private static void change(StringBuffer str11, StringBuffer str12) {
str12 = str11;
str11 = new StringBuffer("new world");
str12.append("new world");
}
public static void main(String[] args) {
StringBuffer str1 = new StringBuffer("good ");
StringBuffer str2 = new StringBuffer("bad ");
change(str1, str2);
System.out.println(str1.toString());
System.out.println(str2.toString());
}
问题难度:中等 但是是基础
2、单例模式有几种?请代码实现至少两种。
问题难度:中等
3、sql 内连接的两种写法?
问题难度:简单
4、java数组({“a”,“d”,“b”,“c”,“c”,“d”,“e”,“e”,“e”,“a”})去重,请代码实现。
问题难度:简单
5、如下代码:
public class A {
private static boolean isTrue;
public static synchronized void staticWrite(boolean b) throws InterruptedException{
isTrue = b;
}
public static synchronized boolean staticRead() throws InterruptedException{
return isTrue;
}
public synchronized void write(boolean b) throws InterruptedException{
isTrue = b;
}
public synchronized boolean read() throws InterruptedException{
return isTrue;
}
问:
1)线程1访问A.staticWrite(true)时,线程2能否访问A.staticRead()方法?
2)线程1访问new A().staticWrite(true)时,线程2能否访问A.staticRead()方法?
3)3、A a= new A(),线程1访问a. .staticWrite(true)时,线程2能否访问A.staticRead()方法?
4)4、A a= new A(),A a1 = new A(),线程 1访问a.write(true)时,线程2能否访问a1.read()?
5)4、A a= new A()线程 1访问a.write(true)时,线程2能否访问a.read()?
问题难度:中等
6、如下代码是否存在问题? 请说明?
public void retrieveObjectById(Long id){
try{
//…抛出 IOException 的代码调用
//…抛出 SQLException 的代码调用
}catch(Exception e){
throw new RuntimeException(“Exception in retieveObjectById”, e);
}
}
问题难度:中等
7、 有以下三张表(学生表、课程表、成绩表),请写出对应的sql(考察点:sql)
~~~sql
Student 学生表
CREATE TABLE student (
sid varchar(10) NOT NULL,
sName varchar(20) DEFAULT NULL,
sAge datetime DEFAULT ‘1980-10-12 23:12:36’,
sSex varchar(10) DEFAULT NULL,
PRIMARY KEY (sid)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Course 课程表
CREATE TABLE course (
cid varchar(10) NOT NULL,
cName varchar(10) DEFAULT NULL,
tid int(20) DEFAULT NULL,
PRIMARY KEY (cid)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
SC 成绩表
CREATE TABLE sc (
sid varchar(10) DEFAULT NULL,
cid varchar(10) DEFAULT NULL,
score int(10) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
问题:
1、查询“001”课程比“002”课程成绩高的所有学生的学号;
2、查询平均成绩大于60分的同学的学号和平均成绩;
3、查询所有同学的学号、姓名、选课数、总成绩;
问题难度:中等
答案解析:
1. 如图:
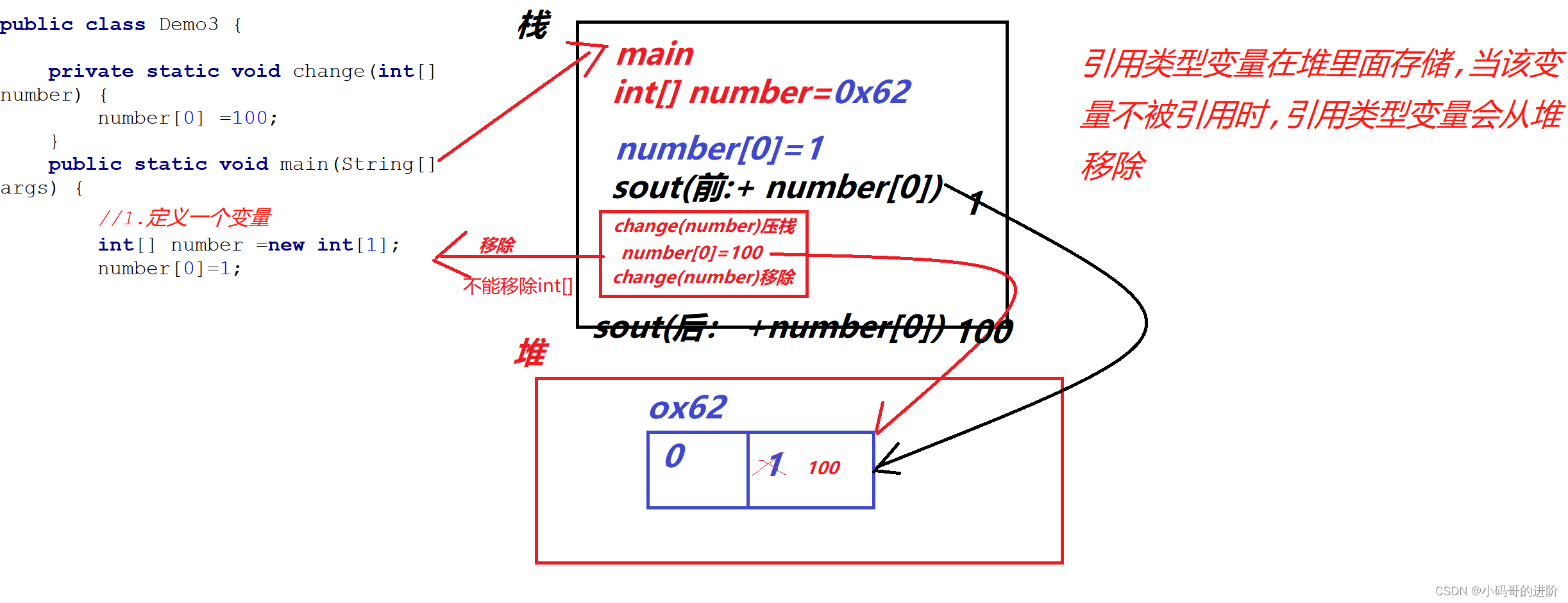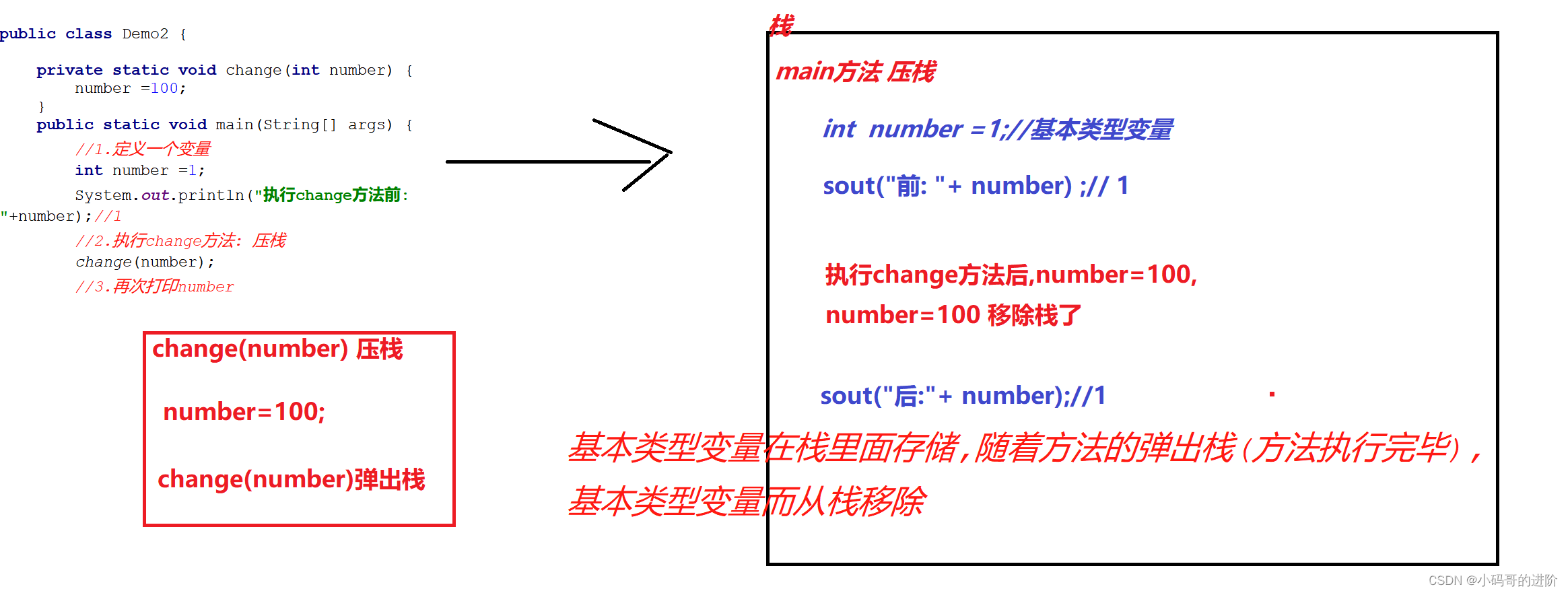
2.问题2:
~~~java
package cn.tedu;
import sun.applet.Main;
/**
* 单列: 在java内存中, 只有一个对象
* 单列模式的规则:
* 1.不能在类的外部随意new对象
* 构造方法私有化
* 2.对外提供一个方法获取单列对象
* 对外提供的方法必须是静态方法,获取单列对象
*/
public class Demo4 {
public static void main(String[] args) {
SingeTon s1 = SingeTon.getSingeTon();
System.out.println(s1.hashCode());
SingeTon s2 = SingeTon.getSingeTon();
System.out.println(s2.hashCode());
SingeTon s3 = SingeTon.getSingeTon();
System.out.println(s3.hashCode());
}
}
class SingeTon{
//0.定义一个私有的静态的对象: 饿汗式, 立即创建对象
private static SingeTon s = new SingeTon();
//1.私有化构造方法
private SingeTon(){
System.out.println("创建对象");
}
//2.对外提供一个静态方法获取单列对象
public static SingeTon getSingeTon(){
return s;
}
}
package cn.tedu;
/**
* 单列: 在java内存中, 只有一个对象
* 单列模式的规则:
* 1.不能在类的外部随意new对象
* 构造方法私有化
* 2.对外提供一个方法获取单列对象
* 对外提供的方法必须是静态方法,获取单列对象
*/
public class Demo5 {
public static void main(String[] args) {
SingeTon2 s1 = SingeTon2.getSingeTon();
System.out.println(s1.hashCode());
SingeTon2 s2 = SingeTon2.getSingeTon();
System.out.println(s2.hashCode());
SingeTon2 s3 = SingeTon2.getSingeTon();
System.out.println(s3.hashCode());
}
}
/**
* 单列模式: 线程安全问题.
* 常见的线程安全问题:
* 当多个用户(多线程)同时访问单列类,如果单利类存在成员变量,
* 可能多个用户同时拿到s时,就出现了线程安全问题了.
* 比如: 五个男孩--->同时爱上一个女孩s
*/
class SingeTon2{
//0.定义一个私有的静态的对象, 懒汉式: 用到了在创建
private static SingeTon2 s =null;
//1.私有化构造方法
private SingeTon2(){
System.out.println("创建对象");
}
//2.对外提供一个静态方法获取单列对象
//静态方法的锁对象: 类本身, 就是类的字节码class
//一个类A.java------>只有一个:A.class
public synchronized static SingeTon2 getSingeTon(){
if(s==null){
s = new SingeTon2();
}
return s;
}
}
5.问题5:
package cn.tedu;
/**
* 1.普通方法的锁对象是谁?: 类的实例化对象(this也可以)
* 那个实例对象调用普通方法,那么普通方法的锁对象就是那个实例对象
* 2.静态方法的锁对象是谁?: 当前类(class)
* 不管怎么调用静态方法,静态方法锁对象依然是当前类(class)
*/
public class A {
//成员变量: 会引起线程安全
private static boolean isTrue;
public static synchronized void staticWrite(boolean b) throws InterruptedException {
isTrue = b;
}
public static synchronized boolean staticRead() throws InterruptedException {
return isTrue;
}
public synchronized void write(boolean b) throws InterruptedException {
isTrue = b;
}
public synchronized boolean read() throws InterruptedException {
return isTrue;
}
}
7.sql语句
1.查询“001”课程比“002”课程成绩高的所有学生的学号;
SELECT DISTINCT s1.sid
FROM sc s1, sc s2
WHERE (s1.cid=‘001’ AND s2.cid=‘002’) AND (s1.score>s2.score);
2、查询平均成绩大于60分的同学的学号和平均成绩;
SELECT s.sid,AVG(c.score)
FROM student s, sc c
WHERE s.sid = c.sid
GROUP BY s.sid
HAVING AVG(c.score)>60;
3、查询所有同学的学号、姓名、选课数、总成绩;
SELECT COUNT(c.cid) AS 选课数,c.sid 学号, s.sName
,SUM(c.score)
FROM sc c, student s
WHERE c.sid
= s.sid
GROUP BY c.sid