var p = GetTimerData(new TimerData<T>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
return AddTimer(p);
}
public static uint AddTimer<T, U>(uint start, int interval, Action<T, U> handler, T arg1, U arg2)
{
var p = GetTimerData(new TimerData<T, U>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
p.Arg2 = arg2;
return AddTimer(p);
}
public static uint AddTimer<T, U, V>(uint start, int interval, Action<T, U, V> handler, T arg1, U arg2, V arg3)
{
var p = GetTimerData(new TimerData<T, U, V>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
p.Arg2 = arg2;
p.Arg3 = arg3;
return AddTimer(p);
}
public static void DelTimer(uint timerId)
{
lock (m_queueLock)
m_queue.Remove(timerId);
}
public static void Tick()
{
m_unTick += (uint)m_stopWatch.ElapsedMilliseconds;
m_stopWatch.Reset();
m_stopWatch.Start();
while (m_queue.Count != 0)
{
AbsTimerData p;
lock (m_queueLock)
p = m_queue.Peek();
if (m_unTick < p.UnNextTick)
{
break;
}
lock (m_queueLock)
m_queue.Dequeue();
if (p.NInterval > 0)
{
p.UnNextTick += (ulong)p.NInterval;
lock (m_queueLock)
m_queue.Enqueue(p.NTimerId, p, p.UnNextTick);
p.DoAction();
}
else
{
p.DoAction();
}
}
}
public static void Reset()
{
m_unTick = 0;
m_nNextTimerId = 0;
lock (m_queueLock)
while (m_queue.Count != 0)
m_queue.Dequeue();
}
private static uint AddTimer(AbsTimerData p)
{
lock (m_queueLock)
m_queue.Enqueue(p.NTimerId, p, p.UnNextTick);
return p.NTimerId;
}
private static T GetTimerData<T>(T p, uint start, int interval) where T : AbsTimerData
{
p.NInterval = interval;
p.NTimerId = ++m_nNextTimerId;
p.UnNextTick = m_unTick + 1 + start;
return p;
}
}
- FrameTimerHeap.cs
public class FrameTimerHeap
{
private static uint m_nNextTimerId;
private static uint m_unTick;
private static KeyedPriorityQueue<uint, AbsTimerData, ulong> m_queue;
private static readonly object m_queueLock = new object();
private FrameTimerHeap() { }
static FrameTimerHeap()
{
m_queue = new KeyedPriorityQueue<uint, AbsTimerData, ulong>();
}
public static uint AddTimer(uint start, int interval, Action handler)
{
var p = GetTimerData(new TimerData(), start, interval);
p.Action = handler;
return AddTimer(p);
}
public static uint AddTimer<T>(uint start, int interval, Action<T> handler, T arg1)
{
var p = GetTimerData(new TimerData<T>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
return AddTimer(p);
}
public static uint AddTimer<T, U>(uint start, int interval, Action<T, U> handler, T arg1, U arg2)
{
var p = GetTimerData(new TimerData<T, U>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
p.Arg2 = arg2;
return AddTimer(p);
}
public static uint AddTimer<T, U, V>(uint start, int interval, Action<T, U, V> handler, T arg1, U arg2, V arg3)
{
var p = GetTimerData(new TimerData<T, U, V>(), start, interval);
p.Action = handler;
p.Arg1 = arg1;
p.Arg2 = arg2;
p.Arg3 = arg3;
return AddTimer(p);
}
public static void DelTimer(uint timerId)
{
lock (m_queueLock)
m_queue.Remove(timerId);
}
public static void Tick()
{
m_unTick += (uint)(1000 * Time.deltaTime);
while (m_queue.Count != 0)
{
AbsTimerData p;
lock (m_queueLock)
p = m_queue.Peek();
if (m_unTick < p.UnNextTick)
{
break;
}
lock (m_queueLock)
m_queue.Dequeue();
if (p.NInterval > 0)
{
p.UnNextTick += (ulong)p.NInterval;
lock (m_queueLock)
m_queue.Enqueue(p.NTimerId, p, p.UnNextTick);
p.DoAction();
}
else
{
p.DoAction();
}
}
}
public static void Reset()
{
m_unTick = 0;
m_nNextTimerId = 0;
lock (m_queueLock)
while (m_queue.Count != 0)
m_queue.Dequeue();
}
private static uint AddTimer(AbsTimerData p)
{
lock (m_queueLock)
m_queue.Enqueue(p.NTimerId, p, p.UnNextTick);
return p.NTimerId;
}
private static T GetTimerData<T>(T p, uint start, int interval) where T : AbsTimerData
{
p.NInterval = interval;
p.NTimerId = ++m_nNextTimerId;
p.UnNextTick = m_unTick + 1 + start;
return p;
}
}
支付宝捐赠
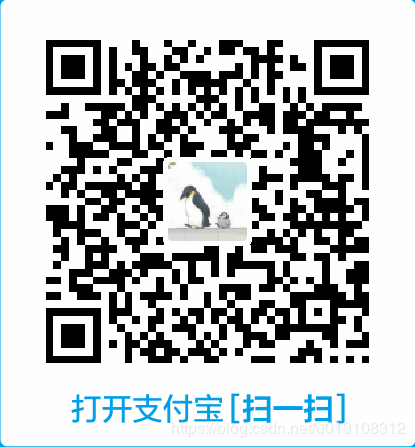