写在前面
- 他也是一个java和java web新秀。此前有过接触java web发展
- 我想一个小项目。要熟悉struts2开发过程
- 一个有趣的想法源于教研室项目上的一个功能实现–自己主动识别运营商,去做不同的处理。项目上採用的是IP地址库的方式,在本地做处理。这里为了简单就採用了淘宝提供的接口服务
- 已经将该项目作为开源项目放在:IP地址仓库 欢迎大家前来点赞
能够学到什么
- struts2的基本执行流程
- HttpClient和org.json库的使用
- 前端採用了bootstrap和ajax。做到了网页的自适应,后端返回json数据和前端交互
步入正题
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.3//EN" "http://struts.apache.org/dtds/struts-2.3.dtd">
<struts>
<constant name="struts.enable.DynamicMethodInvocation" value="true" />
<package name="hello" extends="json-default">
<default-action-ref name="index" />
<action name="index" class="action.IPSearchAction" method="index">
<result name="success">/WEB-INF/pages/ip.jsp </result>
</action>
<action name="search" class="action.IPSearchAction" method="search">
<result name="success" type="json">
<param name="root">responseMap</param>
</result>
</action>
</package>
</struts>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0">
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>/WEB-INF/pages/ip.jsp</welcome-file>
</welcome-file-list>
</web-app>
package action
import java.io.IOException
import java.net.URI
import java.net.URISyntaxException
import java.util.HashMap
import java.util.Map
import org.apache.http.HttpResponse
import org.apache.http.HttpStatus
import org.apache.http.ParseException
import org.apache.http.client.ClientProtocolException
import org.apache.http.client.HttpClient
import org.apache.http.client.methods.HttpGet
import org.apache.http.client.utils.URIBuilder
import org.apache.http.impl.client.HttpClients
import org.apache.http.util.EntityUtils
import org.json.JSONObject
public class IPSearchAction {
String ip
Map<String, Object> responseMap
public Map<String, Object> getResponseMap() {
return responseMap
}
public void setResponseMap(Map<String, Object> responseMap) {
this.responseMap = responseMap
}
public String getIp() {
return ip
}
public void setIp(String ip) {
System.out.println("input ip is: "+ip)
this.ip = ip
}
public String search(){
setOutputValue()
return "success"
}
public String index(){
return "success"
}
public void setOutputValue() {
HttpClient httpclient = HttpClients.createDefault()
System.out.println("the input ip is" + ip)
URI uri = null
try {
uri = new URIBuilder()
.setScheme("http")
.setHost("ip.taobao.com")
.setPath("/service/getIpInfo.php")
.setParameter("ip", ip)
.build()
} catch (URISyntaxException e) {
// TODO Auto-generated catch block
e.printStackTrace()
}
HttpGet httpget = new HttpGet(uri)
HttpResponse response = null
try {
response = httpclient.execute(httpget)
} catch (ClientProtocolException e) {
// TODO Auto-generated catch block
e.printStackTrace()
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace()
}
int statusCode = response.getStatusLine().getStatusCode()
if(statusCode == HttpStatus.SC_OK) { //状态==200。返回成功
String result = null
try {
result = EntityUtils.toString(response.getEntity())
} catch (ParseException e) {
// TODO Auto-generated catch block
e.printStackTrace()
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace()
}
System.out.println(result)
JSONObject resultJson = new JSONObject(result)
int code = resultJson.getInt("code")
String country = null
String region = null
String city = null
String county = null
String isp = null
if(code == 0) {
country = resultJson.getJSONObject("data").getString("country")
region = resultJson.getJSONObject("data").getString("region")
city = resultJson.getJSONObject("data").getString("city")
county = resultJson.getJSONObject("data").getString("county")
isp = resultJson.getJSONObject("data").getString("isp")
System.out.println("code is: "+ code + "country is: " + country + "area is "+region+"county is "+county+
"isp is "+isp)
}
responseMap = new HashMap<String, Object>()
responseMap.clear()
responseMap.put("country", country)
responseMap.put("region", region)
responseMap.put("city", city)
responseMap.put("county", county)
responseMap.put("isp", isp)
}
}
}
- 依赖的库文件
说明:
- project依赖org.json库。採用的是:org.json ,下载的是源码。能够打包成json.jar,更方便的使用
- project依赖httpclient。下载地址:httpclient ,使用能够查看它提供的手冊
- project还依赖struts2提供的某些jar包,记得加入
终于效果
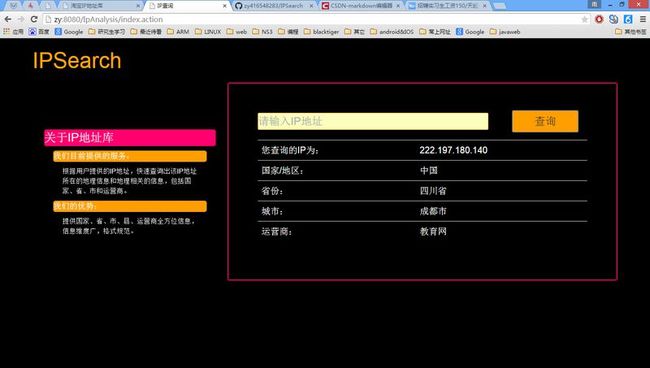
PS:
一些问题记录:
參考资源