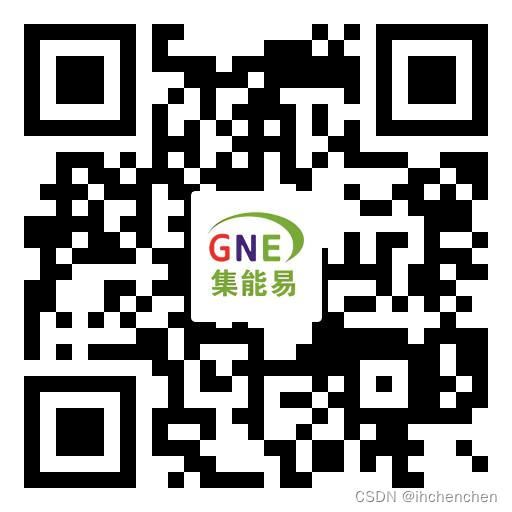

一、导入zxing包
com.google.zxing
core
3.5.2
com.google.zxing
javase
3.5.2
二、生成带logo的二维码工具类
package com.example.demo.utils;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.RoundRectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.util.Hashtable;
public class QrCodeUtil {
//编码格式,采用utf-8
private static final String UNICODE = "utf-8";
//图片格式
private static final String FORMAT = "JPG";
//二维码宽度像素pixels数量
private static final int QRCODE_WIDTH = 512;
//二维码高度像素pixels数量
private static final int QRCODE_HEIGHT = 512;
//LOGO宽度像素pixels数量
private static final int LOGO_WIDTH = 128;
//LOGO高度像素pixels数量
private static final int LOGO_HEIGHT = 128;
/**
* 生成二维码图片
* @param content 二维码内容
* @param logoPath logo图片地址
* @return
* @throws Exception
*/
private static BufferedImage createQrCode(String content, String logoPath) throws Exception {
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, UNICODE);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content,
BarcodeFormat.QR_CODE, QRCODE_WIDTH, QRCODE_HEIGHT,
hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
if (logoPath == null || "".equals(logoPath)) {
return image;
}
// 插入图片
insertLogo(image, logoPath);
return image;
}
//在图片上插入LOGO
//source 二维码图片内容
//logoPath LOGO图片地址
private static void insertLogo(BufferedImage source, String logoPath) throws Exception {
File file = new File(logoPath);
if (!file.exists()) {
throw new Exception("logo file not found.");
}
Image src = ImageIO.read(new File(logoPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (width > LOGO_WIDTH) {
width = LOGO_WIDTH;
}
if (height > LOGO_HEIGHT) {
height = LOGO_HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); // 绘制缩小后的图
g.dispose();
src = image;
// 插入LOGO
Graphics2D graph = source.createGraphics();
setGraphics2D(graph);
int x = (QRCODE_WIDTH - width) / 2;
int y = (QRCODE_HEIGHT - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
/**
* 设置 Graphics2D 属性 (抗锯齿)
*
* @param g2d Graphics2D提供对几何形状、坐标转换、颜色管理和文本布局更为复杂的控制
*/
private static void setGraphics2D(Graphics2D g2d) {
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setRenderingHint(RenderingHints.KEY_STROKE_CONTROL, RenderingHints.VALUE_STROKE_DEFAULT);
Stroke s = new BasicStroke(1, BasicStroke.CAP_ROUND, BasicStroke.JOIN_MITER);
g2d.setStroke(s);
}
public static void main(String[] args) {
try {
ImageIO.write(createQrCode("www.gnetek.com", "D:/logo.png"), FORMAT, new File("D:/bbb.jpg"));
} catch (Exception e) {
e.printStackTrace();
}
}
}
三、生成带内容的条形码工具类
package com.example.demo.utils;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.oned.Code128Writer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.Hashtable;
public class BarCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT = "JPG";
/**
* 条形码宽度
*/
private static final int WIDTH = 512;
/**
* 条形码高度
*/
private static final int HEIGHT = 128;
/**
* 字体大小
*/
private static final int FONT_SIZE = 32;
/**
* 加文字 条形码
*/
private static final int WORD_HEIGHT = HEIGHT + FONT_SIZE;
public static BufferedImage createBarCode(String code){
Hashtable hints = new Hashtable<>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
Code128Writer writer = new Code128Writer();
// 编码内容, 编码类型, 宽度, 高度, 设置参数
BitMatrix bitMatrix = writer.encode(code, BarcodeFormat.CODE_128, WIDTH, HEIGHT, hints);
BufferedImage image = MatrixToImageWriter.toBufferedImage(bitMatrix);
BufferedImage outImage = new BufferedImage(WIDTH, WORD_HEIGHT, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = outImage.createGraphics();
// 抗锯齿
setGraphics2D(g2d);
// 设置白色
setColorWhite(g2d);
// 画条形码到新的面板
g2d.drawImage(image, 0, 5, image.getWidth(), image.getHeight(), null);
// 画文字到新的面板
g2d.setColor(Color.BLACK);
// 字体、字型、字号
g2d.setFont(new Font("黑体", Font.PLAIN, FONT_SIZE));
//设置条形码下面的文本的纵坐标
int wordStartY = HEIGHT + 26;
String[] split = code.split("");
int len = split.length;
int stepWidth = WIDTH / len;
for (int i = 0; i < len; i++) {
int x = stepWidth*(i+1);
g2d.drawString(split[i], x, wordStartY);
}
outImage.flush();
return outImage;
}
/**
* 设置 Graphics2D 属性 (抗锯齿)
*
* @param g2d Graphics2D提供对几何形状、坐标转换、颜色管理和文本布局更为复杂的控制
*/
private static void setGraphics2D(Graphics2D g2d) {
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setRenderingHint(RenderingHints.KEY_STROKE_CONTROL, RenderingHints.VALUE_STROKE_DEFAULT);
Stroke s = new BasicStroke(1, BasicStroke.CAP_ROUND, BasicStroke.JOIN_MITER);
g2d.setStroke(s);
}
/**
* 设置背景为白色
*
* @param g2d Graphics2D提供对几何形状、坐标转换、颜色管理和文本布局更为复杂的控制
*/
private static void setColorWhite(Graphics2D g2d) {
g2d.setColor(Color.WHITE);
// 填充整个屏幕
g2d.fillRect(0, 0, 600, 600);
// 设置笔刷
g2d.setColor(Color.BLACK);
}
public static void main(String[] args) {
try {
ImageIO.write(createBarCode("9111119911"), FORMAT, new File("D:/aaa.jpg"));
} catch (IOException e) {
e.printStackTrace();
}
}
}