实现效果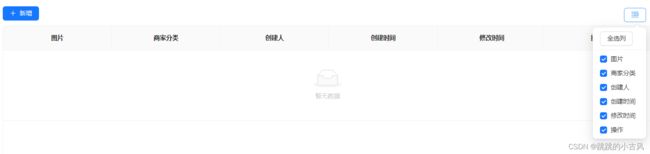
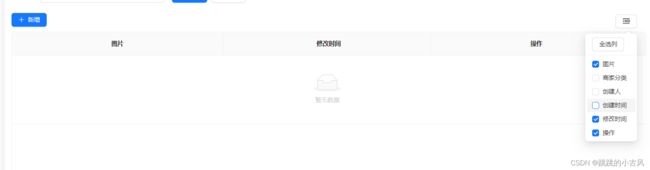
import { Table, Pagination, Button, Dropdown, Checkbox, message } from 'antd';
import { useState, useEffect } from 'react';
import { PicRightOutlined } from '@ant-design/icons';
import './index.less';
const TableComponent = (props) => {
const powerList = JSON.parse(localStorage.getItem('powerList'));
const isShow = powerList.includes(props.auth);
const [open, setOpen] = useState(false);
const [items, setItems] = useState([]);
const [columns, setColumns] = useState(
props.columns.map((item) => {
return {
...item,
align: 'center',
ellipsis: {
showTitle: false,
},
};
}),
);
const [starCol, setStarCol] = useState(
props.columns.map((item) => {
return {
...item,
check: true,
align: 'center',
ellipsis: {
showTitle: false,
},
};
}),
);
useEffect(() => {
changItem(starCol);
}, [props]);
const serialNumber = (pageIndex, pageSize, index) => {
return (pageIndex - 1) * pageSize + index;
};
const onClick = ({ key }) => {
if (key == 'all') {
const newDrop = starCol;
newDrop.map((o, index) => {
o.check = true;
});
changItem(starCol);
setColumns(
props.columns.map((item) => {
return {
...item,
check: true,
align: 'center',
ellipsis: {
showTitle: false,
},
};
}),
);
} else {
const newDrop = starCol;
newDrop.map((o, index) => {
if (index == key) {
o.check = !o.check;
}
});
let newColumns = newDrop.filter((o) => o.check);
if (newColumns.length == 0) {
message.warning('请至少选择一列');
} else {
setStarCol(newDrop);
changItem(newDrop);
setColumns(newColumns);
}
}
};
const changItem = (data) => {
let opitems = data.map((item, index) => {
return {
key: index,
label: <Checkbox checked={item.check}>{item.title}</Checkbox>,
};
});
opitems.unshift(
{
key: 'all',
label: <Button>{'全选列'}</Button>,
},
{
type: 'divider',
},
);
setItems(opitems);
};
const dataSource = props.dataSource?.map((item, index) => {
return {
index: serialNumber(props.defaultCurrent, props.size, index + 1),
...item,
};
});
return (
<div className="table">
<Dropdown
menu={{
items,
onClick,
}}
overlayClassName="drop"
trigger={['click']}
onOpenChange={() => setOpen(!open)}
open={open}
arrow
placement="bottomRight"
>
<a onClick={(e) => e.preventDefault()}>
<Button icon={<PicRightOutlined />} title="显示/隐藏列"></Button>
</a>
</Dropdown>
<Table
bordered
columns={columns}
rowSelection={props?.rowSelection}
dataSource={isShow ? dataSource : null}
rowKey={(item) => item.id}
pagination={false}
className="insiadeTable"
scroll={{
y: 450,
...props?.scroll,
}}
onRow={props?.onRow}
rowClassName={props?.rowClassName}
summary={props?.summary}
/>
<div className="pagination">
{props.total ? (
<Pagination
showQuickJumper
defaultCurrent={props.defaultCurrent}
total={props.total}
showTotal={(total) => `共 ${total} 条`}
size={props.size}
onChange={props.paginationChange}
pageSizeOptions={[10, 50, 100, 500]}
/>
) : (
''
)}
</div>
</div>
);
};
export default TableComponent;
页面使用
<TableComponent
auth="/psychologyReservationDate/pageList"
className="list"
columns={columns}
dataSource={roleList}
scroll={{
x: 1600,
}}
total={listTotal}
size={listSize}
defaultCurrent={current}
paginationChange={paginationChange}
/>