一、下载sdk
服务端SDK下载 - 钉钉开放平台
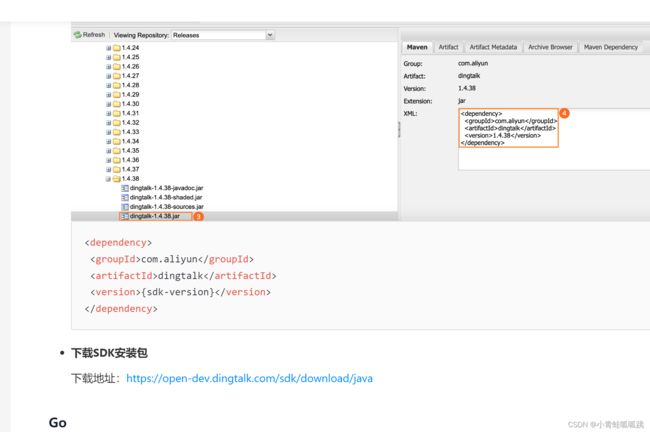
二、将包加入本地仓库
mvn org.apache.maven.plugins:maven-install-plugin:2.5.2:install-file \
-Dfile=D:\taobao-sdk-java-auto_1479188381469-20211105.jar \
-DgroupId=com.aliyun \
-DartifactId=dingtalk \
-Dversion=1.1.96 \
-Dpackaging=jar
pom.xml
com.aliyun
dingtalk
1.1.96
三、代码
package com.sdmktech.mep.energy.monitor.utils;
import cn.hutool.http.HttpUtil;
import com.alibaba.fastjson.JSONObject;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import org.apache.commons.codec.binary.Base64;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.util.StringUtils;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.text.SimpleDateFormat;
import java.util.*;
/**
* 自定义钉钉群组发送消息
*
*/
public class DingTalkPushUtilDemo {
/**
* 日志注入
*/
private static final Logger logger = LoggerFactory.getLogger(DingTalkPushUtilDemo.class);
/**
* 群机器人复制到的秘钥secret
*/
private static final String SECRET = "bacb272562e9fb003cc68ebcff69dd99965de71a7b98ead92eae8a73135e5a13hlw";
/**
* 配置机器人的webhook
*/
private static final String WEBHOOK = "https://oapi.dingtalk.com/robot/send?access_token="+SECRET;
private static final String dingTemplate = "**告警名称** : %s \n\n **告警级别** : warning \n\n " +
"**告警内容** : %s \n\n **告警时间** : %s \n\n ";
private static SimpleDateFormat format1 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
public static void main(String[] args) {
//testSendTextMsg();
testSendMarkdownMsg();
}
/**
* 文本消息
*/
public static void testSendTextMsg() {
try {
sendTextMsg(String.format(dingTemplate,"内存溢出","内存使用超过90%",format1.format(new Date())), Lists.newArrayList(), Lists.newLinkedList(), false);} catch (Exception e) {
logger.error("钉钉群消息发送异常,异常原因:{}", e.toString());
}
}
/**
* link类型
*/
public static void testSendLinkMsg() {
try {
sendLinkMsg("时代的火车向前开",
"这个即将发布的新版本,创始人xx称它为红树林。而在此之前,每当面临重大升级,产品经理们都会取一个应景的代号,这一次,为什么是红树林",
"https://www.dingtalk.com/s?__biz=MzA4NjMwMTA2Ng==&mid=2650316842&idx=1&sn=60da3ea2b29f1dcc43a7c8e4a7c97a16&scene=2&srcid=09189AnRJEdIiWVaKltFzNTw&from=timeline&isappinstalled=0&key=&ascene=2&uin=&devicetype=android-23&version=26031933&nettype=WIFI",
"");
} catch (Exception e) {
logger.error("钉钉群消息发送异常,异常原因:{}", e.toString());
}
}
/**
* 整体跳转ActionCard类型
*/
public static void testWholeSendActionCardMsg() {
try {
sendActionCardMsg("打造一间咖啡厅", "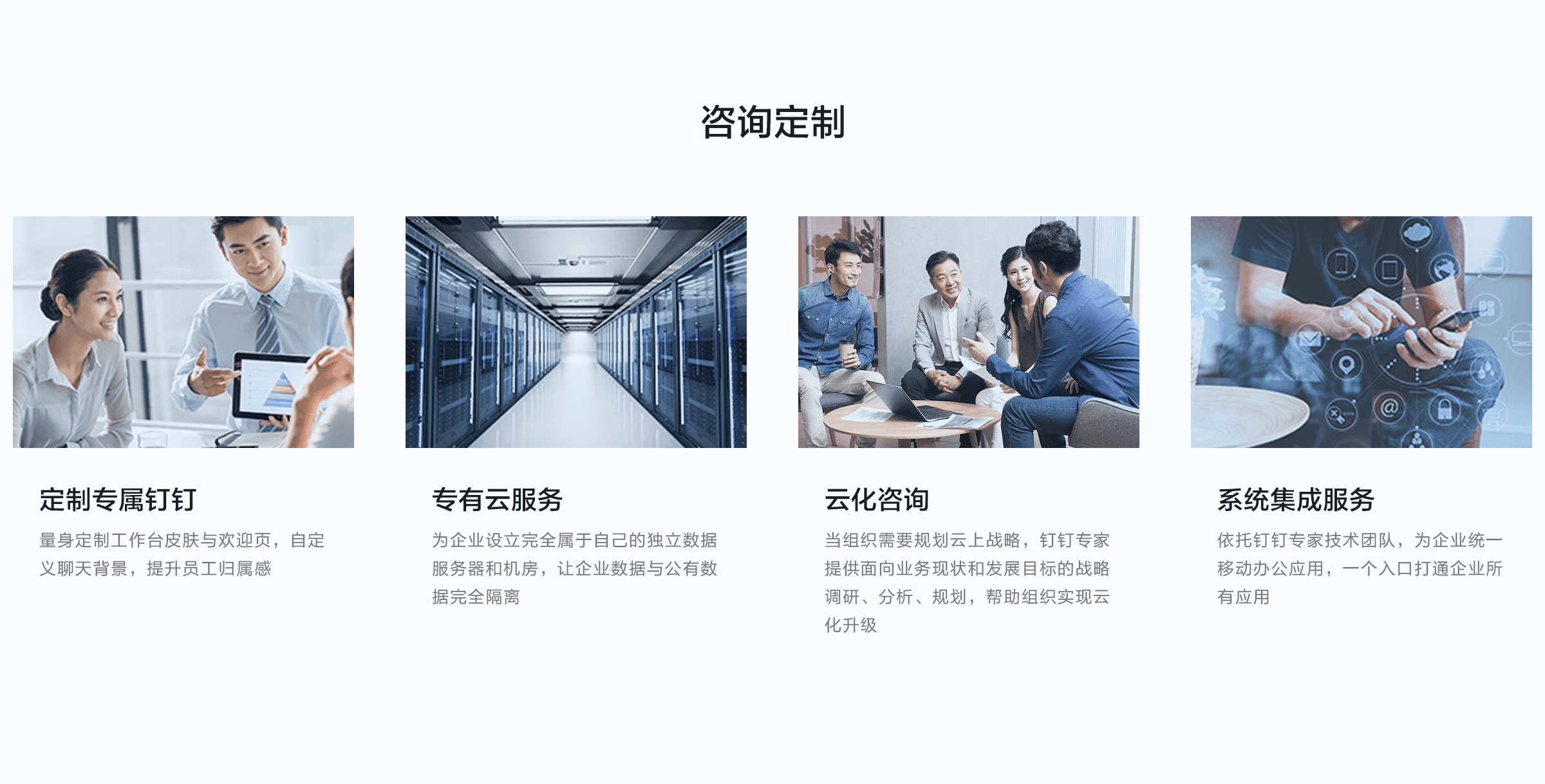 \\n #### 乔布斯 20 年前想打造的苹果咖啡厅 \\n\\n Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划",
"0", "阅读全文", "https://www.dingtalk.com/");
} catch (Exception e) {
logger.error("钉钉群消息发送异常,异常原因:{}", e.toString());
}
}
/**
* 独立跳转ActionCard类型
*/
public static void testIndependentSendActionCardMsg() {
try {
List