- 10 个你项目里可能漏掉的 TypeScript 技巧
JaysonJin
TypeScripttypescriptjavascript前端
10个你项目里可能漏掉的TypeScript技巧点赞收藏关注不迷路!你是不是经常在用TypeScript,却总感觉“还差点火候”?其实,TS的威力并不只在于类型检查,更在于那些你可能漏掉的小技巧:它们不复杂,却能显著提升代码的安全性、可读性、工程效率!本文为你整理了10个容易被忽略、但非常实用的TypeScript技巧,看完一定会让你的项目更“TypeSafety”。1.类型别名+联合类型,替代硬
- 【DC综合 】简单的dc综合脚本
oahrzvq
学习
目录检查脚本是否有语法错误启动dc退出dc重新加载脚本文件打开dc帮助手册辅助设置路径和库文件设置读入设计文件保存映射前的ddc文件时序约束编译结果检查和保存输出文件/报告在开始之前,我们首先来了解一下提升dc使用感受的几个小技巧。检查脚本是否有语法错误dcprocheck./run.tcl启动dc使用dc的批处理模式启动dc,告诉dc执行哪个脚本文件,并告诉dc把启动过程中显示在终端的信息,也就
- 力扣刷题笔记 贪心篇
INlinKC
leetcode
总结先放在前面:贪心的本质是选择每一阶段的局部最优,从而达到全局最优。解答贪心时的一些小技巧与注意点:1.贪心问题在需要多维度进行考虑时,可以先从某一个维度开始贪心,然后再从另一个,比如NO.135.分发糖果中,既需要考虑左边孩子的糖果数又需要考虑右边孩子的糖果数,我们先从左往右保证右边一定大于左边,再从右往左保证左边一定大于右边(注意次数需要对比一下两次遍历中糖果数可能会不同,不同时需要去较大的
- CSS知识复习2
Savior`L
前端css前端
文章目录盒子模型CSS长度单位元素的显示模式修改元素的显示模式盒子模型的组成关于默认高度盒子内边距(padding)盒子边框(border)盒子外边距(margin)注意事项margin塌陷margin合并处理内容溢出隐藏元素方式样式继承默认样式布局小技巧浮动浮动特点浮动影响以及解决方法浮动相关属性定位相对定位绝对定位固定定位粘性定位定位层级布局版心常用布局名词重置默认样式盒子模型CSS长度单位p
- 每天一道大厂SQL题【Day25】脉脉真题实战(一)每日活跃用户_用户每日登陆脉脉会访问app不同的模块,现有两个表 表1记录了每日脉脉活跃用户的ui(1)
文章目录每天一道大厂SQL题【Day25】脉脉真题实战(一)每日活跃用户每日语录第25题:1.需求列表1.初级题:每日活跃用户思路分析(1)创建表(2)思路答案获取加技术群讨论附表文末SQL小技巧后记每天一道大厂SQL题【Day25】脉脉真题实战(一)每日活跃用户大家好,我是Maynor。相信大家和我一样,都有一个大厂梦,作为一名资深大数据选手,深知SQL重要性,接下来我准备用100天时间,基于大
- FPGA设计中的数据存储
cycf
FPGA之道fpga开发
文章目录FPGA设计中的数据存储为什么需要数据存储FPGA芯片内部的载体触发器查找表块存储FPGA芯片外部的资源RAM应用场合ROM特征简介实现载体应用场合FIFO特征简介FIFO使用小技巧之冗余法FIFO写接口缓存FIFO读接口缓存“冗余法”总结根据数据流的稳定性与存储操作的容错性,决定采用RAM模式还是FIFO模式STACK特征简介实现载体应用场合SummaryFPGA设计中的数据存储为什么需
- WHAT - React Native 中 Light and Dark mode 深色模式(黑暗模式)机制
文章目录一、Light/DarkMode的原理1.操作系统层2.ReactNative如何获取?3.样式怎么跟着变?二、关键代码示例讲解代码讲解:三、自定义主题四、运行时自动更新五、核心原理一张图组件应用例子最小示例:动态样式按钮的动态样式如何封装一套自定义主题四、如何和ThemeProvider配合?小技巧总结总结一句话这其实是现代移动应用开发中非常常用的功能:自动适配浅色/深色模式(Light
- 应届生实习转正工作述职小技巧
gjh1208
经验总结giteejava
实习转正工作述职小技巧前言:在准备转正汇报时我发现,尽管日常工作中我频繁参与需求编写与大量bug的修复,但这些努力在向上级汇报时难以有效体现。而领导更倾向于通过直观成果来评估工作表现。git命令体现直接在对应的文件进入git输入以下命令,author换成自己就行了gitlog--author="gjh"--numstat--pretty=tformat:--no-merges|awk'{add+=
- Word转LaTeX排版6大技巧
加油吧zkf
目标跟踪计算机视觉目标检测机器学习人工智能python
Word内容快速排版到TeX格式的技巧分享(含多种实用方法)在科研论文、技术报告或毕业论文写作中,很多同学喜欢先用Word写初稿,再迁移到LaTeX(.tex文件)进行排版。但迁移过程中常常遇到这些麻烦:Word中的公式复制过去乱码或无法编译排版格式对不上期刊/会议模板自己新写的tex文件总是出错,编译困难今天分享我在实际论文写作中总结出的几条小技巧,帮大家快速把Word内容排到LaTeX,而且能
- 【EXCEL_VBA_基础知识】02 使用IF进行逻辑判断
南工说焊接
软件应用excel
课程来源:王佩丰老师的《王佩丰学VBA视频教程》,如有侵权,请联系删除!相信大家在平时使用EXCEL时,经常会使用到IF函数进行判断。那么,IF函数在VBA是怎么写呢?让我们跟随王佩丰老师课程,一起一探究竟!另外,从王佩丰老师的课程中,还掌握了一个小技巧:当我们不知道一段代码如何编写时,可以通过录制宏的方式来查看功能实现的代码!目录1.0小知识点1.0.1Range("单元格或区域位置"):代表取
- 从 Vue 到 React:React.memo + useCallback 组合技
目录一、Vue与React的组件更新机制对比二、React.memo是什么?三、常见坑:为什么我用了React.memo还是会重新渲染?四、解决方案:useMemo/useCallback缓存引用五、Vue3中有类似的性能控制需求吗?六、组合优化小技巧总结七、不过话又说回来一、Vue与React的组件更新机制对比在Vue中,组件的更新依赖于响应式系统的依赖追踪:父组件更新时,Vue会判断data是
- 嵌入式视频编解码入门保姆级教程
大模型大数据攻城狮
视频编解码cocos2d游戏引擎b帧p帧H.264h.265
目录章节1:视频编解码的“榨汁机”是怎么回事?1.1为什么需要视频编解码?1.2视频编解码的核心原理1.3嵌入式设备上的挑战章节2:认识视频数据的基本“零件”2.1像素、帧和分辨率2.2帧的类型:I、P、B帧2.3码流和容器章节3:H.264编解码的“魔法书”3.1H.264的核心技术3.2嵌入式设备上的H.2643.3动手实践:用FFmpeg编码H.264视频3.4调试小技巧章节4:帧间预测与运
- 下完安装好python后,想查看python的安装位置的几种方法
大模型猫叔
python开发语言爬虫
查看python的路径基于windows系统,按下win+r(也就是命令提示符),输入cmd,进入查看当前的python的版本的话输入python-V1,查看当前下载的python类型和路径则可以输入py-0(加*的是你使用python的默认版本)2,还可以使用命令wherepython查看路径(这样就不会显示你python默认使用的是哪个)小技巧:如果想清除命令行的话可以输入命令cls然后ent
- 10个可以快速用Python进行数据分析的小技巧_python 通径分析
2401_86043917
python数据分析开发语言
df.iplot()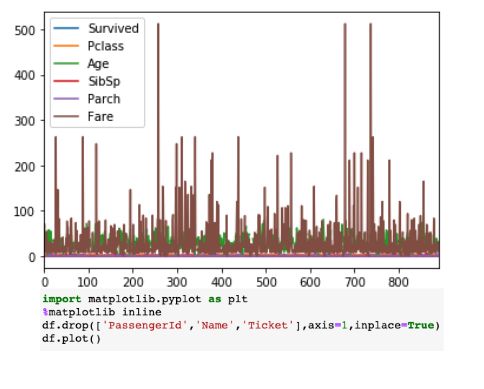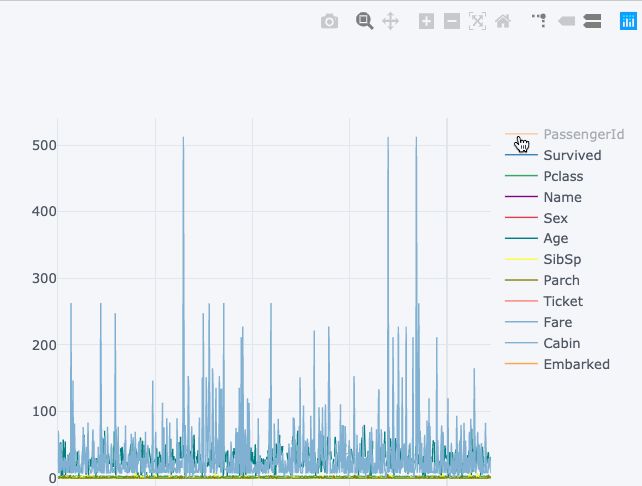df.iplot()vsdf.plot()右侧的可视
- Swift 小技巧:用单边区间优雅处理模糊范围
狼_夏天
SwiftTipsswift开发语言ios
进入正题之前先科普一下Swift区间的知识。Swift中的区间有两种类型:闭区间和半开区间。闭区间:用a...b表示,包含a和b。半开区间:用a..=0&&number=2.0&&score=3.5&&score<=5.0{print("好评")}else{print("评分超出范围")}3.用单边区间优雅处理letscore=4.2guard(0.0...5.0).contains(score)
- 电商数据分析--常见的数据采集工具及方法
2501_91048859
python爬虫数据采集AI爬虫
大家好,我是老张,一个在IT圈子里摸爬滚打了十几年的老程序员。今天我想和大家分享一下我在电商数据分析领域的一些实操经验,特别是关于数据采集工具和方法的使用心得。首先,让我们聊聊数据采集的重要性。在电商领域,数据就是金矿,而采集工具就是我们的挖掘机。没有好的工具,再丰富的矿藏也难以开采。今天,我主要想介绍几种我常用的数据采集工具,并分享一些实操中的小技巧。###1.火车采集器火车采集器是我早期使用的
- GitHub 使用小技巧
千空
搜索技巧信息检索
GitHub现在成了程序员最流行的社交网站,可能也是最大的代码托管仓库。然而除了代码托管外,GitHub还提供其它服务:代码仓库(https://github.com),代码片段(https://gist.github.com),短网址(https://git.io),主页(http://username.github.io),工作(https://jobs.github.com)。这里记录一下G
- C#工程中输出类型转换以及程序运行后控制台窗口不退出设置
nanke_yh
C#c#输出类型切换控制台窗口暂停
本想调试一个小的代码,无意间发现的两个C#工程中的小技巧点,在此记录一下。一、窗口不退出调试的代码主要是时间信息的转换与输出,为此新建了控制台应用工程,可以将调试信息打印出来。但执行后发现直接结束,控制台信息都没能看到就退出了。我们知道在C/C++中遇到这种情况一般是加上:getchar();或者system("pause");为了防止C#控制台窗口执行后闪退,需要在代码最后加上一句代码:Cons
- 鸿蒙5开发案例分享揭秘---一多开发实例(商务办公)
【鸿蒙开发宝藏案例大揭秘】原来官方文档里藏了这么多好东西!大家好呀~最近在肝鸿蒙项目时意外扒出了官方文档里的"藏宝库"!原来那些让人头秃的跨端适配难题,官方早就准备好了参考答案!今天就带大家挖一挖这些实战案例,手把手教你玩转"一次开发,多端部署"!(文末有惊喜小技巧哦~)一、商务办公应用案例(官方王炸模板)案例亮点:这个模板直接解决了三大致命痛点——侧边栏适配、分栏布局切换、多端卡片排列,连华为工
- OS X快捷键小技巧
weixin_30443075
退出command+Q,关分页Command+W,刷新Command+R,新开分页Command+T全屏ctrl+command+F每个Mac使用者都知道点击下窗口左上角黄色圆形的按钮就可以最小化窗口,但是这种方式不是很快捷,有快捷的方法吗?MacGG今天介绍两个:使用快捷键最小化窗口:每当你在Mac上要最小化窗口,你可以直接按下键盘上的Command+M就OK了。这是简单的,在给大家来个强大点的
- MAC使用小技巧
lwq421336220
本文转自http://www.macx.cn/thread-2050934-1-1.html1、前往文件夹在Finder下按+Shift+G,可以开启“前往文件夹”对话框,之后你可以输入路径来快速访问Finder目录位置,进行定位文件操作,这毫无疑问是最快的方法。另外你不必输入全部的路径,可以直接输入当前文件夹往下的路径就可以。另外在打开或保存对话框也可以使用这个快捷键。(什么是打开或保存对话框?
- 【excel入门学习】
秃顶少女
办公excel
excel学习excel学习快速填充/智能填充快速分析特殊格式的录入时间百分比分数身份证(过长数字的录入)原位填充从网上获取数据清洗数据自定义格式:数字格式代码数值占位符:0#?文本占位符:@组合设置0;-0;-;@日期格式设置:ymda颜色设置条件设置格式数据验证条件格式查找函数函数使用方法绝对引用与相对引用常用函数统计函数通配符求和排名vlookup函数查找替换分列一些小技巧自用!excel学
- FastStone Capture 除了截图还能干啥?压缩图片也是一把好手!
简鹿办公
软件教程faststonecapture
使用电脑或手机的过程中,我们经常需要上传图片,比如头像、证件照、产品图等。但有时候系统会提示“图片不能超过200KB”,这时候你可能会犯愁:明明拍得挺清楚的,怎么就超了呢?别担心!今天简鹿办公就来聊一个非常实用的小技巧——如何把图片压缩到200KB以下,而且操作简单,不需要专业软件,普通人也能轻松上手。为什么图片文件会很大?首先我们要明白,一张图片的大小主要取决于两个因素:图片的尺寸(像素):分辨
- iOS-开发小技巧
XR_Code
ios开发小技巧ios开发uitableviewuiview
在这里总结一些iOS开发中的小技巧,能大大方便我们的开发,持续更新。UITableView的Group样式下顶部空白处理//分组列表头部空白处理UIView*view=[[UIViewalloc]initWithFrame:CGRectMake(0,0,0,0.1)];self.tableView.tableHeaderView=view;获取某个view所在的控制器-(UIViewControl
- 5个Python冷门小技巧
JoySSL证书厂商
python开发语言
1.动态导入模块(__import__)#替代importlib的原始方法math=__import__("math")print(math.sqrt(4)) #输出2.0用途:动态字符串名称导入模块(如插件系统)。2.else子句在循环中foriinrange(5): ifi==3: breakelse: print("循环未被break中断") #不会执行用途:检测循环是否自然结束(
- Linux 命令:pwd 与 which 的简单用法
郝学胜@无限畅想大公司
Liunxlinux运维服务器
在Linux系统中,`pwd`和`which`是两个常用命令,但它们的用途截然不同。1.pwd:查看当前目录`pwd`(PrintWorkingDirectory)用于显示当前终端所在的路径。示例:$pwd /home/user/documents 场景:当你不确定当前在哪个目录时,`pwd`能快速帮你确认位置。小技巧:结合`cd`命令,可快速跳转到其他目录。2.which:查找命令路径`whic
- 解决maven项目打包时处理本地依赖包的问题
将本地引入的依赖包生成maven坐标后通过pom引入mvninstall将本地依赖包生成maven坐标由于之前一直是在基于osp环境下进行开发,没怎么使用过springboot,简直像个假的程序员///,所以正好趁最近有个小需求,练练springboot入门,包括基础的配置、打包、idea使用小技巧等等都会记录一下,免得自己脑子不好哪天又忘了~在进行开发时,如果遇到需要引入本地依赖包的情况,没有办
- 算法题练习小技巧之区间合并--套路详细讲解带例题和源码(Python,C++)
立志成为算法讲师
基础算法详解算法pythonc++开发语言
(本文源于最近刷题刷到区间合并的问题,第一次写极其不熟练,在这里总结并写一点相关套路)所谓区间合并,可以理解成一个n行两列的二维数组,每一行的两个数字表示一个区间的左右端点,现在需要对这些区间进行相关操作(根据题目情况来定),但是有些区间有重合部分,实际操作起来就很麻烦,此时可以将区间进行合并减少思维难度那么怎么进行合并呢?请看例题一。例题一:LCR074.合并区间-力扣(LeetCode)之后碰
- css 使用小技巧
1css图片与文字对齐方式需要把样式设置给图片同时适用于文本域1 居中对齐 vertical-align:middle;2 底线对齐 vertical-align:bottom;3顶部对齐 vertical-align:top;2.行内块元素在同一行会有缝隙解决只需要给当前行内块元素加一个一个浮动就能够解决这种问题3怎么查看元素的hoveractivefocusvisited等css样
- 让 CSS 布局不再头疼!10 个超实用的 CSS 一行代码技巧
瑆箫
css前端
你是否也经常被复杂的CSS布局搞得焦头烂额?别担心!今天就来分享一些简单却超实用的CSS一行代码技巧,让你轻松搞定布局,提升效率,还能让代码更优雅。1.带背景渐变的文字想要让标题更吸引眼球吗?试试给文字添加背景渐变!实现这个效果需要一个小技巧,因为通常情况下渐变属性不能直接应用于文字。我们可以把渐变作为背景,然后剪切它,只显示文字所在的区域。酷炫的渐变文字.gradient-text { bac
- java数字签名三种方式
知了ing
javajdk
以下3钟数字签名都是基于jdk7的
1,RSA
String password="test";
// 1.初始化密钥
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(51
- Hibernate学习笔记
caoyong
Hibernate
1>、Hibernate是数据访问层框架,是一个ORM(Object Relation Mapping)框架,作者为:Gavin King
2>、搭建Hibernate的开发环境
a>、添加jar包:
aa>、hibernatte开发包中/lib/required/所
- 设计模式之装饰器模式Decorator(结构型)
漂泊一剑客
Decorator
1. 概述
若你从事过面向对象开发,实现给一个类或对象增加行为,使用继承机制,这是所有面向对象语言的一个基本特性。如果已经存在的一个类缺少某些方法,或者须要给方法添加更多的功能(魅力),你也许会仅仅继承这个类来产生一个新类—这建立在额外的代码上。
- 读取磁盘文件txt,并输入String
一炮送你回车库
String
public static void main(String[] args) throws IOException {
String fileContent = readFileContent("d:/aaa.txt");
System.out.println(fileContent);
- js三级联动下拉框
3213213333332132
三级联动
//三级联动
省/直辖市<select id="province"></select>
市/省直辖<select id="city"></select>
县/区 <select id="area"></select>
- erlang之parse_transform编译选项的应用
616050468
parse_transform游戏服务器属性同步abstract_code
最近使用erlang重构了游戏服务器的所有代码,之前看过C++/lua写的服务器引擎代码,引擎实现了玩家属性自动同步给前端和增量更新玩家数据到数据库的功能,这也是现在很多游戏服务器的优化方向,在引擎层面去解决数据同步和数据持久化,数据发生变化了业务层不需要关心怎么去同步给前端。由于游戏过程中玩家每个业务中玩家数据更改的量其实是很少
- JAVA JSON的解析
darkranger
java
// {
// “Total”:“条数”,
// Code: 1,
//
// “PaymentItems”:[
// {
// “PaymentItemID”:”支款单ID”,
// “PaymentCode”:”支款单编号”,
// “PaymentTime”:”支款日期”,
// ”ContractNo”:”合同号”,
//
- POJ-1273-Drainage Ditches
aijuans
ACM_POJ
POJ-1273-Drainage Ditches
http://poj.org/problem?id=1273
基本的最大流,按LRJ的白书写的
#include<iostream>
#include<cstring>
#include<queue>
using namespace std;
#define INF 0x7fffffff
int ma
- 工作流Activiti5表的命名及含义
atongyeye
工作流Activiti
activiti5 - http://activiti.org/designer/update在线插件安装
activiti5一共23张表
Activiti的表都以ACT_开头。 第二部分是表示表的用途的两个字母标识。 用途也和服务的API对应。
ACT_RE_*: 'RE'表示repository。 这个前缀的表包含了流程定义和流程静态资源 (图片,规则,等等)。
A
- android的广播机制和广播的简单使用
百合不是茶
android广播机制广播的注册
Android广播机制简介 在Android中,有一些操作完成以后,会发送广播,比如说发出一条短信,或打出一个电话,如果某个程序接收了这个广播,就会做相应的处理。这个广播跟我们传统意义中的电台广播有些相似之处。之所以叫做广播,就是因为它只负责“说”而不管你“听不听”,也就是不管你接收方如何处理。另外,广播可以被不只一个应用程序所接收,当然也可能不被任何应
- Spring事务传播行为详解
bijian1013
javaspring事务传播行为
在service类前加上@Transactional,声明这个service所有方法需要事务管理。每一个业务方法开始时都会打开一个事务。
Spring默认情况下会对运行期例外(RunTimeException)进行事务回滚。这
- eidtplus operate
征客丶
eidtplus
开启列模式: Alt+C 鼠标选择 OR Alt+鼠标左键拖动
列模式替换或复制内容(多行):
右键-->格式-->填充所选内容-->选择相应操作
OR
Ctrl+Shift+V(复制多行数据,必须行数一致)
-------------------------------------------------------
- 【Kafka一】Kafka入门
bit1129
kafka
这篇文章来自Spark集成Kafka(http://bit1129.iteye.com/blog/2174765),这里把它单独取出来,作为Kafka的入门吧
下载Kafka
http://mirror.bit.edu.cn/apache/kafka/0.8.1.1/kafka_2.10-0.8.1.1.tgz
2.10表示Scala的版本,而0.8.1.1表示Kafka
- Spring 事务实现机制
BlueSkator
spring代理事务
Spring是以代理的方式实现对事务的管理。我们在Action中所使用的Service对象,其实是代理对象的实例,并不是我们所写的Service对象实例。既然是两个不同的对象,那为什么我们在Action中可以象使用Service对象一样的使用代理对象呢?为了说明问题,假设有个Service类叫AService,它的Spring事务代理类为AProxyService,AService实现了一个接口
- bootstrap源码学习与示例:bootstrap-dropdown(转帖)
BreakingBad
bootstrapdropdown
bootstrap-dropdown组件是个烂东西,我读后的整体感觉。
一个下拉开菜单的设计:
<ul class="nav pull-right">
<li id="fat-menu" class="dropdown">
- 读《研磨设计模式》-代码笔记-中介者模式-Mediator
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
/*
* 中介者模式(Mediator):用一个中介对象来封装一系列的对象交互。
* 中介者使各对象不需要显式地相互引用,从而使其耦合松散,而且可以独立地改变它们之间的交互。
*
* 在我看来,Mediator模式是把多个对象(
- 常用代码记录
chenjunt3
UIExcelJ#
1、单据设置某行或某字段不能修改
//i是行号,"cash"是字段名称
getBillCardPanelWrapper().getBillCardPanel().getBillModel().setCellEditable(i, "cash", false);
//取得单据表体所有项用以上语句做循环就能设置整行了
getBillC
- 搜索引擎与工作流引擎
comsci
算法工作搜索引擎网络应用
最近在公司做和搜索有关的工作,(只是简单的应用开源工具集成到自己的产品中)工作流系统的进一步设计暂时放在一边了,偶然看到谷歌的研究员吴军写的数学之美系列中的搜索引擎与图论这篇文章中的介绍,我发现这样一个关系(仅仅是猜想)
-----搜索引擎和流程引擎的基础--都是图论,至少像在我在JWFD中引擎算法中用到的是自定义的广度优先
- oracle Health Monitor
daizj
oracleHealth Monitor
About Health Monitor
Beginning with Release 11g, Oracle Database includes a framework called Health Monitor for running diagnostic checks on the database.
About Health Monitor Checks
Health M
- JSON字符串转换为对象
dieslrae
javajson
作为前言,首先是要吐槽一下公司的脑残编译部署方式,web和core分开部署本来没什么问题,但是这丫居然不把json的包作为基础包而作为web的包,导致了core端不能使用,而且我们的core是可以当web来用的(不要在意这些细节),所以在core中处理json串就是个问题.没办法,跟编译那帮人也扯不清楚,只有自己写json的解析了.
- C语言学习八结构体,综合应用,学生管理系统
dcj3sjt126com
C语言
实现功能的代码:
# include <stdio.h>
# include <malloc.h>
struct Student
{
int age;
float score;
char name[100];
};
int main(void)
{
int len;
struct Student * pArr;
int i,
- vagrant学习笔记
dcj3sjt126com
vagrant
想了解多主机是如何定义和使用的, 所以又学习了一遍vagrant
1. vagrant virtualbox 下载安装
https://www.vagrantup.com/downloads.html
https://www.virtualbox.org/wiki/Downloads
查看安装在命令行输入vagrant
2.
- 14.性能优化-优化-软件配置优化
frank1234
软件配置性能优化
1.Tomcat线程池
修改tomcat的server.xml文件:
<Connector port="8080" protocol="HTTP/1.1" connectionTimeout="20000" redirectPort="8443" maxThreads="1200" m
- 一个不错的shell 脚本教程 入门级
HarborChung
linuxshell
一个不错的shell 脚本教程 入门级
建立一个脚本 Linux中有好多中不同的shell,但是通常我们使用bash (bourne again shell) 进行shell编程,因为bash是免费的并且很容易使用。所以在本文中笔者所提供的脚本都是使用bash(但是在大多数情况下,这些脚本同样可以在 bash的大姐,bourne shell中运行)。 如同其他语言一样
- Spring4新特性——核心容器的其他改进
jinnianshilongnian
spring动态代理spring4依赖注入
Spring4新特性——泛型限定式依赖注入
Spring4新特性——核心容器的其他改进
Spring4新特性——Web开发的增强
Spring4新特性——集成Bean Validation 1.1(JSR-349)到SpringMVC
Spring4新特性——Groovy Bean定义DSL
Spring4新特性——更好的Java泛型操作API
Spring4新
- Linux设置tomcat开机启动
liuxingguome
tomcatlinux开机自启动
执行命令sudo gedit /etc/init.d/tomcat6
然后把以下英文部分复制过去。(注意第一句#!/bin/sh如果不写,就不是一个shell文件。然后将对应的jdk和tomcat换成你自己的目录就行了。
#!/bin/bash
#
# /etc/rc.d/init.d/tomcat
# init script for tomcat precesses
- 第13章 Ajax进阶(下)
onestopweb
Ajax
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/
- Troubleshooting Crystal Reports off BW
blueoxygen
BO
http://wiki.sdn.sap.com/wiki/display/BOBJ/Troubleshooting+Crystal+Reports+off+BW#TroubleshootingCrystalReportsoffBW-TracingBOE
Quite useful, especially this part:
SAP BW connectivity
For t
- Java开发熟手该当心的11个错误
tomcat_oracle
javajvm多线程单元测试
#1、不在属性文件或XML文件中外化配置属性。比如,没有把批处理使用的线程数设置成可在属性文件中配置。你的批处理程序无论在DEV环境中,还是UAT(用户验收
测试)环境中,都可以顺畅无阻地运行,但是一旦部署在PROD 上,把它作为多线程程序处理更大的数据集时,就会抛出IOException,原因可能是JDBC驱动版本不同,也可能是#2中讨论的问题。如果线程数目 可以在属性文件中配置,那么使它成为
- 正则表达式大全
yang852220741
html编程正则表达式
今天向大家分享正则表达式大全,它可以大提高你的工作效率
正则表达式也可以被当作是一门语言,当你学习一门新的编程语言的时候,他们是一个小的子语言。初看时觉得它没有任何的意义,但是很多时候,你不得不阅读一些教程,或文章来理解这些简单的描述模式。
一、校验数字的表达式
数字:^[0-9]*$
n位的数字:^\d{n}$
至少n位的数字:^\d{n,}$
m-n位的数字:^\d{m,n}$