参阅微信支付文档:https://pay.weixin.qq.com/wiki/doc/api/app/app.php?chapter=8_5
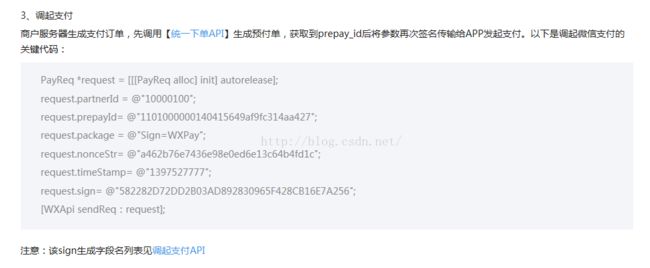
这一部分说的已经很详细了;
1.app将订单信息传给自己的后台服务;
2.后台服务接受到订单信息后,构建预支付信息,回传给APP;
接下来的过程是:
3.app获取预支付信息。调起微信支付,完成支付;
4.微信同步返回,接收通知给APP;异步调用我方后台服务提供的接口。返回成功或失败的处理结果;
贴一段别人实现的代码:
package com.epeit.api.service.wechatPay.wxpay;
import com.epeit.api.annotations.FunctionCode;
import com.epeit.api.dao.ShoppingOrderMapper;
import com.epeit.api.dao.TenpayParamsMapper;
import com.epeit.api.dao.UserAddressMapper;
import com.epeit.api.dao.WxpayInfoMapper;
import com.epeit.api.dto.ApiRequest;
import com.epeit.api.dto.ApiResponse;
import com.epeit.api.enums.RestResultEnum;
import com.epeit.api.model.ShoppingOrderPo;
import com.epeit.api.model.TenpayParams;
import com.epeit.api.model.UserAddressPo;
import com.epeit.api.model.WxpayInfo;
import com.epeit.api.service.inner.CommonService;
import com.epeit.api.service.wechatPay.RequestHandler;
import com.epeit.api.service.wechatPay.client.TenpayHttpClient;
import com.epeit.api.service.wechatPay.util.Sha1Util;
import com.epeit.api.service.wechatPay.util.TenpayUtils;
import com.epeit.api.service.wechatPay.util.WxPayConfig;
import com.epeit.api.util.SerialNumberUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.*;
/**
* Created by user on 2015/3/11.
*/
@FunctionCode(value = "wxPay", descript = "微信支付相关接口")
@Service
public class WxPayServiceImpl implements WxPayService {
@Autowired
private WxpayInfoMapper wxpayInfoMapper;
@Autowired
private TenpayParamsMapper tenpayParamsMapper;
@Autowired
private ShoppingOrderMapper shoppingOrderMapper;
@Autowired
private UserAddressMapper userAddressMapper;
@Value("${epeit.api.server}")
private String defaultApiServer;
private TenpayParams tenpayParams;
@FunctionCode(value = "wxPay.wxPayGoods", descript = "支付订单处理接口")
@Override
public ApiResponse