GridView控件修改、删除例子,修改时含有DropDownList控件。
示例运行效果图:
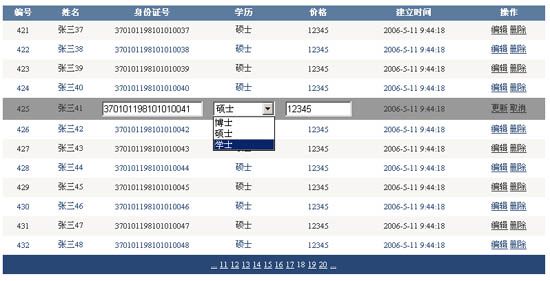
GridViewUp.aspx文件代码:
<%
@ Page Language
=
"
C#
"
AutoEventWireup
=
"
true
"
CodeFile
=
"
GridViewUp.aspx.cs
"
Inherits
=
"
gridview_GridViewUp
"
%>
<!
DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"
>
<
html
xmlns
="http://www.w3.org/1999/xhtml"
>
<
head
runat
="server"
>
<
title
>
无标题页
</
title
>
</
head
>
<
body
>
<
form
id
="form1"
runat
="server"
>
<
div
>
<
table
cellpadding
="0"
cellspacing
="0"
border
="0"
width
="80%"
style
="font-size: 11px"
>
<
tr
>
<
td
align
="center"
>
<
asp:GridView
ID
="GridView1"
runat
="server"
Width
="100%"
CellPadding
="4"
ForeColor
="#333333"
AutoGenerateColumns
="False"
AllowPaging
="True"
PageSize
="12"
OnRowCancelingEdit
="GridView1_RowCancelingEdit"
OnRowEditing
="GridView1_RowEditing"
OnRowUpdating
="GridView1_RowUpdating"
OnRowDeleting
="GridView1_RowDeleting"
DataKeyNames
="id,name"
OnPageIndexChanging
="GridView1_PageIndexChanging"
DataMember
="card,price"
OnRowDataBound
="GridView1_RowDataBound"
GridLines
="None"
>
<
Columns
>
<
asp:BoundField
HeaderText
="编号"
DataField
="id"
ReadOnly
="True"
/>
<
asp:BoundField
DataField
="name"
HeaderText
="姓名"
ReadOnly
="True"
/>
<
asp:TemplateField
HeaderText
="身份证号"
>
<
ItemTemplate
>
<%
#
Eval
(
"
card
"
)
%>
</
ItemTemplate
>
<
EditItemTemplate
>
<
asp:TextBox
ID
="TBCard"
Text
='<%#
Eval("card") %
>
' runat="server" Width="140px" />
</
EditItemTemplate
>
<
ItemStyle
Width
="150px"
/>
</
asp:TemplateField
>
<
asp:TemplateField
HeaderText
="学历"
>
<
ItemTemplate
>
<%
#
Eval
(
"
xueliText
"
)
%>
</
ItemTemplate
>
<
EditItemTemplate
>
<
asp:HiddenField
ID
="HDFXueli"
runat
="server"
Value
='<%#
Eval("xueli") %
>
' />
<
asp:DropDownList
ID
="DDLXueli"
runat
="server"
Width
="90px"
/>
</
EditItemTemplate
>
<
ItemStyle
Width
="100px"
/>
</
asp:TemplateField
>
<
asp:TemplateField
HeaderText
="价格"
>
<
ItemTemplate
>
<%
#
Eval
(
"
price
"
)
%>
</
ItemTemplate
>
<
EditItemTemplate
>
<
asp:TextBox
ID
="TBPrice"
Text
='<%#
Eval("price") %
>
' runat="server" Width="90px" />
</
EditItemTemplate
>
<
ItemStyle
Width
="100px"
/>
</
asp:TemplateField
>
<
asp:BoundField
HeaderText
="建立时间"
DataField
="createdate"
ReadOnly
="True"
/>
<
asp:CommandField
ShowDeleteButton
="True"
ShowEditButton
="True"
HeaderText
="操作"
/>
</
Columns
>
<
PagerSettings
FirstPageText
=""
LastPageText
=""
NextPageText
=""
PreviousPageText
=""
/>
<
RowStyle
Height
="20px"
BackColor
="#F7F6F3"
ForeColor
="#333333"
/>
<
FooterStyle
BackColor
="#5D7B9D"
Font-Bold
="True"
ForeColor
="White"
/>
<
EditRowStyle
BackColor
="#999999"
/>
<
SelectedRowStyle
BackColor
="#E2DED6"
Font-Bold
="True"
ForeColor
="#333333"
/>
<
PagerStyle
BackColor
="#284775"
ForeColor
="White"
HorizontalAlign
="Center"
/>
<
HeaderStyle
BackColor
="#5D7B9D"
Font-Bold
="True"
ForeColor
="White"
/>
<
AlternatingRowStyle
BackColor
="White"
ForeColor
="#284775"
/>
</
asp:GridView
>
</
td
>
</
tr
>
</
table
>
</
div
>
</
form
>
</
body
>
</
html
>
GridViewUp.aspx.cs文件代码:
using
System;
using
System.Data;
using
System.Configuration;
using
System.Collections;
using
System.Web;
using
System.Web.Security;
using
System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Web.UI.WebControls.WebParts;
using
System.Web.UI.HtmlControls;
using
System.Data.SqlClient;
public
partial
class
gridview_GridViewUp : System.Web.UI.Page
{
protected
void
Page_Load(
object
sender, EventArgs e)
{
if
(
!
IsPostBack)
{
GridViewBind();
}
}
protected
void
GridView1_PageIndexChanging(
object
sender, GridViewPageEventArgs e)
{
GridView1.PageIndex
=
e.NewPageIndex;
GridViewBind();
}
private
void
GridViewBind()
{
string
connStr
=
ConfigurationManager.ConnectionStrings[
"
ConnString
"
].ConnectionString;
string
SqlStr
=
"
SELECT *, case xueli when '1' then '博士' when '2' then '硕士' when '3' then '学士 ' else '' end as xueliText FROM test01 where id<1000 and id>200
"
;
DataSet ds
=
new
DataSet();
try
{
SqlConnection conn
=
new
SqlConnection(connStr);
if
(conn.State.ToString()
==
"
Closed
"
) conn.Open();
SqlDataAdapter da
=
new
SqlDataAdapter(SqlStr, conn);
da.Fill(ds,
"
test01
"
);
if
(conn.State.ToString()
==
"
Open
"
) conn.Close();
GridView1.DataSource
=
ds.Tables[
0
].DefaultView;
GridView1.DataBind();
}
catch
(Exception ex)
{
Response.Write(
"
数据库错误,错误原因:
"
+
ex.Message);
Response.End();
}
}
protected
void
GridView1_RowDataBound(
object
sender, GridViewRowEventArgs e)
{
if
(((DropDownList)e.Row.FindControl(
"
DDLXueli
"
))
!=
null
)
{
DropDownList ddlxueli
=
(DropDownList)e.Row.FindControl(
"
DDLXueli
"
);
//
生成 DropDownList 的值,也可以取得数据库中的数据绑定
ddlxueli.Items.Clear();
ddlxueli.Items.Add(
new
ListItem(
"
博士
"
,
"
1
"
));
ddlxueli.Items.Add(
new
ListItem(
"
硕士
"
,
"
2
"
));
ddlxueli.Items.Add(
new
ListItem(
"
学士
"
,
"
3
"
));
//
//
选中 DropDownList
ddlxueli.SelectedValue
=
((HiddenField)e.Row.FindControl(
"
HDFXueli
"
)).Value;
//
}
}
protected
void
GridView1_RowEditing(
object
sender, GridViewEditEventArgs e)
{
GridView1.EditIndex
=
e.NewEditIndex;
GridViewBind();
}
protected
void
GridView1_RowCancelingEdit(
object
sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex
=
-
1
;
GridViewBind();
}
protected
void
GridView1_RowUpdating(
object
sender, GridViewUpdateEventArgs e)
{
string
id
=
GridView1.DataKeys[e.RowIndex].Values[
0
].ToString();
string
card
=
((TextBox)GridView1.Rows[e.RowIndex].FindControl(
"
TBCard
"
)).Text;
string
xueli
=
((DropDownList)GridView1.Rows[e.RowIndex].FindControl(
"
DDLXueli
"
)).SelectedValue;
string
price
=
((TextBox)GridView1.Rows[e.RowIndex].FindControl(
"
TBPrice
"
)).Text;
string
connStr
=
ConfigurationManager.ConnectionStrings[
"
ConnString
"
].ConnectionString;
string
SqlStr
=
"
update test01 set card='
"
+
card
+
"
',xueli='
"
+
xueli
+
"
',price='
"
+
price
+
"
' where id=
"
+
id;
try
{
SqlConnection conn
=
new
SqlConnection(connStr);
if
(conn.State.ToString()
==
"
Closed
"
) conn.Open();
SqlCommand comm
=
new
SqlCommand(SqlStr, conn);
comm.ExecuteNonQuery();
comm.Dispose();
if
(conn.State.ToString()
==
"
Open
"
) conn.Close();
GridView1.EditIndex
=
-
1
;
GridViewBind();
}
catch
(Exception ex)
{
Response.Write(
"
数据库错误,错误原因:
"
+
ex.Message);
Response.End();
}
}
protected
void
GridView1_RowDeleting(
object
sender, GridViewDeleteEventArgs e)
{
string
id
=
GridView1.DataKeys[e.RowIndex].Values[
0
].ToString();
string
connStr
=
ConfigurationManager.ConnectionStrings[
"
ConnString
"
].ConnectionString;
string
SqlStr
=
"
delete from test01 where id=
"
+
id;
try
{
SqlConnection conn
=
new
SqlConnection(connStr);
if
(conn.State.ToString()
==
"
Closed
"
) conn.Open();
SqlCommand comm
=
new
SqlCommand(SqlStr, conn);
comm.ExecuteNonQuery();
comm.Dispose();
if
(conn.State.ToString()
==
"
Open
"
) conn.Close();
GridView1.EditIndex
=
-
1
;
GridViewBind();
}
catch
(Exception ex)
{
Response.Write(
"
数据库错误,错误原因:
"
+
ex.Message);
Response.End();
}
}
}
sql server2000生成表代码:
CREATE
TABLE
[
dbo
]
.
[
test01
]
(
[
id
]
[
decimal
]
(
18
,
0
)
IDENTITY
(
1
,
1
)
NOT
NULL
,
[
name
]
[
varchar
]
(
50
) COLLATE Chinese_PRC_CI_AS
NULL
,
[
card
]
[
varchar
]
(
50
) COLLATE Chinese_PRC_CI_AS
NULL
,
[
xueli
]
[
varchar
]
(
50
) COLLATE Chinese_PRC_CI_AS
NULL
,
[
price
]
[
decimal
]
(
18
,
0
)
NULL
,
[
createdate
]
[
datetime
]
NULL
)
ON
[
PRIMARY
]
GO
ALTER
TABLE
[
dbo
]
.
[
test01
]
ADD
CONSTRAINT
[
DF_test01_createdate
]
DEFAULT
(
getdate
())
FOR
[
createdate
]
,
CONSTRAINT
[
PK_test01
]
PRIMARY
KEY
CLUSTERED
(
[
id
]
)
ON
[
PRIMARY
]
GO