一、异常
1.异常的捕获和返回
"""
try:
尝试执行的代码
except:
出现错误的处理
"""
try:
num = int(input('请输入一个数字: '))
except:
print('请输入正确的整数')
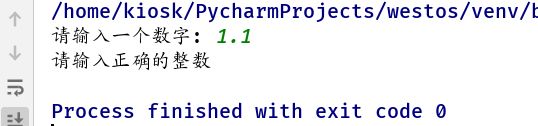
"""
举个简单的例子
题目要求:
1.提示用户输入一个整数
2.使用8来除以用户输入的数
try:
尝试执行的代码
except 错误类型1:
出现错误1的处理方式
except 错误类型2:
出现错误2的处理方式
finally
不管有没有错误都执行
"""
try:
num = int(input('请输入一个整数: '))
result = 8 / num
print(result)
except ValueError:
print('输入的值不是合法的整数')
except ZeroDivisionError:
print('0不能做除数')
except Exception as r:
print('未知错误 %s' %(r))
finally:
print('~~~~~~~~~~~~')
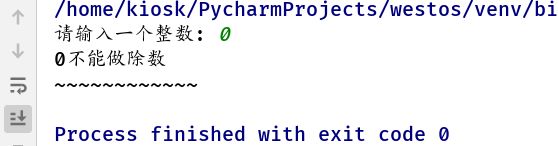
2.主动抛出异常
"""
需求: 提示用户输入密码,如果长度小于8位就抛出异常,符合要求就输出密码
"""
def input_passwd():
pwd = input('请输入密码: ')
if len(pwd) >= 8:
return pwd
print('主动抛出异常')
ex = Exception('密码长度不足!')
raise ex
try:
print(input_passwd())
except Exception as r:
print(r)
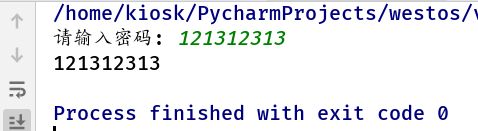
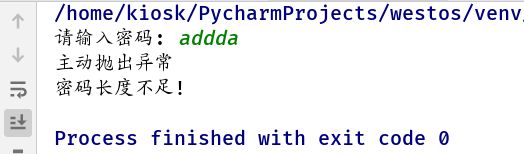
二、paramiko远程连接
1.paramiko远程密码连接
"""
基于ssh用于连接远程服务器做操作:
远程执行命令
上传/下载文件
"""
import paramiko
client = paramiko.SSHClient()
"""
The authenticity of host '172.25.254.221 (172.25.254.221)' can't be established.
ECDSA key fingerprint is 4c:98:51:43:65:46:66:fd:af:5b:ea:cc:d9:97:c0:74.
Are you sure you want to continue connecting (yes/no)?
"""
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(
hostname='172.25.254.221',
username='root',
password='redhat'
)
stdin,stdout,stderr = client.exec_command('route -n')
print(stdout.read().decode('utf-8'))
client.close()

2.批量连接主机
from paramiko.ssh_exception import NoValidConnectionsError,AuthenticationException
def connect(cmd,hostname,user,password):
import paramiko
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
client.connect(
hostname=hostname,
username=user,
password=password
)
stdin, stdout, stderr = client.exec_command(cmd)
a = stdout.read().decode('utf-8')
print(stdout.read().decode('utf-8'))
return a
except NoValidConnectionsError as e:
return '主机%s连接失败' %(hostname)
except AuthenticationException as e:
return '主机%s密码错误' %(hostname)
except Exception as e:
return '未知错误:',e
finally:
client.close()
with open('/home/kiosk/passwd') as f:
for line in f:
hostname,username,password = line.strip().split(':')
res = connect('hostname',hostname,username,password)
print('主机名:', res)
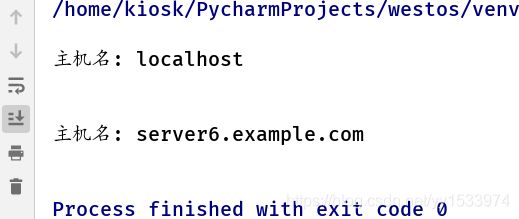
3.文件的上传和下载
'''给远程主机上传一个文件'''
from paramiko.ssh_exception import NoValidConnectionsError,AuthenticationException
import paramiko
def put(hostname,password,local_name,remote_name):
try:
transport = paramiko.Transport((hostname,22))
transport.connect(username='root',password=password)
sftp = paramiko.SFTPClient.from_transport(transport)
except AuthenticationException as e:
return '主机%s密码错误' %(hostname)
except Exception as e:
return '未知错误: ',e
else:
sftp.put(local_name,remote_name)
try:
sftp.file(remote_name)
print("上传成功.")
except IOError:
print("上传失败!")
finally:
transport.close()
put('172.25.254.221','redhat','/home/kiosk/passwd','/mnt/test')

'''从远程主机下载文件'''
from paramiko.ssh_exception import NoValidConnectionsError,AuthenticationException
import paramiko
import os
def get(hostname,password,remote_name,local_name):
try:
transport = paramiko.Transport((hostname,22))
transport.connect(username='root',password=password)
sftp = paramiko.SFTPClient.from_transport(transport)
except AuthenticationException as e:
return '主机%s密码错误' %(hostname)
except Exception as e:
return '未知错误: ',e
else:
sftp.get(remote_name,local_name)
if os.path.exists(local_name):
print('下载成功.')
else:
print('下载失败')
finally:
transport.close()
get('172.25.254.221','redhat','/mnt/test','/home/kiosk/test111.txt')

'''
sftp是安全文件传输协议,提供一种安全的加密方法,
sftp是SSH的一部分,SFTPClient类实现了sftp客户端,通过已建立的SSH通道传输文件,与其他的操作,常用的命令如下:
'''
sftp.close() |
关闭sftp |
sftp.file(filename, mode=‘r’, bufsize=-1) |
读取文件 |
sftp.from_transport(s) |
创建客户端通道 |
sftp.open(filename, mode=‘r’, bufsize=-1) |
在远程服务器打开文件 |
sftp.put(localpath, remotepath, callback=None) |
localpath文件上传到远程服务器remotepath |
sftp.get(remotepath, localpath, callback=None) |
从远程服务器remotepath拉文件到本地localpath |
sftp.remove(path) |
删除文件 |