1:application.yml文件配置
server:
port: 8088
servlet:
context-path: /test
spring:
datasource:
name: text
url: jdbc:mysql://localhost:3306/dsdd?serverTimezone=GMT&useUnicode=true&characterEncoding=utf-8&useSSL=true
username: root
password: root
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis-plus:
mapper-locations: classpath*:com/example/poi/mapper/**/xml/*Mapper.xml
global-config:
banner: false
db-config:
id-type: ASSIGN_ID
table-underline: true
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
call-setters-on-nulls: true
2:pom.xml文件依赖管理,web服务必须按以下依赖
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0modelVersion>
<parent>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-parentartifactId>
<version>2.2.7.RELEASEversion>
<relativePath/>
parent>
<groupId>com.examplegroupId>
<artifactId>poiartifactId>
<version>0.0.1-SNAPSHOTversion>
<name>poiname>
<description>poidescription>
<properties>
<java.version>8java.version>
properties>
<dependencies>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starterartifactId>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-testartifactId>
<scope>testscope>
dependency>
<dependency>
<groupId>com.baomidougroupId>
<artifactId>mybatis-plus-boot-starterartifactId>
<version>3.5.1version>
dependency>
<dependency>
<groupId>mysqlgroupId>
<artifactId>mysql-connector-javaartifactId>
<version>8.0.13version>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-maven-pluginartifactId>
plugin>
plugins>
<resources>
<resource>
<directory>src/main/resourcesdirectory>
<includes>
<include>**/*.propertiesinclude>
<include>**/*.ymlinclude>
<include>**/*.xmlinclude>
includes>
<filtering>truefiltering>
resource>
<resource>
<directory>src/main/javadirectory>
<includes>
<include>**/*.propertiesinclude>
<include>**/*.xmlinclude>
includes>
<filtering>truefiltering>
resource>
resources>
build>
project>
注意:1-使用web服务controller访问必须导入以下依赖
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
注意:2-使用mybatis必须导入以下依赖
<dependency>
<groupId>com.baomidougroupId>
<artifactId>mybatis-plus-boot-starterartifactId>
<version>3.5.1version>
dependency>
注意:3-打开项目的target目录,观察里面是否有对应的××Mapper.xml文件,若没有,则在pom.xml文件中加入如下配置
<resources>
<resource>
<directory>src/main/resourcesdirectory>
<includes>
<include>**/*.propertiesinclude>
<include>**/*.ymlinclude>
<include>**/*.xmlinclude>
includes>
<filtering>truefiltering>
resource>
<resource>
<directory>src/main/javadirectory>
<includes>
<include>**/*.propertiesinclude>
<include>**/*.xmlinclude>
includes>
<filtering>truefiltering>
resource>
resources>
3:项目结构图
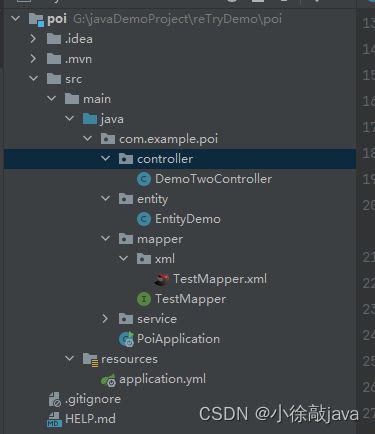
4:启动类
package com.example.poi;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan(basePackages = "com.example.poi.mapper")
public class PoiApplication {
public static void main(String[] args) {
SpringApplication.run(PoiApplication.class, args);
}
}
5:controller类
package com.example.poi.controller;
import com.example.poi.service.ITest;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletResponse;
@RestController
@RequestMapping("/customDemo")
public class DemoTwoController {
@Resource
ITest iTest;
@GetMapping("/export")
public void exportExcel(HttpServletResponse response) {
String age = "20";
String phone = iTest.getPhone(age);
}
}
6:service和impl类
package com.example.poi.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.example.poi.entity.EntityDemo;
public interface ITest extends IService<EntityDemo> {
String getPhone(String age);
}
package com.example.poi.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.example.poi.entity.EntityDemo;
import com.example.poi.mapper.TestMapper;
import com.example.poi.service.ITest;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
@Service
public class TestImpl extends ServiceImpl<TestMapper, EntityDemo> implements ITest {
@Resource
TestMapper testMapper;
@Override
public String getPhone(String age) {
EntityDemo list = testMapper.selectName();
this.baseMapper.selectOne(new LambdaQueryWrapper<EntityDemo>().eq(EntityDemo::getAge, age));
return "entityDemo.getPhone()";
}
}
7:mapper类和xml文件
package com.example.poi.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.poi.entity.EntityDemo;
public interface TestMapper extends BaseMapper<EntityDemo> {
EntityDemo selectName();
}
DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.poi.mapper.TestMapper">
<select id="selectName" resultType="com.example.poi.entity.EntityDemo">
select * from entity_demo where age='20'
select>
mapper>