#include
#include
#include
#include
#define PI 3.14159265358979 / 180
#define PI_ 3.14159265358979
using namespace std;
using namespace cv;
Mat img(Size(1000, 1000), CV_8UC3, Scalar(0, 0, 0));
void cantor(Point p1, Point p2);
void Koch(Point p1, Point p2, int c);
void Ramus(Point p, double alpha, double L, int n);
void tree(int x, int y, double L, double alpha);
int main()
{
Point p1(0,500);
Point p2(500, 500);
Point p(500, 1000);
//cantor(p1, p2);
//Koch(p1, p2, 100);
//Ramus(p, 45, 250, 16);
tree(p.x, p.y, 240, 270);
imshow("图片", img);
imwrite(to_string(clock())+".png", img);
waitKey();
return 0;
}
void cantor(Point p1, Point p2) {
Point p3, p4;
int d = 30;
if ((p2.x - p1.x) <1) {
line(img, p1, p2, Scalar(255, 255, 255), 1, 8, 0);
}
else {
line(img, p1, p2, Scalar(255, 255, 255), 1, 8, 0);
p3.x = p1.x + (p2.x - p1.x) / 3;
p3.y = p1.y + d;
p4.x = p2.x - (p2.x - p1.x) / 3;
p4.y = p2.y + d;
p1.y += d;
p2.y += d;
cantor(p1, p3);
cantor(p4, p2);
}
}
void Ramus(Point p, double alpha, double L, int n) {
Point t;
double alpha_L, alpha_R;
if (n > 0) {
t.x = p.x + cos(alpha)*L;
t.y = p.y - sin(alpha)*L;
line(img, p, t, Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
alpha_L = alpha + PI_ / 8;
alpha_R = alpha - PI_ / 8;
L = 2 * L / 3;
Ramus(t, alpha_L, L, n - 1);
Ramus(t, alpha_R, L, n - 1);
}
}
void tree(int x, int y, double L, double A) {
double B, C, s1, s2, s3;
B = 50;
C = 9;
s1 = 2;
s2 = 3;
s3 = 1.3;
int x1, y1, x1L, y1L, x1R, y1R, x2, y2, x2R, y2R, x2L, y2L;
if (L > 2) {
x2 = int(x + L * cos(A * PI));
y2 = int(y + L * sin(A * PI));
x2R = int(x2 + L / s2 * cos((A + B) * PI));
y2R = int(y2 + L / s2 * sin((A + B) * PI));
x2L = int(x2 + L / s2 * cos((A - B) * PI));
y2L = int(y2 + L / s2 * sin((A - B) * PI));
x1 = int(x + L / s2 * cos(A * PI));
y1 = int(y + L / s2 * sin(A * PI));
x1L = int(x1 + L / s2 * cos((A - B) * PI));
y1L = int(y1 + L / s2 * sin((A - B) * PI));
x1R = int(x1 + L / s2 * cos((A + B) * PI));
y1R = int(y1 + L / s2 * sin((A + B) * PI));
line(img, Point(x,y), Point(x2,y2), Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
line(img, Point(x2, y2), Point(x2L, y2L), Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
line(img, Point(x2, y2), Point(x2R, y2R), Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
line(img, Point(x1, y1), Point(x1R, y1R), Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
line(img, Point(x1, y1), Point(x1L, y1L), Scalar(rand() % 255, rand() % 255, rand() % 255), 1, 8, 0);
tree(int(x2), int(y2), int(L / s3), A + C);
tree(int(x2R), int(y2R), int(L / s2), A + B);
tree(int(x2L), int(y2L), int(L / s2), A - B);
tree(int(x1L), int(y1L), int(L / s2), A - B);
tree(int(x1R), int(y1R), int(L / s2), A + B);
}
}
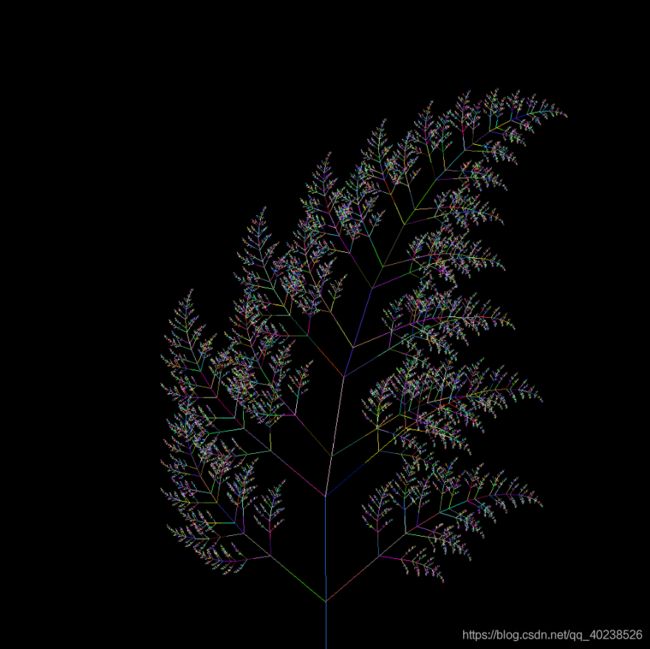