hbase shell 通用命令 status:提供hbase的状况 version:提供正在使用Hbase版本 table_help :表引用命令提供帮助 whoami 提供用户的信息 数据定义语言: 这些是关于HBase在表中操作的命令。 create: 创建一个表。 create 'test', 'cf1 name','cf1 age' list: 列出HBase的所有表。 list disable: 禁用表。 disable ‘trst’ is_disabled: 验证表是否被禁用。 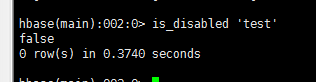 enable: 启用一个表 enable ‘test’ is_enabled: 验证表是否已启用。 is_enable ‘test’ describe: 提供了一个表的描述。 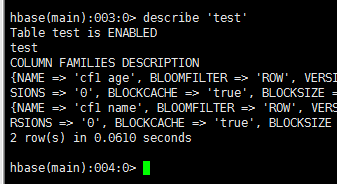 alter: 改变一个表。 修改: 语法:alter 'tablename' ,NAME=>'列族',VERSIONS=>5 alter 'emp', NAME=>'personal age',VERSIONS=>5 使用alter,可以设置和删除表范围,运算符,如MAX_FILESIZE,READONLY,MEMSTORE_FLUSHSIZE,DEFERRED_LOG_FLUSH等。 alter 'emp',READONLY 将emp表设为只读 删除表范围运算符 alter '表名',METHOD=>'table_att_set',NAME=>"MAX_FILESIZE" 删除列族 alter ‘tablename’,'delete'=>'column family' 例:alter 'emp','delete'=>'professional' 删除表前必须先禁用表 disable 'table name' drop 'table name' 批量删除表 disable_all 't.*' exists: 验证表是否存在。 Exists ‘test’ drop: 从HBase中删除表。 Drop ‘test’ drop_all: 丢弃在命令中给出匹配“regex”的表。 #添加数据 ##查看表数据 Scan ‘test’ 数据操作语言 put: 把指定列在指定的行中单元格的值在一个特定的表。 put 'test','1','cf1 name','zhangsan' get: 取行或单元格的内容。 Get ‘test’,’1’ delete: 删除表中的单元格值。 delete 'emp','1','personal data:city','时间戳' deleteall: 删除给定行的所有单元格。 deleteall 'emp','1' scan: 扫描并返回表数据。 count: 计数并返回表中的行的数目。 Count 'emp' truncate 此命令将禁止删除并重新创建一个表 truncate 'emp' grant 名利授予特定的权限,如读写,执行和管理表给一个特定的用户 R W X C(创建) A(管理权限) grant 'Turorialspoint','RWXCA' revoke 命令用于撤销用户访问表的权限 revoke 'Turorialspoint' user_permission 列出特定表的所有用户权限 user_permission 'emp' ###测试HBASE ##添加maven依赖
org.apache.flink
flink-hbase_2.12
${flink.version}
package xpu.cheng.FlinkCase.Hbase;
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.hbase.*; import org.apache.hadoop.hbase.client.*; import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException; import java.util.ArrayList; import java.util.List;
public class HbaseTest { /** * 连接hbase * 声明静态配置 */ static Configuration conf=null; static Connection conn=null; static Admin admin=null; static { conf = HBaseConfiguration.create(); conf.set("hbase.zookeeper.quorum", "master,slave2,slave3"); conf.set("hbase.zookeeper.property,clinet", "2181"); try{ conn=ConnectionFactory.createConnection(conf); admin=conn.getAdmin(); } catch (IOException e) { e.printStackTrace(); } }
/** * 创建只有一个列族的表 * 创建HBase表,是通过Admin来执行的, * 表和列簇则是分别通过TableDescriptorBuilder和ColumnFamilyDescriptorBuilder来构建 * @throws IOException */ public static void createTable() throws IOException { /**判断emp表是否存在*/ if(!admin.tableExists(TableName.valueOf("emp"))){ TableName tableName=TableName.valueOf("emp"); //表描述构造器 HTableDescriptor tdb=new HTableDescriptor(tableName); //获得列族描述 HColumnDescriptor hcd=new HColumnDescriptor("personal"); HColumnDescriptor hcd1=new HColumnDescriptor("professional"); //加入列族 tdb.addFamily(hcd); tdb.addFamily(hcd1); admin.createTable(tdb); System.out.println("Table created"); } }
/** * 查看table表 * @throws IOException */ public static void list() throws IOException { HTableDescriptor[] tableDescriptors=admin.listTables(); for(int i=0;i System.out.println(tableDescriptors[i].getNameAsString()); } } public static void scantable(TableName tb) throws IOException { Table table=conn.getTable(tb); Scan s = new Scan(); ResultScanner rs = table.getScanner(s); for (Result r : rs) { for (KeyValue kv : r.raw()) { System.out.print("row : " + new String(kv.getRow()) + " "); System.out.print("family : " + new String(kv.getFamily()) + " "); System.out.print("qualifier : " + new String(kv.getQualifier()) + " "); System.out.print("Timestamp : " + kv.getTimestamp() + " "); System.out.println("value : " + new String(kv.getValue()) + " "); } }
} public static void getResult(TableName tableName,String rowkey) throws IOException {
Table table=conn.getTable(tableName); //获取一行 Get get=new Get(Bytes.toBytes(rowkey)); Result set=table.get(get); Cell[] cells=set.rawCells(); for(Cell cell:cells){ System.out.println(Bytes.toString(cell.getQualifierArray(),cell.getValueOffset(),cell.getQualifierLength()) +"::"+ Bytes.toString(cell.getValueArray(),cell.getValueOffset(),cell.getValueLength())); } table.close();
} /** * 根据rowKey删除一行数据、或者删除某一行的某个列簇,或者某一行某个列簇某列 * @param tableName * @param rowKey * @throws Exception */ public static void deleteData(TableName tableName, String rowKey, String columnFamily, String columnName) throws Exception{ Table table = conn.getTable(tableName); Delete delete = new Delete(Bytes.toBytes(rowKey)); //①根据rowKey删除一行数据 table.delete(delete);
//②删除某一行的某一个列簇内容 delete.addFamily(Bytes.toBytes(columnFamily));
//③删除某一行某个列簇某列的值 delete.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(columnName)); table.close(); } /** * 根据RowKey , 列簇, 列名修改值 * @param tableName * @param rowKey * @param columnFamily * @param columnName * @param columnValue * @throws Exception */ public static void updateData(TableName tableName, String rowKey, String columnFamily, String columnName, String columnValue) throws Exception{ Table table = conn.getTable(tableName); Put put1 = new Put(Bytes.toBytes(rowKey)); put1.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(columnName), Bytes.toBytes(columnValue)); table.put(put1); table.close(); } /** * 添加数据(多个rowKey,多个列族) * @throws Exception */ public static void insertMany() throws Exception{ Table table = conn.getTable(TableName.valueOf("emp")); List puts = new ArrayList(); Put put1 = new Put(Bytes.toBytes("rowKey1")); put1.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("name"), Bytes.toBytes("wd")); put1.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("age"), Bytes.toBytes("23")); put1.addColumn(Bytes.toBytes("professional"), Bytes.toBytes("name"), Bytes.toBytes("jingli"));
Put put2 = new Put(Bytes.toBytes("rowKey2")); put2.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("name"), Bytes.toBytes("wh")); put2.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("age"), Bytes.toBytes("25")); put2.addColumn(Bytes.toBytes("professional"), Bytes.toBytes("name"), Bytes.toBytes("jingli"));
Put put3 = new Put(Bytes.toBytes("rowKey3")); put3.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("name"), Bytes.toBytes("wd")); put3.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("age"), Bytes.toBytes("33")); put3.addColumn(Bytes.toBytes("professional"), Bytes.toBytes("name"), Bytes.toBytes("jingli"));
Put put4 = new Put(Bytes.toBytes("rowKey4")); put4.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("name"), Bytes.toBytes("wd")); put4.addColumn(Bytes.toBytes("personal"), Bytes.toBytes("age"), Bytes.toBytes("88")); put4.addColumn(Bytes.toBytes("professional"), Bytes.toBytes("name"), Bytes.toBytes("jingli")); puts.add(put1); puts.add(put2); puts.add(put3); puts.add(put4); table.put(puts); table.close(); }
public static void main(String[] args) throws Exception { TableName tb=TableName.valueOf("emp"); String row="rowKey4";
/* getResult(tb,row); list(); createTable(); */ insertMany(); scantable(tb); } } ###flink读取hbase中的数据 ##创建数据表 create 'test', 'cf1 name','cf1 age' ##添加数据 put 'test','1','cf1 name','zhangsan' 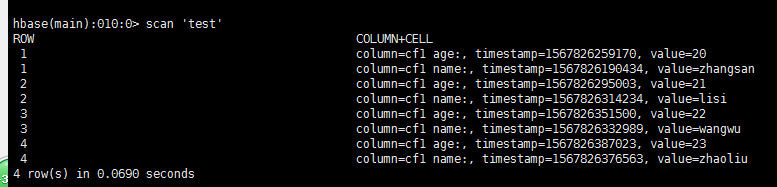 /**
* 以hbase为数据源,从HBASE中获取数据,然后一流的形式发送
*继承RichSourceFunction重写父类方法 */
public class HBaseReader extends RichSourceFunction {
private Connection conn=null;
private Table table=null;
private Scan scan=null;
/**
* 在open方法中使用hbase的客户端连接
* @param
* @throws Exception
*/
@Override
public void open(Configuration parameters) throws Exception {
super.open(parameters);
org.apache.hadoop.conf.Configuration config=HBaseConfiguration.create();
config.set(HConstants.ZOOKEEPER_QUORUM,"master");
config.set(HConstants.ZOOKEEPER_CLIENT_PORT,"2181");
config.setInt(HConstants.HBASE_CLIENT_OPERATION_TIMEOUT,3000);
config.setInt(HConstants.HBASE_CLIENT_SCANNER_TIMEOUT_PERIOD,3000);
TableName tableName= TableName.valueOf("test");
conn=ConnectionFactory.createConnection(config);
table=conn.getTable(tableName);
scan=new Scan();
}
/**
* run方法来自java接口文件sourceFunction
* @param sourceContext
* @throws Exception
*/
@Override
public void run(SourceContext sourceContext) throws Exception {
Iterator iterator = table.getScanner(scan).iterator();
while(iterator.hasNext()){
Result next = iterator.next();
String string = Bytes.toString(next.getRow());
StringBuffer sb=new StringBuffer();
for(Cell cell:next.listCells()){
String s = Bytes.toString(cell.getValueArray(), cell.getValueOffset(), cell.getValueLength());
sb.append("age "+s).append("_name ");
}
String result = sb.replace(sb.length() - 1, sb.length(), "").toString();
Tuple2 tuple2=new Tuple2<>(string,result);
sourceContext.collect(tuple2.toString());
}
}
@Override
public void cancel() {
}
@Override
public void close() throws Exception {
super.close();
if(table!=null){
table.close();
}
if(conn!=null){
conn.close();
}
}
} ###flink读取hbase public class FlinkReadHbase {
public static void main(String[] args) throws Exception {
StreamExecutionEnvironment env=StreamExecutionEnvironment.getExecutionEnvironment();
env.enableCheckpointing(5000);
env.setStreamTimeCharacteristic(TimeCharacteristic.EventTime);
env.getCheckpointConfig().setCheckpointingMode(CheckpointingMode.EXACTLY_ONCE);
DataStream dataStream=env.addSource(new HBaseReader());
dataStream.print();
env.execute("flink read hbase");
} 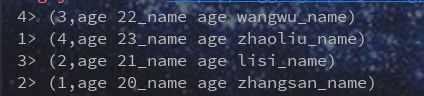 |