.NET 2.0中,WinForm应用程序的配置已经非常方便。默认情况下,我们可以利用Properties文件夹里默认的Settings.setting文件进行Application和User两个层级配置信息的设置。在Settings.setting里进行的修改保存后,均自动在后部cs文件里自动生成相关代码,同时在应用程序配置文件(app.config)里存储相关信息。比如,我们在TestWinForm项目里对Settings.setting进行如下修改:
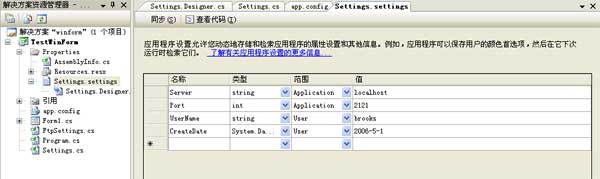
保存后,Settings.Designer.cs文件自动增加如下代码:
Settings.Designer.cs
Code
1
namespace
TestWinForm.Properties {
2
3
4
[
global
::System.Runtime.CompilerServices.CompilerGeneratedAttribute()]
5
[
global
::System.CodeDom.Compiler.GeneratedCodeAttribute(
"
Microsoft.VisualStudio.Editors.SettingsDesigner.SettingsSingleFileGenerator
"
,
"
8.0.0.0
"
)]
6
internal
sealed
partial
class
Settings :
global
::System.Configuration.ApplicationSettingsBase {
7
8
private
static
Settings defaultInstance
=
((Settings)(
global
::System.Configuration.ApplicationSettingsBase.Synchronized(
new
Settings())));
9
10
public
static
Settings Default {
11
get
{
12
return
defaultInstance;
13
}
14
}
15
16
[
global
::System.Configuration.ApplicationScopedSettingAttribute()]
17
[
global
::System.Diagnostics.DebuggerNonUserCodeAttribute()]
18
[
global
::System.Configuration.DefaultSettingValueAttribute(
"
localhost
"
)]
19
public
string
Server {
20
get
{
21
return
((
string
)(
this
[
"
Server
"
]));
22
}
23
}
24
25
[
global
::System.Configuration.ApplicationScopedSettingAttribute()]
26
[
global
::System.Diagnostics.DebuggerNonUserCodeAttribute()]
27
[
global
::System.Configuration.DefaultSettingValueAttribute(
"
2121
"
)]
28
public
int
Port {
29
get
{
30
return
((
int
)(
this
[
"
Port
"
]));
31
}
32
}
33
34
[
global
::System.Configuration.UserScopedSettingAttribute()]
35
[
global
::System.Diagnostics.DebuggerNonUserCodeAttribute()]
36
[
global
::System.Configuration.DefaultSettingValueAttribute(
"
brooks
"
)]
37
public
string
UserName {
38
get
{
39
return
((
string
)(
this
[
"
UserName
"
]));
40
}
41
set
{
42
this
[
"
UserName
"
]
=
value;
43
}
44
}
45
46
[
global
::System.Configuration.UserScopedSettingAttribute()]
47
[
global
::System.Diagnostics.DebuggerNonUserCodeAttribute()]
48
[
global
::System.Configuration.DefaultSettingValueAttribute(
"
2006-05-01
"
)]
49
public
global
::System.DateTime CreateDate {
50
get
{
51
return
((
global
::System.DateTime)(
this
[
"
CreateDate
"
]));
52
}
53
set
{
54
this
[
"
CreateDate
"
]
=
value;
55
}
56
}
57
}
58
}
59
同时,app.config也发生了变化:
Code
1
<?
xml version="1.0" encoding="utf-8"
?>
2
<
configuration
>
3
<
configSections
>
4
<
sectionGroup
name
="applicationSettings"
type
="System.Configuration.ApplicationSettingsGroup, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"
>
5
<
section
name
="TestWinForm.Properties.Settings"
type
="System.Configuration.ClientSettingsSection, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"
requirePermission
="false"
/>
6
</
sectionGroup
>
7
<
sectionGroup
name
="userSettings"
type
="System.Configuration.UserSettingsGroup, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"
>
8
<
section
name
="TestWinForm.Properties.Settings"
type
="System.Configuration.ClientSettingsSection, System, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"
allowExeDefinition
="MachineToLocalUser"
requirePermission
="false"
/>
9
</
sectionGroup
>
10
</
configSections
>
11
<
applicationSettings
>
12
<
TestWinForm.Properties.Settings
>
13
<
setting
name
="Server"
serializeAs
="String"
>
14
<
value
>
localhost
</
value
>
15
</
setting
>
16
<
setting
name
="Port"
serializeAs
="String"
>
17
<
value
>
2121
</
value
>
18
</
setting
>
19
</
TestWinForm.Properties.Settings
>
20
</
applicationSettings
>
21
<
userSettings
>
22
<
TestWinForm.Properties.Settings
>
23
<
setting
name
="UserName"
serializeAs
="String"
>
24
<
value
>
brooks
</
value
>
25
</
setting
>
26
<
setting
name
="CreateDate"
serializeAs
="String"
>
27
<
value
>
2006-05-01
</
value
>
28
</
setting
>
29
</
TestWinForm.Properties.Settings
>
30
</
userSettings
>
31
</
configuration
>
32
要在具体代码中使用配置信息就非常非常的方便了。
Code
1
private
void
button1_Click(
object
sender, EventArgs e)
2
{
3
string
msg
=
TestWinForm.Properties.Settings.Default.Server
+
"
:
"
+
TestWinForm.Properties.Settings.Default.Port.ToString();
4
MessageBox.Show(msg);
5
}
OK,鬼扯了这么多,用意在于让我们再熟悉下.NET2.0的配置。现在,我们不满足他所提供的默认配置,于是我们创建了自己的一个Demo用的配置类 FtpSetting。在WinForm应用程序里,一切配置类都得继承自 ApplicationSettingsBase 类。
Code
1
sealed
class
FtpSettings : ApplicationSettingsBase
2
{
3
[UserScopedSetting]
4
[DefaultSettingValue(
"
127.0.0.1
"
)]
5
public
string
Server
6
{
7
get
{
return
(
string
)
this
[
"
Server
"
]; }
8
set
{
this
[
"
Server
"
]
=
value; }
9
}
10
11
[UserScopedSetting]
12
[DefaultSettingValue(
"
21
"
)]
13
public
int
Port
14
{
15
get
{
return
(
int
)
this
[
"
Port
"
]; }
16
set
{
this
[
"
Port
"
]
=
value; }
17
}
18
}
如果要使用上述配置类,可以用:
Code
1
private
void
button2_Click(
object
sender, EventArgs e)
2
{
3
FtpSettings ftp
=
new
FtpSettings();
4
5
string
msg
=
ftp.server
/
+
"
:
"
+
ftp.port.tostring
/
();
6
MessageBox.Show(msg);
7
}
好,似乎还在鬼扯。这个Tip已经进入尾声了,主题正式登场。:) 我们在使用上述 FtpSetting 配置时,当然要先进行赋值保存,然后再使用,后面再修改,再保存,再使用。
Code
1
private
void
button2_Click(
object
sender, EventArgs e)
2
{
3
FtpSettings ftp
=
new
FtpSettings();
4
ftp.server
=
"
ftp://ftp.test.com/
"
;
5
ftp.port
/
=
8021
;
6
7
ftp.save
/
();
8
ftp.reload
/
();
9
10
string
msg
=
ftp.server
/
+
"
:
"
+
ftp.port.tostring
/
();
11
MessageBox.Show(msg);
12
}
13
嗯。已经Save了,你可能会在应用程序文件夹里找不到它到底保存到哪里去了。由于我们是用UserScope的,所以其实该配置信息是保存到了你的Windows的个人文件夹里去了。比如我的就是 C:\Documents and Settings\brooks\Local Settings\Application Data\TestWinForm目录了。哈~当作Tip吧,免得大伙一时找不到Save后的配置文件。