springBoot集成华为云obs对象存储
一、准备工作:华为云平台注册账号
- 获取 AK、SK
- 获取 endPoint
- 创建桶
二、springBoot项目集成obs服务器
- spring-boot的pom.xml中添加组件【这里使用的是v=3.21.8】
<dependency>
<groupId>com.huaweicloud</groupId>
<artifactId>esdk-obs-java</artifactId>
<version>3.21.8</version>
</dependency>
<!--
必须制定okhttp3的最新版本号,
否则会出现java.lang.NoSuchMethodError: okhttp3.RequestBody.create
-->
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.3</version>
</dependency>
<!--
必须指定与okhttp相匹配的okio的版本,否则会出现okio和okhttp的包冲突问题
java.lang.NoSuchMethodError: kotlin
-->
<dependency>
<groupId>com.squareup.okio</groupId>
<artifactId>okio</artifactId>
<version>2.8.0</version>
</dependency>
- spring-boot的application.properties文件中配置
#华为云obs配置
hwyun.obs.accessKey=****
hwyun.obs.securityKey=***
hwyun.obs.endPoint=***
hwyun.obs.bucketName=***
- 创建对应的HweiOBSConfig参数配置类
package com.elink;
import com.obs.services.ObsClient;
import com.obs.services.exception.ObsException;
import lombok.Data;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import java.text.SimpleDateFormat;
import java.util.Date;
@Data
@Configuration
public class HweiOBSConfig {
private static final Logger log = Logger.getLogger(HweiOBSConfig.class);
@Value("${hwyun.obs.accessKey}")
private String accessKey;
@Value("${hwyun.obs.securityKey}")
private String securityKey;
@Value("${hwyun.obs.endPoint}")
private String endPoint;
@Value("${hwyun.obs.bucketName}")
private String bucketName;
public ObsClient getInstance() {
return new ObsClient(accessKey, securityKey, endPoint);
}
public void destroy(ObsClient obsClient){
try {
obsClient.close();
} catch (ObsException e) {
log.error("obs执行失败", e);
} catch (Exception e) {
log.error("执行失败", e);
}
}
public static String getObjectKey() {
return "Hwei" + "/" + new SimpleDateFormat("yyyy-MM-dd").format(new Date() )+ "/";
}
}
- 创建业务层接口
package com.elink.license.service;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.InputStream;
import java.util.List;
public interface IHweiYunOBSService {
boolean delete(String objectKey);
boolean deleteFileByPath(String objectKey);
boolean delete(List<String> objectKeys);
String fileUpload(MultipartFile uploadFile, String objectKey);
String uploadFileByte(byte data[], String objectKey);
String uploadFile(File file);
InputStream fileDownload(String objectKey);
}
package com.elink.license.service.impl;
import com.elink.HweiOBSConfig;
import com.elink.base.util.PropertiesUtil;
import com.elink.license.service.IHweiYunOBSService;
import com.obs.services.ObsClient;
import com.obs.services.exception.ObsException;
import com.obs.services.model.*;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.UUID;
@Service
public class HweiYunOBSServiceImpl implements IHweiYunOBSService {
private static final Logger log = Logger.getLogger(HweiYunOBSServiceImpl.class);
@Autowired
private HweiOBSConfig hweiOBSConfig;
@Override
public boolean delete(String objectKey) {
ObsClient obsClient = null;
try {
obsClient = hweiOBSConfig.getInstance();
obsClient.deleteObject(hweiOBSConfig.getBucketName(), objectKey);
} catch (ObsException e) {
log.error("obs[delete]删除保存失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return true;
}
@Override
public boolean deleteFileByPath(String path) {
ObsClient obsClient = null;
try {
obsClient = hweiOBSConfig.getInstance();
String file_http_url = PropertiesUtil.readValue("file_http_url");
File file = new File(path);
String key = file.getName();
String realPath = path.replace(file_http_url, "");
realPath = realPath.substring(0, realPath.lastIndexOf('/'));
obsClient.deleteObject(hweiOBSConfig.getBucketName(), realPath + "/" + key);
} catch (ObsException e) {
log.error("obs[deleteFileByPath]删除保存失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return false;
}
@Override
public boolean delete(List<String> objectKeys) {
ObsClient obsClient = null;
try {
obsClient = hweiOBSConfig.getInstance();
DeleteObjectsRequest deleteObjectsRequest = new DeleteObjectsRequest(hweiOBSConfig.getBucketName());
objectKeys.forEach(x -> deleteObjectsRequest.addKeyAndVersion(x));
obsClient.deleteObjects(deleteObjectsRequest);
return true;
} catch (ObsException e) {
log.error("obs[delete]删除保存失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return false;
}
@Override
public String fileUpload(MultipartFile uploadFile, String objectKey) {
ObsClient obsClient = null;
try {
String bucketName = hweiOBSConfig.getBucketName();
obsClient = hweiOBSConfig.getInstance();
boolean exists = obsClient.headBucket(bucketName);
if (!exists) {
HeaderResponse response = obsClient.createBucket(bucketName);
log.info("创建桶成功" + response.getRequestId());
}
InputStream inputStream = uploadFile.getInputStream();
long available = inputStream.available();
PutObjectRequest request = new PutObjectRequest(bucketName, objectKey, inputStream);
ObjectMetadata objectMetadata = new ObjectMetadata();
objectMetadata.setContentLength(available);
request.setMetadata(objectMetadata);
request.setAcl(AccessControlList.REST_CANNED_PUBLIC_READ);
PutObjectResult result = obsClient.putObject(request);
log.info("已上传对象的URL" + result.getObjectUrl());
return result.getObjectUrl();
} catch (ObsException e) {
log.error("obs[fileUpload]上传失败", e);
} catch (IOException e) {
log.error("[fileUpload]上传失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return null;
}
@Override
public String uploadFileByte(byte[] data, String fileName) {
try {
ObsClient obsClient = null;
String bucketName = hweiOBSConfig.getBucketName();
obsClient = hweiOBSConfig.getInstance();
boolean exists = obsClient.headBucket(bucketName);
if (!exists) {
HeaderResponse response = obsClient.createBucket(bucketName);
log.info("创建桶成功" + response.getRequestId());
}
String file_http_url = PropertiesUtil.readValue("file_http_url");
InputStream inputStream = new ByteArrayInputStream(data);
String prefix = fileName.substring(fileName.lastIndexOf(".") + 1);
String uuid = UUID.randomUUID().toString().replace("-", "");
fileName = uuid + "." + prefix;
obsClient.putObject(bucketName, fileName.substring(0, fileName.lastIndexOf("/")) + "/" + fileName, inputStream);
return file_http_url + fileName;
} catch (ObsException e) {
log.error("obs[uploadFileByte]上传失败", e);
}
return null;
}
@Override
public String uploadFile(File file) {
ObsClient obsClient = null;
try {
String bucketName = hweiOBSConfig.getBucketName();
obsClient = hweiOBSConfig.getInstance();
boolean exists = obsClient.headBucket(bucketName);
if (!exists) {
HeaderResponse response = obsClient.createBucket(bucketName);
log.info("创建桶成功" + response.getRequestId());
}
String file_http_url = PropertiesUtil.readValue("file_http_url");
String filename = file.getName();
String prefix = filename.substring(filename.lastIndexOf(".") + 1);
String uuid = UUID.randomUUID().toString().replace("-", "");
String fileName = uuid + "." + prefix;
obsClient.putObject(bucketName, fileName.substring(0, fileName.lastIndexOf("/")) + "/" + fileName, file);
return file_http_url + fileName;
} catch (Exception e) {
log.error("obs[uploadFile]上传失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return null;
}
@Override
public InputStream fileDownload(String objectKey) {
ObsClient obsClient = null;
try {
String bucketName = hweiOBSConfig.getBucketName();
obsClient = hweiOBSConfig.getInstance();
ObsObject obsObject = obsClient.getObject(bucketName, objectKey);
return obsObject.getObjectContent();
} catch (ObsException e) {
log.error("obs[fileDownload]文件下载失败", e);
} finally {
hweiOBSConfig.destroy(obsClient);
}
return null;
}
}
- 添加Controller层
package com.elink.license.controller;
import com.elink.license.service.IHweiYunOBSService;
import com.elink.license.util.StringUtils;
import com.elink.share.result.Result;
import com.elink.share.result.ResultCodeWraper;
import com.obs.services.exception.ObsException;
import org.apache.commons.io.IOUtils;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.List;
import java.util.Map;
import java.util.UUID;
@RestController
@RequestMapping({"file"})
public class HweiYunOBSController {
@Resource
private IHweiYunOBSService hweiYunOBSService;
@RequestMapping(value = "upload", method = RequestMethod.POST)
public Map save(@RequestParam(value = "file", required = false) MultipartFile file) {
if (StringUtils.isEmpty(file)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "文件为空");
}
final String test = hweiYunOBSService.fileUpload(file, file.getOriginalFilename());
return new Result<>(ResultCodeWraper.SUCCESS, test);
}
@RequestMapping(value = "{fileName}/download", method = RequestMethod.POST)
public Map download(HttpServletRequest request, HttpServletResponse response, @PathVariable String fileName) {
if (StringUtils.isEmpty(fileName)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "下载文件为空");
}
try (
InputStream inputStream = hweiYunOBSService.fileDownload(fileName);
BufferedOutputStream outputStream = new BufferedOutputStream(response.getOutputStream())) {
if (inputStream == null) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "内部错误");
}
final String userAgent = request.getHeader("USER-AGENT");
if (userAgent.indexOf("MSIE") > -1) {
fileName = URLEncoder.encode(fileName, "UTF-8");
} else {
if (userAgent.indexOf("Mozilla") > -1) {
fileName = new String(fileName.getBytes(), "ISO8859-1");
} else {
fileName = URLEncoder.encode(fileName, "UTF-8");
}
}
response.setContentType("application/x-download");
response.addHeader("Content-Disposition", "attachment;filename=" + fileName);
IOUtils.copy(inputStream, outputStream);
return null;
} catch (IOException | ObsException e) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "内部错误");
}
}
public Map uploadFile(File file) {
if (StringUtils.isEmpty(file)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "文件为空");
}
final String test = hweiYunOBSService.uploadFile(file);
return new Result<>(ResultCodeWraper.SUCCESS, test);
}
public Map uploadFileByte(byte data[], String fileName) {
if (StringUtils.isEmpty(fileName)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "文件为空");
}
final String test = hweiYunOBSService.uploadFileByte(data, fileName);
return new Result<>(ResultCodeWraper.SUCCESS, test);
}
public byte[] getFileByteArray(String filePath) throws IOException {
InputStream input = hweiYunOBSService.fileDownload(filePath.substring(0,filePath.lastIndexOf("/")) + "/" + filePath);
byte[] b = new byte[1024];
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int len;
while ((len = input.read(b)) != -1){
bos.write(b, 0, len);
}
bos.close();
input.close();
return bos.toByteArray();
}
@RequestMapping(value = "{fileName}/delete", method = RequestMethod.POST)
public Map delete(@PathVariable String fileName) {
if (StringUtils.isEmpty(fileName)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "删除文件为空");
}
final boolean delete = hweiYunOBSService.delete(fileName);
return new Result<>(delete ? ResultCodeWraper.SUCCESS : ResultCodeWraper.REQUEST_ERROR, delete);
}
@RequestMapping("/deleteFileByPath")
public Map deleteFileByPath(HttpServletRequest request, @RequestParam Map param) {
String path = StringUtils.getStringByObj(param.get("path"));
if (StringUtils.isEmpty(path)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "文件path为空");
}
final boolean delete = hweiYunOBSService.deleteFileByPath(path);
return new Result<>(delete ? ResultCodeWraper.SUCCESS : ResultCodeWraper.REQUEST_ERROR, delete);
}
@RequestMapping(value = "deletes", method = RequestMethod.POST)
public Map delete(@RequestParam("fileNames") List<String> fileNames) {
if (StringUtils.isEmpty(fileNames)) {
return new Result<>(ResultCodeWraper.REQUEST_ERROR, "删除文件为空");
}
final boolean delete = hweiYunOBSService.delete(fileNames);
return new Result<>(delete ? ResultCodeWraper.SUCCESS : ResultCodeWraper.REQUEST_ERROR, delete);
}
public static void dfsdelete(String path) {
File file = new File(path);
if (file.isFile()) {
file.delete();
return;
}
String[] list = file.list();
for (int i = 0; i < list.length; i++) {
dfsdelete(path + File.separator + list[i]);
}
file.delete();
return;
}
public static byte[] getFileStream(String url) {
try {
URL httpUrl = new URL(url);
HttpURLConnection conn = (HttpURLConnection) httpUrl.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5 * 1000);
InputStream inStream = conn.getInputStream();
byte[] btImg = readInputStream(inStream);
return btImg;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static byte[] readInputStream(InputStream inStream) throws Exception {
ByteArrayOutputStream outStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len = 0;
while ((len = inStream.read(buffer)) != -1) {
outStream.write(buffer, 0, len);
}
inStream.close();
return outStream.toByteArray();
}
public static byte[] file2byte(File file) {
byte[] buffer = null;
try {
FileInputStream fis = new FileInputStream(file);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
int n;
while ((n = fis.read(b)) != -1) {
bos.write(b, 0, n);
}
fis.close();
bos.close();
buffer = bos.toByteArray();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return buffer;
}
public static File byte2File(byte[] buf, String filePath, String fileName) {
BufferedOutputStream bos = null;
FileOutputStream fos = null;
File file = null;
try {
File dir = new File(filePath);
if (!dir.exists() && dir.isDirectory()) {
dir.mkdirs();
}
file = new File(filePath + File.separator + fileName);
fos = new FileOutputStream(file);
bos = new BufferedOutputStream(fos);
bos.write(buf);
} catch (Exception e) {
e.printStackTrace();
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return file;
}
public static File multipartFile2File(MultipartFile multipartFile) {
File file = null;
if (multipartFile != null) {
try {
file = File.createTempFile("tmp", null);
multipartFile.transferTo(file);
System.gc();
file.deleteOnExit();
} catch (Exception e) {
e.printStackTrace();
}
}
return file;
}
}
三、测试接口
- 上传
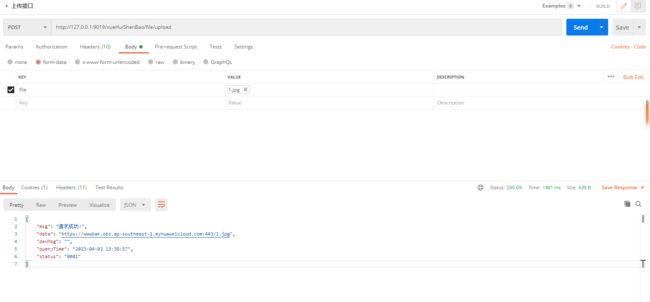
- 下载
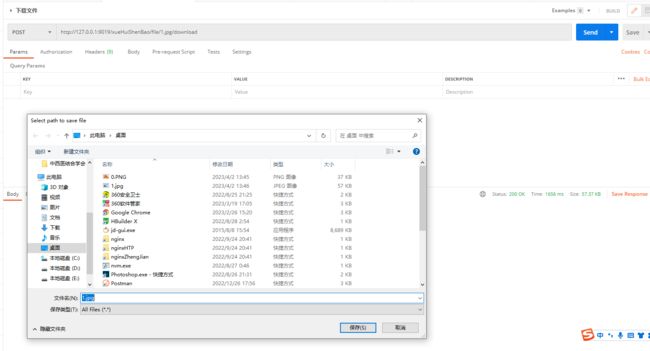
- 删除
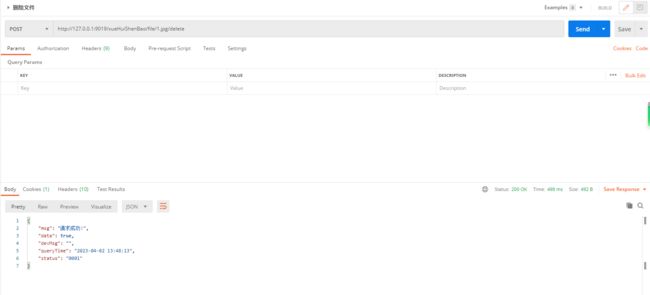
注意:上面使用的springBoot版本是1.5.4,使用上述pom依赖没有问题,如果springBoot的版本是2.1.5,则依赖引入如下
<dependency>
<groupId>com.huaweicloudgroupId>
<artifactId>esdk-obs-javaartifactId>
<version>3.21.8version>
dependency>
<dependency>
<groupId>com.squareup.okhttp3groupId>
<artifactId>okhttpartifactId>
<version>4.9.3version>
dependency>
<dependency>
<groupId>com.squareup.okiogroupId>
<artifactId>okioartifactId>
<version>2.8.0version>
dependency>
<dependency>
<groupId>org.jetbrains.kotlingroupId>
<artifactId>kotlin-stdlibartifactId>
<version>1.3.50version>
dependency>