文章目录
- 1. JDBC 基本概念
-
- 1.1 什么是 JDBC ?
- 1.2 JDBC 能干什么?
- 1.3 安装 MySQL
- 2. JDBC 数据库编程的基本步骤
-
- 2.1 将驱动程序导入到工程,在程序中加载驱动
-
- 2.1.1 下载 JDBC 驱动
- 2.1.2 加载驱动程序
- 2.1.3 不同数据库的 JDBC 驱动的类名
- 2.2 创建连接对象 Connection
-
- 2.2.1 解释 getConnection()函数
- 2.2.2 连接数据库
- 2.3 在连接对象上创建命令对象 Statement
-
- 2.3.1 解释原因
- 2.3.2 创建命令对象
- 2.3.3 Statement 类提供的常用方法
- 2.4 执行 SQL 语句
-
- 2.4.1 简单示例
- 2.4.2 ResultSet 接口常用的方法
- 2.5 关闭连接
- 3. 完整示例
-
- 3.1 源码
- 3.2 控制台输出
- 3.3 MySQL输出
1. JDBC 基本概念
1.1 什么是 JDBC ?
- JDBC 是Java DataBase Connectivity 的缩写。
- 是一种可用来执行 SQL 语句的 Java API。
- 通过使用 JDBC, 开发人员可以很方便的将 SQL 语句传送给几乎任何一种数据库。
1.2 JDBC 能干什么?
- 同一个数据库建立连接。
- 向数据库发送 SQL 语句。
- 处理数据库返回的结果。
1.3 安装 MySQL
- MySQL 与 Java 是好朋友。
- 安装要自己琢磨。
- 我假设现在你已经安装起了 MySQL。
2. JDBC 数据库编程的基本步骤
2.1 将驱动程序导入到工程,在程序中加载驱动
2.1.1 下载 JDBC 驱动
- 登入官网
- 下载 “mysql-connector-java-xxx-bin.jar”
- 记得选择:平台无关
- 得到的压缩包,解压得到第二部的 jar 文件
- 与导入 jdom 一样的操作,在 XML 编程那一篇 blog 上。
- 简单来说就是 构建路径。
- 最后如果有 modul-info.java, 写入requires java.sql;
- 成功。
2.1.2 加载驱动程序
String driver = "com.mysql.jdbc.Driver";
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
2.1.3 不同数据库的 JDBC 驱动的类名
String driver = "oracle.jdbc.driver.OracleDriver"
String driver = "com.microsoft.sqlserver.jdbc.SQLServerDriver"
String driver = "com.mysql.jdbc.Driver"
2.2 创建连接对象 Connection
2.2.1 解释 getConnection()函数
public static Connection getConnection(String url, String user, String password)
throws SQLException {}
建立到给定数据库 URL 的连接。user 是用户名, password 是用户的密码。
- url
url = "jdbc:subprotocol:data source identifier"
subprotocol: 表示与数据库系统相关的子协议。
data source identifier: 表示数据源信息。
- 例如:
url = "jdbc:mysql://127.0.0.1:3306/mydb"
- 格式为:
"jdbc:mysql://安装数据库主机的IP地址:端口/已建立的数据库名"
- 本机:
如果是在本机,IP地址可用 localhost 代替。
2.2.2 连接数据库
String url = "jdbc:mysql://localhost:3306/students";
String user = "root";
String passwd = "123456";
Connection con = null;
try {
con = DriverManager.getConnection(url, user, passwd);
} catch (SQLException e) {
e.printStackTrace();
}
2.3 在连接对象上创建命令对象 Statement
2.3.1 解释原因
- 创建命令对象 Statement,通过 Statement 类提供的方法。
- 可以利用标准的 SQL 命令,对数据库直接进行增删查改。
2.3.2 创建命令对象
Statement cmd = null;
try {
cmd = con.createStatement();
} catch (SQLException e) {
e.printStackTrace();
}
2.3.3 Statement 类提供的常用方法
boolean execute(String sql) throws SQLException {}
- 执行 INSERT, UPDATE, DELETE 语句等
int executeUpdate(String sql) throws SQLException {}
ResultSet executeQuery(String sql) throws SQLException {}
2.4 执行 SQL 语句
2.4.1 简单示例
ResultSet rs = null;
try {
rs = cmd.executeQuery("select * from customers");
rs.getString(1);
} catch (SQLException e) {
e.printStackTrace();
}
ResultSet rs = null;
String sql = "select * from students";
try {
rs = cmd.executeQuery(sql);
while(rs.next()) {
String sno = rs.getString("sno");
String sname = new String(rs.getString(2).getBytes("iso-8859-1"), "gb2312");
String ssex = new String(rs.getString("ssex").getBytes("iso-8859-1"), "gb2312");
System.out.printf("%-8s%-6s%-3s\n", sno, sname, ssex);
}
} catch (SQLException e) {
e.printStackTrace();
}
2.4.2 ResultSet 接口常用的方法
- String getString(int columnIndex) throws SQLException
- 获取此 ResultSet 对象当前行中指定列的值。
- 参数 columnIndex 代表字段的索引位置。
- 例如:ResultSet.getString(2) : 获取当前行的第2列
- String getString(String columnLabel) throws SQLException
- 获取此 ResultSet 对象当前行中指定列的值。
- 参数 columnLabel 代表字段值。
- int getInt(int columnIndex) throws SQLException
- 获取此 ResultSet 对象当前行中指定列的值。
- 参数 columnIndex 代表字段的索引位置。
- int getInt(String columnLabel) throws SQLException
- 获取此 ResultSet 对象当前行中指定列的值。
- 参数 columnLabel 代表字段值。
- boolean absolute(int row) throws SQLException
- 将游标移动到此 ResultSet 对象的给定行编号。
- boolean previous() throws SQLException
- 将游标移动到此 ResultSet 对象的的上一行。
- first(),第一行
- last(),最后一行
- next(),下一行
2.5 关闭连接
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
3. 完整示例
3.1 源码
package javaweb.database;
import java.io.UnsupportedEncodingException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class JDBC {
public static void readMySQL() throws Exception {
String driver = "com.mysql.jdbc.Driver";
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String passwd = "123456";
Connection con = null;
try {
con = DriverManager.getConnection(url, user, passwd);
} catch (SQLException e) {
e.printStackTrace();
}
Statement cmd = null;
try {
cmd = con.createStatement();
} catch (SQLException e) {
e.printStackTrace();
}
ResultSet rs = null;
String sql = "select * from students";
try {
rs = cmd.executeQuery(sql);
while (rs.next()) {
String sno = rs.getString("sno");
String sname = rs.getString(2);
String ssex = rs.getString("ssex");
System.out.printf("%-8s%-6s%-3s\n", sno, sname, ssex);
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void writeAndRead() throws UnsupportedEncodingException {
Connection con = null;
Statement cmd = null;
ResultSet rs = null;
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "root";
String passwd = "123456";
try {
Class.forName("com.mysql.jdbc.Driver");
con = DriverManager.getConnection(url, user, passwd);
cmd = con.createStatement();
String sql1 = "insert into students(sno, sname, ssex) values(\"1004\", \"张三\", \"男\");";
cmd.executeUpdate(sql1);
String sql2 = "select * from students";
rs = cmd.executeQuery(sql2);
while (rs.next()) {
String sno = rs.getString("sno");
String sname = rs.getString(2);
String ssex = rs.getString("ssex");
System.out.printf("%-8s%-6s%-3s\n", sno, sname, ssex);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
rs = null;
}
if (cmd != null) {
cmd.close();
cmd = null;
}
if (con != null) {
con.close();
con = null;
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
try {
readMySQL();
} catch (Exception e) {
e.printStackTrace();
}
}
}
3.2 控制台输出
1001 李晨 男
1002 王丽 女
1003 陈军 男
1004 张三 男
3.3 MySQL输出
插入前:
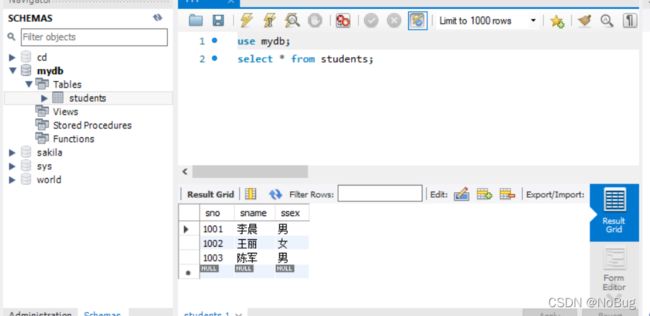
插入后:
