习题选自:C++ Primer Plus(第六版)
内容仅供参考,如有错误,欢迎指正 !c++的基本类型分为两组:一组由存储为整数的值组成,另外一组由存储为浮点格式的值组成。整数之间通过存储时使用的内存量及有无符号来区分。
- short至少16位
- int至少与short一样长
- long至少32位,且至少与int一样长
- long long至少64位,且至少与long一样长
复习题
1.为什么c++有多种整形?
有多种整形类型,可以根据特定需求选择最合适的类型。例如,可以使用short来存储空格,使用long来确保存储容量,也可以寻找可提高特定计算的速度的类型。
2.声明与下述描述相符的变量。
a.short整数,值为80
b.unsigned int整数,值为42110
c.值为3000000000的整数
short a=80; //or short int a=80;
unsigned int b=42110; //or unsigned b=42110;
unsigned long long c=3000000000; //or unsigned long c=3000000000;
//(注意:不要指望int变量能够存储300000000)
3.c++提供了什么措施来防止超出整形的范围?
c++没有提供自动防止超出整型限制的功能,可以使用头文件climits来确定限制情况。
4.33L与33之间有什么区别?
常量33L的类型为long,常量33类型为int。
5.下面两条c++语句是否等价?
char grade =45;
char grade = 'A';
这两条语句并不真正等价,虽然对于某些系统来说,他们是等效的。最重要的是,只有在使用ASCII码的系统上,第一条语句才将得分设置为字母A,而第二条语句还可用于使用其他编码的系统。其次,65是一个int常量,而‘A’是一个char常量。
6.如何使用c++来找出编码88表示的字符?指出至少两种方法。
//method 1
char c=88;
cout<
7.将long赋值给float变量会导致舍入误差,将long值赋给double变量呢?将long long值赋给double变量呢?
这个问题取决于这两个类型的长度。如果long为4个字节,则没有损失。因为最大的long值是20亿,及有10位数。由于double提供至少13位有效数字,因而不需要进行任何舍入。long long类型可提供19位有效数字,超过了double保证的13位有效数字。
8.下列c++表达式的结果分别是多少?
a.89+2
b.63/4
c.3/46
d.6.03/4
e.15%4
a=74;b=4;c=0;d=4.5;e=3
9.假设x1和x2是两个double变量,你要将他们作为整数相加,再将结果赋给一个整型变量。请编写一条完成这项任务的c++语句。如果要将他们作为double值相加并转换为int呢?
int a=(int)x1+(int)x2;
int a=int(x1)+int(x2);
要将他们作为double类型相加,在进行转换,可采取下述方式之一:
int a=(int)(x1+x2);
int a=int(x1+x2);
10.下面每一条语句的变量都是什么类型?
a.auto cars=15;
b.auto iou=150.37f;
c.auto level='B';
d.auto crat=U'/U00002155';
e.auto fract=8.25f/2.5;
a. int
b. float
c. char
d. char32_t
e. double
编程练习
1.编写一个小程序,要求用户使用一个整数指出自己的身高(单位为英寸),然后将身高转换为英尺,该程序使用下划线字符来指示输入位置。另外,使用一个const符号来表示转换因子。
#include
const double Inch_per_feet = 12.0;
using namespace std;
int main(void)
{
cout << "Please enter your height:_____\b\b\b\b\b";
double ht_inch;
cin >> ht_inch;
double ht_feet = ht_inch/Inch_per_feet;
cout << "Your height is:" << ht_feet << " feets<
2.编写一个程序,要求以几英尺几英寸的方式输入其身高,并以磅为单位输入其体重。(使用3个变量来存储这些信息。)该程序报告其BMI(Body Mass Index ,体重指数)。为了计算BMI,该程序以英寸的方式指出用户的身高(一英尺为十二英寸),并将以英寸为单位的身高身高转换为以米为单位的身高(1英寸=0.0254米)。然后,将以磅为单位的体重转换为千克为单位的体重(1千克=2.2磅)。最后,计算相应的BMI-体重(千克)除以身高(米)的平方。用符号常量表示各种转换因子。
#include
using namespace std;
const double Inch_per_feet = 12.0;
const double Meter_per_inch = 0.0254;
const double Pound_per_kilogram = 2.2;
int main()
{
cout << "Enter your height of feet:";
double ht_feet;
cin >> ht_feet;
cout << "Enter your height of inch:";
double ht_inch;
cin >> ht_inch;
double ht_meter = (ht_feet*Inch_per_feet + ht_inch)*Meter_per_inch;
cout << "Enter your weight in pound: ";
double wt_pound;
cin >> wt_pound;
double wt_kilogram = wt_pound / Pound_per_kilogram;
double BMI = wt_kilogram / ht_meter/ht_meter;
cout << "BMI:" << BMI << endl;
system("pause");
return 0;
}
3.编写一个程序,要求用户以度分秒的方式输入一个纬度,然后以度为单位显示该纬度。1度为60分,1分等于60秒,请以符号常量的方式表示这些值。对于每个输入值,应使用一个独立的变量存储它。下面是该程序运行时的情况:
Enter a latitude in degrees,minutes,and seconds:
First,enter the degrees:37
Next,enter the minutes of arc:51
Finally,enter the seconds of arc:19
37 degrees,51 minutes,19 seconds =37.8553 degrees
#include
using namespace std;
const double Minute_per_degree = 60.0;
const double Second_per_minute = 60.0;
int main()
{
cout << "Enter a latitude in degrees,minutes,and seconds:"<> degrees;
cout << "Next,enter the minutes of arc:";
int minutes;
cin >> minutes;
cout << "Finally,enter the seconds of arc:";
int seconds;
cin >> seconds;
double Degree = degrees + minutes / Minute_per_degree + seconds / Second_per_minute / Minute_per_degree;
cout << degrees << " degrees, " << minutes << " minutes, " << seconds << " seconds = " << Degree<< " degrees";
system("pause");
return 0;
}
4.编写一个程序,要求用户以整数方式输入秒数(使用long或long long变量存储),然后以天、小时、分钟和秒的方式显示这段时间。使用符号常量来表示每天有多少小时、每小时有多少分钟以及每分钟有多少秒。该程序的输出应与下面类似:
Enter the number of seconds:3160000
3160000 seconds = 365 days,17 hours,46 minutes,40 seconds
#include
using namespace std;
const int Sec_per_min = 60;
const int Min_per_hour = 60;
const int Hour_per_day = 24;
int main()
{
cout << "Enter the number of seconds:";
long long total_sec;
cin >> total_sec;
int sec = total_sec%Sec_per_min;
int total_min = total_sec / Sec_per_min;
int min = total_min%Min_per_hour;
int total_hour = total_min / Min_per_hour;
int hour= total_hour%Hour_per_day;
int day = total_hour / Hour_per_day;
cout << total_sec << " seconds = " << day << " days," << hour << " hours," << min << " minutes," << sec << " seconds";
system("pause");
return 0;
}
5.编写一个程序,要求用户输入全球当前的人口和美国当前的人口(或其他国家的人口)。将这些信息存储在long long变量中,并让程序显示美国(或其他国家)的人口占全球人口的百分比。该程序的输出与下面类似:
Enter the world's population:6898758899
Enter the population of the US:310783781
The population of the US is 4.50492% of the world population.
#include
using namespace std;
const int Sec_per_min = 60;
const int Min_per_hour = 60;
const int Hour_per_day = 24;
int main()
{
cout << "Enter the world's population:";
long long tW_population;
cin >> tW_population;
cout << "Enter the population of the US:";
long long US_population;
cin >> US_population;
cout << "The population of the Us is " << double(US_population) / double(tW_population) *100<< "% of the world population";
system("pause");
return 0;
}
6.编写一个程序,要求用户输入驱车里程(英里)和使用汽油量(加仑),然后指出汽车耗油量为一加仑的里程。如果愿意,也可以让程序要求用户以公里为单位输入距离,并以升为单位输入汽油量,然后指出欧洲风格的结果-即每100公里的耗油量(升)。
#include
using namespace std;
int main()
{
cout << "Please enter the distance(miles):";
double distance;
cin >> distance;
cout << "Please enter volume of gasoline(gallon):";
double volume;
cin >> volume;
cout << "You can run " << distance / volume << " miles per gallon" << endl;
system("pause");
return 0;
}
7.编写一个程序,要求每个用户按欧洲风格输入汽车的耗油量(每100公里消耗汽油量(升 )),然后将其转换为美国风格的耗油量-每加仑多少英里。注意,除了使用不同的单位计量外,美国方法(距离/燃料)与欧洲方法(燃料/距离)相反。100公里等于62.14英里,1加仑等于3.875升。因此,19mpg大约合12.41/100km,127mpg大约合8.71/100km。
#include
using namespace std;
int main()
{
cout << "Please enter fuel consumption per 100 km(UN):";
double UN;
cin >> UN;
cout <<" Gallons per mile(US) : "<< 1 / UN*62.14*3.875;
system("pause");
return 0;
}
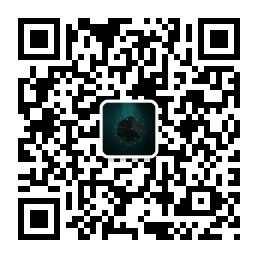