c++之json的创建,解析,增加,删除
-
- 1.存储方式,类型
- 2.宏
- 3.创建,保存json
- 4.解析
- 5.删除
- 6.修改
1.存储方式,类型
typedef struct cJSON {
struct cJSON *next,prev; / next是获取下一个元素数据,prev是获取前一个元素数据 */
struct cJSON child; / 获取第一个元素数据,当需要获取下一个时,就得使用next了. */
int type;
char *valuestring;
int valueint;
double valuedouble;
char *string;
} cJSON;
2.宏
#define cJSON_False 0
#define cJSON_True 1
#define cJSON_NULL 2
#define cJSON_Number 3
#define cJSON_String 4
#define cJSON_Array 5
#define cJSON_Object 6
3.创建,保存json
#include
#include
#include
#include
#include
#include
#include
#include "cJSON.h"
int main(){
cJSON* interest = cJSON_CreateObject();
cJSON_AddItemToObject(interest, "combat", cJSON_CreateString("热血"));
cJSON_AddItemToObject(interest, "reasoning", cJSON_CreateString("推理"));
cJSON* final = cJSON_CreateArray();
cJSON_AddItemToArray(final, cJSON_CreateString("BE"));
cJSON_AddItemToArray(final, cJSON_CreateString("HE"));
cJSON* likeObject1 = cJSON_CreateObject();
cJSON_AddItemToObject(likeObject1, "game", cJSON_CreateString("斩赤红之瞳"));
cJSON_AddItemToObject(likeObject1, "Episodes", cJSON_CreateNumber(22));
cJSON* likeObject2 = cJSON_CreateObject();
cJSON_AddItemToObject(likeObject2, "game", cJSON_CreateString("文豪野犬"));
cJSON_AddItemToObject(likeObject2, "Episodes", cJSON_CreateNumber(84));
cJSON* like = cJSON_CreateArray();
cJSON_AddItemToArray(like, likeObject1);
cJSON_AddItemToArray(like, likeObject2);
cJSON* education1 = cJSON_CreateArray();
cJSON_AddItemToArray(education1, cJSON_CreateString("战斗"));
cJSON_AddItemToArray(education1, cJSON_CreateString("热血"));
cJSON* education2 = cJSON_CreateArray();
cJSON_AddItemToArray(education2, cJSON_CreateString("推理"));
cJSON_AddItemToArray(education2, cJSON_CreateString("格斗"));
cJSON* education = cJSON_CreateArray();
cJSON_AddItemToArray(education, education1);
cJSON_AddItemToArray(education, education2);
cJSON* serialOne = cJSON_CreateObject();
cJSON_AddItemToObject(serialOne, "language", cJSON_CreateString("汉语"));
cJSON_AddItemToObject(serialOne, "grade", cJSON_CreateNumber(10));
cJSON* serialTwo = cJSON_CreateObject();
cJSON_AddItemToObject(serialTwo, "language", cJSON_CreateString("英语"));
cJSON_AddItemToObject(serialTwo, "grade", cJSON_CreateNumber(6));
cJSON* languages = cJSON_CreateObject();
cJSON_AddItemToObject(languages, "serialOne", serialOne);
cJSON_AddItemToObject(languages, "serialTwo", serialTwo);
cJSON* root = cJSON_CreateObject();
cJSON_AddItemToObject(root, "name", cJSON_CreateString("Blue"));
cJSON_AddItemToObject(root, "age", cJSON_CreateNumber(25));
cJSON_AddItemToObject(root, "interest", interest);
cJSON_AddItemToObject(root, "final", final);
cJSON_AddItemToObject(root, "like", like);
cJSON_AddItemToObject(root, "education", education);
cJSON_AddItemToObject(root, "languages", languages);
cJSON_AddItemToObject(root, "vip", cJSON_CreateTrue());
cJSON_AddItemToObject(root, "address", cJSON_CreateNull());
char* ALL_JSON = cJSON_Print(root);
char* ALL_JSONUnformatted = cJSON_PrintUnformatted(root);
printf("ALL_JSON:\n%s\n", ALL_JSON);
printf("ALL_JSONUnformatted:\n%s\n", ALL_JSONUnformatted);
free(ALL_JSON);
free(ALL_JSONUnformatted);
FILE* file = NULL;
file = fopen("E:\\abs\\test.json", "w");
if (file == NULL) {
printf("Open file fail!\n");
cJSON_Delete(root);
return;
}
char* cjValue = cJSON_Print(root);
int ret = fputs(cjValue, file);
if (ret == EOF) {
printf("写入文件失败!\n");
}
fclose(file);
free(cjValue);
cJSON_Delete(root);
}
打印:
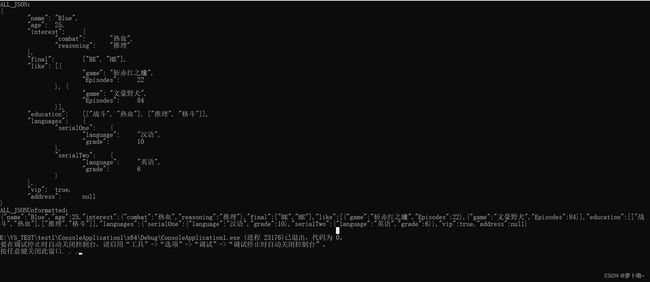
4.解析
#include
#include
#include
#include
#include
#include
#include
#include "cJSON.h"
using namespace std;
cJSON* item = NULL;
char* v_str = NULL;
double v_double = 0.0;
int v_int = 0;
bool v_bool = false;
void main(){
FILE* file = NULL;
const char* FILE_NAME = "E:\\abs\\test.json";
file = fopen(FILE_NAME, "r");
if (file == NULL) {
printf("Open file fail!\n");
return;
}
struct stat statbuf;
stat(FILE_NAME, &statbuf);
int fileSize = statbuf.st_size;
printf("文件大小:%d\n", fileSize);
char* jsonStr = (char*)malloc(sizeof(char) * fileSize + 1);
memset(jsonStr, 0, fileSize + 1);
int size = fread(jsonStr, sizeof(char), fileSize, file);
if (size == 0) {
printf("读取文件失败!\n");
fclose(file);
return;
}
cJSON* root = cJSON_Parse(jsonStr);
if (!root) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
free(jsonStr);
return;
}
free(jsonStr);
printf("*******************************1**************************************\n");
{
item = cJSON_GetObjectItem(root, "name");
if (item != NULL) {
if (item->type == cJSON_String) {
v_str = cJSON_Print(item);
printf("name = %s\n", v_str);
free(v_str);
v_str = NULL;
}
if (item->type == cJSON_String) {
v_str = item->valuestring;
printf("name = %s\n", v_str);
}
}
}
printf("*******************************2**************************************\n");
{
item = cJSON_GetObjectItem(root, "age");
if (item != NULL) {
if (item->type == cJSON_Number) {
v_int = item->valueint;
printf("age = %d\n", v_int);
}
}
}
printf("*******************************3**************************************\n");
{
item = cJSON_GetObjectItem(root, "vip");
if (item != NULL) {
if (item->type == cJSON_True || item->type == cJSON_False) {
v_str = cJSON_Print(item);
printf("vip = %s\n", v_str);
free(v_str);
v_str = NULL;
}
}
}
printf("*******************************4**************************************\n");
{
item = cJSON_GetObjectItem(root, "address");
if (item != NULL && item->type == cJSON_NULL) {
v_str = cJSON_Print(item);
printf("address = %s\n", v_str);
free(v_str);
v_str = NULL;
}
}
printf("*******************************5**************************************\n");
{
item = cJSON_GetObjectItem(root, "interest");
if (item != NULL) {
cJSON* val = NULL;
val = cJSON_GetObjectItem(item, "combat");
if (val != NULL && val->type == cJSON_String) {
v_str = val->valuestring;
printf("combat = %s\n", v_str);
}
val = cJSON_GetObjectItem(item, "reasoning");
if (val != NULL && val->type == cJSON_String) {
v_str = val->valuestring;
printf("reasoning = %s\n", v_str);
}
}
item = cJSON_GetObjectItem(root, "interest");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_String) {
char* v_str = obj->valuestring;
printf("%s = %s\n", obj->string, v_str);
}
obj = obj->next;
}
}
}
printf("*******************************6**************************************\n");
{
item = cJSON_GetObjectItem(root, "final");
if (item != NULL) {
int size = cJSON_GetArraySize(item);
for (int i = 0; i < size; i++) {
cJSON* arr = cJSON_GetArrayItem(item, i);
if (arr != NULL && arr->type == cJSON_String) {
v_str = arr->valuestring;
printf("final = %s\n", v_str);
}
}
}
item = cJSON_GetObjectItem(root, "final");
if (item != NULL) {
cJSON* arr = item->child;
while (arr) {
if (arr->type == cJSON_String) {
char* v_str = arr->valuestring;
printf("final = %s\n", v_str);
}
arr = arr->next;
}
}
}
printf("*******************************7**************************************\n");
{
item = cJSON_GetObjectItem(root, "like");
if (item != NULL) {
int size = cJSON_GetArraySize(item);
for (int i = 0; i < size; i++) {
cJSON* obj = cJSON_GetArrayItem(item, i);
cJSON* val = NULL;
if (obj != NULL && obj->type == cJSON_Object) {
val = cJSON_GetObjectItem(obj, "game");
if (val != NULL && val->type == cJSON_String) {
v_str = val->valuestring;
printf("game = %s\n", v_str);
}
val = cJSON_GetObjectItem(obj, "Episodes");
if (val != NULL && val->type == cJSON_Number) {
v_double = val->valuedouble;
printf("Episodes = %.2f\n", v_double);
}
}
}
}
item = cJSON_GetObjectItem(root, "like");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_Object) {
cJSON* objValue = obj->child;
while (objValue) {
if (objValue->type == cJSON_String) {
char* v_str = objValue->valuestring;
printf("%s = %s\n", objValue->string, v_str);
}
else if (objValue->type == cJSON_Number) {
double v_double = objValue->valuedouble;
printf("%s = %.2f\n", objValue->string, v_double);
}
objValue = objValue->next;
}
}
obj = obj->next;
}
}
}
printf("*******************************8**************************************\n");
{
item = cJSON_GetObjectItem(root, "education");
if (item != NULL) {
int size = cJSON_GetArraySize(item);
for (int i = 0; i < size; i++) {
cJSON* arr = cJSON_GetArrayItem(item, i);
if (arr != NULL && arr->type == cJSON_Array) {
int arrSize = cJSON_GetArraySize(arr);
for (int j = 0; j < arrSize; j++) {
cJSON* arr2 = cJSON_GetArrayItem(arr, j);
if (arr2 != NULL && arr2->type == cJSON_String) {
v_str = arr2->valuestring;
printf("education = %s\n", v_str);
}
}
}
}
}
item = cJSON_GetObjectItem(root, "education");
if (item != NULL) {
cJSON* arr = item->child;
while (arr) {
if (arr->type == cJSON_Array) {
cJSON* arrValue = arr->child;
while (arrValue) {
if (arrValue->type == cJSON_String) {
char* v_str = arrValue->valuestring;
printf("education = %s\n", v_str);
}
arrValue = arrValue->next;
}
}
arr = arr->next;
}
}
}
printf("*******************************9**************************************\n");
{
const char* arrStr[] = { "serialOne", "serialTwo" };
item = cJSON_GetObjectItem(root, "languages");
if (item != NULL) {
cJSON* val = NULL;
int size = sizeof(arrStr) / sizeof(char*);
for (int i = 0; i < size; i++) {
cJSON* obj1 = cJSON_GetObjectItem(item, arrStr[i]);
if (obj1 != NULL && obj1->type == cJSON_Object) {
val = cJSON_GetObjectItem(obj1, "language");
if (val != NULL && val->type == cJSON_String) {
v_str = val->valuestring;
printf("education = %s\n", v_str);
}
val = cJSON_GetObjectItem(obj1, "grade");
if (val != NULL && val->type == cJSON_Number) {
v_int = val->valueint;
printf("grade = %d\n", v_int);
}
}
}
}
item = cJSON_GetObjectItem(root, "languages");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_Object) {
cJSON* value = obj->child;
while (value) {
if (value->type == cJSON_String) {
printf("%s = %s\n", value->string, value->valuestring);
}
else if (value->type == cJSON_Number) {
printf("%s = %d\n", value->string, value->valueint);
}
value = value->next;
}
}
obj = obj->next;
}
}
}
fclose(file);
}
5.删除
#include
#include
#include
#include
#include
#include
#include
#include "cJSON.h"
void main() {
FILE* file = NULL;
const char* FILE_NAME = "E:\\abs\\test.json";
file = fopen(FILE_NAME, "r");
if (file == NULL) {
printf("Open file fail!\n");
return;
}
struct stat statbuf;
stat(FILE_NAME, &statbuf);
int fileSize = statbuf.st_size;
printf("文件大小:%d\n", fileSize);
char* jsonStr = (char*)malloc(sizeof(char) * fileSize + 1);
memset(jsonStr, 0, fileSize + 1);
int size = fread(jsonStr, sizeof(char), fileSize, file);
if (size == 0) {
printf("读取文件失败!\n");
fclose(file);
return;
}
printf("%s\n", jsonStr);
fclose(file);
cJSON* root = cJSON_Parse(jsonStr);
if (!root) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
free(jsonStr);
return;
}
free(jsonStr);
cJSON* item = NULL;
cJSON_ReplaceItemInObject(root, "name", cJSON_CreateString("蓝色"));
item = cJSON_GetObjectItem(root, "name");
if (item != NULL) {
if (item->type == cJSON_String) {
char* v_str = item->valuestring;
printf("name = %s\n", v_str);
}
}
cJSON_ReplaceItemInObject(root, "age", cJSON_CreateNumber(25));
item = cJSON_GetObjectItem(root, "age");
if (item != NULL) {
if (item->type == cJSON_Number) {
int v_int = item->valueint;
printf("age = %d\n", v_int);
}
}
cJSON_ReplaceItemInObject(root, "vip", cJSON_CreateBool(false));
item = cJSON_GetObjectItem(root, "vip");
if (item != NULL) {
if (item->type == cJSON_False || item->type == cJSON_True) {
char* v_str = cJSON_Print(item);
printf("vip = %s\n", v_str);
free(v_str);
v_str = NULL;
}
}
item = cJSON_GetObjectItem(root, "interest");
if (item != NULL) {
cJSON_ReplaceItemInObject(item, "combat", cJSON_CreateString("推理"));
cJSON_ReplaceItemInObject(item, "reasoning", cJSON_CreateString("热血"));
}
item = cJSON_GetObjectItem(root, "interest");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_String) {
char* v_str = obj->valuestring;
printf("%s = %s\n", obj->string, v_str);
}
obj = obj->next;
}
}
item = cJSON_GetObjectItem(root, "final");
if (item != NULL) {
cJSON_ReplaceItemInArray(item, 0, cJSON_CreateString("BEE"));
cJSON_ReplaceItemInArray(item, 1, cJSON_CreateString("HEE"));
}
item = cJSON_GetObjectItem(root, "final");
if (item != NULL) {
cJSON* arr = item->child;
while (arr) {
if (arr->type == cJSON_String) {
char* v_str = arr->valuestring;
printf("final = %s\n", v_str);
}
arr = arr->next;
}
}
item = cJSON_GetObjectItem(root, "like");
if (item != NULL) {
cJSON* arrObj = NULL;
arrObj = cJSON_GetArrayItem(item, 0);
cJSON_ReplaceItemInObject(arrObj, "game", cJSON_CreateString("钢之炼金术士FA"));
cJSON_ReplaceItemInObject(arrObj, "Episodes", cJSON_CreateNumber(64));
arrObj = cJSON_GetArrayItem(item, 1);
cJSON_ReplaceItemInObject(arrObj, "game", cJSON_CreateString("夏日重现"));
cJSON_ReplaceItemInObject(arrObj, "Episodes", cJSON_CreateNumber(25));
}
item = cJSON_GetObjectItem(root, "like");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_Object) {
cJSON* objValue = obj->child;
while (objValue) {
if (objValue->type == cJSON_String) {
char* v_str = objValue->valuestring;
printf("%s = %s\n", objValue->string, v_str);
}
else if (objValue->type == cJSON_Number) {
double v_double = objValue->valuedouble;
printf("%s = %.2f\n", objValue->string, v_double);
}
objValue = objValue->next;
}
}
obj = obj->next;
}
}
item = cJSON_GetObjectItem(root, "education");
if (item != NULL) {
cJSON* arrArr = NULL;
arrArr = cJSON_GetArrayItem(item, 0);
cJSON_ReplaceItemInArray(arrArr, 0, cJSON_CreateString("小学六年级"));
cJSON_ReplaceItemInArray(arrArr, 1, cJSON_CreateString("初中初三"));
arrArr = cJSON_GetArrayItem(item, 1);
cJSON_ReplaceItemInArray(arrArr, 0, cJSON_CreateString("高中高三"));
cJSON_ReplaceItemInArray(arrArr, 1, cJSON_CreateString("大学大四"));
}
item = cJSON_GetObjectItem(root, "education");
if (item != NULL) {
cJSON* arr = item->child;
while (arr) {
if (arr->type == cJSON_Array) {
cJSON* arrValue = arr->child;
while (arrValue) {
if (arrValue->type == cJSON_String) {
char* v_str = arrValue->valuestring;
printf("education = %s\n", v_str);
}
arrValue = arrValue->next;
}
}
arr = arr->next;
}
}
item = cJSON_GetObjectItem(root, "languages");
if (item != NULL) {
cJSON* obj = NULL;
obj = cJSON_GetObjectItem(item, "serialOne");
cJSON_ReplaceItemInObject(obj, "language", cJSON_CreateString("粤语"));
cJSON_ReplaceItemInObject(obj, "grade", cJSON_CreateNumber(9));
obj = cJSON_GetObjectItem(item, "serialTwo");
cJSON_ReplaceItemInObject(obj, "language", cJSON_CreateString("白话"));
cJSON_ReplaceItemInObject(obj, "grade", cJSON_CreateNumber(8));
}
item = cJSON_GetObjectItem(root, "languages");
if (item != NULL) {
cJSON* obj = item->child;
while (obj) {
if (obj->type == cJSON_Object) {
cJSON* value = obj->child;
while (value) {
if (value->type == cJSON_String) {
printf("%s = %s\n", value->string, value->valuestring);
}
else if (value->type == cJSON_Number) {
printf("%s = %d\n", value->string, value->valueint);
}
value = value->next;
}
}
obj = obj->next;
}
}
file = fopen(FILE_NAME, "w");
if (file == NULL) {
printf("Open file fail!\n");
cJSON_Delete(root);
return;
}
char* cjValue = cJSON_Print(root);
int ret = fwrite(cjValue, sizeof(char), strlen(cjValue), file);
if (ret == 0) {
printf("写入文件失败!\n");
}
fclose(file);
free(cjValue);
cJSON_Delete(root);
}
6.修改
#include
#include
#include
#include
#include
#include
#include
#include "cJSON.h"
void main() {
FILE* file = NULL;
const char* FILE_NAME = "E:\\abs\\test.json";
file = fopen(FILE_NAME, "r");
if (file == NULL) {
printf("Open file fail!\n");
return;
}
struct stat statbuf;
stat(FILE_NAME, &statbuf);
int fileSize = statbuf.st_size;
printf("文件大小:%d\n", fileSize);
char* jsonStr = (char*)malloc(sizeof(char) * fileSize + 1);
memset(jsonStr, 0, fileSize + 1);
int size = fread(jsonStr, sizeof(char), fileSize, file);
if (size == 0) {
printf("读取文件失败!\n");
fclose(file);
return;
}
printf("%s\n", jsonStr);
fclose(file);
cJSON* root = cJSON_Parse(jsonStr);
if (!root) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
free(jsonStr);
return;
}
free(jsonStr);
cJSON* item = NULL;
cJSON_DeleteItemFromObject(root, "name");
item = cJSON_GetObjectItem(root, "interest");
if (item != NULL) {
cJSON_DeleteItemFromObject(item, "combat");
}
item = cJSON_GetObjectItem(root, "final");
if (item != NULL) {
cJSON_DeleteItemFromArray(item, 1);
}
item = cJSON_GetObjectItem(root, "like");
if (item != NULL) {
cJSON_DeleteItemFromArray(item, 0);
}
item = cJSON_GetObjectItem(root, "education");
if (item != NULL) {
cJSON_DeleteItemFromArray(item, 1);
}
item = cJSON_GetObjectItem(root, "languages");
if (item != NULL) {
cJSON_DeleteItemFromObject(item, "serialTwo");
}
file = fopen(FILE_NAME, "w");
if (file == NULL) {
printf("Open file fail!\n");
cJSON_Delete(root);
return;
}
char* cjValue = cJSON_Print(root);
int ret = fwrite(cjValue, sizeof(char), strlen(cjValue), file);
if (ret == 0) {
printf("写入文件失败!\n");
}
fclose(file);
free(cjValue);
cJSON_Delete(root);
}