1.文字绘制
<script>
Cesium.Camera.DEFAULT_VIEW_RECTANGLE = Cesium.Rectangle.fromDegrees(90, -20, 110, 90);
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false,
});
var entity = viewer.entities.add({
position: Cesium.Cartesian3.fromDegrees(120, 30, 200),
point: {
color: Cesium.Color.RED,
pixelSize: 10
},
label: {
text: '测试点',
font: '14pt Source Han Sans CN',
fillColor: Cesium.Color.BLACK,
backgroundColor: Cesium.Color.AQUA,
showBackground: true,
outline: true,
outlineColor: Cesium.Color.WHITE,
outlineWidth: 10,
scale: 1.0,
style: Cesium.LabelStyle.FILL_AND_OUTLINE,
verticalOrigin: Cesium.VerticalOrigin.CENTER,
horizontalOrigin: Cesium.HorizontalOrigin.LEFT,
pixelOffset: new Cesium.Cartesian2(10, 0),
show: true
}
});
viewer.zoomTo(entity);
</script>
效果图
2.图标绘制
<script>
Cesium.Camera.DEFAULT_VIEW_RECTANGLE = Cesium.Rectangle.fromDegrees(90, -20, 110, 90);
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false,
});
var entity = viewer.entities.add({
position: Cesium.Cartesian3.fromDegrees(110.20, 34.55, 2000),
billboard: {
image: '../../static/image/device.png',
color: Cesium.Color.WHITE.withAlpha(0.8),
height: 50,
width: 50,
sizeInMeters: false,
verticalOrigin: Cesium.VerticalOrigin.CENTER,
horizontalOrigin: Cesium.HorizontalOrigin.LEFT,
scale: 1.0,
show: true
}
});
viewer.zoomTo(entity);
</script>
效果图
3.立方体
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var blueBox = viewer.entities.add({
name: 'Blue box',
position: Cesium.Cartesian3.fromDegrees(-114.0, 40.0, 300000.0),
box: {
dimensions: new Cesium.Cartesian3(400000.0, 300000.0, 500000.0),
material: Cesium.Color.BLUE
}
});
var redBox = viewer.entities.add({
name: 'Red box with black outline',
position: Cesium.Cartesian3.fromDegrees(-107.0, 40.0, 300000.0),
box: {
dimensions: new Cesium.Cartesian3(400000.0, 300000.0, 500000.0),
material: Cesium.Color.RED,
outline: true,
outlineColor: Cesium.Color.BLACK
}
});
var outlineOnly = viewer.entities.add({
name: 'Yellow box outline',
position: Cesium.Cartesian3.fromDegrees(-100.0, 40.0, 300000.0),
box: {
dimensions: new Cesium.Cartesian3(400000.0, 300000.0, 500000.0),
fill: false,
outline: true,
outlineColor: Cesium.Color.YELLOW
}
});
viewer.zoomTo(redBox);
</script>
效果图
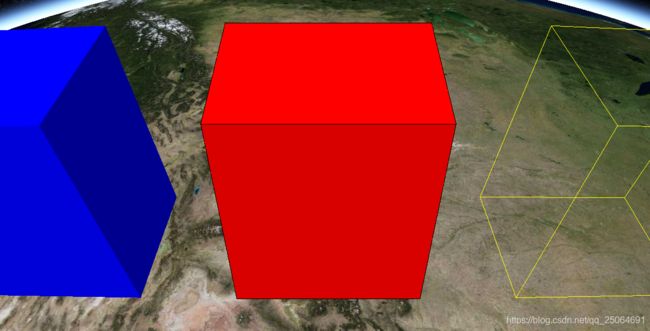
4.圆和椭圆
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var greenCircle = viewer.entities.add({
position: Cesium.Cartesian3.fromDegrees(-111.0, 40.0, 150000.0),
name: 'Green circle at height',
ellipse: {
semiMinorAxis: 300000.0,
semiMajorAxis: 300000.0,
height: 200000.0,
material: Cesium.Color.GREEN
}
});
var redEllipse = viewer.entities.add({
position: Cesium.Cartesian3.fromDegrees(-103.0, 40.0),
name: 'Red ellipse on surface with outline',
ellipse: {
semiMinorAxis: 250000.0,
semiMajorAxis: 400000.0,
material: Cesium.Color.RED.withAlpha(0.5),
outline: true,
outlineColor: Cesium.Color.RED
}
});
var blueEllipse = viewer.entities.add({
position: Cesium.Cartesian3.fromDegrees(-95.0, 40.0, 100000.0),
name: 'Blue translucent, rotated, and extruded ellipse',
ellipse: {
semiMinorAxis: 150000.0,
semiMajorAxis: 300000.0,
extrudedHeight: 200000.0,
rotation: Cesium.Math.toRadians(45),
material: Cesium.Color.BLUE.withAlpha(0.7),
outline: true
}
});
viewer.zoomTo(viewer.entities);
viewer.zoomTo(redBox);
</script>
效果图
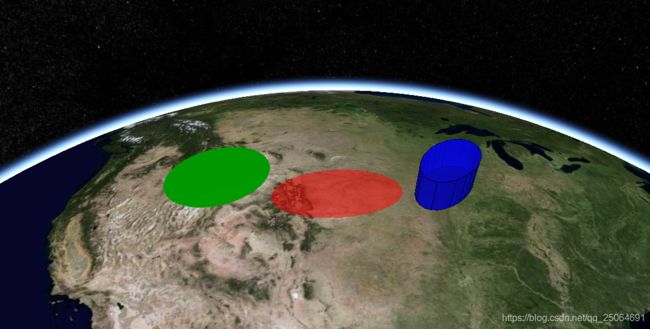
5.走廊
<script>
Cesium.Camera.DEFAULT_VIEW_RECTANGLE = Cesium.Rectangle.fromDegrees(90, -20, 110, 90);
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var redCorridor = viewer.entities.add({
name: 'Red corridor on surface with rounded corners and outline',
corridor: {
positions: Cesium.Cartesian3.fromDegreesArray([
-100.0, 40.0,
-105.0, 40.0,
-105.0, 35.0
]),
width: 200000.0,
material: Cesium.Color.RED.withAlpha(0.5),
outline: true,
outlineColor: Cesium.Color.RED
}
});
var greenCorridor = viewer.entities.add({
name: 'Green corridor at height with mitered corners',
corridor: {
positions: Cesium.Cartesian3.fromDegreesArray(
[-90.0, 40.0,
-95.0, 40.0,
-95.0, 35.0
]),
height: 100000.0,
width: 200000.0,
cornerType: Cesium.CornerType.MITERED,
material: Cesium.Color.GREEN
}
});
var blueCorridor = viewer.entities.add({
name: 'Blue extruded corridor with beveled corners and outline',
corridor: {
positions: Cesium.Cartesian3.fromDegreesArray(
[80.0, 40.0,
-85.0, 40.0,
-85.0, 35.0
]),
height: 200000.0,
extrudedHeight: 100000.0,
width: 200000.0,
cornerType: Cesium.CornerType.BEVELED,
material: Cesium.Color.BLUE.withAlpha(0.5),
outline: true,
outlineColor: Cesium.Color.BLUE
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图
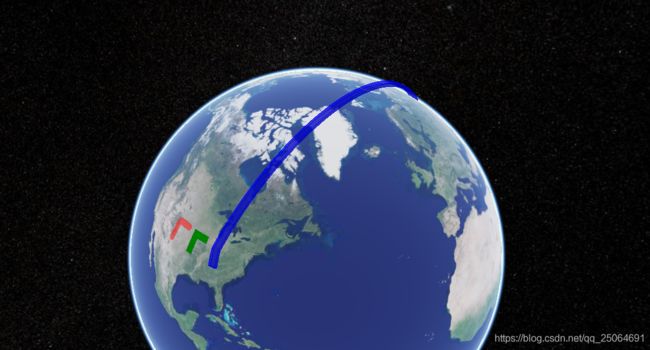
6.圆柱和圆锥
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var greenCylinder = viewer.entities.add({
name: 'Green cylinder with black outline',
position: Cesium.Cartesian3.fromDegrees(-100.0, 40.0, 200000.0),
cylinder: {
length: 400000.0,
topRadius: 200000.0,
bottomRadius: 200000.0,
material: Cesium.Color.GREEN,
outline: true,
outlineColor: Cesium.Color.DARK_GREEN
}
});
var redCone = viewer.entities.add({
name: 'Red cone',
position: Cesium.Cartesian3.fromDegrees(-105.0, 40.0, 200000.0),
cylinder: {
length: 400000.0,
topRadius: 0.0,
bottomRadius: 200000.0,
material: Cesium.Color.RED
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图
7.多边形
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var redPolygon = viewer.entities.add({
name : '贴着地表的多边形',
polygon : {
hierarchy : Cesium.Cartesian3.fromDegreesArray([-115.0, 37.0,
-115.0, 32.0,
-107.0, 33.0,
-102.0, 31.0,
-102.0, 35.0]),
material : Cesium.Color.RED
}
});
var greenPolygon = viewer.entities.add({
name : '绿色拉伸多边形',
polygon : {
hierarchy : Cesium.Cartesian3.fromDegreesArray([-108.0, 42.0,
-100.0, 42.0,
-104.0, 40.0]),
extrudedHeight: 500000.0,
material : Cesium.Color.GREEN
}
});
var orangePolygon = viewer.entities.add({
name : '每个顶点具有不同拉伸高度的橘色多边形',
polygon : {
hierarchy : Cesium.Cartesian3.fromDegreesArrayHeights(
[-108.0, 25.0, 100000,
-100.0, 25.0, 100000,
-100.0, 30.0, 100000,
-108.0, 30.0, 300000]),
extrudedHeight: 0,
perPositionHeight : true,
material : Cesium.Color.ORANGE,
outline : true,
outlineColor : Cesium.Color.BLACK
}
});
var bluePolygon = viewer.entities.add({
name : '具有挖空效果的蓝色多边形',
polygon : {
hierarchy : {
positions : Cesium.Cartesian3.fromDegreesArray(
[-99.0, 30.0,
-85.0, 30.0,
-85.0, 40.0,
-99.0, 40.0]),
holes : [{
positions : Cesium.Cartesian3.fromDegreesArray([
-97.0, 31.0,
-97.0, 39.0,
-87.0, 39.0,
-87.0, 31.0
]),
holes : [{
positions : Cesium.Cartesian3.fromDegreesArray([
-95.0, 33.0,
-89.0, 33.0,
-89.0, 37.0,
-95.0, 37.0
]),
holes : [{
positions : Cesium.Cartesian3.fromDegreesArray([
-93.0, 34.0,
-91.0, 34.0,
-91.0, 36.0,
-93.0, 36.0
])
}]
}]
}]
},
material : Cesium.Color.BLUE,
outline : true,
outlineColor : Cesium.Color.BLACK
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图

8.线段
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var redLine = viewer.entities.add({
name : '沿着地球表面的红线',
polyline : {
positions : Cesium.Cartesian3.fromDegreesArray(
[-75, 35,
-125, 35]),
width : 5,
material : Cesium.Color.RED
}
});
var glowingLine = viewer.entities.add({
name : '具有发光效果的线',
polyline : {
positions : Cesium.Cartesian3.fromDegreesArray(
[-75, 37, -125, 37]
),
width : 10,
material : new Cesium.PolylineGlowMaterialProperty({
glowPower : 0.2,
color : Cesium.Color.BLUE
})
}
});
var orangeOutlined = viewer.entities.add({
name : '具有一定高度的线',
polyline : {
positions : Cesium.Cartesian3.fromDegreesArrayHeights(
[-75, 39, 250000,-125, 39, 250000]
),
width : 5,
material : new Cesium.PolylineOutlineMaterialProperty({
color : Cesium.Color.ORANGE,
outlineWidth : 2,
outlineColor : Cesium.Color.BLACK
})
}
});
var yellowLine = viewer.entities.add({
name : '不贴着地表的线',
polyline : {
positions : Cesium.Cartesian3.fromDegreesArrayHeights(
[-75, 43, 500000,-125, 43, 500000]
),
width : 3,
followSurface : false,
material : Cesium.Color.PURPLE
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图
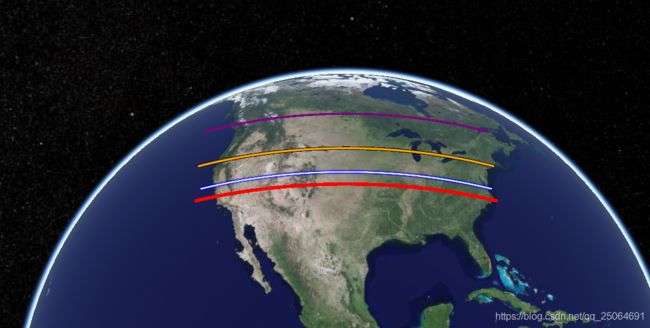
9.球和椭圆
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var blueEllipsoid = viewer.entities.add({
name: 'Blue ellipsoid',
position: Cesium.Cartesian3.fromDegrees(-114.0, 40.0, 300000.0),
ellipsoid: {
radii: new Cesium.Cartesian3(200000.0, 200000.0, 300000.0),
material: Cesium.Color.BLUE
}
});
var redSphere = viewer.entities.add({
name: 'Red sphere with black outline',
position: Cesium.Cartesian3.fromDegrees(-107.0, 40.0, 300000.0),
ellipsoid: {
radii: new Cesium.Cartesian3(300000.0, 300000.0, 300000.0),
material: Cesium.Color.RED,
outline: true,
outlineColor: Cesium.Color.BLACK
}
});
var outlineOnly = viewer.entities.add({
name: 'Yellow ellipsoid outline',
position: Cesium.Cartesian3.fromDegrees(-100.0, 40.0, 300000.0),
ellipsoid: {
radii: new Cesium.Cartesian3(200000.0, 200000.0, 300000.0),
fill: false,
outline: true,
outlineColor: Cesium.Color.YELLOW,
slicePartitions: 24,
stackPartitions: 36
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图
10.墙
<script>
var viewer = new Cesium.Viewer("csiumContain", {
homeButton: true,
animation: false,
timeline: false,
fullscreenButton: false,
baseLayerPicker: false,
sceneModePicker: true,
navigationHelpButton: false,
geocoder: false,
sceneModePicker: false
});
var redWall = viewer.entities.add({
name: 'Red wall at height',
wall: {
positions: Cesium.Cartesian3.fromDegreesArrayHeights(
[-115.0, 44.0, 200000.0,
-90.0, 44.0, 200000.0]
),
minimumHeights: [100000.0, 100000.0],
material: Cesium.Color.RED
}
});
var greenWall = viewer.entities.add({
name: 'Green wall from surface with outline',
wall: {
positions: Cesium.Cartesian3.fromDegreesArrayHeights(
[-107.0, 43.0, 100000.0,
-97.0, 43.0, 100000.0,
-97.0, 40.0, 100000.0,
-107.0, 40.0, 100000.0,
-107.0, 43.0, 100000.0]),
material: Cesium.Color.GREEN,
outline: true,
outlineColor: Cesium.Color.BLACK
}
});
var blueWall = viewer.entities.add({
name: 'Blue wall with sawtooth heights and outline',
wall: {
positions: Cesium.Cartesian3.fromDegreesArray(
[-115.0, 50.0,
-112.5, 50.0,
-110.0, 50.0,
-107.5, 50.0,
-105.0, 50.0,
-102.5, 50.0,
-100.0, 50.0,
-97.5, 50.0,
-95.0, 50.0,
-92.5, 50.0,
-90.0, 50.0]),
maximumHeights: [
100000, 200000, 100000, 200000, 100000, 200000,
100000, 200000, 100000, 200000, 100000],
minimumHeights: [
0, 100000, 0, 100000, 0, 100000, 0, 100000, 0,
100000, 0],
material: Cesium.Color.BLUE,
outline: true,
outlineColor: Cesium.Color.BLACK
}
});
viewer.zoomTo(viewer.entities);
</script>
效果图
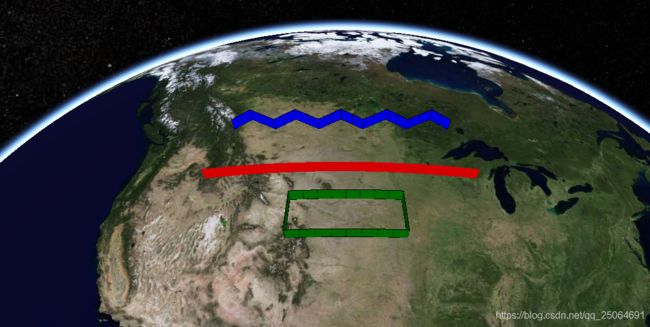