剑指Offer
剑指Offer 06.从尾到头打印链表
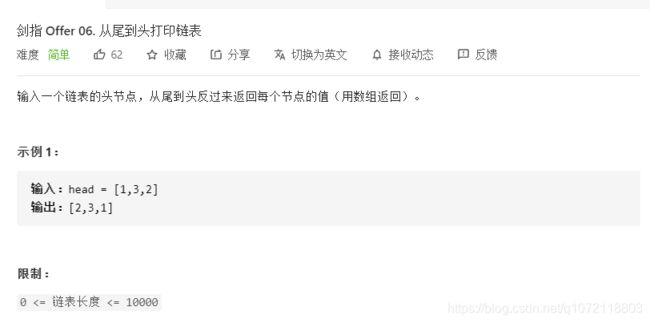
栈
class Solution {
public:
vector<int> reversePrint(ListNode* head) {
int top = -1;
int st[10010];
ListNode* p = head;
while(p!=NULL)
{
st[++top] = p->val;
p = p->next;
}
vector<int> ans(top+1);
int k = 0;
while(top!=-1)
ans[k++] = st[top--];
return ans;
}
};
递归
class Solution {
public:
int k=0;
vector<int> res;
void getLength(ListNode* head)
{
int cnt = 0;
ListNode* p = head;
while(p!=NULL)
{
cnt++;
p = p->next;
}
res.resize(cnt);
}
void reverseP(ListNode* head)
{
if(!head)
return;
reverseP(head->next);
res[k++] = (head->val);
}
vector<int> reversePrint(ListNode* head) {
getLength(head);
reverseP(head);
return res;
}
};
剑指Offer 18.删除链表的节点
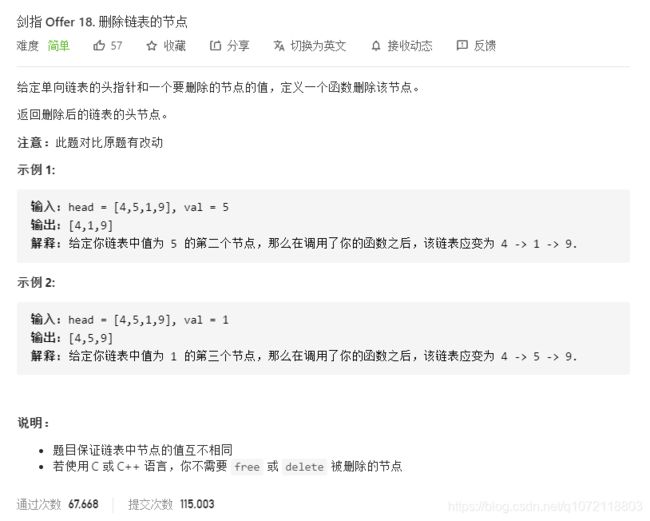
代码
class Solution {
public:
ListNode* deleteNode(ListNode* head, int val) {
ListNode* lfirst = (ListNode*)malloc(sizeof(ListNode));
lfirst->next = head;
ListNode* p = lfirst;
while(p&&p->next!=NULL)
{
if(p->next->val==val)
{
p->next = p->next->next;
}
p = p->next;
}
return lfirst->next;
}
};
剑指Offer 22.链表中倒数第k个节点
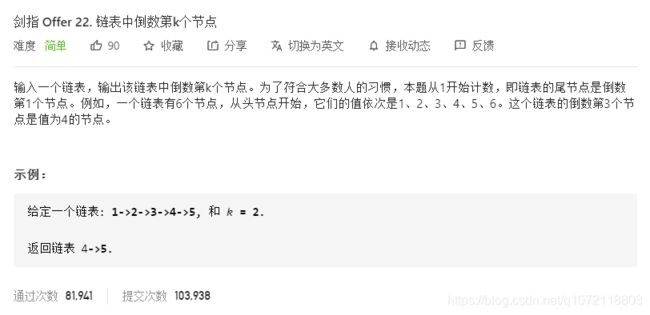
双指针
struct ListNode* getKthFromEnd(struct ListNode* head, int k){
struct ListNode* p = head;
struct ListNode* q = p;
while(q&&k--)
{
q = q->next;
}
if(k>0)
return NULL;
while(q!=NULL)
{
p = p->next;
q = q->next;
}
return p;
}
剑指Offer 24.反转链表
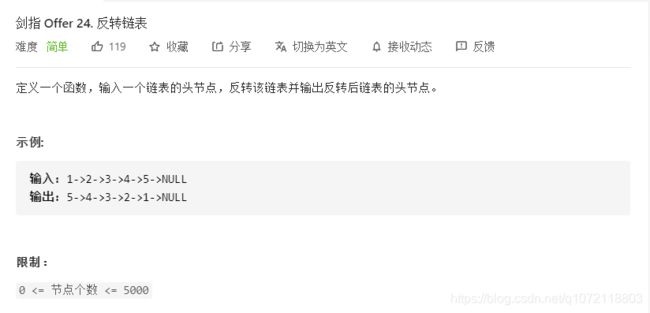
头插法
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* lst = new ListNode(0,NULL);
ListNode* p = head;
ListNode* q;
while(p!=NULL)
{
q = p->next;
p->next = lst->next;
lst->next = p;
p = q;
}
return lst->next;
}
};
剑指offer 35. 复杂链表的复制
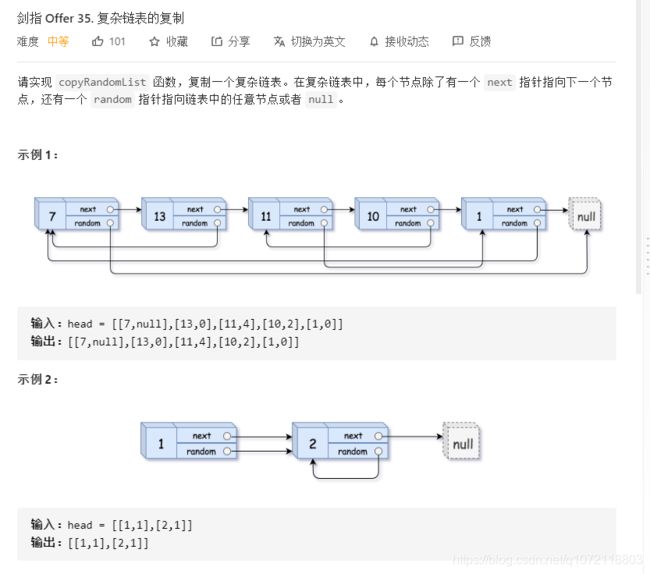
class Solution {
public:
ListNode* deleteNode(ListNode* head, int val) {
ListNode* lfirst = (ListNode*)malloc(sizeof(ListNode));
lfirst->next = head;
ListNode* p = lfirst;
while(p&&p->next!=NULL)
{
if(p->next->val==val)
{
p->next = p->next->next;
}
p = p->next;
}
return lfirst->next;
}
};
hashmap
class Solution {
public:
Node* copyRandomList(Node* head) {
if(!head)
return NULL;
unordered_map<Node*,Node*> match;
Node * newhead = new Node(head->val);
Node* copy = newhead;
Node* p = head;
while(p!=NULL)
{
match[p] = copy;
p = p->next;
if(p==NULL)
{
copy->next = NULL;
match[p] = NULL;
}
else copy->next = new Node(p->val);
copy = copy->next;
}
p = head;
copy = newhead;
while(p!=NULL)
{
copy->random = match[p->random];
p = p->next;
copy = copy->next;
}
return newhead;
}
};
原地复制
class Solution {
public:
Node* copyRandomList(Node* head) {
if(!head)
return NULL;
Node* p = head;
Node* copy = NULL;
while(p!=NULL)
{
copy = new Node(p->val);
copy->next = p->next;
p->next = copy;
p = p->next->next;
}
p = head;
while(p!=NULL)
{
if(p->random!=NULL)
{
p->next->random = p->random->next;
}
p = p->next->next;
}
Node* newhead = head->next;
p = head;
while(p&&p->next)
{
Node* temp = p->next;
p->next = temp->next;
p = temp;
}
return newhead;
}
};
剑指Offer 52.两个链表的第一个公共节点
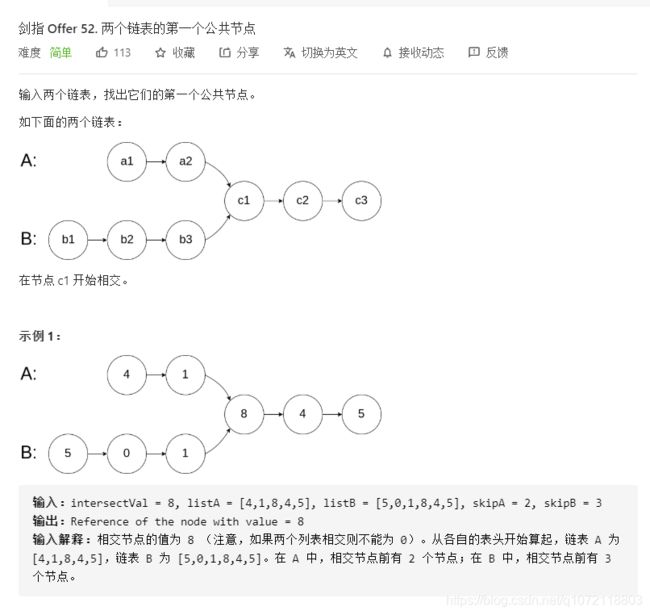
双指针
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
ListNode* node1 = headA, *node2 = headB;
while(node1!=node2)
{
node1 = node1!=NULL? node1->next : headB;
node2 = node2!=NULL? node2->next : headA;
}
return node1;
}
};