一行代码实现iOS项目启动页, 包括加载网络视频, 本地视频, 网络图片, 本地图片
github下载地址:https://github.com/ZHHalsey/AppStart(感觉有用可以点个star)
可以设置倒计时
使用方法很简单
导入写好的类ZHMoviePlayerController, 创建一个对象, 然后根据项目需求调用下面的两个对象方法(分加载视频跟加载图片,可以是网络的也可以是本地的)
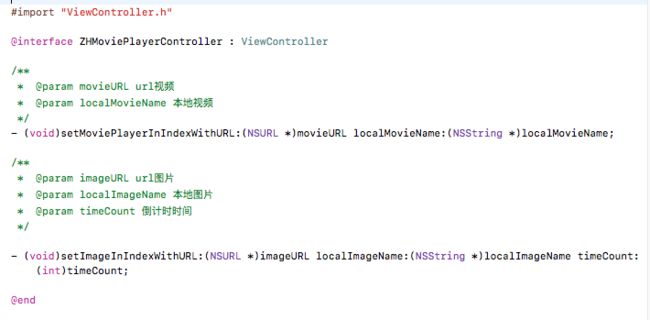
image
如果加载的时候, 如果只加载网络图片不加载本地图片的话,本地图片参数传入为nil就可以,加载视频同理
这里展示的demo没加缓存, 我自己做的项目中加了缓存了,提供下思路在这里:
加缓存的话, 写入沙盒, 设置一个userdefault, 启动的时候先去沙盒找, 如果沙盒有的话就加载沙盒的, 沙盒没有的话就加载网络的, 然后把需要加载的启动页写入沙盒就行
demo展示放不了视频, 所以就放几张图片, 供大家参考
下面这个是视频启动页,可以点击按钮进入应用, 也可以设置几秒倒计时自动进入
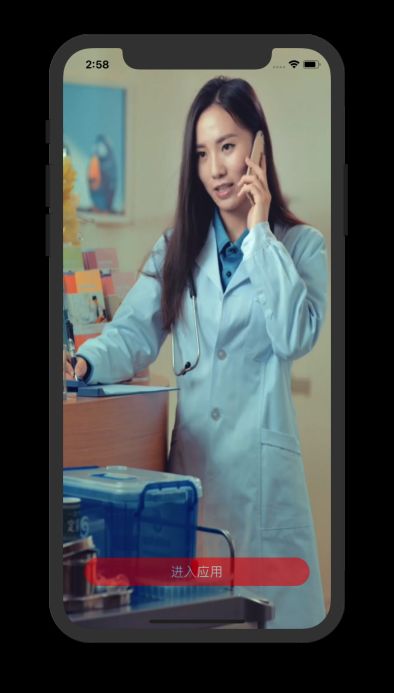
image
下面这个是加载图片启动页, 这里是用的倒计时的加载
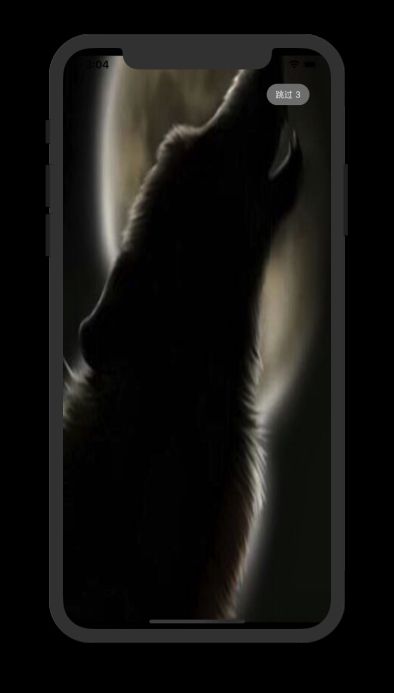
image
贴上源码:
#import "ViewController.h"
@interface ZHMoviePlayerController : ViewController
/**
* @param movieURL 网上url视频
* @param localMovieName 本地视频
*/
- (void)setMoviePlayerInIndexWithURL:(NSURL *)movieURL localMovieName:(NSString *)localMovieName;
/**
* @param imageURL 网上url图片
* @param localImageName 本地图片
* @param timeCount 倒计时时间
*/
- (void)setImageInIndexWithURL:(NSURL *)imageURL localImageName:(NSString *)localImageName timeCount:(int)timeCount;
@end
#define SCREEN_HEIGHT [UIScreen mainScreen].bounds.size.height
#define SCREEN_WIDTH [UIScreen mainScreen].bounds.size.width
#import "ZHMoviePlayerController.h"
#import
#import
#import "ViewController.h"
@interface ZHMoviePlayerController ()
@property (nonatomic, strong)AVPlayerViewController *AVPlayer;
@property (nonatomic, strong)UIButton *enterMainButton;
@property (nonatomic, assign) int timeCount;
@property (nonatomic, weak)NSTimer *timer;
@property (nonatomic, weak)NSTimer *timer1;
@end
@implementation ZHMoviePlayerController
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor orangeColor];
}
- (void)setMoviePlayerInIndexWithURL:(NSURL *)movieURL localMovieName:(NSString *)localMovieName
{
self.AVPlayer = [[AVPlayerViewController alloc]init];
// 取消多分屏功能
self.AVPlayer.allowsPictureInPicturePlayback = NO;
self.AVPlayer.showsPlaybackControls = false;
AVPlayerItem *item;
if (movieURL) {
NSLog(@"传入了网络视频url过来");
item = [[AVPlayerItem alloc]initWithURL:movieURL];
}else if (localMovieName) {
NSLog(@"加载的是本地的视频");
NSString *path = [[NSBundle mainBundle] pathForResource:@"movie.mp4" ofType:nil];
NSLog(@"path---%@", path);
item = [[AVPlayerItem alloc]initWithURL:[NSURL fileURLWithPath:path]];
}
AVPlayer *player = [AVPlayer playerWithPlayerItem:item];
// layer
AVPlayerLayer *layer = [AVPlayerLayer playerLayerWithPlayer:player];
[layer setFrame:CGRectMake(0, 0, SCREEN_WIDTH, SCREEN_HEIGHT)];
// 填充模式
// layer.videoGravity = AVLayerVideoGravityResizeAspect; // 保持视频的纵横比
layer.videoGravity = AVLayerVideoGravityResize; // 填充整个屏幕
self.AVPlayer.player = player;
[self.view.layer addSublayer:layer];
[self.AVPlayer.player play];
// 重复播放。
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(playDidEnd:)
name:AVPlayerItemDidPlayToEndTimeNotification
object:item];
// [self createLoginBtn]; // 3秒后自动就停止(这里自行选择)
[self createLoginBtn1]; // 不点的话 就一直播放视频
}
- (void)setImageInIndexWithURL:(NSURL *)imageURL localImageName:(NSString *)localImageName timeCount:(int)timeCount{
_timeCount = timeCount;
// http://fimg.yucuizhubao.com/img/start.png
UIImageView *imagev1 = [[UIImageView alloc]initWithFrame:CGRectMake(0, 0, SCREEN_WIDTH, SCREEN_HEIGHT)];
if (imageURL) {
NSLog(@"加载的是网络上的图片");
NSData *data = [NSData dataWithContentsOfURL:imageURL];
UIImage *image1 = [UIImage imageWithData:data];
imagev1.image = image1;
}
if (localImageName) {
NSLog(@"加载的是本地的图片");
UIImage *image = [UIImage imageNamed:@"bj.png"];
imagev1.image = image;
}
[self.view addSubview:imagev1];
[self createLoginBtn];
}
// 播放完成代理
- (void)playDidEnd:(NSNotification *)Notification{
// 重新播放
[self.AVPlayer.player seekToTime:CMTimeMake(0, 1)];
[self.AVPlayer.player play];
}
// 用户不用点击, 几秒后自动进入程序
- (void)createLoginBtn
{
// 进入按钮
_enterMainButton = [[UIButton alloc] init];
_enterMainButton.frame = CGRectMake(SCREEN_WIDTH - 90, 50, 60, 30);
_enterMainButton.backgroundColor = [UIColor grayColor];
_enterMainButton.titleLabel.font = [UIFont systemFontOfSize:12];
_enterMainButton.layer.cornerRadius = 15;
NSString *title = [NSString stringWithFormat:@"跳过 %d", _timeCount];
[_enterMainButton setTitle:title forState:UIControlStateNormal];
_timer = [NSTimer scheduledTimerWithTimeInterval:1.0 target:self selector:@selector(DaoJiShi) userInfo:nil repeats:YES];
[self.view addSubview:_enterMainButton];
[_enterMainButton addTarget:self action:@selector(enterMainAction) forControlEvents:UIControlEventTouchUpInside];
}
// 倒计时
- (void)DaoJiShi{
if (_timeCount > 0) {
_timeCount -= 1;
NSString *title = [NSString stringWithFormat:@"跳过 %d", _timeCount];
[_enterMainButton setTitle:title forState:UIControlStateNormal];
}else{
[_timer invalidate];
_timer = nil;
[self enterMainAction];
}
}
// 不会自动停止, 需要用户点击按钮才能进入应用
- (void)createLoginBtn1{ // 这里的时间是3秒后视频页面出现按钮
_timer1 = [NSTimer scheduledTimerWithTimeInterval:3.0 target:self selector:@selector(showClickBtn) userInfo:nil repeats:YES];
}
- (void)showClickBtn{
NSLog(@"显示进入应用按钮");
UIButton *btn = [[UIButton alloc] init];
btn.frame = CGRectMake(30, SCREEN_HEIGHT - 100, SCREEN_WIDTH - 60, 40);
btn.backgroundColor = [UIColor redColor];
btn.layer.cornerRadius = 20;
btn.alpha = 0.5;
[btn setTitle:@"进入应用" forState:UIControlStateNormal];
[self.view addSubview:btn];
[btn addTarget:self action:@selector(enterMainAction) forControlEvents:UIControlEventTouchUpInside];
[_timer1 invalidate];
_timer1 = nil;// timer置为nil
}
- (void)enterMainAction{
NSLog(@"点击了进入应用按钮");
ViewController *vc = [[ViewController alloc]init];
self.view.window.rootViewController = vc;
[self.AVPlayer.player pause];
}
@end
Appdelegate里的调用:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// 这是图片还有视频的url链接
NSString *getUrlStr = @"http://clips.vorwaerts-gmbh.de/big_buck_bunny.mp4"; // 网络视频
// NSString *getUrlStr = @"http://fimg.yucuizhubao.com/img/start.png"; // 网络图片
NSLog(@"后缀是--%@", [getUrlStr substringFromIndex:[getUrlStr length] - 4]);
ZHMoviePlayerController *ZHVC = [[ZHMoviePlayerController alloc]init];
if ([[getUrlStr substringFromIndex:[getUrlStr length] - 4] isEqualToString:@".mp4"] ) {
NSLog(@"加载的是视频");
// [ZHVC setMoviePlayerInIndexWithURL:[NSURL URLWithString:getUrlStr] localMovieName:nil]; // 加载网络url视频
[ZHVC setMoviePlayerInIndexWithURL:nil localMovieName:@"movie.mp4"]; // 加载本地视频
self.window.rootViewController = ZHVC;
}else if ([[getUrlStr substringFromIndex:[getUrlStr length] - 4] isEqualToString:@".png"]){
NSLog(@"加载的是图片");
// [ZHVC setImageInIndexWithURL:[NSURL URLWithString:getUrlStr] localImageName:nil timeCount:4];// 加载网络图片
[ZHVC setImageInIndexWithURL:nil localImageName:@"bj.png" timeCount:4]; // 加载本地图片
self.window.rootViewController = ZHVC;
}
return YES;
}
作者:Haleszh
链接:https://www.jianshu.com/p/240032c245ec
来源:
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。