图像的几何变换包括图像缩放、平移、旋转、仿射变换、透视变换。
代码
import cv2
import numpy as np
from matplotlib import pyplot as plt
src1 = cv2.imread(r'F:\OPENCV\Opencv\flower.jfif', cv2.IMREAD_COLOR)
src2 = cv2.imread(r'F:\OPENCV\Opencv\test4.png', cv2.IMREAD_COLOR)
src = cv2.cvtColor(src1, cv2.COLOR_BGR2RGB)
src0 = cv2.cvtColor(src2, cv2.COLOR_BGR2RGB)
img1 = cv2.resize(src, (500, 500), cv2.INTER_NEAREST)
img2 = cv2.resize(src, (500, 500), cv2.INTER_LINEAR)
img3 = cv2.resize(src, (500, 500), cv2.INTER_CUBIC)
img4 = cv2.resize(src, (500, 500), cv2.INTER_AREA)
img5 = cv2.resize(src, (500, 500), cv2.INTER_LANCZOS4)
plt.rcParams['font.sans-serif'] = ['SimHei']
titles = ['src', '最近邻插值', '双线性插值', '立方插值', '区域插值', 'Lanczos4']
images = [src, img1, img2, img3, img4, img5]
plt.figure(1, figsize=(6, 6))
for i in range(len(images)):
plt.subplot(2, 3, i + 1)
plt.imshow(images[i], 'gray')
plt.title(titles[i])
plt.xticks([])
plt.yticks([])
plt.savefig(r'F:\OPENCV\Opencv\flower.jpg', dpi=200)
M1 = np.array([[1, 0, 100],
[0, 1, 100]]).astype(np.float)
translation = cv2.warpAffine(src0, M1, (src0.shape[1], src0.shape[0]),
borderMode=cv2.BORDER_CONSTANT, borderValue=[0, 0, 255])
M2 = cv2.getRotationMatrix2D((src0.shape[1] / 2, src0.shape[0] / 2), angle=90, scale=1)
print(M1)
dst = cv2.warpAffine(src0, M2, (src0.shape[1], src0.shape[0]),
borderMode=cv2.BORDER_CONSTANT, borderValue=[0, 0, 255])
points1 = np.float32([[40, 40],
[160, 40],
[40, 160]])
points2 = np.float32([[20, 40],
[160, 40],
[40, 160]])
M3 = cv2.getAffineTransform(points1, points2)
dst1 = cv2.warpAffine(src0, M3, (src0.shape[1], src0.shape[0]))
points3 = np.float32([[40, 40],
[160, 40],
[40, 160],
[160, 160]])
points4 = np.float32([[20, 40],
[160, 40],
[40, 160],
[160, 160]])
M4 = cv2.getPerspectiveTransform(points3, points4)
dst2 = cv2.warpPerspective(src0, M4, (src0.shape[1], src0.shape[0]))
titles1 = ['src0', '平移', '旋转', '仿射变换', '透视变换']
images1 = [src0, translation, dst, dst1, dst2]
plt.figure(2, figsize=(6, 6))
for i in range(len(images1)):
plt.subplot(2, 3, i + 1)
plt.imshow(images1[i], 'gray')
plt.title(titles1[i])
plt.xticks([])
plt.yticks([])
plt.show()
结果图像
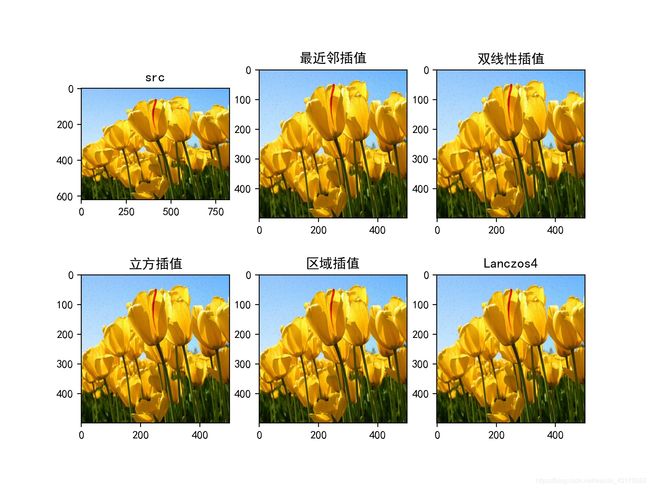
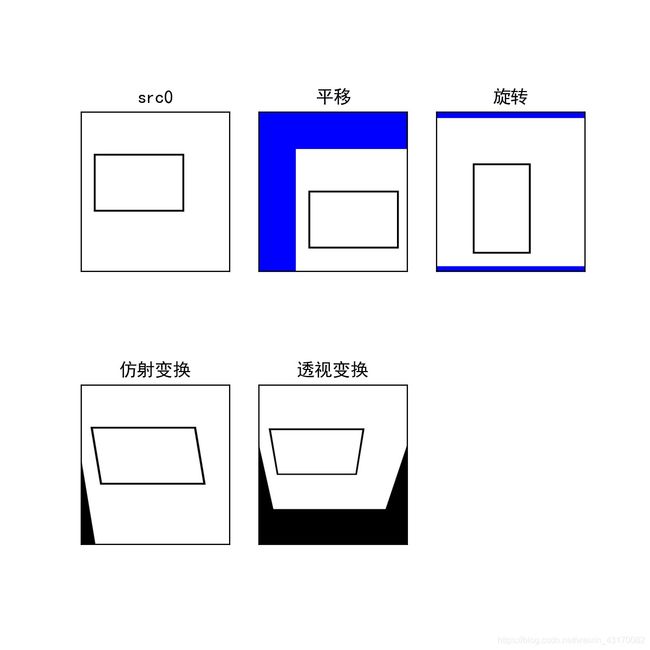