(1)必要变量(字段)定义
private object Nothing = Missing.Value;
private object IsReadOnly = false;
private MSWord._Application wordApp;
private MSWord._Document wordDoc;
private int tableCount;
注:前面4个是操作Word时,必须定义的字段;最后一个适应本程序
(2)读取txt数据
private List<string> TextData = new List<string>();
private void toolStripMenuItem1_Click(object sender, EventArgs e)
{
openFileDialog1.InitialDirectory = "E:\\";
openFileDialog1.Filter = "GSI(*.gsi)|*.gsi";
if(openFileDialog1.ShowDialog()==DialogResult.OK)
{
TextData = File.ReadAllLines(openFileDialog1.FileName).Where(s => (s[0] != '4') && (s != "")).ToList();
}
tableCount = (int)(TextData.Count / 11 * 2 / 7);
MessageBox.Show("共读取" + TextData.Count / 11 + "测段的数据!");
}
(3)打开原本的Word文档,并复制其中的表格
private void toolStripMenuItem2_Click(object sender, EventArgs e)
{
openFileDialog2.InitialDirectory = "e:\\";
openFileDialog2.Filter = "文档(*.docx)|*.docx|(*.doc)|*.doc";
if(openFileDialog2.ShowDialog()==DialogResult.OK)
{
try
{
foreach (Process item in Process.GetProcessesByName("WINWORD"))
{ item.Kill(); }
object filePath=openFileDialog2.FileName;
wordApp = new MSWord.Application();
wordDoc = wordApp.Documents.Open(ref filePath, ref Nothing, ref IsReadOnly, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing);
wordDoc.Tables[1].Select();
wordApp.Selection.Copy();
object myType = MSWord.WdBreakType.wdSectionBreakContinuous;
object myUnit = MSWord.WdUnits.wdStory;
object pBreak = (int)MSWord.WdBreakType.wdPageBreak;
for (int i = 0; i < tableCount-1;i++ )
{
wordApp.Selection.EndKey(ref myUnit, ref Nothing);
wordApp.Selection.InsertBreak(ref pBreak);
wordApp.Selection.PasteAndFormat(MSWord.WdRecoveryType.wdPasteDefault);
}
MessageBox.Show("手簿加载完成!");
}
catch(Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
(4)将txt数据写入变化后的Word文档并保存
//原始数据:TextData中
private void toolStripMenuItem3_Click(object sender, EventArgs e)
{
try
{
#region 文档头
object bm1 = "云量"
wordDoc.Bookmarks.get_Item(ref bm1).Range.Text = "多云"
object bm2 = "风向风速"
wordDoc.Bookmarks.get_Item(ref bm2).Range.Text = "无风"
object bm3 = "天气"
wordDoc.Bookmarks.get_Item(ref bm3).Range.Text = "晴朗"
#endregion
List Data = TextData.Where(s => s.ToCharArray().Count() > 35).ToList()
int tabturn = 0
foreach(MSWord.Table table in wordDoc.Tables)
{
for (int i = 1
{
List tempData = new List()
for (int j = 35 * tabturn + (i - 1) * 5
{
tempData.Add(Data[j])
}
#region 文档表格赋值
table.Cell(4 * i + 1, 1).Range.Text = i + "测站"
table.Cell(4 * i + 1, 5).Range.Text = (double.Parse(tempData[0].Trim().Split(' ')[2].Substring(6, 9)) / 100.0).ToString()
table.Cell(4 * i + 2, 5).Range.Text = (double.Parse(tempData[1].Trim().Split(' ')[2].Substring(6, 9)) / 100.0).ToString()
table.Cell(4 * i + 2, 6).Range.Text = (double.Parse(tempData[2].Trim().Split(' ')[2].Substring(6, 9)) / 100.0).ToString()
table.Cell(4 * i + 1, 6).Range.Text = (double.Parse(tempData[3].Trim().Split(' ')[2].Substring(6, 9)) / 100.0).ToString()
table.Cell(4 * i + 3, 2).Range.Text = ((double.Parse(tempData[0].Trim().Split(' ')[1].Substring(6, 9)) / 1e2 + double.Parse(tempData[3].Trim().Split(' ')[1].Substring(6, 9)) / 1e2) / 2).ToString()
table.Cell(4 * i + 3, 3).Range.Text = ((double.Parse(tempData[1].Trim().Split(' ')[1].Substring(6, 9)) / 1e2 + double.Parse(tempData[2].Trim().Split(' ')[1].Substring(6, 9)) / 1e2) / 2).ToString()
table.Cell(4 * i + 4, 2).Range.Text = ((int)(((double.Parse(tempData[0].Trim().Split(' ')[1].Substring(6, 9)) / 1e5 + double.Parse(tempData[3].Trim().Split(' ')[1].Substring(6, 9)) / 1e5) / 2 - (double.Parse(tempData[1].Trim().Split(' ')[1].Substring(6, 9)) / 1e5 + double.Parse(tempData[2].Trim().Split(' ')[1].Substring(6, 9)) / 1e5) / 2) * 10000 + 0.5) / 10000.0).ToString()
table.Cell(4 * i + 4, 3).Range.Text = (double.Parse(tempData[4].Trim().Split(' ')[3].Substring(6, 9)) / 1e5).ToString()
table.Cell(4 * i + 1, 7).Range.Text = ((int)((double.Parse(tempData[0].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[3].Trim().Split(' ')[2].Substring(6, 9)) / 100.0) * 1000 + 0.5) / 1000.0).ToString()
table.Cell(4 * i + 2, 7).Range.Text = ((int)((double.Parse(tempData[1].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[2].Trim().Split(' ')[2].Substring(6, 9)) / 100.0) * 1000 + 0.5) / 1000.0).ToString()
table.Cell(4 * i + 3, 5).Range.Text = ((int)((double.Parse(tempData[0].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[1].Trim().Split(' ')[2].Substring(6, 9)) / 100.0) * 10000 + 0.5) / 10000.0).ToString()
table.Cell(4 * i + 3, 6).Range.Text = ((int)((double.Parse(tempData[3].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[2].Trim().Split(' ')[2].Substring(6, 9)) / 100.0) * 10000 + 0.5) / 10000.0).ToString()
table.Cell(4 * i + 3, 7).Range.Text = ((int)((double.Parse(tempData[0].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[3].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - (double.Parse(tempData[1].Trim().Split(' ')[2].Substring(6, 9)) / 100.0 - double.Parse(tempData[2].Trim().Split(' ')[2].Substring(6, 9)) / 100.0)) * 1000 + 0.5) / 1000.0).ToString()
table.Cell(4 * i + 4, 6).Range.Text = (double.Parse(tempData[4].Trim().Split(' ')[5].Substring(6, 9)) / 1e5).ToString()
#endregion
}
tabturn += 1
}
//文档另存为并退出
saveFileDialog1.Filter = "文档(*.docx)|*.docx|(*.doc)|*.doc"
if(saveFileDialog1.ShowDialog()==DialogResult.OK)
{
object filePath = saveFileDialog1.FileName
object format = MSWord.WdSaveFormat.wdFormatDocumentDefault
//object format = MSWord.WdSaveFormat.wdFormatDocument
wordDoc.SaveAs(ref filePath, ref format, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing, ref Nothing)
((MSWord._Document)wordDoc).Close(ref Nothing, ref Nothing, ref Nothing)
((MSWord._Application)wordApp).Quit(ref Nothing, ref Nothing, ref Nothing)
}
}
catch(Exception ex)
{
MessageBox.Show(ex.ToString())
}
finally
{
if (wordApp != null)
{
Marshal.FinalReleaseComObject(wordApp)
wordApp = null
}
if (wordDoc != null)
{
Marshal.FinalReleaseComObject(wordDoc)
wordDoc = null
}
}
}
注:当向Word表格中输入数据时,循环处代码较多,但本质上并不复杂。
(5)部分txt文本数据(莱卡数字水准仪数据)
410001+?......2
110002+000000A1 83..28+00000000
110003+000000A1 32...8+01471340 331.08+00096547 390...+00000002 391.08+00000000 71....+00000001
110004+00000001 32...8+01457115 332.08+00144543 390...+00000002 391.08+00000005
110005+00000001 32...8+01456595 336.08+00144553 390...+00000002 391.08+00000002
110006+000000A1 32...8+01470969 335.08+00096549 390...+00000002 391.08+00000001
110007+00000001 571.08+00000008 572.08+00000008 573..8+00014300 574..8+02928010 83..08-00048000
110008+00000001 32...8+01616093 331.08+00143847 390...+00000002 391.08+00000001
110009+00000002 32...8+01626730 332.08+00136480 390...+00000002 391.08+00000000
110010+00000002 32...8+01626589 336.08+00136477 390...+00000002 391.08+00000001
110011+00000001 32...8+01616202 335.08+00143831 390...+00000002 391.08+00000024
110012+00000002 571.08+00000013 572.08+00000021 573..8+00003788 574..8+06170816 83..08-00040640
410013+?......2
110014+000000A1 83..08+00000000
110015+000000A1 32...8+01625880 331.08+00136485 390...+00000002 391.08+00000003
110016+00000001 32...8+01617791 332.08+00143837 390...+00000002 391.08+00000004
110017+00000001 32...8+01617711 336.08+00143834 390...+00000002 391.08+00000003
110018+000000A1 32...8+01624988 335.08+00136499 390...+00000002 391.08+00000002
110019+00000001 571.08-00000017 572.08-00000017 573..8+00007683 574..8+03243185 83..08-00007344
110020+00000001 32...8+01460178 331.08+00144567 390...+00000002 391.08+00000001
110021+00000002 32...8+01469076 332.08+00096601 390...+00000002 391.08+00000000
110022+00000002 32...8+01469139 336.08+00096599 390...+00000002 391.08+00000002
110023+00000001 32...8+01460232 335.08+00144565 390...+00000002 391.08+00000001
110024+00000002 571.08-00000001 572.08-00000018 573..8-00001220 574..8+06172497 83..08+00040622
(6)部分结果截图
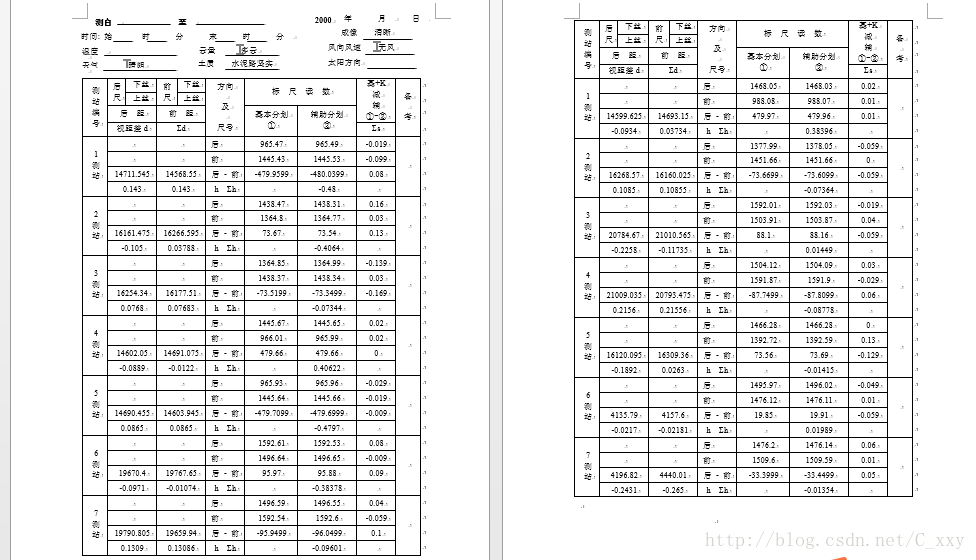