通过一些通信方式传输的数据(比如:串口传输),有时候会保存为char类型的数据数据,我们需要把char数组中的所有数据转化为int整数,方法一是直接通过移位运算来实现:charc[4]={0x3,0x0f,0xf,0xf};chart;intnum=0;intlen=sizeof(c)/2;chard[sizeof(c)/2]{0};for(inti=0,j=0;j
Software PLC Solution for RK3568+Codesys ARM+LINUX Hardware Platform
ARM+FPGA+AI工业主板定制专家
RK+CodesyslinuxCodesysRK3568PLC
CODESYSControlLinuxARMSLAsoftPLCthatcomplieswiththeIEC61131-3standardandissuitableforARM&Linuxhardwareplatforms.ProductDescriptionCODESYSControlLinuxARMSLisaCODESYSRuntimedesignedforARM&Linuxhardwarep
2025最新版二级域名分发最新开心版 支持易支付接口和聚合登录接口
专业软件系统开发
源码下载付费域名分发域名分发系统源码
内容目录一、详细介绍宝塔面板环境PHP版本8.0至8.3PHP扩展SG15Mysql5.6或5.71Panel环境二、效果展示1.部分代码2.效果图展示请添加图片描述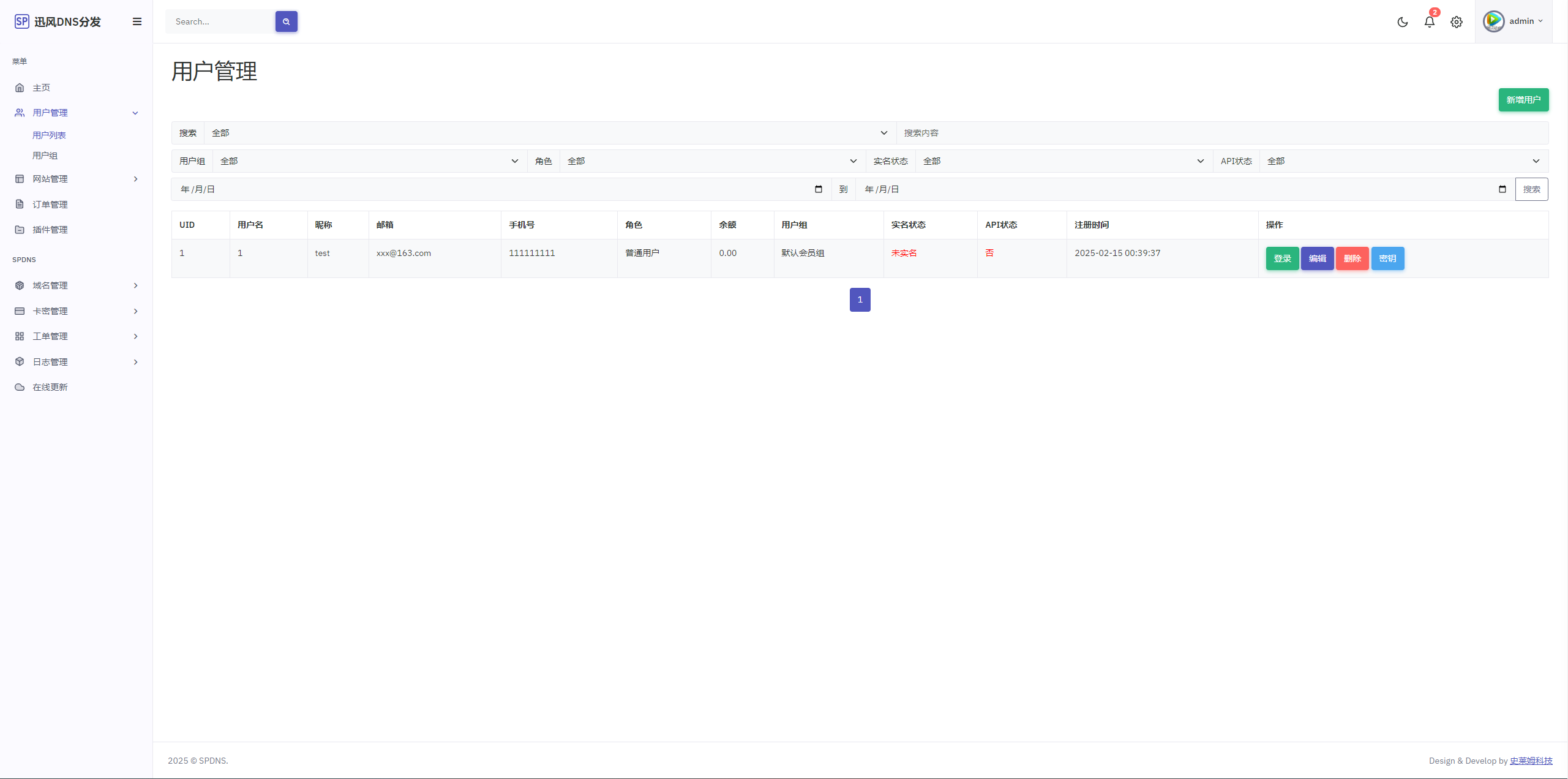三、学习资料下载一、详细介绍一站式对域名进行二级分发,自助添加,自助修改
android database SQLite
一路阳光随行
Androidsqlitedatabaseandroid存储数据库
2.数据库基本知识观花对于一些和我一样还没有真正系统学习数据库技术的同学来说,把SQL92标准中的一些基本概念、基本语句快速的了解一下,是很有必要的,这样待会用Android的database相关方法去执行一些数据库语句时就不会茫然了。①数据库的基本结构——表格表格是数据库中储存资料的基本架构。表格被分为栏位(column)及列位(row)。每一列代表一笔资料,而每一栏代表一笔资料的一部份。举例来
git之reset命令
crayon-shin-chan
surprise#git版本控制git
1.简介git-reset:将当前的HEAD重置为指定状态,也就是重置顶部commit的引用2.概要gitreset[-q][][--]…gitreset[-q][--pathspec-from-file=[--pathspec-file-nul]][]gitreset(--patch|-p)[][--][…]gitreset[--soft|--mixed[-N]|--hard|--merge
在nodejs中使用RabbitMQ(六)sharding消息分片
konglong127
nodejsrabbitmq分布式
RabbitMQ的分片插件(rabbitmq_sharding)允许将消息分布到多个队列中,这在消息量很大或处理速度要求高的情况下非常有用。分片功能通过将消息拆分到多个队列中来平衡负载,从而提升消息处理的吞吐量和可靠性。它能够在多个队列之间分配负载,避免单个队列过载。(注:不能单独消费分片消息。消息分片不利于消息顺序区分)启用消息分片插件。rabbitmq-pluginsenablerabbitm
《Operating System Concepts》阅读笔记:p17-p25
操作系统
《OperatingSystemConcepts》学习第5天,p17-p25总结,总计9页。一、技术总结1.计算机系统的组成结构(1)CPU—Thehardwarethatexecutesinstructions.(2)Processor—AphysicalchipthatcontainsoneormoreCPUs.(3)Core—ThebasiccomputationunitoftheCPU.(
python创建sqlite3数据库_SQLite – Python | 菜鸟教程
weixin_39683144
SQLite-Python安装SQLite3可使用sqlite3模块与Python进行集成。sqlite3模块是由GerhardHaring编写的。它提供了一个与PEP249描述的DB-API2.0规范兼容的SQL接口。您不需要单独安装该模块,因为Python2.5.x以上版本默认自带了该模块。为了使用sqlite3模块,您首先必须创建一个表示数据库的连接对象,然后您可以有选择地创建光标对象,这将
CP AUTOSAR标准之IOHardwareAbstraction(AUTOSAR_SWS_IOHardwareAbstraction)(更新中……)
瑟寒凌风
经典autosar(CP)平台嵌入式硬件linux汽车车载系统单片机
1简介和功能概述 AUTOSAR基础软件I/O硬件抽象的功能和配置。I/O硬件抽象是ECU抽象层的一部分。 I/O硬件抽象不应被视为单个模块,因为它可以作为多个模块实现。本I/O硬件抽象规范并非旨在标准化此模块或模块组。相反,它旨在作为其与其他模块功能接口实现的指南。 通过将I/O硬件抽象端口映射到ECU信号来提供对MCAL驱动程序的访问。提供给软件组件的数据完全从物理层值中抽象出来。因此,
Spring Boot集成ShardingSphere实现读写分离 | Spring Cloud 43
gmHappy
springbootspringcloud数据库
一、读写分离1.1背景面对日益增加的系统访问量,数据库的吞吐量面临着巨大瓶颈。对于同一时刻有大量并发读操作和较少写操作类型的应用系统来说,将数据库拆分为主库和从库,主库负责处理事务性的增删改操作,从库负责处理查询操作,能够有效的避免由数据更新导致的行锁,使得整个系统的查询性能得到极大的改善。通过一主多从的配置方式,可以将查询请求均匀的分散到多个数据副本,能够进一步的提升系统的处理能力。使用多主多从
数据库扩展之道:分区、分片与大表优化实战
title:数据库扩展之道:分区、分片与大表优化实战date:2025/2/15updated:2025/2/15author:cmdragonexcerpt:随着数据量的爆炸式增长,传统单机数据库的性能和存储能力逐渐成为瓶颈。数据库扩展的核心技术——分区(Partitioning)与分片(Sharding),并结合大表管理优化策略,提供从理论到实践的完整解决方案。通过实际案例(如MySQL分区实
牛客网面试必刷TOP101-09双指针BM92 最长无重复子数组
bingw0114
面试算法职场和发展
描述给定一个长度为n的数组arr,返回arr的最长无重复元素子数组的长度,无重复指的是所有数字都不相同。子数组是连续的,比如[1,3,5,7,9]的子数组有[1,3],[3,5,7]等等,但是[1,3,7]不是子数组数据范围:0≤arr.length≤10^5,0max)max=count;}else{while(arr[left]!=arr[right]){num[arr[left]]=0;co
基于开源千文模型(如Qwen、ChatGLM等)实施如何进行动态蒸馏,详细说明操作步骤.
墨者清风
模型训练人工智能技术发展模型动态蒸馏人工智能深度学习语言模型
基于开源千文模型(如Qwen、ChatGLM等)实施如何进行动态蒸馏,详细说明操作步骤.1.动态蒸馏的核心思想动态蒸馏的目标是通过教师模型(通常是一个较大的预训练模型)的输出,指导学生模型(较小的模型)的训练。具体来说:教师模型:提供软标签(softlabels),即概率分布,而不是硬标签(hardlabels)。学生模型:通过模仿教师模型的输出分布,学习更丰富的知识。动态蒸馏:在训练过程中,教师
influxdb数据过期_「监控」InfluxDB系统架构分析
云锋金融
influxdb数据过期
InfluxDB系统架构参考:时序数据库技术体系–初识InfluxDB简单的理解:DatabaseInfluxDB中有着和传统数据库一样的Database的概念RetentionPolicy(RP)数据保留策略。核心作用有3个:指定数据的过期时间,指定数据副本数量以及指定ShardGroupDuration.RP创建语句如下:CREATERETENTIONPOLICYONONDURATIONREP
LLM大模型中文开源数据集集锦(三)
悟乙己
付费-智能写作专栏LLM大模型开源大模型LLMGPT微调
文章目录1ChatGLM-Med:基于中文医学知识的ChatGLM模型微调1.1数据集1.2ChatGLM+P-tuningV2微调1.3Llama+Alpaca的Lora微调版本2LawGPT_zh:中文法律大模型(獬豸)2.1数据集2.1.1利用ChatGPT清洗CrimeKgAssitant数据集得到52k单轮问答:2.1.2带有法律依据的情景问答92k:2.1.3法律知识问答2.2模型3C
Linux Python2.7离线安装request库
Citrus-
centospython
1.官网下载相应依赖包,涉及依赖包如下:现成打包好的:https://cloud.189.cn/t/v2m22i326zAb(访问码:j847)官网地址:https://pypi.org/setuptools-41.1.0.post1.tarpip-19.2.2.tar.gzcertifi-2019.9.11-py2.py3-none-any.whlchardet-3.0.4-py2.py3-no
unittest自动化测试-requests库实现http请求与requests库离线安装
herryone123
自动化测试自动化httppython
一、requests库离线安装1.1安装requests模块所需依赖包(1)所需依赖包chardet,idna,urllib3,certifi(2)下载地址:https://www.lfd.uci.edu/~gohlke/pythonlibs/chardet·PyPI(3)安装方式pipinstallxx.whlpycharmTerminal窗口执行pipinstallcertifi-2019.1
flutter启动后不显示文字,中文字体不显示
tower888
flutter前端dart
Flutter3.29.0-1.0.pre.114运行报错:FailedtoloadfontRobotoathttps://fonts.gstatic.com/s/roboto/v32/KFOmCnqEu92Fr1Me4GZLCzYlKw.woff2解决:下载roboto字体,并将字体(Roboto-Regular.ttf)放入app代码下,配置默认fonts:flutter:uses-mater
Java后端微服务架构下的数据库分库分表:Sharding-Sphere
微赚淘客机器人开发者联盟@聚娃科技
架构java微服务
Java后端微服务架构下的数据库分库分表:Sharding-Sphere大家好,我是微赚淘客返利系统3.0的小编,是个冬天不穿秋裤,天冷也要风度的程序猿!随着微服务架构的广泛应用,数据库层面的扩展性问题逐渐凸显。Sharding-Sphere作为一个分布式数据库中间件,提供了数据库分库分表的能力,帮助开发者解决数据水平拆分的问题。数据库分库分表概述数据库分库分表是将数据分布到不同的数据库和表中,以
使用 TinyGo 和 Gopherbot 进行硬件hacking
后端go
本篇内容是根据2019年4月份#84HardwarehackingwithTinyGoandGopherbot音频录制内容的整理与翻译MatRyer与特邀嘉宾RonEvans共同主持了第一期一对一采访式节目。Mat请Ron教我们有关IoT中的Go、Gophercon、TinyGo和Gopherbot的硬件黑客技术。过程中为符合中文惯用表达有适当删改,版权归原作者所有.MatRyer:大家好,我是M
MySQL-this is incompatible with sql_mode=only_full_group_by错误解决方案
IT_狂奔者
MySQLmysqlsql
出现"thisisincompatiblewithsql_mode=only_full_group_by"问题的解决方案一、原因(1)原理层面这个错误发生在mysql5.7版本及以上版本会出现的问题:mysql5.7版本默认的sql配置是:sql_mode="ONLY_FULL_GROUP_BY",这个配置严格执行了"SQL92标准"。很多从5.7升级到8.0时,为了语法兼容,大部分都会选择调整s
pico-sdk(五)-程序架构之库结构(2)
qichengzong_right
linux树莓派linux单片机c++
pico-sdk(五)-程序架构之库结构(2)硬件结构体库硬件寄存器库TinyUSB端口FreeRTOS端口在PicoW上使用Wi-Fi在PicoW上使用蓝牙硬件结构体库hardware_structs库提供了一组C结构体,这些结构体表示了系统地址空间中RP系列微控制器寄存器的内存映射布局1。能够用来替换较低层级的接口调用(这些内容原本需要用较低级别的hardware_regs中的宏定义来编写)。
AUTOSAR之CAN Driver
&等风来
AUTOSAR网络mcu单片机汽车驱动开发
一、CANDriver简介CANDriver在CAN通讯中的位置一个CANHardwareUint由一个或多个Controller组成,Controller可以位于芯片上,也可以作为外部独立的设备。一个Controller对应一个CAN节点即一个物理通道,一个一般包含多个报文邮箱(Mailbox)。二、CANDriver状态机CAN模块驱动状态机有两个状态,在上电或重置后,CAN模块进入CAN_U
MCU应用踩坑笔记(ADC 中断 / 查询法)
woainizhongguo.
常见问题/疑难杂症51/STM32单片机单片机笔记嵌入式硬件
问题描述IC:SC92F7596,在使用过程中,发现一个问题,就是我们使用到了ADC功能,程序的代码如下:ADC采样周期200ms,采样个数:4在使用过程中,因配置了ADC中断使能,在中断服务程序中,清除了了中断标志位。因为我开500ms的看门狗溢出复位,但是因为(1)当温度上升到45℃以上之后,ADC的采集时间会从us级别变到800ms,从而触发了看门狗溢出复位,红灯常亮(2)当温度下降到30℃
WPF入门_06资源和样式
思忖小下
WPFwpf资源和样式
目录1、资源基础介绍2、静态资源和动态资源区别3、资源字典4、共享资源的方法5、在CustomControlLibrary中定义和使用共享资源6、样式7、样式触发器1、资源基础介绍尽管每个元素都提供了Resources属性,但通常在窗口级别上定义资源,如下定义一个字符串资源LearningHard博客:http://www.cnblogs.com/zhili/2、静态资源和动态资源区别(参照代码:
Linux设备 (转)
timequark
EmbeddedSystem/RTOSlinux数据结构structbufferlinux内核cache
5.3.2设备类型分类纵览linux/drivers目录,大概还有35个以上的子目录,每个子目录基本上就代表了一种设备驱动,有atm、block、char、misc、input、net、usb、sound、video等。这里只描述在嵌入式系统里面用得最为广泛的3种设备。1.字符设备(chardevice)字符设备是Linux最简单的设备,可以像文件一样访问。初始化字符设备时,它的设备驱动程序向Li
力扣周赛:第419场周赛
布布要成为最强的人
力扣测试专栏leetcode算法javalambda数据结构
作者简介:爱好技术和算法的研究生上期文章:力扣周赛:第415场周赛订阅专栏:力扣周赛希望文章对你们有所帮助因为一些特殊原因,这场比赛就打了1h,所以只AC了前面两题。第三题后面补题自己AC了,第三个居然是个hard题,居然暴力+记忆化就AC了。第四题不会做,面试机试也不会考这么难的,第四题就不补了。力扣周赛:第419场周赛计算子数组的x-sumI第K大的完美二叉子树的大小统计能获胜的出招序列数计
LeetCode[位运算] - #137 Single Number II
Cwind
javaAlgorithmLeetCode题解位运算
原题链接:#137 Single Number II
要求:
给定一个整型数组,其中除了一个元素之外,每个元素都出现三次。找出这个元素
注意:算法的时间复杂度应为O(n),最好不使用额外的内存空间
难度:中等
分析:
与#136类似,都是考察位运算。不过出现两次的可以使用异或运算的特性 n XOR n = 0, n XOR 0 = n,即某一
《JavaScript语言精粹》笔记
aijuans
JavaScript
0、JavaScript的简单数据类型包括数字、字符创、布尔值(true/false)、null和undefined值,其它值都是对象。
1、JavaScript只有一个数字类型,它在内部被表示为64位的浮点数。没有分离出整数,所以1和1.0的值相同。
2、NaN是一个数值,表示一个不能产生正常结果的运算结果。NaN不等于任何值,包括它本身。可以用函数isNaN(number)检测NaN,但是
你应该更新的Java知识之常用程序库
Kai_Ge
java
在很多人眼中,Java 已经是一门垂垂老矣的语言,但并不妨碍 Java 世界依然在前进。如果你曾离开 Java,云游于其它世界,或是每日只在遗留代码中挣扎,或许是时候抬起头,看看老 Java 中的新东西。
Guava
Guava[gwɑ:və],一句话,只要你做Java项目,就应该用Guava(Github)。
guava 是 Google 出品的一套 Java 核心库,在我看来,它甚至应该
HttpClient
120153216
httpclient
/**
* 可以传对象的请求转发,对象已流形式放入HTTP中
*/
public static Object doPost(Map<String,Object> parmMap,String url)
{
Object object = null;
HttpClient hc = new HttpClient();
String fullURL
Django model字段类型清单
2002wmj
django
Django 通过 models 实现数据库的创建、修改、删除等操作,本文为模型中一般常用的类型的清单,便于查询和使用: AutoField:一个自动递增的整型字段,添加记录时它会自动增长。你通常不需要直接使用这个字段;如果你不指定主键的话,系统会自动添加一个主键字段到你的model。(参阅自动主键字段) BooleanField:布尔字段,管理工具里会自动将其描述为checkbox。 Cha
在SQLSERVER中查找消耗CPU最多的SQL
357029540
SQL Server
返回消耗CPU数目最多的10条语句
SELECT TOP 10
total_worker_time/execution_count AS avg_cpu_cost, plan_handle,
execution_count,
(SELECT SUBSTRING(text, statement_start_of
Myeclipse项目无法部署,Undefined exploded archive location
7454103
eclipseMyEclipse
做个备忘!
错误信息为:
Undefined exploded archive location
原因:
在工程转移过程中,导致工程的配置文件出错;
解决方法:
 
GMT时间格式转换
adminjun
GMT时间转换
普通的时间转换问题我这里就不再罗嗦了,我想大家应该都会那种低级的转换问题吧,现在我向大家总结一下如何转换GMT时间格式,这种格式的转换方法网上还不是很多,所以有必要总结一下,也算给有需要的朋友一个小小的帮助啦。
1、可以使用
SimpleDateFormat SimpleDateFormat
EEE-三位星期
d-天
MMM-月
yyyy-四位年
Oracle数据库新装连接串问题
aijuans
oracle数据库
割接新装了数据库,客户端登陆无问题,apache/cgi-bin程序有问题,sqlnet.log日志如下:
Fatal NI connect error 12170.
VERSION INFORMATION: TNS for Linux: Version 10.2.0.4.0 - Product
回顾java数组复制
ayaoxinchao
java数组
在写这篇文章之前,也看了一些别人写的,基本上都是大同小异。文章是对java数组复制基础知识的回顾,算是作为学习笔记,供以后自己翻阅。首先,简单想一下这个问题:为什么要复制数组?我的个人理解:在我们在利用一个数组时,在每一次使用,我们都希望它的值是初始值。这时我们就要对数组进行复制,以达到原始数组值的安全性。java数组复制大致分为3种方式:①for循环方式 ②clone方式 ③arrayCopy方
java web会话监听并使用spring注入
bewithme
Java Web
在java web应用中,当你想在建立会话或移除会话时,让系统做某些事情,比如说,统计在线用户,每当有用户登录时,或退出时,那么可以用下面这个监听器来监听。
import java.util.ArrayList;
import java.ut
NoSQL数据库之Redis数据库管理(Redis的常用命令及高级应用)
bijian1013
redis数据库NoSQL
一 .Redis常用命令
Redis提供了丰富的命令对数据库和各种数据库类型进行操作,这些命令可以在Linux终端使用。
a.键值相关命令
b.服务器相关命令
1.键值相关命令
&
java枚举序列化问题
bingyingao
java枚举序列化
对象在网络中传输离不开序列化和反序列化。而如果序列化的对象中有枚举值就要特别注意一些发布兼容问题:
1.加一个枚举值
新机器代码读分布式缓存中老对象,没有问题,不会抛异常。
老机器代码读分布式缓存中新对像,反序列化会中断,所以在所有机器发布完成之前要避免出现新对象,或者提前让老机器拥有新增枚举的jar。
2.删一个枚举值
新机器代码读分布式缓存中老对象,反序列
【Spark七十八】Spark Kyro序列化
bit1129
spark
当使用SparkContext的saveAsObjectFile方法将对象序列化到文件,以及通过objectFile方法将对象从文件反序列出来的时候,Spark默认使用Java的序列化以及反序列化机制,通常情况下,这种序列化机制是很低效的,Spark支持使用Kyro作为对象的序列化和反序列化机制,序列化的速度比java更快,但是使用Kyro时要注意,Kyro目前还是有些bug。
Spark
Hybridizing OO and Functional Design
bookjovi
erlanghaskell
推荐博文:
Tell Above, and Ask Below - Hybridizing OO and Functional Design
文章中把OO和FP讲的深入透彻,里面把smalltalk和haskell作为典型的两种编程范式代表语言,此点本人极为同意,smalltalk可以说是最能体现OO设计的面向对象语言,smalltalk的作者Alan kay也是OO的最早先驱,
Java-Collections Framework学习与总结-HashMap
BrokenDreams
Collections
开发中常常会用到这样一种数据结构,根据一个关键字,找到所需的信息。这个过程有点像查字典,拿到一个key,去字典表中查找对应的value。Java1.0版本提供了这样的类java.util.Dictionary(抽象类),基本上支持字典表的操作。后来引入了Map接口,更好的描述的这种数据结构。
&nb
读《研磨设计模式》-代码笔记-职责链模式-Chain Of Responsibility
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
/**
* 业务逻辑:项目经理只能处理500以下的费用申请,部门经理是1000,总经理不设限。简单起见,只同意“Tom”的申请
* bylijinnan
*/
abstract class Handler {
/*
Android中启动外部程序
cherishLC
android
1、启动外部程序
引用自:
http://blog.csdn.net/linxcool/article/details/7692374
//方法一
Intent intent=new Intent();
//包名 包名+类名(全路径)
intent.setClassName("com.linxcool", "com.linxcool.PlaneActi
summary_keep_rate
coollyj
SUM
BEGIN
/*DECLARE minDate varchar(20) ;
DECLARE maxDate varchar(20) ;*/
DECLARE stkDate varchar(20) ;
DECLARE done int default -1;
/* 游标中 注册服务器地址 */
DE
hadoop hdfs 添加数据目录出错
daizj
hadoophdfs扩容
由于原来配置的hadoop data目录快要用满了,故准备修改配置文件增加数据目录,以便扩容,但由于疏忽,把core-site.xml, hdfs-site.xml配置文件dfs.datanode.data.dir 配置项增加了配置目录,但未创建实际目录,重启datanode服务时,报如下错误:
2014-11-18 08:51:39,128 WARN org.apache.hadoop.h
grep 目录级联查找
dongwei_6688
grep
在Mac或者Linux下使用grep进行文件内容查找时,如果给定的目标搜索路径是当前目录,那么它默认只搜索当前目录下的文件,而不会搜索其下面子目录中的文件内容,如果想级联搜索下级目录,需要使用一个“-r”参数:
grep -n -r "GET" .
上面的命令将会找出当前目录“.”及当前目录中所有下级目录
yii 修改模块使用的布局文件
dcj3sjt126com
yiilayouts
方法一:yii模块默认使用系统当前的主题布局文件,如果在主配置文件中配置了主题比如: 'theme'=>'mythm', 那么yii的模块就使用 protected/themes/mythm/views/layouts 下的布局文件; 如果未配置主题,那么 yii的模块就使用 protected/views/layouts 下的布局文件, 总之默认不是使用自身目录 pr
设计模式之单例模式
come_for_dream
设计模式单例模式懒汉式饿汉式双重检验锁失败无序写入
今天该来的面试还没来,这个店估计不会来电话了,安静下来写写博客也不错,没事翻了翻小易哥的博客甚至与大牛们之间的差距,基础知识不扎实建起来的楼再高也只能是危楼罢了,陈下心回归基础把以前学过的东西总结一下。
*********************************
8、数组
豆豆咖啡
二维数组数组一维数组
一、概念
数组是同一种类型数据的集合。其实数组就是一个容器。
二、好处
可以自动给数组中的元素从0开始编号,方便操作这些元素
三、格式
//一维数组
1,元素类型[] 变量名 = new 元素类型[元素的个数]
int[] arr =
Decode Ways
hcx2013
decode
A message containing letters from A-Z is being encoded to numbers using the following mapping:
'A' -> 1
'B' -> 2
...
'Z' -> 26
Given an encoded message containing digits, det
Spring4.1新特性——异步调度和事件机制的异常处理
jinnianshilongnian
spring 4.1
目录
Spring4.1新特性——综述
Spring4.1新特性——Spring核心部分及其他
Spring4.1新特性——Spring缓存框架增强
Spring4.1新特性——异步调用和事件机制的异常处理
Spring4.1新特性——数据库集成测试脚本初始化
Spring4.1新特性——Spring MVC增强
Spring4.1新特性——页面自动化测试框架Spring MVC T
squid3(高命中率)缓存服务器配置
liyonghui160com
系统:centos 5.x
需要的软件:squid-3.0.STABLE25.tar.gz
1.下载squid
wget http://www.squid-cache.org/Versions/v3/3.0/squid-3.0.STABLE25.tar.gz
tar zxf squid-3.0.STABLE25.tar.gz &&
避免Java应用中NullPointerException的技巧和最佳实践
pda158
java
1) 从已知的String对象中调用equals()和equalsIgnoreCase()方法,而非未知对象。 总是从已知的非空String对象中调用equals()方法。因为equals()方法是对称的,调用a.equals(b)和调用b.equals(a)是完全相同的,这也是为什么程序员对于对象a和b这么不上心。如果调用者是空指针,这种调用可能导致一个空指针异常
Object unk
如何在Swift语言中创建http请求
shoothao
httpswift
概述:本文通过实例从同步和异步两种方式上回答了”如何在Swift语言中创建http请求“的问题。
如果你对Objective-C比较了解的话,对于如何创建http请求你一定驾轻就熟了,而新语言Swift与其相比只有语法上的区别。但是,对才接触到这个崭新平台的初学者来说,他们仍然想知道“如何在Swift语言中创建http请求?”。
在这里,我将作出一些建议来回答上述问题。常见的
Spring事务的传播方式
uule
spring事务
传播方式:
新建事务
required
required_new - 挂起当前
非事务方式运行
supports
&nbs