Description
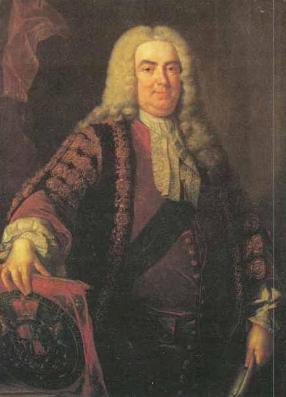
The ministers of the cabinet were quite upset by the message from the Chief of Security stating that they would all have to change the four-digit room numbers on their offices.
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033
1733
3733
3739
3779
8779
8179
The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
Input
One line with a positive number: the number of test cases (at most 100). Then for each test case, one line with two numbers separated by a blank. Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3
1033 8179
1373 8017
1033 1033
Sample Output
6
7
0
Source
大意:从一个素数到另一个素数,每次只能改变一个数,要求每次改变后的数都是素数,要求算出转换的最小次数,简单BFS,不过注意d要还原
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<queue>
using namespace std;
const int MAX = 10000;
bool prime[MAX];
void pri()
{
int i,j;
for( i = 1000; i < MAX; i++){
for( j = 2; j < i;j++){
if(i%j == 0){
prime[i] = false;
break;}
}
if( i == j)
prime[i] = true;
}
}
int d[5];
int num[MAX],k[MAX];
int bfs(int first,int last)
{
int vis;
memset(num,0,sizeof(num));
memset(k,-1,sizeof(k));
queue <int> q;
while(!q.empty())
q.pop();
q.push(first);
k[first] = 0;
while(!q.empty()){
int now = q.front();
q.pop();
d[0] = now/1000;
d[1] = now%1000/100;
d[2] = now%100/10;
d[3] = now%10;
for(int i = 0; i <4; i++){
int temp = d[i];
for(int j = 0; j <= 9;j++){
if(d[i] == j) continue;
d[i] = j;
vis = d[0]*1000+d[1]*100+d[2]*10+d[3];
if(prime[vis] == true && k[vis] == -1){
q.push(vis);
k[vis] = 0;
num[vis] = num[now] + 1;
}
if(vis == last) return num[vis];
}
d[i] = temp;
}
if(now == last) return num[now];
}
return -1;
}
int main()
{
int T,n,m;
scanf("%d",&T);
pri();
while(T--){
scanf("%d%d",&n,&m);
if(bfs(n,m)== -1) printf("Impossible\n");
else
printf("%d\n",bfs(n,m));
}
return 0;
}
View Code