Go基本数据类型
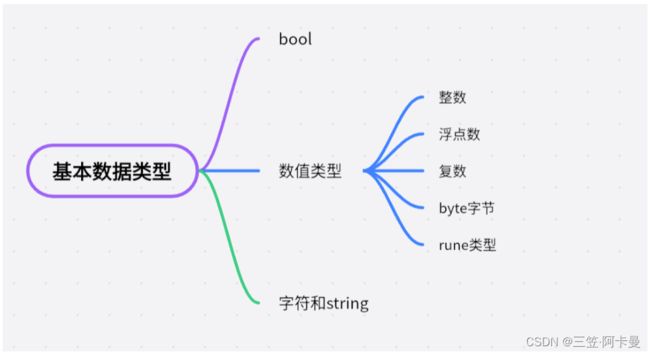
bool
bool型值可以为true
或者false
,例子:
var b bool = true
数值型
类型 |
表示 |
范围 |
int8 |
有符号8位整型 |
-128 ~ 127 |
int16 |
有符号16位整型 |
-32768 ~ 32767 |
int32 |
有符号32位整型 |
-2147783648 ~ 2147483647 |
int64 |
有符号64位整型 |
··· |
uint8 |
无符号8位整型 |
0 ~ 255 |
uint16 |
无符号16位整型 |
0 ~ 65535 |
uint32 |
无符号32位整型 |
0 ~ 4294967295 |
uint64 |
无符号64位整型 |
··· |
浮点型
类型 |
表示 |
float32 |
32位浮点数 |
float64 |
64位浮点数 |
其他
类型 |
表示 |
byte |
uint8 |
rune |
int32 |
数据类型的转换
简单的转换操作
package main
import (
"fmt"
"strconv"
)
func main() {
var a int8 = 12
var b = uint8(a)
var f float32 = 3.14
var c = int32(f)
fmt.Println(b, c)
var f64 = float64(a)
fmt.Println(f64)
type IT int
var d IT = 13
fmt.Println(d)
var istr = "12a"
mint, err := strconv.Atoi(istr)
if err != nil {
fmt.Println("convert error!")
} else {
fmt.Println(mint)
}
var myi = 32
mstr := strconv.Itoa(myi)
fmt.Println(mstr)
fl, err2 := strconv.ParseFloat("3.1415926", 64)
if err2 != nil {
return
}
fmt.Println(fl)
parseInt, err3 := strconv.ParseInt("-42", 10, 64)
if err3 != nil {
return
}
fmt.Println(parseInt)
parseBool, parseBoolErr := strconv.ParseBool("true")
if parseBoolErr != nil {
return
}
fmt.Println(parseBool)
boolStr := strconv.FormatBool(true)
fmt.Println(boolStr)
floatStr := strconv.FormatFloat(3.1415926, 'f', -1, 64)
fmt.Println(floatStr)
fmt.Println(strconv.FormatInt(42, 16))
}
运算符和表达式
package main
import "fmt"
func main() {
var a, b = 1, 2
fmt.Println(a + b)
var astr, bstr = "hello", "zed"
fmt.Println(astr + bstr)
fmt.Println(3 % 2)
a++
fmt.Println(a)
var abool, bbool = true, false
fmt.Println(abool && bbool)
fmt.Println(abool || bbool)
fmt.Println(!abool)
var A = 60
var B = 13
fmt.Println(A & B)
}
字符串
格式化输出
package main
import (
"fmt"
"strconv"
"strings"
)
func main() {
courseName := "go\"体系课\""
fmt.Println(courseName)
username := "bobby"
out := "hello" + username
fmt.Println(out)
age := 18
address := "北京"
fmt.Println("用户名: " + username + "," + "年龄: " + strconv.Itoa(age) + ",地址: " + address)
fmt.Printf("用户名: %s, 年龄: %d, 地址: %s", username, age, address)
userMsg := fmt.Sprintf("用户名: %s, 年龄: %d, 地址: %s", username, age, address)
fmt.Println(userMsg)
var builder strings.Builder
builder.WriteString("用户名: ")
builder.WriteString(username)
builder.WriteString(", 年龄: ")
builder.WriteString(strconv.Itoa(age))
builder.WriteString(", 地址: ")
builder.WriteString(address)
re := builder.String()
fmt.Println(re)
}
字符串的比较
package main
import "fmt"
func main() {
a := "hello"
b := "hello"
fmt.Println(a == b)
c := "bello"
fmt.Println(a > c)
}
字符串的操作方法
package main
import (
"fmt"
"strings"
)
func main() {
a := "hello"
b := "hello"
fmt.Println(a == b)
c := "bello"
fmt.Println(a > c)
name := "goland-工程师"
isContains := strings.Contains(name, "goland")
fmt.Println(isContains)
fmt.Println(strings.Count(name, "o"))
fmt.Println(strings.Split(name, "-"))
fmt.Println(strings.HasPrefix(name, "g"))
fmt.Println(strings.HasSuffix(name, "g"))
fmt.Println(strings.Index(name, "师"))
fmt.Println(strings.Replace(name, "goland", "java", 1))
fmt.Println(strings.ToLower(name))
fmt.Println(strings.ToUpper(name))
fmt.Println(strings.Trim("hello go ", " "))
}
条件判断与for循环
条件判断
package main
import "fmt"
func main() {
age := 22
country := "中国"
if age < 18 && country == "中国" {
fmt.Println("未成年人")
} else if age == 18 {
fmt.Println("刚好是成年人")
} else {
fmt.Println("成年人")
}
}
for循环
package main
import "fmt"
func main() {
for i := 0; i < 3; i++ {
fmt.Println(i)
}
for i := 1; i <= 9; i++ {
for j := 1; j <= i; j++ {
fmt.Printf("%d * %d = %d ", i, j, i*j)
}
fmt.Println()
}
name := "hello, go"
for index, value := range name {
fmt.Printf("%d %c\r\n", index, value)
}
fmt.Println("----------------------------")
for _, value := range name {
fmt.Printf("%c\r\n", value)
}
fmt.Println("----------------------------")
for index := range name {
fmt.Printf("%c\r\n", name[index])
}
}
goto
package main
import "fmt"
func main() {
for i := 0; i < 5; i++ {
for j := 0; j < 4; j++ {
if j == 2 {
goto over
}
fmt.Println(i, j)
}
}
over:
fmt.Println("over")
}
switch
package main
import "fmt"
func main() {
day := "周五"
switch day {
case "周一":
fmt.Println("Mongday")
case "周五":
fmt.Println("Friday")
case "周三":
fmt.Println("Wednesday")
default:
fmt.Println("Saturday")
}
score := 95
switch {
case score < 60:
fmt.Println("E")
case score >= 60 && score < 70:
fmt.Println("D")
case score >= 70 && score < 80:
fmt.Println("C")
case score >= 80 && score < 90:
fmt.Println("b")
case score >= 90 && score <= 100:
fmt.Println("A")
}
switch score {
case 60, 70, 80:
fmt.Println("牛")
default:
fmt.Println("牛牛")
}
}
Go语言的容器
数组、切片(slice)、map、list
Go–数组
package main
import "fmt"
func main() {
var courses1 [3]string
var courses2 [4]string
fmt.Printf("%T\r\n", courses1)
fmt.Printf("%T\r\n", courses2)
courses1[0] = "go"
courses1[1] = "grpc"
courses1[2] = "gin"
fmt.Println(courses1)
for _, value := range courses1 {
fmt.Println(value)
}
}
Go–Slice切片
package main
import "fmt"
func main() {
var courses []string
fmt.Printf("%T\r\n", courses)
courses = append(courses, "Java", "Python", "Golang")
fmt.Println(courses)
fmt.Println(courses[2])
coursesSlice := []string{"Java", "Go", "mysql", "Kafka", "Redis", "ElasticSearch"}
fmt.Println(coursesSlice[1:3])
fmt.Println(coursesSlice[0:])
fmt.Println(coursesSlice[:3])
fmt.Println(coursesSlice[:])
coursesSlice2 := []string{"go", "grpc"}
coursesSlice3 := []string{"python", "kafka"}
for _, val := range coursesSlice3 {
coursesSlice2 = append(coursesSlice2, val)
}
coursesSlice2 = append(coursesSlice2, coursesSlice3...)
coursesSlice2 = append(coursesSlice2, "gin", "mysql", "es")
fmt.Println(coursesSlice2)
coursesSlice4 := []string{"go", "Java", "Python", "MySQL"}
newSlice := append(coursesSlice4[:2], coursesSlice4[3:]...)
fmt.Println(newSlice)
coursesSlice4Copy := coursesSlice4
coursesSlice4Copy2 := coursesSlice4[:]
fmt.Println(coursesSlice4Copy)
fmt.Println(coursesSlice4Copy2)
var newCoursesSliceCopy = make([]string, len(coursesSlice4))
copy(newCoursesSliceCopy, coursesSlice4)
fmt.Println(newCoursesSliceCopy)
fmt.Println("--------直接赋值和使用copy函数的区别--------")
coursesSlice4[0] = "golang"
fmt.Println(coursesSlice4Copy[0])
fmt.Println(newCoursesSliceCopy[0])
}
切片在函数参数传递时是值传递还是引用传递
package main
import (
"fmt"
"strconv"
)
func printSlice(data []string) {
data[0] = "java"
for i := 0; i < 10; i++ {
data = append(data, strconv.Itoa(i))
}
}
func main() {
courses := []string{"go", "grpc", "gin"}
printSlice(courses)
fmt.Println(courses)
}
Go容器–map
package main
import "fmt"
func main() {
var coursesMap = map[string]string{
"go": "golang工程师",
"grpc": "grpc入门",
"gin": "gin深入理解",
}
fmt.Println(coursesMap["grpc"])
coursesMap["mysql"] = "mysql原理"
var nullMap2 = map[string]string{}
nullMap2["mysql"] = "mysql原理"
fmt.Println(nullMap2)
var nullMap3 = make(map[string]string, 3)
nullMap3["mysql"] = "mysql原理"
fmt.Println(nullMap3)
var slirceTest []string
if slirceTest == nil {
fmt.Println("slirceTest is nil")
}
slirceTest = append(slirceTest, "a")
fmt.Println("-------------map的遍历---------------")
for key, value := range coursesMap {
fmt.Println(key, value)
}
fmt.Println("-------------map的遍历,只打印key---------------")
for key := range coursesMap {
fmt.Println(key, coursesMap[key])
}
fmt.Println("-------------map寻找key不存在的数据---------------")
d := coursesMap["java"]
fmt.Println(d)
if _, ok := coursesMap["java"]; !ok {
fmt.Println("not in")
} else {
fmt.Println("in")
}
delete(coursesMap, "grpc")
fmt.Println(coursesMap)
delete(coursesMap, "rpc")
}
Go容器 – list(链表)
package main
import (
"container/list"
"fmt"
)
func main() {
var mylist = list.List{}
mylist.PushBack("go")
mylist.PushBack("grpc")
mylist.PushBack("gin")
fmt.Println(mylist)
for i := mylist.Front(); i != nil; i = i.Next() {
fmt.Println(i.Value)
}
fmt.Println("----------反向遍历----------")
for i := mylist.Back(); i != nil; i = i.Prev() {
fmt.Println(i.Value)
}
fmt.Println("----------初始化list的方法----------")
newList := list.New()
newList.PushFront("mysql")
for i := newList.Front(); i != nil; i = i.Next() {
fmt.Println(i.Value)
}
fmt.Println("----------插入指定元素之前或者之后----------")
i := newList.Front()
for ; i != nil; i = i.Next() {
if i.Value.(string) == "mysql" {
break
}
}
newList.InsertBefore("oracle", i)
for i := newList.Front(); i != nil; i = i.Next() {
fmt.Println(i.Value)
}
fmt.Println("----------newList删除----------")
newList.Remove(i)
for i := newList.Front(); i != nil; i = i.Next() {
fmt.Println(i.Value)
}
}