1 C++概述
1.2 移植性和标准
2 c++初识
- 引入头文件 #include 标准输入输出流
- 使用标准命名空间 using namespace std;
- 标准输出流对象 cout << “…” << 1234 << 3.14 << endl;
#include
using namespace std;
int main()
{
cout << "Hello World!" << endl;
system("pause");
return EXIT_SUCCESS;
}
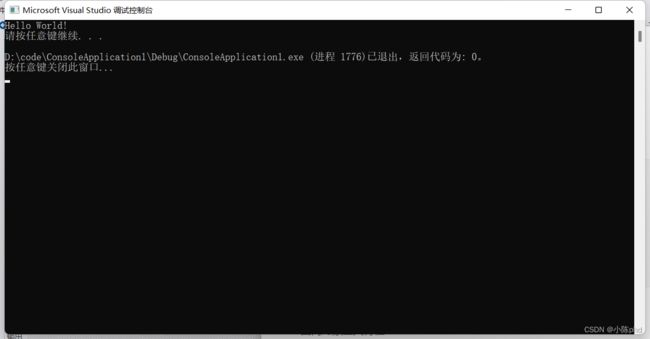
3 双冒号作用域运算符
- ::代表作用域 如果前面什么都不添加 代表全局作用域
#include
using namespace std;
int atk = 1000;
void test01() {
int atk = 2000;
cout << "局部atk=" << atk << endl;
// ::代表作用域 如果前面什么都不添加 代表全局作用域
cout << "全局atk=" << ::atk << endl;
}
int main()
{
test01();
return EXIT_SUCCESS;
}
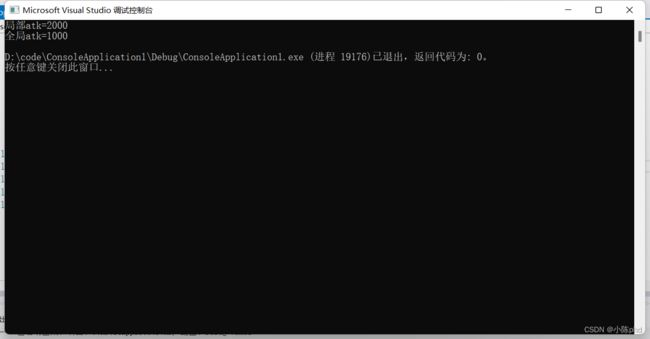
4 namespace命名空间
- 命名空间用途:解决名称冲突,不同空间的方法可以相同,可以解决名称名相同导致冲突的问题。
测试文件如下:
main.cpp
#include
#include"game1.h"
#include"game2.h"
using namespace std;
int main()
{
A::getName();
B::getName();
return EXIT_SUCCESS;
}
game1.h
#pragma once
#include
using namespace std;
namespace A {
void getName();
}
game2.h
#pragma once
#include
using namespace std;
namespace B {
void getName();
}
game1.cpp
#include"game1.h"
void A::getName() {
cout << "这是A的调用" << endl;
}
game2.cpp
#include"game2.h"
void B::getName() {
cout << "这是B的调用" << endl;
}
运行mian.cpp
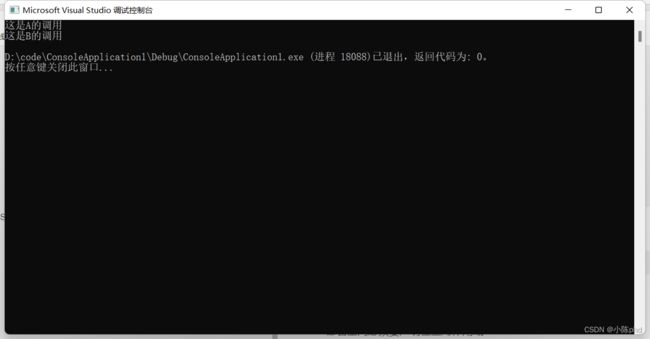
#include
namespace A {
//命名空间下 可以放 变量、函数、结构体、类...
int m_A=10;
void func() {
cout << "this is a test!" << endl;
};
struct Person{
int name;
};
class Animal {
public:
int age;
};
}
using namespace std;
int main()
{
cout << A::m_A << endl;
A::func() ;
A::Person p;
p.name = 2;
A::Animal a;
a.age = 20;
return EXIT_SUCCESS;
}
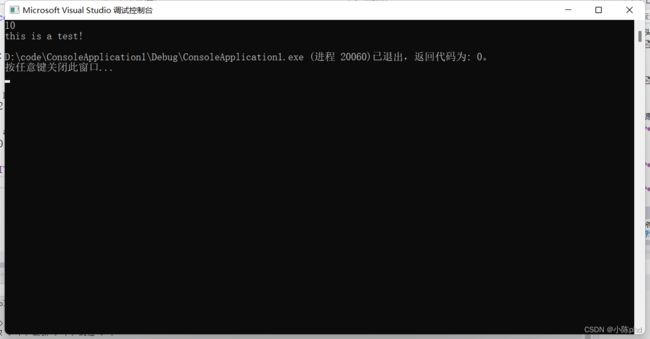
#include
using namespace std;
void test01() {
namespace A {
};
}
int main()
{
return EXIT_SUCCESS;
}
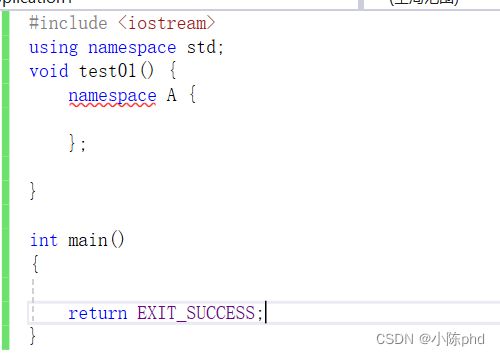
#include
using namespace std;
namespace S {
namespace B {
int age = 0;
};
};
int main()
{
cout << S::B::age << endl;
return EXIT_SUCCESS;
}
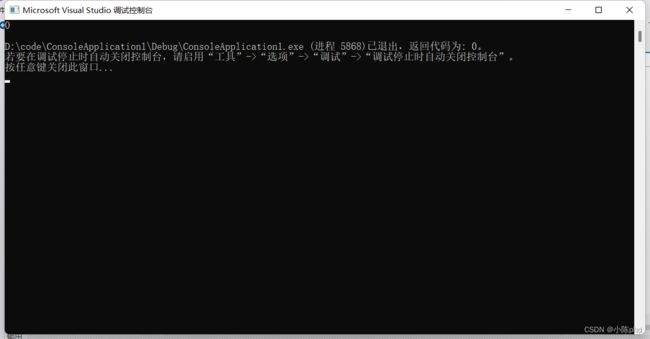
- 命名空间是开放的,可以随时将新成员添加到命名空间下
#include
using namespace std;
namespace A {
int m_A = 10;
namespace C {
int m_c = 100;
};
}
// 添加成员
namespace A {
int m_B = 1000;
};
void test()
{
cout << "A空间下的m_A = " << A::m_A << endl;
cout << "A空间下的m_B = " << A::m_B << endl;
}
int main()
{
test();
return EXIT_SUCCESS;
}
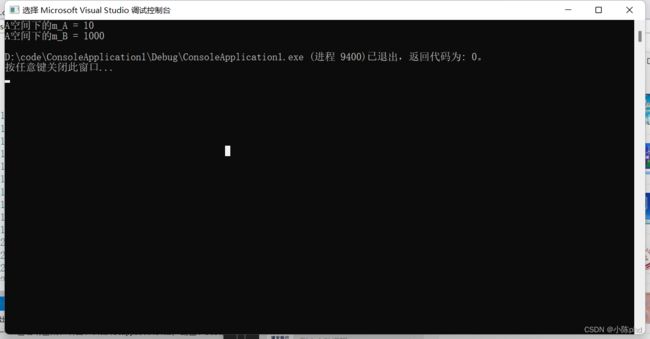
- 命名空间可以匿名的
- 当写的命名空间的匿名的,相当于写了 static
#include
using namespace std;
//命名空间可以是匿名的
namespace
{
int m_C = 1000;
int m_D = 2000;
//当写的命名空间的匿名的,相当于写了 static int m_C = 1000; static int m_D = 2000;
}
void test() {
cout << "m_C = " << m_C << endl;
cout << "m_D = " << ::m_D << endl;
};
int main()
{
test();
return EXIT_SUCCESS;
}
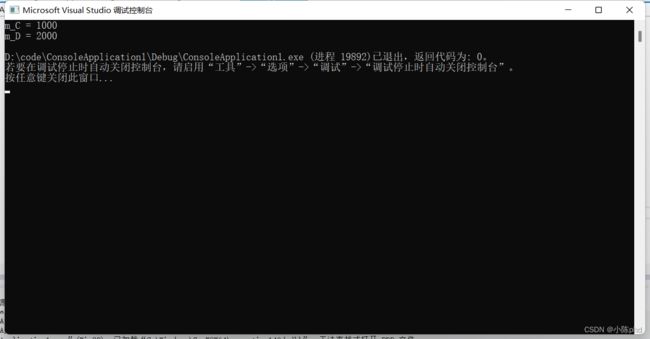
#include
using namespace std;
namespace A
{
int m_C = 1000;
int m_D = 2000;
}
int main()
{
namespace B = A;
cout << B::m_C << endl;
cout << A::m_C << endl;
return EXIT_SUCCESS;
}
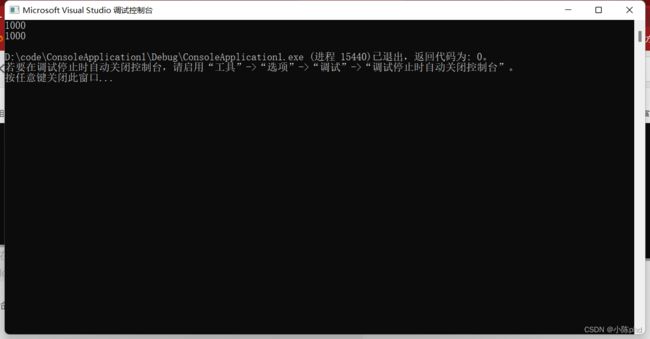
5 using声明以及using编译指令
#include
using namespace std;
namespace A
{
int id = 1;
}
namespace B
{
int id = 3;
}
void test01()
{
int id = 2;
//1、using声明
//using KingGlory::sunwukongId ;
//当using声明与 就近原则同时出现,出错,尽量避免
cout << id << endl;
}
int main()
{
test01();
return EXIT_SUCCESS;
}
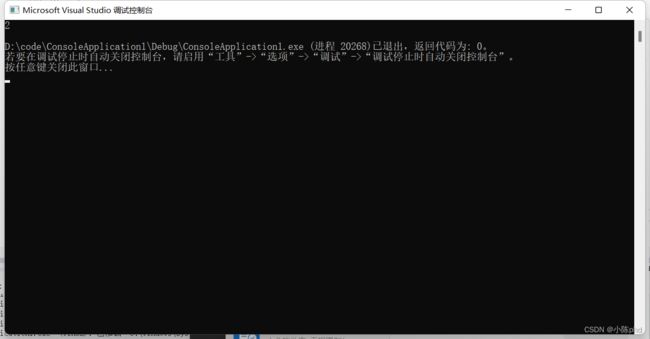
- 当using声明与 就近原则同时出现,出错,尽量避免
#include
using namespace std;
namespace A
{
int id = 1;
}
namespace B
{
int id = 3;
}
void test01()
{
int id = 2;
//1、using声明
using A::id ;
//当using声明与 就近原则同时出现,出错,尽量避免
cout << id << endl;
}
int main()
{
test01();
return EXIT_SUCCESS;
}
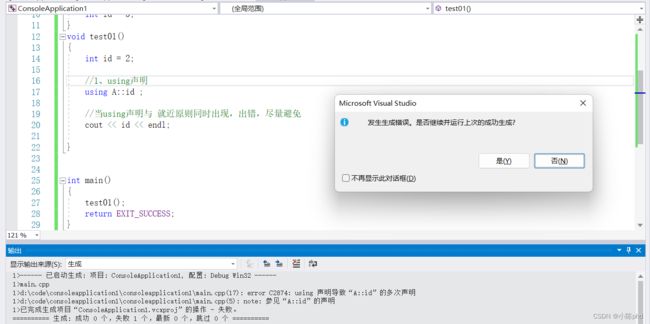
#include
using namespace std;
namespace A
{
int id = 1;
}
namespace B
{
int id = 3;
}
void test01()
{
int id = 2;
//就近原则,先搜索全局,再搜索命名空间
using namespace A;
cout << id << endl;
}
int main()
{
test01();
return EXIT_SUCCESS;
}
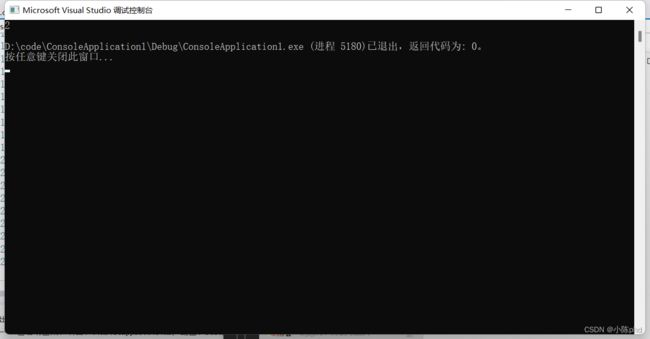