球体(实体)通常被认为是二维图形,即使该图形从其中心在三个平面上可见。造成这种情况的主要原因是,球体仅使用其半径进行测量。
然而,空心球体被认为是三维图形,因为它在其球壁内包含空间,并且有两个不同的半径来测量其尺寸。
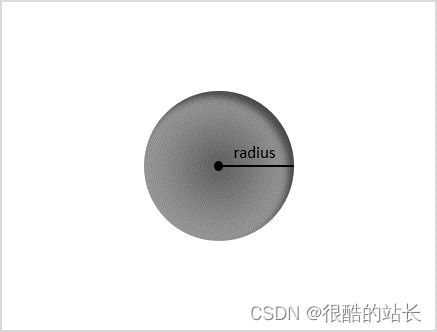
球形图形只有总表面积,因为只有一个维度来测量整个物体。计算球体表面积的公式为 -
固体球体面积 −[数学处理错误]4πR2
球体的体积被认为是物体的圆形壁所保持的质量。计算球体体积的公式为−
固体球体体积 −[数学处理错误]43 πr3
空心球体积 −[数学处理错误]43 π(R−r)3
其中,R 是外球体的半径,r 是内球体的半径。
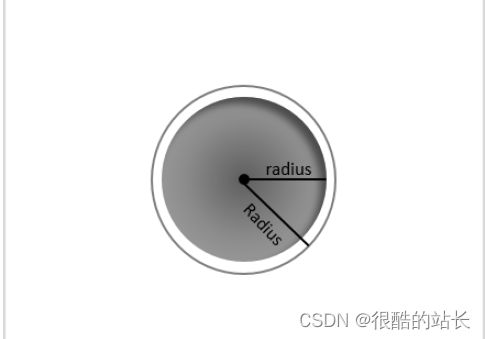
输入输出方案
让我们看一些输入输出场景来计算球体的面积和体积 -
假设要找到的面积和体积是一个实心球体 -
Input: 7 // 7 is the radius
Result: Area - 314.1592653589793
Volume - 359.188760060433
假设要找到的面积和体积是一个空心球体 -
Input: (7, 5) // 7 is the outer radius, 5 is the inner radius
Result: Area - 314.1592653589793 // Area is same
Volume - 100.53096491487338
使用数学公式
在python程序中,我们使用讨论的数学公式并计算球体的面积和体积。我们导入匹配库以使用 pi 常量。
例
以下是查找球形 3D 图形的面积和体积的示例 -
import math R = 7 #outer radius of sphere r = 5 # inner radius of sphere #calculating area of solid sphere area = 4 * (math .pi ) * (r ) * (r ) #calculating volume of hollow sphere volume_hollow = 4 * (math .pi ) * (R - r ) * (R - r ) * (R - r ) #calculating volume of solid sphere volume_solid = ( 1 / 3 ) * (math .pi ) * (R ) * (R ) * (R ) #displaying output print ( "Area of the sphere: " , str (area ) ) print ( "Volume of the hollow sphere: " , str (volume_hollow ) ) print ( "Volume of the solid sphere: " , str (volume_solid ) )
输出
输出如下所示 -
Area of the sphere: 314.1592653589793
Volume of the hollow sphere: 100.53096491487338
Volume of the solid sphere: 359.188760060433
计算面积和体积的功能
Python还利用函数来提高程序的模块化。在这种情况下,我们使用一个计算球体面积和体积的函数。
例
在下面的python程序中,我们使用用户定义的函数计算实心球和空心球体的面积和体积 -
import math def sphere_area_volume (R , r ) : #calculating area of solid sphere area = 4 * (math .pi ) * (r ) * (r ) #calculating volume of hollow sphere volume_hollow = 4 * (math .pi ) * (R - r ) * (R - r ) * (R - r ) #calculating volume of solid sphere volume_solid = ( 1 / 3 ) * (math .pi ) * (R ) * (R ) * (R ) #displaying output print ( "Area of the sphere: " , str (area ) ) print ( "Volume of the hollow sphere: " , str (volume_hollow ) ) print ( "Volume of the solid sphere: " , str (volume_solid ) ) R = 7 #outer radius of sphere r = 5 # inner radius of sphere sphere_area_volume (R , r )
输出
在执行上述代码时,输出以 -
Area of the sphere: 314.1592653589793
Volume of the hollow sphere: 100.53096491487338
Volume of the solid sphere: 359.188760060433