这篇文章将介绍如何通过弹出一个ChildWindow来让用户输入登录信息。
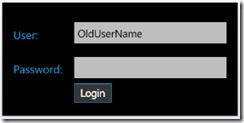
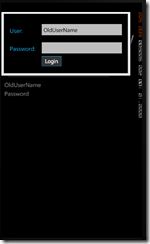
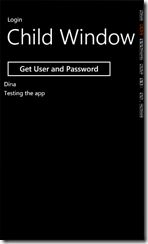
下面的几篇文章对我提供了帮助
1. Jesse Liberty’s video “Creating a custom popup in Window Phone 7”
2. Shawn Wildermuth’s “Using ChildWindow in Windows Phone 7 Project”
3. Raj Kumar’s “How to Implement ChildWindow in Windows Phone 7”
4.Coding4Fun Source code at Codeplex
LoginChildWindow控件继承自 System.Windows.Controls.ChildWindow,所以你必须添加dll引用
LoginChildWindow.xaml 代码如下:
<
tk:ChildWindow
x:Class
="LoginChildWindow.LoginChildWindow"
xmlns
="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x
="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d
="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc
="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:tk
="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls"
mc:Ignorable
="d"
VerticalAlignment
="Top"
HorizontalAlignment
="Left"
BorderBrush
="Black"
BorderThickness
="2"
FontFamily
="{StaticResource PhoneFontFamilyNormal}"
FontSize
="{StaticResource PhoneFontSizeNormal}"
Foreground
="{StaticResource PhoneAccentBrush}"
d:DesignHeight
="256"
d:DesignWidth
="480"
HasCloseButton
="false"
Title
="Login"
>
<
Grid
x:Name
="LayoutRoot"
Height
="202"
Background
="{StaticResource PhoneBackgroundBrush}"
>
<
TextBlock
HorizontalAlignment
="Left"
TextWrapping
="Wrap"
Text
="User: "
VerticalAlignment
="Top"
Margin
="20,43,0,0"
/>
<
TextBlock
HorizontalAlignment
="Left"
TextWrapping
="Wrap"
Text
="Password: "
VerticalAlignment
="Top"
Margin
="20,104,0,0"
/>
<
TextBox
x:Name
="txtUserId"
VerticalAlignment
="Top"
Margin
="117,23,8,0"
Height
="62"
FontSize
="18.667"
TabIndex
="1"
KeyUp
="txtUserId_KeyUp"
/>
<
PasswordBox
x:Name
="txtPassword"
VerticalAlignment
="Top"
Margin
="117,85,8,0"
Height
="62"
FontSize
="18.667"
TabIndex
="2"
KeyUp
="txtPassword_KeyUp"
MouseLeftButtonUp
="txtPassword_MouseLeftButtonUp"
/>
<
Button
x:Name
="btnLogin"
Content
="Login"
Margin
="117,132,214,0"
d:LayoutOverrides
="Width"
Click
="btnLogin_Click"
FontSize
="18.667"
BorderBrush
="{StaticResource PhoneAccentBrush}"
BorderThickness
="1"
Foreground
="{StaticResource PhoneForegroundBrush}"
Background
="{StaticResource PhoneInactiveBrush}"
Height
="58"
VerticalAlignment
="Top"
FontFamily
="Tahoma"
TabIndex
="3"
/>
<!--
<Button x:Name="btnCancel" Content="Cancel" HorizontalAlignment="Left" Margin="232,132,0,0" VerticalAlignment="Top" FontSize="18.667" BorderBrush="{StaticResource PhoneAccentBrush}" BorderThickness="1" Foreground="{StaticResource PhoneForegroundBrush}" Background="{StaticResource PhoneInactiveBrush}" Height="58" FontFamily="Tahoma" Click="btnCancel_Click" />
-->
</
Grid
>
</
tk:ChildWindow
>
VerticalAlignment和HorizontalAlignment设置使窗口在屏幕的上方弹出,如果不这样设置的话,屏幕上的键盘会覆盖掉子窗口。
一般来说在登录按钮旁边还要有一个取消按钮,但是我的程序中要求用户必须登录,所以就将取消按钮给注释掉了。子窗口的右上方本来有一个小x图,
通过设置 HasCloseButton="false"去掉了,出于同样的原因。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace LoginChildWindow
{
public
partial
class LoginChildWindow : ChildWindow
{
public
string Login {
get;
set; }
public
string Password {
get;
set; }
public LoginChildWindow()
{
InitializeComponent();
}
protected
override
void OnOpened()
{
base.OnOpened();
this.txtUserId.Text =
this.Login;
}
private
void btnLogin_Click(
object sender, RoutedEventArgs e)
{
//
test values
if ((txtUserId.Text ==
null) || (txtPassword.Password ==
null))
{
MessageBox.Show(
"
WP7: Username & Password must be filled in before logging on.
");
//
this.DialogResult = false;
}
if ((txtUserId.Text.Trim() ==
string.Empty) || (txtPassword.Password.Trim() ==
string.Empty))
{
MessageBox.Show(
"
WP7: Username & Password must be filled in before logging on.
");
//
this.DialogResult = false;
}
else
if (txtUserId.Text.Trim().Length <
2)
{
MessageBox.Show(
"
WP7: Invalid username or password. Be sure to use the WAZUp website login, not the Windows Azure login.
");
//
this.DialogResult = false;
}
else
{
//
values are good so close this childwindow
this.Login =
this.txtUserId.Text.Trim();
this.Password =
this.txtPassword.Password.Trim();
this.DialogResult =
true;
}
}
//
private void btnCancel_Click(object sender, RoutedEventArgs e)
//
{
//
this.DialogResult = false;
//
}
private
void txtUserId_KeyUp(
object sender, KeyEventArgs e)
{
if (e.Key == Key.Enter)
{
if (txtPassword.Password.Length ==
0)
{
txtPassword.Focus();
}
else
{
btnLogin_Click(sender, e);
}
}
}
private
void txtPassword_KeyUp(
object sender, KeyEventArgs e)
{
if (e.Key == Key.Enter)
{
btnLogin_Click(sender, e);
}
}
private
void txtPassword_MouseLeftButtonUp(
object sender, MouseButtonEventArgs e)
{
btnLogin_Click(sender, e);
}
}
}
LoginChildWindow.xaml.cs 代码如下:
using System;
using System.Net;
using System.Windows;
using System.Windows.Input;
using Microsoft.Phone.Controls;
namespace LoginChildWindow
{
public
partial
class MainPage : PhoneApplicationPage
{
public
string Username {
get;
set; }
public
string Password {
get;
set; }
//
Constructor
public MainPage()
{
Username =
"
OldUserName
";
InitializeComponent();
this.txtBlockUsername.Text = Username;
}
private
void button1_Click(
object sender, RoutedEventArgs e)
{
LoginChildWindow loginWindow =
new LoginChildWindow();
loginWindow.Login = Username;
loginWindow.Closed +=
new EventHandler(OnLoginChildWindowShow);
loginWindow.Show();
}
private
void OnLoginChildWindowShow(
object sender, EventArgs e)
{
LoginChildWindow loginChildWindow = sender
as LoginChildWindow;
if (loginChildWindow.DialogResult ==
true)
{
this.txtBlockUsername.Text = loginChildWindow.Login;
this.txtBlockPassword.Text = loginChildWindow.Password;
//
;
}
}
}
}
这是一个使用pop up简单的例子,如果你想找一个更好的解决方案,可以在CodePlex获取Coding4Fun源码,这个ToolKit有一个更强大的idea!~

原文地址: http://www.31a2ba2a-b718-11dc-8314-0800200c9a66.com/2011/06/how-to-create-childwindow-login-popup.html