缓动系统
import { _decorator, v3, tween, Component, EventTouch, Node, log } from 'cc';
const { ccclass, property } = _decorator;
@ccclass('GameRoot')
export class GameRoot extends Component {
@property(Node) button: Node;
@property(Node) a: Node;
start() {
this.button.on(Node.EventType.TOUCH_START, (event: EventTouch) => {
this.button.setScale(0.9, 0.9)
})
this.button.on(Node.EventType.TOUCH_END, (event: EventTouch) => {
this.button.setScale(1, 1)
this.myAnimation()
})
}
myAnimation() {
tween(this.a)
.to(1, { position: v3(0, 200, 0) })
.start()
const obj = {
n: 0
}
tween(obj)
.to(3, { n: 1000 }, {
onUpdate: (target, ratio) => {
console.log(target,ratio);
}
})
.start()
}
update(deltaTime: number) {
}
}
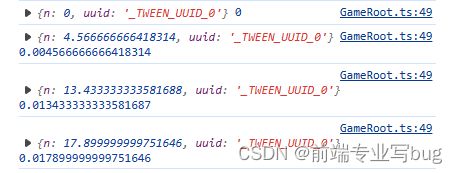
修改label组件文字 0 ~ 1000
import { _decorator, v3, tween, Component, EventTouch, Node, log,Label } from 'cc';
@property(Node) LableNode: Node;
const comp = this.LableNode.getComponent(Label)
tween(obj)
.to(3, { n: 1000 }, {
onUpdate: (target, ratio) => {
console.log(target,ratio);
comp.string = `${obj.n}`
}
})
.start()
加入动画
tween(obj)
.to(3, { n: 200 }, {
onUpdate: (target, ratio) => {
console.log(target,ratio);
comp.string = `${obj.n.toFixed(2)}`
},
easing:'elasticOut'
})
.start()
跳跃效果 tween
tween(obj)
.to(3, { n: 200 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadOut'
})
.to(3, { n: 0 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadIn'
})
.start()
直接拿到Label
@property(Label) LableCom: Label;
const comp = this.LableCom
循环跳跃 tween
tween(obj)
.to(0.5, { n: 200 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadOut'
})
.to(0.5, { n: 0 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadIn'
})
.union()
.repeatForever()
.start()
循环跳跃第二种写法
tween(obj)
.repeatForever(
tween(obj)
.to(0.5, { n: 200 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadOut'
})
.to(0.5, { n: 0 }, {
onUpdate: (target, ratio) => {
this.a.setPosition(0,obj.n)
},
easing:'quadIn'
})
)
.start()
停止tween tag
tween(obj)
.tag(111)
.to(10,{n:this.a.position.x - 300}, {
onUpdate: (target, ratio) => {
this.a.setPosition(obj.n,this.a.position.y)
},
})
.start()
Tween.stopAllByTag(111)
停止tween 方法二 this.leftTween.stop()
import { _decorator, v3, tween, Component, EventTouch, Node, log,Label, Tween } from 'cc';
leftTween: Tween<object>
this.leftTween = tween(obj)
.to(10,{n:this.a.position.x - 300}, {
onUpdate: (target, ratio) => {
this.a.setPosition(obj.n,this.a.position.y)
},
})
.start()
this.leftTween.stop()
例如颜色变换
const color = new Vec3(255,255,255)
tween(color)
.to(3,{x:10,y:50,z:0},{
onUpdate:()=> {
console.log(color.x,color.y,color.z);
this.LableCom.color = new Color(color.x,color.y,color.z)
},
})
.start()
循环变色
tween(color)
.to(3,{x:10,y:50,z:0},{
onUpdate:()=> {
console.log(color.x,color.y,color.z);
this.LableCom.color = new Color(color.x,color.y,color.z)
},
})
.to(3,{x:10,y:50,z:100},{
onUpdate:()=> {
console.log(color.x,color.y,color.z);
this.LableCom.color = new Color(color.x,color.y,color.z)
},
})
.union()
.repeatForever()
.start()