文章目录
- 一、需求说明
- 二、软件设计结构
- 三、设计
-
- 1、创建项目基本组件
- 2、Equipment接口及其子类的设计
- 3、实现Service包中的类(重要)
-
- NameListService
- TeamSeivice
- 4、TeamView
一、需求说明
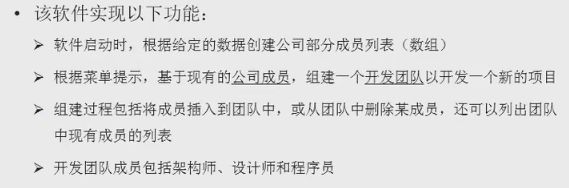
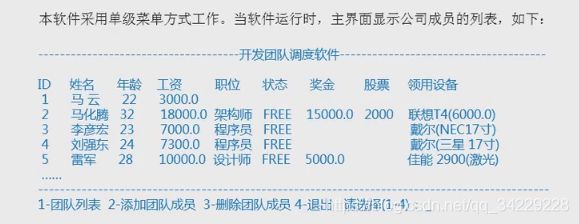
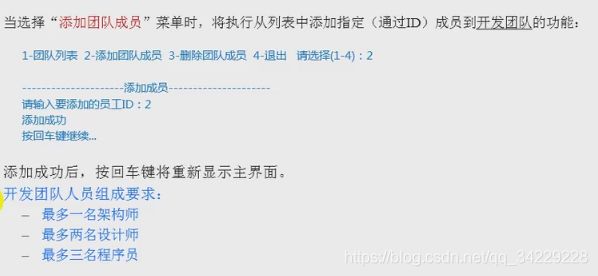

二、软件设计结构
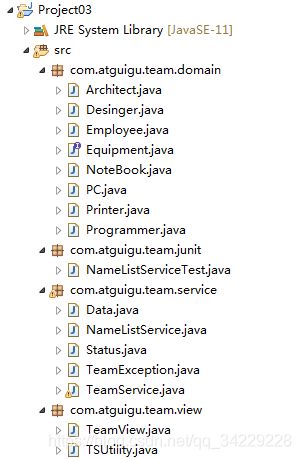
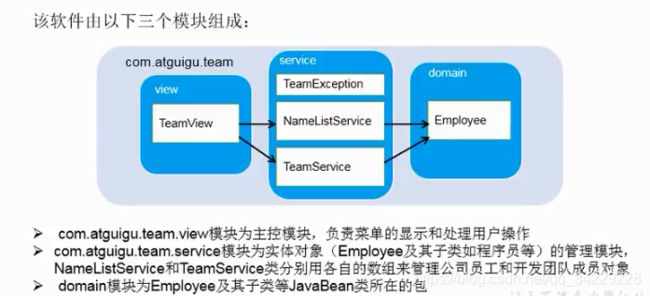
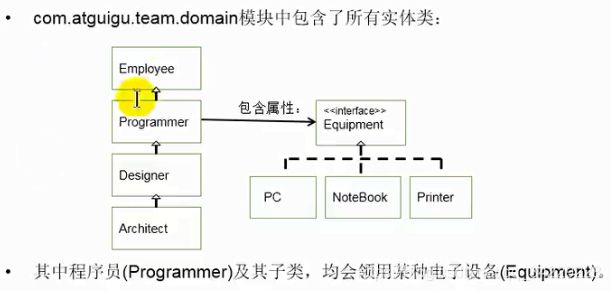
三、设计
1、创建项目基本组件
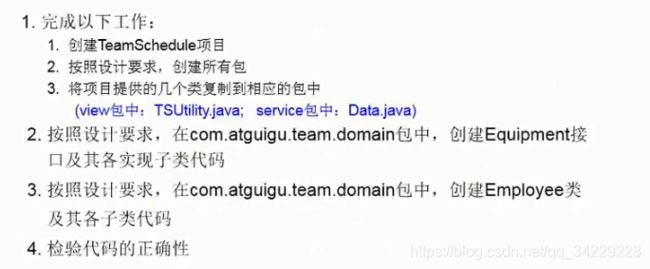
- 1)键盘访问的实现
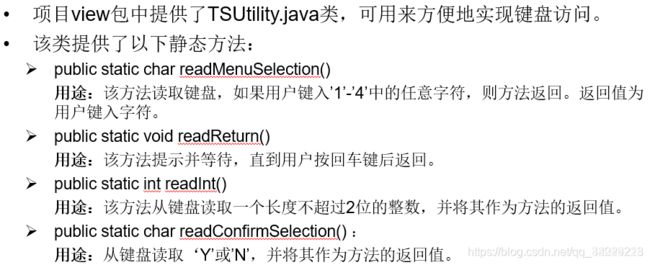
package com.atguigu.team.view;
import java.util.*;
public class TSUtility {
private static Scanner scanner = new Scanner(System.in);
public static char readMenuSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '1' && c != '2' &&
c != '3' && c != '4') {
System.out.print("选择错误,请重新输入:");
} else break;
}
return c;
}
public static void readReturn() {
System.out.print("按回车键继续...");
readKeyBoard(100, true);
}
public static int readInt() {
int n;
for (; ; ) {
String str = readKeyBoard(2, false);
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
public static char readConfirmSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false).toUpperCase();
c = str.charAt(0);
if (c == 'Y' || c == 'N') {
break;
} else {
System.out.print("选择错误,请重新输入:");
}
}
return c;
}
private static String readKeyBoard(int limit, boolean blankReturn) {
String line = "";
while (scanner.hasNextLine()) {
line = scanner.nextLine();
if (line.length() == 0) {
if (blankReturn) return line;
else continue;
}
if (line.length() < 1 || line.length() > limit) {
System.out.print("输入长度(不大于" + limit + ")错误,请重新输入:");
continue;
}
break;
}
return line;
}
}
package com.atguigu.team.service;
public class Data {
public static final int EMPLOYEE = 10;
public static final int PROGRAMMER = 11;
public static final int DESIGNER = 12;
public static final int ARCHITECT = 13;
public static final int PC = 21;
public static final int NOTEBOOK = 22;
public static final int PRINTER = 23;
public static final String[][] EMPLOYEES = {
{"10", "1", "马云", "22", "3000"},
{"13", "2", "马化腾", "32", "18000", "15000", "2000"},
{"11", "3", "李彦宏", "23", "7000"},
{"11", "4", "刘强东", "24", "7300"},
{"12", "5", "雷军", "28", "10000", "5000"},
{"11", "6", "任志强", "22", "6800"},
{"12", "7", "柳传志", "29", "10800","5200"},
{"13", "8", "杨元庆", "30", "19800", "15000", "2500"},
{"12", "9", "史玉柱", "26", "9800", "5500"},
{"11", "10", "丁磊", "21", "6600"},
{"11", "11", "张朝阳", "25", "7100"},
{"12", "12", "杨致远", "27", "9600", "4800"}
};
public static final String[][] EQIPMENTS = {
{},
{"22", "联想T4", "6000"},
{"21", "戴尔", "NEC17寸"},
{"21", "戴尔", "三星 17寸"},
{"23", "激光", "佳能 2900"},
{"21", "华硕", "三星 17寸"},
{"21", "华硕", "三星 17寸"},
{"23", "针式", "爱普生20K"},
{"22", "惠普m6", "5800"},
{"21", "戴尔", "NEC 17寸"},
{"21", "华硕","三星 17寸"},
{"22", "惠普m6", "5800"}
};
}
2、Equipment接口及其子类的设计
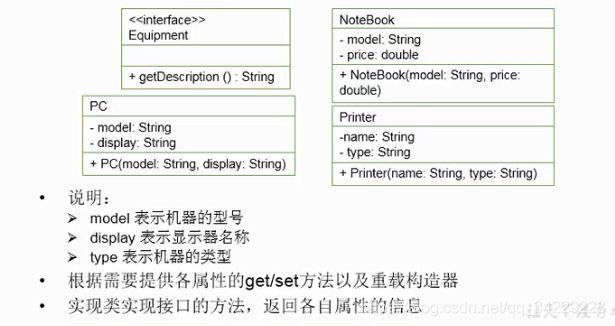
package com.atguigu.team.domain;
public interface Equipment {
public abstract String getDescription();
}
package com.atguigu.team.domain;
public class NoteBook implements Equipment{
private String model;
private double price;
public NoteBook() {
super();
}
public NoteBook(String model, double price) {
this.model = model;
this.price = price;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String getDescription() {
return model + "(" + price + ")";
}
}
package com.atguigu.team.domain;
public class PC implements Equipment {
private String model;
private String display;
public PC() {
super();
}
public PC(String model, String display) {
this.model = model;
this.display = display;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getDisplay() {
return display;
}
public void setDisplay(String display) {
this.display = display;
}
@Override
public String getDescription() {
return model + "(" + display + ")";
}
}
package com.atguigu.team.domain;
public class Printer implements Equipment {
private String name;
private String type;
public Printer() {
super();
}
public Printer(String name, String type) {
this.name = name;
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public String getDescription() {
return name + "(" + type + ")";
}
}
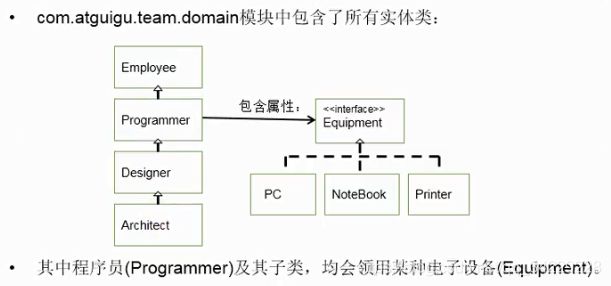
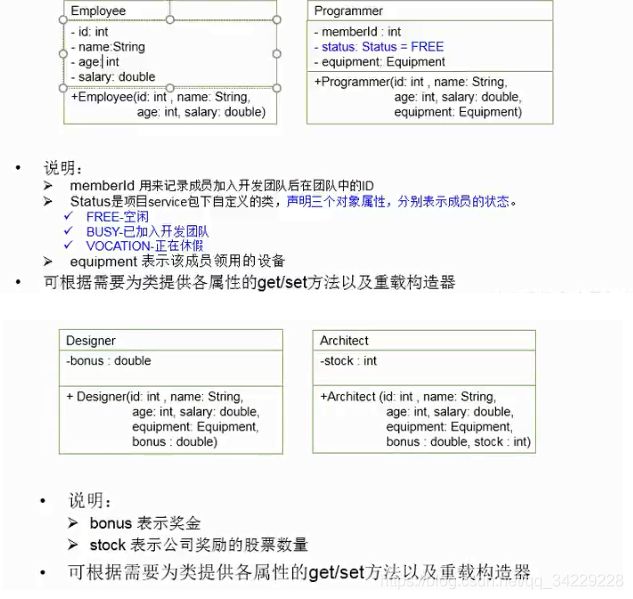
package com.atguigu.team.domain;
public class Employee {
private int id;
private String name;
private int age;
private double salary;
public Employee() {
}
public Employee(int id, String name, int age, double salary) {
super();
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public String getDetails() {
return id+"\t"+name+"\t"+age+"\t"+salary;
}
@Override
public String toString() {
return getDetails();
}
}
package com.atguigu.team.domain;
import com.atguigu.team.service.Status;
public class Programmer extends Employee {
@Override
public String toString() {
return super.getDetails() + "\t程序员\t" + status + "\t\t\t" + equipment.getDescription();
}
private int memberId;
private Status status = Status.FREE;
private Equipment equipment;
public Programmer() {
}
public Programmer(int id, String name, int age, double salary, Equipment equipment) {
super(id, name, age, salary);
this.equipment = equipment;
}
public int getMemberId() {
return memberId;
}
public void setMemberId(int memberId) {
this.memberId = memberId;
}
public Status getStatus() {
return status;
}
public void setStatus(Status status) {
this.status = status;
}
public Equipment getEquipment() {
return equipment;
}
public void setEquipment(Equipment equipment) {
this.equipment = equipment;
}
}
package com.atguigu.team.domain;
public class Desinger extends Programmer {
@Override
public String toString() {
return super.getDetails() + "\t设计师\t" + getStatus() + "\t" + bonus + "\t\t" + getEquipment();
}
private double bonus;
public Desinger() {
}
public Desinger(int id, String name, int age, double salary, Equipment equipment, double bonus) {
super(id, name, age, salary, equipment);
this.bonus = bonus;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
}
package com.atguigu.team.domain;
public class Architect extends Desinger {
private int stock;
public Architect() {
}
public Architect(int id, String name, int age, double salary, Equipment equipment, double bonus, int stock) {
super(id, name, age, salary, equipment, bonus);
this.stock = stock;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
@Override
public String toString() {
return super.getDetails() + "\t架构师\t" + getStatus() + "\t" + getBonus() + "\t" + stock + "" + getEquipment();
}
}
package com.atguigu.team.service;
public class Status {
private final String NAME;
private Status(String name) {
this.NAME = name;
};
public static final Status FREE = new Status("FREE");
public static final Status BUSY = new Status("BUSY");
public static final Status VOCATION = new Status("VOCATION");
public String getNAME() {
return NAME;
}
}
3、实现Service包中的类(重要)
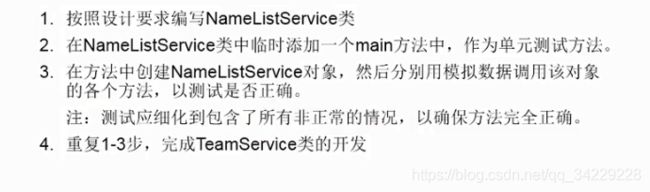
NameListService
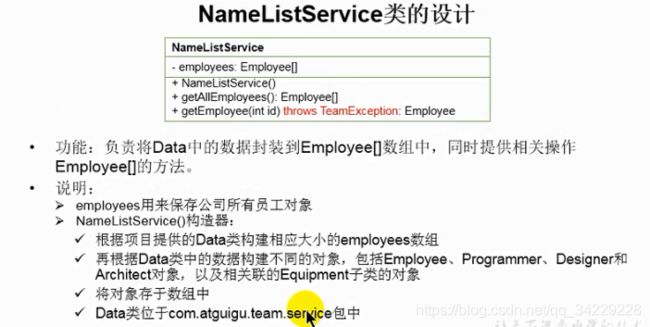

package com.atguigu.team.service;
import com.atguigu.team.domain.Architect;
import com.atguigu.team.domain.Desinger;
import com.atguigu.team.domain.Employee;
import com.atguigu.team.domain.Equipment;
import com.atguigu.team.domain.NoteBook;
import com.atguigu.team.domain.PC;
import com.atguigu.team.domain.Printer;
import com.atguigu.team.domain.Programmer;
import static com.atguigu.team.service.Data.*;
public class NameListService {
private Employee[] employees;
public NameListService() {
employees = new Employee[EMPLOYEES.length];
for(int i=0;i< EMPLOYEES.length;i++) {
int type = Integer.parseInt(EMPLOYEES[i][0]);
int id = Integer.parseInt(Data.EMPLOYEES[i][1]);
String name = Data.EMPLOYEES[i][2];
int age = Integer.parseInt(Data.EMPLOYEES[i][3]);
Double salary = Double.parseDouble(Data.EMPLOYEES[i][4]);
Equipment e ;
switch(type) {
case EMPLOYEE:
employees[i] = new Employee(id, name, age, salary);
break;
case PROGRAMMER:
e = createEquipment(i);
employees[i] = new Programmer(Integer.parseInt(EMPLOYEES[i][1]), EMPLOYEES[i][2], Integer.parseInt(EMPLOYEES[i][3]), Double.parseDouble(EMPLOYEES[i][4]), e);
break;
case DESIGNER:
e = createEquipment(i);
employees[i] = new Desinger(id, name, age, salary, e, Double.parseDouble(EMPLOYEES[i][5]));
break;
case ARCHITECT:
e = createEquipment(i);
employees[i] = new Architect(id, name, age, salary, e, Double.parseDouble(EMPLOYEES[i][5]), Integer.parseInt(EMPLOYEES[i][6]));
break;
}
}
}
private Equipment createEquipment(int i) {
int type = Integer.parseInt(EQUIPMENTS[i][0]);
switch (type) {
case PC:
return new PC(Data.EQUIPMENTS[i][1], Data.EQUIPMENTS[i][2]);
case NOTEBOOK:
return new NoteBook(Data.EQUIPMENTS[i][1], Double.parseDouble(Data.EQUIPMENTS[i][2]));
case PRINTER:
return new Printer(Data.EQUIPMENTS[i][1], Data.EQUIPMENTS[i][2]);
}
return null;
}
public Employee[] getAllEmployees() {
return employees;
}
public Employee getEmployee(int id) throws TeamException {
for(int i=0;i<employees.length;i++) {
if(employees[i].getId()==id) {
return employees[i];
}
}
throw new TeamException("添加失败,不存在这个员工");
}
}
TeamSeivice
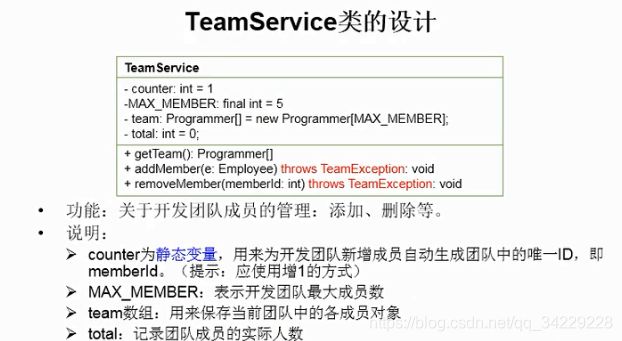
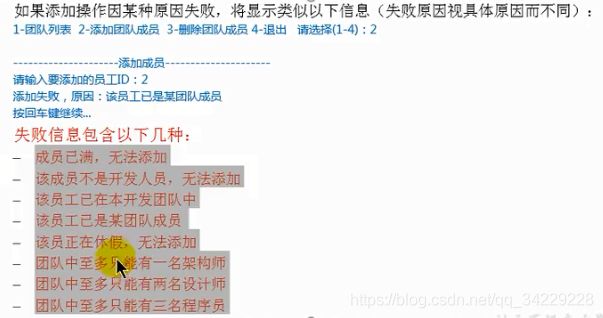
package com.atguigu.team.service;
import org.hamcrest.core.IsInstanceOf;
import org.hamcrest.core.IsNot;
import com.atguigu.team.domain.Architect;
import com.atguigu.team.domain.Desinger;
import com.atguigu.team.domain.Employee;
import com.atguigu.team.domain.Programmer;
import sun.jvm.hotspot.debugger.SymbolLookup;
public class TeamService {
private static int counter = 1;
private final int MAX_MEMBER = 5;
private Programmer[] team = new Programmer[MAX_MEMBER];
private int total = 0;
public TeamService() {
}
public Programmer[] getTeam() {
Programmer[] teamNow = new Programmer[total];
for (int i = 0; i < total; i++) {
teamNow[i] = team[i];
}
return teamNow;
}
public void addMember(Employee e) throws TeamException {
if (total >= MAX_MEMBER) {
throw new TeamException("成员已满,无法添加!");
}
if (!(e instanceof Programmer)) {
throw new TeamException("该成员不是开发人员,无法添加!");
}
if (isExist(e)) {
throw new TeamException("该成员已在本开发团队中,无法添加!");
}
Programmer e1 = (Programmer) e;
if ("BUSY".equals(e1.getStatus().getNAME())) {
throw new TeamException("该成员已是某团队成员,无法添加!");
}
if ("VOCATION".equals(e1.getStatus().getNAME())) {
throw new TeamException("该成员正在休假,无法添加!");
}
int numOfArch = 0, numOfDes = 0, numOfPro = 0;
for (int i = 0; i < total; i++) {
if (team[i] instanceof Architect) {
numOfArch++;
} else if (team[i] instanceof Desinger) {
numOfDes++;
} else {
numOfPro++;
}
}
if (e1 instanceof Architect) {
if (numOfArch >= 1) {
throw new TeamException("团队只能有一名架构师,无法添加!");
}
} else if (e1 instanceof Desinger) {
if (numOfDes >= 2) {
throw new TeamException("团队只能有2名设计,无法添加!");
}
} else if (e1 instanceof Desinger) {
if (numOfPro >= 3) {
throw new TeamException("团队只能有3名程序员,无法添加!");
}
}
team[total++] = e1;
e1.setStatus(Status.BUSY);
e1.setMemberId(counter++);
}
public boolean isExist(Employee e) {
for (int i = 0; i < total; i++) {
if (e.getId() == getTeam()[i].getId()) {
return true;
}
}
return false;
}
public void removeMember(int memberId) throws TeamException {
int i = 0;
boolean flag = true;
for (; i < total; i++) {
if (team[i].getMemberId() == memberId) {
team[i].setStatus(Status.FREE);
flag = false;
break;
}
}
if (flag) {
throw new TeamException("团队没有该成员,删除失败!");
}
for (int j = i + 1; j < total; j++) {
team[j - 1] = team[j];
}
team[--total] = null;
}
}
4、TeamView
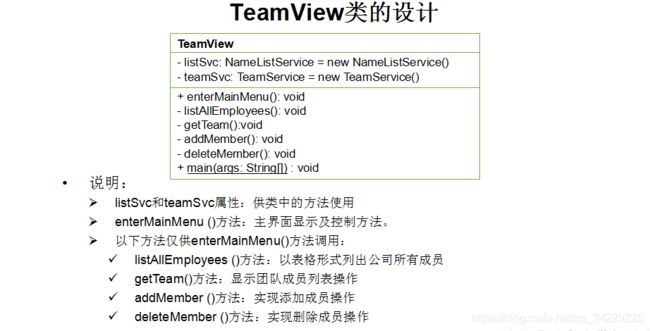
package com.atguigu.team.view;
import com.atguigu.team.domain.Employee;
import com.atguigu.team.domain.Programmer;
import com.atguigu.team.service.NameListService;
import com.atguigu.team.service.TeamException;
import com.atguigu.team.service.TeamService;
public class TeamView {
private NameListService listSvc = new NameListService();
private TeamService teamSvc = new TeamService();
public void enterMainMenu() {
boolean flag = true;
char menu = 0;
while (flag) {
if(menu != '1') {
listAllEmployees();}
System.out.print("1-团队列表 2-添加团队成员 3-删除团队成员 4-退出 请选择(1-4):");
menu = TSUtility.readMenuSelection();
switch (menu) {
case '1':
getTeam();
break;
case '2':
addMember();
break;
case '3':
deleteMember();
break;
case '4':
System.out.println("是否确认退出(y/n)");
char isExit = TSUtility.readConfirmSelection();
if(isExit == 'Y') {
flag = false;
}
break;
}
}
}
private void listAllEmployees() {
System.out.println("\n-------------------------------开发团队调度软件--------------------------------\n");
Employee[] employees = listSvc.getAllEmployees();
if(employees==null||employees.length == 0) {
System.out.println("没有员工信息");
}else {
System.out.println("ID\t姓名\t年龄\t工资\t职位\t状态\t奖金\t股票\t领用设备");
for (int i = 0; i < employees.length; i++) {
System.out.println(employees[i]);
}
}
System.out.println("-------------------------------------------------------------------------------");
}
private void getTeam() {
System.out.print("\n-------------------------------团队成员列表--------------------------------\n");
Programmer[] team = teamSvc.getTeam();
if(team == null||team.length == 0) {
System.out.println("开发团队目前没有成员信息");
}else {
System.out.println("TID/ID\t姓名\t年龄\t工资\t职位\t奖金\t股票");
for (int i = 0; i < team.length; i++) {
System.out.println(team[i].getDetailsForTeam());
}
}
System.out.println("-------------------------------------------------------------------------------");
}
private void addMember() {
System.out.print("\n-------------------------------添加成员--------------------------------\n");
System.out.println("请输入要添加员工的id:");
int id = TSUtility.readInt();
Employee emp = null;
try {
emp = listSvc.getEmployee(id);
} catch (TeamException e) {
e.getMessage();
}
try {
teamSvc.addMember(emp);
System.out.println("添加成功");
} catch (TeamException e) {
System.out.println(e.getMessage());
}
TSUtility.readReturn();
}
private void deleteMember() {
System.out.print("\n-------------------------------删除成员--------------------------------\n");
System.out.println("请输入要删除员工的TID:");
int id = TSUtility.readInt();
System.out.println("是否确认删除(y/n)");
char isDelete = TSUtility.readConfirmSelection();
if(isDelete=='Y') {
try {
teamSvc.removeMember(id);
System.out.println("删除成功");
} catch (TeamException e) {
System.out.println("删除失败:"+e.getMessage());
}
TSUtility.readReturn();
}
}
public static void main(String[] args) {
TeamView view = new TeamView();
view.enterMainMenu();
}
}