Python之函数进阶-nonlocal和LEGB
nonlocal语句
- nonlocal:将变量标记为不在本地作用域定义,而是在上级的某一级局部作用域中定义,但不能是全局作用域中定义。
函数的销毁
- 定义一个函数就是生成一个函数对象,函数名指向的就是函数对象。
- 可以使用del语句删除函数,使其引用计数减1。
- 可以使用同名标识符覆盖原有定义,本质上也是使其引用计数减1。
- Python程序结束时,所有对象销毁。
- 函数也是对象,也不例外,是否销毁,还是看引用计数是否减为0。
变量名解析原则LEGB
- Local,本地作用域、局部作用域的local命名空间。函数调用时创建,调用结束消亡
- Enclosing,Python2.2时引入了嵌套函数,实现了闭包,这个就是嵌套函数的外部函数的命名空间
- Global,全局作用域,即一个模块的命名空间。模块被import时创建,解释器退出时消亡
- Build-in,内置模块的命名空间,生命周期从python解释器启动时创建到解释器退出时消亡。例如 print(open),print和open都是内置的变量
图一:LEGB
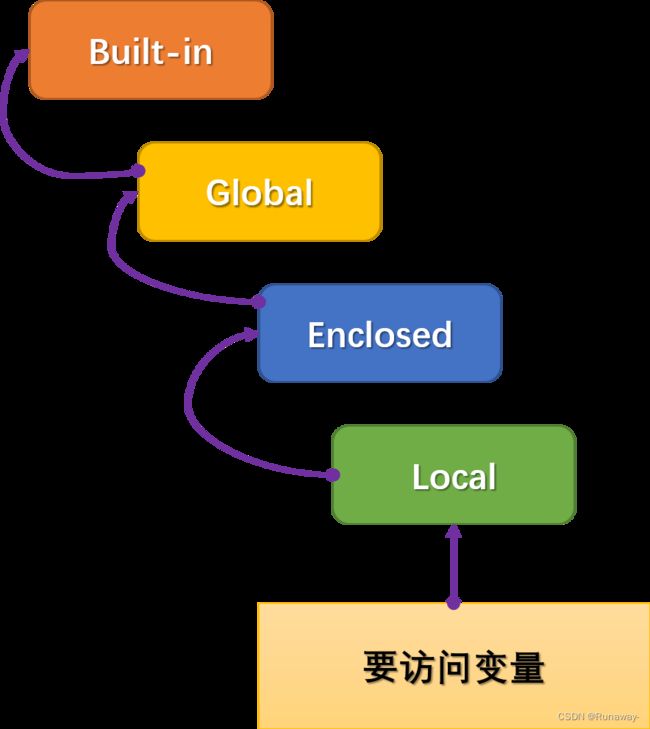
def inc():
c = 0
def inner():
c += 1
return c
return inner
f = inc()
print(1, f())
print(2, f())
def inc():
c = 0
def inner():
global c
c += 1
return c
return inner
f = inc()
print(1, f())
print(2, f())
def inc():
global c
c = 0
def inner():
global c
c += 1
return c
return inner
f = inc()
print(1, f())
print(2, f())
c
del c
def inc():
c = 0
def inner():
nonlocal c
c += 1
return c
return inner
f = inc()
print(1, f())
print(2, f())
def a():
nonlocal c
c = 100
print(a())
def inc():
a = 1000
c = 0
def inner():
nonlocal c
c += 1
return c
return inner
f = inc()
print(1, f())
print(2, f())
def inc():
a = 1000
c = 0
def inner():
nonlocal c
c += 1
return c
def t():
nonlocal a
print(a)
print(t.__closure__)
return inner
return t
f = inc()
print(1, f())
print(2, f())
c = 0
def a():
c = 1
def b():
c = 2
def c():
c = 3
print(c)