pandas学习笔记(3)
追加(Append)
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(3,3), columns=['A', 'B', 'C'])
print(df)
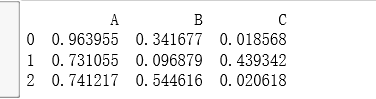
s =df.ix[0]
print(s)
a=df.append(s, ignore_index=True)
print(a)
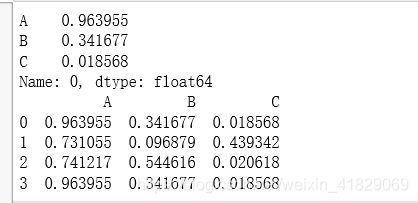
简单的计算
df = pd.DataFrame(np.random.randint(0,7,(3,3)), columns=['A', 'B', 'C'])
print(df)
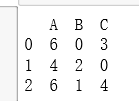
print(df.sum())
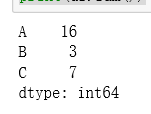
print(df.sum(axis=1))
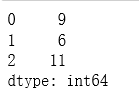
print(df.max())
print()
print(df.min())
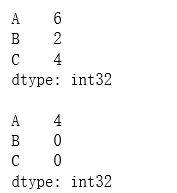
排序
df = pd.DataFrame(np.random.randint(0,7,(7,3)), columns=['A', 'B', 'C'])
print(df)
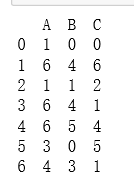
print(df.sort_values('A',ascending=False))
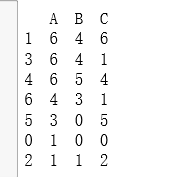
print(df.sort_values(by=0,axis=1))
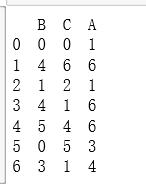
Apply函数
df = pd.DataFrame(np.random.randint(0,7,(3,3)), columns=['A', 'B', 'C'])
print(df)
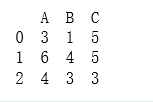
def f(x):
return x.min()
print(df.apply(f,axis=0))
print()
print(df.apply(f,axis=1))
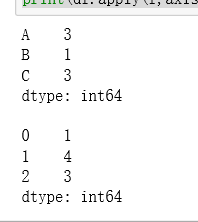
分组(Grouping)
c=pd.DataFrame({
'call':4,
'age':[5,4,3,2,1],
'sex':['M','F','M','F','M'],
'num':[12,39,45,67,45]
})
print(c)
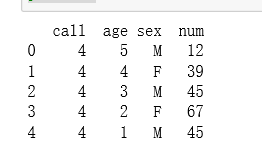
print(c.groupby('sex').sum())
堆叠(Stack)
new_stsck=c.stack()
print(new_stsck)
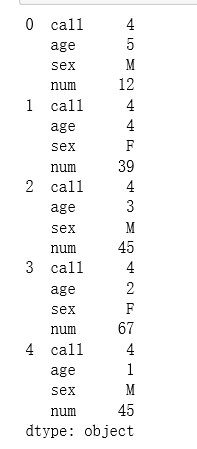
print(new_stsck.unstack())
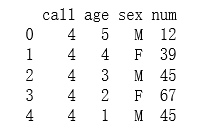
print(new_stsck.unstack(level=0))
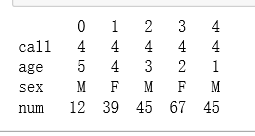
数据透视表(PivotTables)
df = pd.DataFrame({
'购买者': ['小米', '小华', '小魅', '小V'] * 3,
'水果种类': ['苹果', '葡萄', '柚子'] * 4,
'信息': ['价格','数量' ] * 6,
'2019': np.random.randint(12,size=12),
'2018': np.random.randint(12,size=12)})
print(df)
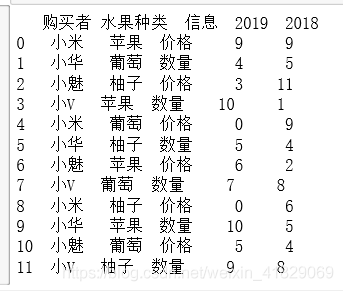
c=pd.pivot_table(df, values='2019', index=['购买者', '水果种类'], columns=['信息'])
print(c)
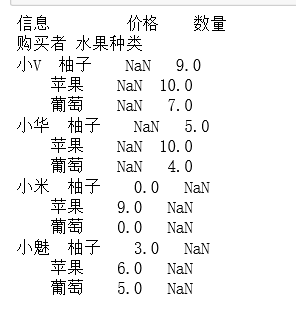