Android动画
View动画(补间动画)、帧动画、属性动画
1. View动画
使用View动画框架可以在Views上执行补间动画。 补间动画是指只要指定动画的开始、动画结束的"关键帧",而动画变化的"中间帧"由系统计算并补齐;无论动画怎样改变View的显示区域(移动或者改变大小),持有该动画的View的边界不会自动调整来适应View的显示区域, 即使如此,该动画仍将被绘制在超出其视图边界并且不会被剪裁, 但是,如果动画超过父视图的边界,则会出现裁剪。
- 平移动画
- 缩放动画
- 旋转动画
- 透明度动画
名称 | 标签 | Animation子类 | 效果 |
---|---|---|---|
平移动画 | TranslateAnimation | 移动View | |
缩放动画 | ScaleAnimation | 放大或缩小View | |
旋转动画 | RotateAnimation | 旋转View | |
透明度动画 | AlphaAnimation | 改变View透明度 |
动画介绍
...
android:interpolator
动画所采用的插值器,插值器影响动画的速度,比如非匀速运动动画就需要通过插值器来控制动画的播放过程。默认为@android:anim/accelerate_decelerate_interpolator,即加速减速插值器。
android:shareInterpolator
表示集合中的动画是否和集合共享一个插值器。如果集合不指定插值器,那么子动画就需要单独指定插值器或者使用默认值。
平移动画
标签属性含义:
- android:fromXDelta
Float or percentage. 移动起始点的x坐标. 表示形式有三种:
1 相对于自己的左边界的距离,单位像素值。(例如 "5")
2 相对于自己的左边界的距离与自身宽度的百分比。(例如 "5%")
3 相对于父View的左边界的距离与父View宽度的百分比。(例如 "5%p") - android:toXDelta
Float or percentage. 移动结束点的x坐标. 表现形式同上 - android:fromYDelta
Float or percentage. 移动起始点的y坐标. 表示形式有三种:
1 相对于自己的上边界的距离,单位像素值。(例如 "5")
2 相对于自己的上边界的距离与自身高度的百分比。(例如 "5%")
3 相对于父View的上边界的距离与父View高度的百分比。(例如 "5%p") - android:toYDelta
Float or percentage. 移动结束点的y坐标. 表现形式同上
缩放动画
标签属性含义:
- android:fromXScale
Float. 水平方向缩放比例的初始值,其中1.0是没有任何变化。 - android:toXScale
Float. 水平方向缩放比例的结束值,其中1.0是没有任何变化。 - android:fromYScale
Float. 竖直方向缩放比例的初始值,其中1.0是没有任何变化。 - android:toYScale
Float. 竖直方向缩放比例的结束值,其中1.0是没有任何变化。 - android:pivotX
缩放轴点的x坐标,会影响缩放的效果,比如viewX轴中心点50% - android:pivotY
缩放轴点的y坐标,会影响缩放的效果,比如viewY轴中心点50%
默认情况下轴点是view的中心点,这时候在水平方向进行缩放会导致View向左右两个方向同时进行缩放;但是如果把轴点设置再View的右边界,那么View就会向左边进行缩放,反之向右进行缩放。
旋转动画
标签属性含义:
- android:fromDegrees
Float. 旋转初始的角度。0度为X轴 - android:toDegrees
Float. 旋转结束的角度,正值为顺时针方向旋转 - android:pivotX
Float or percentage. 旋转中心点x坐标,表示形式有三种:
1 相对于自己的左边界的距离,单位像素值。(例如 "5")
2 相对于自己的左边界的距离与自身宽度的百分比。(例如 "5%")
3 相对于父View的左边界的距离与父View宽度的百分比。(例如 "5%p") - android:pivotY
Float or percentage. 旋转中心点y坐标,表示形式有三种:
1 相对于自己的上边界的距离,单位像素值。(例如 "5")
2 相对于自己的上边界的距离与自身宽度的百分比。(例如 "5%")
3 相对于父View的上边界的距离与父View高度的百分比。(例如 "5%p")
Android RotateAnimation详解
旋转动画中轴点扮演着旋转轴的的角色,即View是围绕着轴点进行旋转的,默认情况下轴点为View的中心点。旋转坐标系为以旋转点为坐标系(0,0)点。x轴为0度,顺时针方向旋转一定的角度。
透明度动画
标签属性含义:
- android:fromAlpha
Float. 设置透明度的初始值,其中0.0是透明,1.0是不透明的。 - android:toAlpha
Float. 设置透明度的结束值,其中0.0是透明,1.0是不透明的。
View动画常用属性
- android:duration
动画的持续时间 - android:fillAfter
动画结束后View是否停留在结束位置,true表示停留再结束位置
使用方式
1. 使用XML文件描述动画
Button button = (Button) findViewById(R.id.button1);
Animation animation = AnimationUtils.loadAnimation(this, R.anim.animation_test);
button.startAnimation(animation);
2. 使用Animation子类
AlphaAnimation animation = new AlphaAnimation(0, 1);
animation.setDuration(300);
button.startAnimation(animation);
Animation的动画监听setAnimationListener
public static interface AnimationListener{
void onAnimationStart(Animation animation);
void onAnimationEnd(Animation animation);
void onAnimationRepeat(Animation animation);
}
关于插值器
时间插值器(TimeInterpolator)的作用是根据时间流逝的百分比计算出动画进度的百分比。
Android提供了常用的时间插值器如下表所示:
Interpolator class | Resource ID |
---|---|
AccelerateDecelerateInterpolator | @android:anim/accelerate_decelerate_interpolator |
AccelerateInterpolator | @android:anim/accelerate_interpolator |
AnticipateInterpolator | @android:anim/anticipate_interpolator |
AnticipateOvershootInterpolator | @android:anim/anticipate_overshoot_interpolator |
BounceInterpolator | @android:anim/bounce_interpolator |
CycleInterpolator | @android:anim/cycle_interpolator |
DecelerateInterpolator | @android:anim/decelerate_interpolator |
LinearInterpolator | @android:anim/linear_interpolator |
OvershootInterpolator | @android:anim/overshoot_interpolator |
对应的类与时间插值器(TimeInterpolator)之间的继承关系如下图所示:
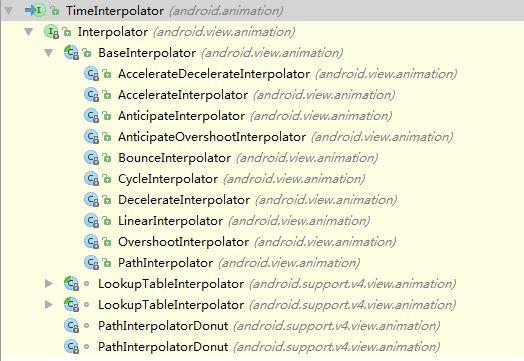
为了比较直观的感受到Android提供的常用时间插值器的效果,我通过程序绘制出了动画进度的百分比随着时间流逝的百分比变化的波形图(波形图的横向代表时间流逝的百分比,纵向代表动画进度的百分比,时间流逝的百分比我一共选取了11个值,分别为0、0.1、以此类推一直到1),如下所示:
AccelerateDecelerateInterpolator
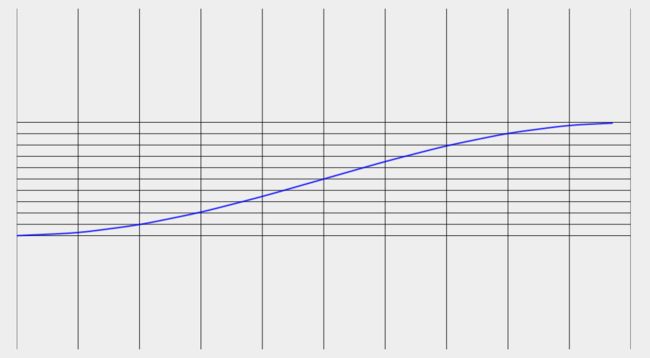
AccelerateInterpolator
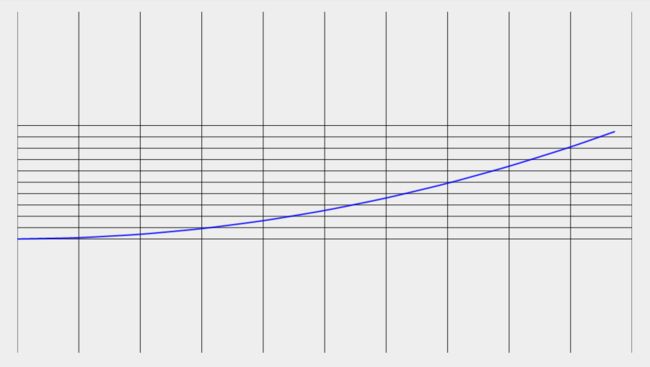
AnticipateInterpolator
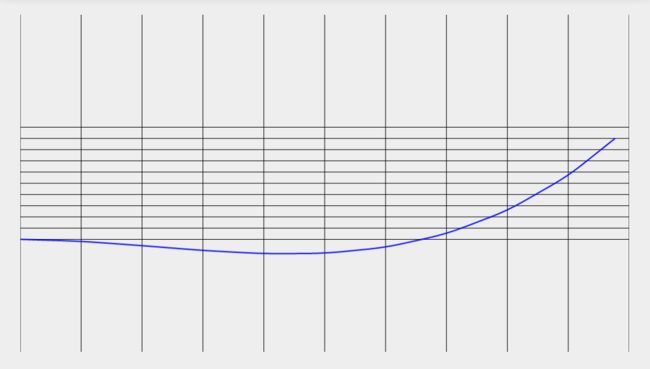
AnticipateOvershootInterpolator
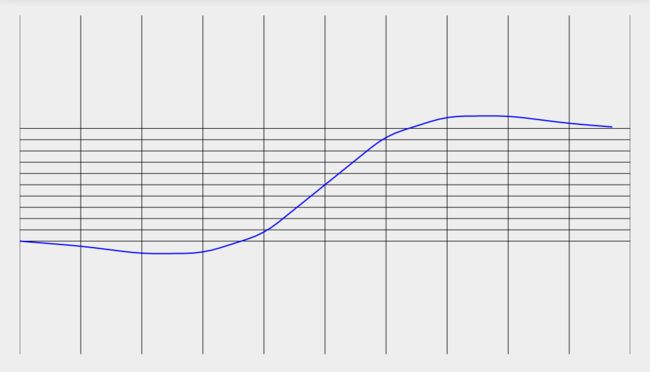
BounceInterpolator
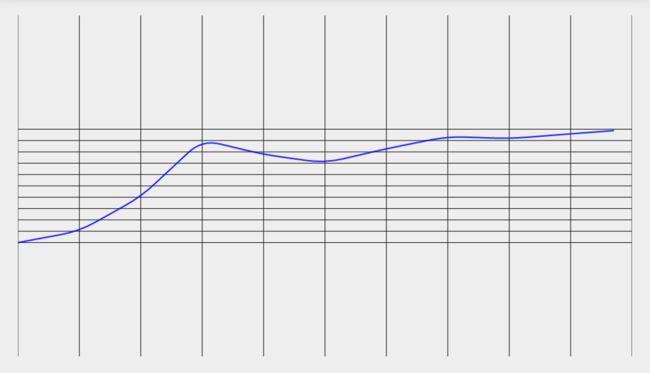
CycleInterpolator
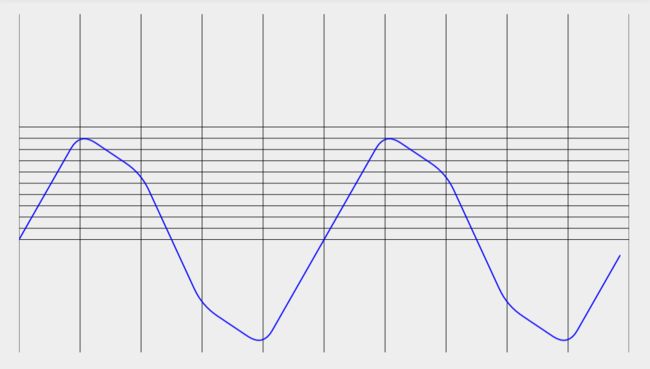
DecelerateInterpolator
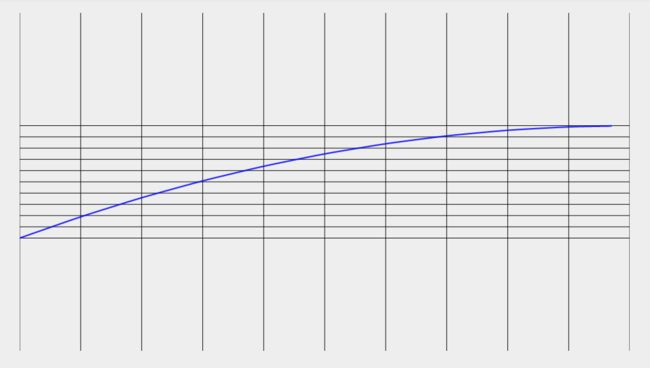
LinearInterpolator
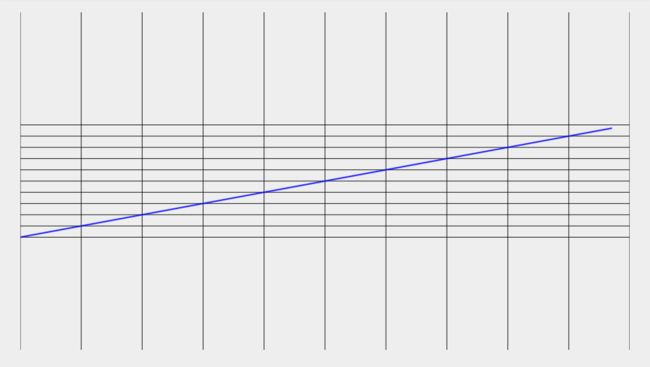
OvershootInterpolator
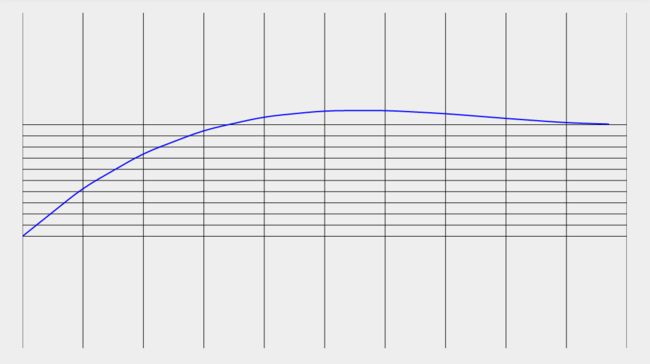
更多插值器介绍
View动画的特殊使用场景
ViewGroup中控制子元素的出场效果;Activity中实现不同Activity之间的切换效果
LayoutAnimation
LayoutAnimation作用于ViewGroup,为ViewGroup指定一个动画,这样当他的子元素出场都会有这种动画效果。比如应用在ListView,RecyclerView中,他们的每一个item都以一定的动画形式出现。
(1) 定义LayoutAnimation
上述几个属性介绍:
android:delay
表示子元素开始动画的延迟,比如子元素入场动画的时间周期为300ms,那么0.5表示每个子元素都需要延迟150ms才能播放入场动画。总体来说,第一个元素延迟150ms开始播放入场动画,第2个元素延迟300ms开始播放入场动画,依次类推。
android:animationOrder
表示子元素动画的顺序,有三种选项:normal,reverse,random,其中normal表示顺序显示,即排在前面的子元素先开始播放入场动画;reverse表示逆向显示;random则是随机播放入场动画。
android:animation
为子元素指定入场动画
(2)指定入场动画
(3)为ViewGroup指定android:layoutAnimation属性:android:layoutAnimation="@anim/anim_layout",这种方式适用于所有的ViewGroup。
还可以通过LayoutAnimationController来实现,
Animation animation = AnimationUtils.loadAnimation(this,R.anim.anim_layout);
LayoutAnimationController controller = new LayoutAnimationController(animation);
controller.setDelay(0.5f);
controller.setOrder(LayoutAnimationController.ORDER_NORMAL);
recyclerView.setLayoutAnimation(controller);
2. 属性动画
关于属性动画框架工作原理的总结:
顾名思义只要某个类具有属性(即该类含有某个字段的set和get方法),那么属性动画框架就可以对该类的对象进行动画操作(其实就是通过反射技术来获取和执行属性的get,set方法),因此属性动画框架可以实现View动画框架的所有动画效果并且还能实现View动画框架无法实现的动画效果。属性动画框架工作原理可以总结为如下三步:
- 在创建属性动画时如果没有设置属性的初始值,此时Android系统就会通过该属性的get方法获取初始值,所以在没有设置属性的初始值时,必须提供该属性的get方法,否则程序会Crash。
- 在动画播放的过程中,属性动画框架会利用时间流逝的百分比获取属性值改变的百分比(即通过时间插值器),接着利用获取的属性值改变的百分比获取改变后的属性值(即通过类型估值器)。
- 通过该属性的set方法将改变后的属性值设置到对象中。
比较常用的几个动画类:ValueAnimator、ObjectAnimator和AnimationSet。其中ObjectAnimator继承自ValueAnimator,AnimatorSet是动画集合。
使用方式
代码实现
(1) 改变一个对象的translationY属性
ObjectAnimator.ofFloat(myObject, "translationY", -myObject.getHeight()).start();
(2)改变一个对象的背景色属性
ValueAnimator colorAnim = ObjectAnimator.ofInt(this, "backgroundColor", 0xFFFF8080, 0XFF8080FF);
colorAnim.serDuration(3000);
colorAnim.setEvaluator(new ArgbEvaluator());
colorAnim.setRepeatCount(ValueAnimator.INFINITE);
colorAnim.setRepeatMode(ValueAnimator.REVERSE);
colorAnim.start();
(3)动画集合,5秒内对View的旋转、平移、缩放和透明度进行改变
AnimatorSet set = new AnimatorSet();
set.playTogether(
// 绕X轴顺时针(右侧基准)旋转360度
ObjectAnimator.ofFloat(textView, "rotationX", 0, 360),
// 绕Y轴顺时针(俯视基准)旋转180度
ObjectAnimator.ofFloat(textView, "rotationY", 0, -180),
// 绕view中心,(正视基准)逆时针旋转90度
ObjectAnimator.ofFloat(textView, "rotation", 0, -90),
ObjectAnimator.ofFloat(textView, "translationX", 0, 90),
ObjectAnimator.ofFloat(textView, "translationY", 0, 90),
ObjectAnimator.ofFloat(textView, "scaleX", 1, 1.5f),
ObjectAnimator.ofFloat(textView, "scaleY", 1, 0.5f),
ObjectAnimator.ofFloat(textView, "alpha", 1, 0.25f, 1)
);
set.setDuration(5000).start();
(4)利用ObjectAnimator实现与补间动画中4个实例相同的动画效果,代码如下:
//利用ObjectAnimator实现透明度动画
ObjectAnimator.ofFloat(textView, "alpha", 1, 0, 1).setDuration(2000).start();
//利用AnimatorSet和ObjectAnimator实现缩放动画
final AnimatorSet animatorSet = new AnimatorSet();
textView.setPivotX(textView.getWidth() / 2);
textView.setPivotY(textView.getHeight() / 2);
animatorSet.playTogether(
ObjectAnimator.ofFloat(textView, "scaleX", 1, 0).setDuration(5000),
ObjectAnimator.ofFloat(textView, "scaleY", 1, 0).setDuration(5000));
animatorSet.start();
//利用AnimatorSet和ObjectAnimator实现平移动画
AnimatorSet animatorSet1 = new AnimatorSet();
animatorSet1.playTogether(
ObjectAnimator.ofFloat(textView, "translationX", 20, 100).setDuration(2000),
ObjectAnimator.ofFloat(textView, "translationY", 20, 100).setDuration(2000));
animatorSet1.start();
//利用ObjectAnimator实现旋转动画
textView.setPivotX(textView.getWidth() / 2);
textView.setPivotY(textView.getHeight() / 2);
ObjectAnimator.ofFloat(textView, "rotation", 0, 360).setDuration(1000).start();
XML定义
属性动画需要定义再res/animator/目录下,如下所示:
...
在XML中可以定义ValueAnimator\ObjectAnimator和AnimatorSet,其中
各标签属性介绍如下:
属性 | 描述 | 可选值 |
---|---|---|
android:ordering | 子动画的播放顺序 | together:同时播放,sequentially:按照前后顺序依次播放 |
属性 | 描述 | 可选值 |
---|---|---|
android:propertyName | 表示属性动画作用的作用对象的属性名称 | String. Required. |
android:duration | 动画的时长 | 默认值为300毫秒 |
android:valueFrom | 属性的起始值 | float, int, or color |
android:valueTo | 属性的结束值 | float, int, or color |
android:startOffset | 动画的延迟时间,当动画结束后需要延迟多少毫秒才真正播放 | |
android:repeatCount | 动画的重复次数 | 默认值是0,-1表示无限循环, |
android:repeatMode | 动画的重复模式 | restart和reverse,分别表示连续重复和逆向重复 |
android:valueType | 表示android:propertyName所指定的属性类型,有intType和floatType两个选项;如果android:propertyName所指定的属性表示的是颜色,则不需要指定android:valueType |
只是比
如何使用上述描述的动画呢?如下
AnimatorSet set1 = (AnimatorSet) AnimatorInflater.loadAnimator(this,R.animator.animator_set);
set1.setTarget(textView);
set1.start();
3. 帧动画
帧动画是播放一组预先定义好的图片,类似于电影播放。不同于View动画,系统提供了一个类AnimationDrawable来使用帧动画。首先通过一个xml来定义AnimationDrawable
属性介绍:
- android:oneshot
Boolean. 当设置为true时动画只会播放一次,否则会循环播放。
(1)然后将上述的drawable作为view背景并通过Drawable来播放动画即可:
Button button = (Button) findViewById(R.id.button);
button.setBackgroundResource(R.drawable.frame_animation);
AnimationDrawable drawable = (AnimationDrawable) button.getBackground();
drawable.start();
//animationDrawable.stop(); //如果oneshot为false,必要时要停止动画
(2)使用代码
//代码定义、创建、执行动画
AnimationDrawable animationDrawable = new AnimationDrawable();
animationDrawable.addFrame(getResources().getDrawable(R.drawable.first_pic), 1000);
animationDrawable.addFrame(getResources().getDrawable(R.drawable.second_pic), 1000);
animationDrawable.addFrame(getResources().getDrawable(R.drawable.third_pic), 1000);
animationDrawable.addFrame(getResources().getDrawable(R.drawable.fourth_pic), 1000);
animationDrawable.addFrame(getResources().getDrawable(R.drawable.fifth_pic), 1000);
animationDrawable.addFrame(getResources().getDrawable(R.drawable.sixth_pic), 1000);
animationDrawable.setOneShot(true);
image.setImageDrawable(animationDrawable);
animationDrawable.start();
注意:重要的是要注意,在Activity的onCreate()方法中不能调用AnimationDrawable的start()方法,因为AnimationDrawable尚未完全附加到窗口。 如果你想立即播放动画,而不需要交互,那么你可能想从Activity的onWindowFocusChanged()方法调用它,当Android将你的窗口聚焦时,它会被调用。