文章目录
- 一【题目类别】
- 二【题目难度】
- 三【题目编号】
- 四【题目描述】
- 五【题目示例】
- 六【题目提示】
- 七【解题思路】
- 八【时间频度】
- 九【代码实现】
- 十【提交结果】
一【题目类别】
二【题目难度】
三【题目编号】
四【题目描述】
- 给定一个二维网格和一个单词,找出该单词是否存在于网格中。
- 单词必须按照字母顺序,通过相邻的单元格内的字母构成,其中“相邻”单元格是那些水平相邻或垂直相邻的单元格。同一个单元格内的字母不允许被重复使用。
五【题目示例】
- 示例:
board =
[
[‘A’,‘B’,‘C’,‘E’],
[‘S’,‘F’,‘C’,‘S’],
[‘A’,‘D’,‘E’,‘E’]
]
给定 word = “ABCCED”, 返回 true
给定 word = “SEE”, 返回 true
给定 word = “ABCB”, 返回 false
六【题目提示】
- b o a r d 和 w o r d 中 只 包 含 大 写 和 小 写 英 文 字 母 。 board 和 word 中只包含大写和小写英文字母。 board和word中只包含大写和小写英文字母。
- 1 < = b o a r d . l e n g t h < = 200 1 <= board.length <= 200 1<=board.length<=200
- 1 < = b o a r d [ i ] . l e n g t h < = 200 1 <= board[i].length <= 200 1<=board[i].length<=200
- 1 < = w o r d . l e n g t h < = 1 0 3 1 <= word.length <= 10^3 1<=word.length<=103
七【解题思路】
- 使用回溯+剪枝的方法,遍历整个表格,从每一个位置开始作为起点判断和word是否相等,递归的时候通过新组成的路径字符和word开始是否一样,如果不一样就返回false,这就是剪枝操作。遇到一个字符加入到路径字符,并将对应的位置置为true(表示已经访问过了),防止二次遍历,接下来就是向上下左右寻找的过程,最后回溯的时候说明上下左右都没有由当前字符组成的路径字符,那么回到上一个状态,将刚加入的字符删除,另外将对应的位置置为true(表示还没有访问过)
八【时间频度】
- 时间复杂度: M M M:表示表格的宽度, N N N:表示表格的长度, L L L:表示word的长度,另外只有第一次遍历需要走四个方向,其余最多走三个方向,因为走过的方向不能再走了,所以时间复杂度为 O ( M ∗ N ∗ 3 L ) O(M*N*3^L) O(M∗N∗3L)
九【代码实现】
- Java语言版
package ToFlashBack;
public class p79_WordSearch {
private static int[][] dires = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
private int row, col;
private boolean hasFind;
private boolean[][] visited;
public static void main(String[] args) {
p79_WordSearch p79_wordSearch = new p79_WordSearch();
char[][] board = {
{'A', 'B', 'C', 'E'},
{'S', 'F', 'C', 'S'},
{'A', 'D', 'E', 'E'}
};
String word = "ABCCED";
boolean res = p79_wordSearch.exist(board, word);
if (res) {
System.out.println("找到!");
} else {
System.out.println("未找到!");
}
}
public boolean exist(char[][] board, String word) {
row = board.length;
col = board[0].length;
hasFind = false;
if (row * col < word.length()) {
return false;
}
visited = new boolean[row][col];
char[] chars = word.toCharArray();
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (board[i][j] == chars[0]) {
backTrack(board, chars, 1, i, j);
if (hasFind) {
return true;
}
}
}
}
return false;
}
private void backTrack(char[][] board, char[] word, int curIndex, int x, int y) {
if (hasFind) {
return;
}
if (curIndex == word.length) {
hasFind = true;
return;
}
visited[x][y] = true;
for (int[] dire : dires) {
int newX = x + dire[0];
int newY = y + dire[1];
if (isIn(newX, newY) && !visited[newX][newY] && board[newX][newY] == word[curIndex]) {
backTrack(board, word, curIndex + 1, newX, newY);
}
}
visited[x][y] = false;
}
private boolean isIn(int x, int y) {
return x >= 0 && x < row && y >= 0 && y < col;
}
}
- C语言版
#include
#include
#include
#include
bool p79_WordSearch_backTrack(char** board, int** visited, char* word, int wordIndex, char* path, int pathIndex, int x, int y, int row, int col)
{
if (x < 0 || y < 0 || x >= row || y >= col || visited[x][y] || board[x][y] != word[wordIndex])
{
return false;
}
if (wordIndex + 1 == strlen(word))
{
return true;
}
path[pathIndex++] = board[x][y];
visited[x][y] = 1;
if (p79_WordSearch_backTrack(board, visited, word, wordIndex + 1, path, pathIndex, x, y + 1, row, col))
{
return true;
}
else if (p79_WordSearch_backTrack(board, visited, word, wordIndex + 1, path, pathIndex, x - 1, y, row, col))
{
return true;
}
else if (p79_WordSearch_backTrack(board, visited, word, wordIndex + 1, path, pathIndex, x + 1, y, row, col))
{
return true;
}
else if (p79_WordSearch_backTrack(board, visited, word, wordIndex + 1, path, pathIndex, x, y - 1, row, col))
{
return true;
}
else
{
path[--pathIndex] == 0;
visited[x][y] = 0;
return false;
}
}
bool exist(char** board, int boardSize, int* boardColSize, char * word)
{
int row = boardSize;
int col = boardColSize[0];
if (row * col < strlen(word) || strlen(word) == 0)
{
return false;
}
int** visited = (int**)malloc(row * sizeof(int*));
for (int i = 0; i < row; i++)
{
visited[i] = (int*)calloc(col, sizeof(int));
}
char* path = (char*)malloc(strlen(word) * sizeof(char));
for (int i = 0; i < strlen(word); i++)
{
path[i] = 0;
}
for (int x = 0; x < row; x++)
{
for (int y = 0; y < col; y++)
{
if (p79_WordSearch_backTrack(board, visited, word, 0, path, 0, x, y, row, col))
{
return true;
}
}
}
return false;
}
十【提交结果】
-
Java语言版
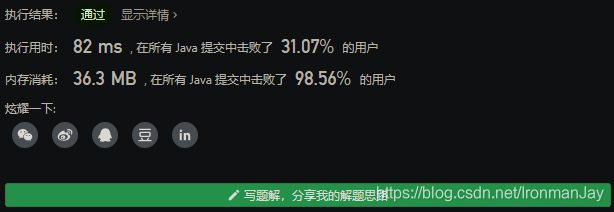
-
C语言版
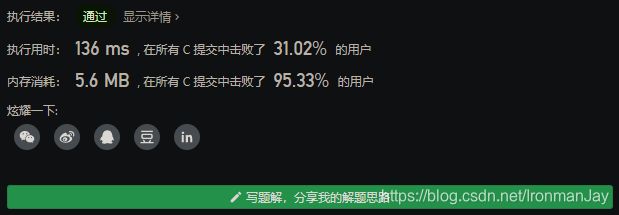