from tkinter import *
import numpy as np
import time
import math
tk = Tk()
tk.title('smile')
tk.wm_attributes("-topmost", 1)
Width = 600
Height = 600
canvas = Canvas(tk, width=Width, height=Height, bg='white', highlightthickness=0)
canvas.pack()
canvas.create_line(Width / 2, 0, Width / 2, Height)
canvas.create_line(0, Height / 2, Height, Height / 2)
tk.update()
class Agent:
def __init__(self, x, y, u, v, color):
self.canvas = canvas
self.color = color
self.id = self.canvas.create_oval(x, y, u, v, fill=self.color)
self.x = np.random.uniform(-1, 1)
self.y = np.random.uniform(-1, 1)
self.canvas_height = self.canvas.winfo_height()
self.canvas_width = self.canvas.winfo_width()
self.tag = True
def position(self):
""""返回目标当前的位置"""
pos = self.canvas.coords(self.id)
return pos
def draw(self):
"""绘制目标对象的运动状态"""
if self.tag:
pos = self.canvas.coords(self.id)
if pos[0] <= 0:
self.x = 10
if pos[1] <= 0:
self.y = 10
if pos[2] > self.canvas_width:
self.x = -10
if pos[3] > self.canvas_height:
self.y = -10
else:
self.x = 0
self.y = 0
self.canvas.move(self.id, self.x, self.y)
def position2point(self):
"""把目标的两个坐标转换为中心的一个坐标"""
pos = self.position()
point = [0.0, 0.0]
point[0] = (pos[0] + pos[2]) / 2
point[1] = (pos[1] + pos[3]) / 2
return np.array(point)
colors = ['black', 'green', 'blue', 'yellow', 'orange', 'pink', 'purple']
cent = np.array([300, 300])
eyel = np.array([250, 250])
eyer = np.array([350, 250])
mouth = np.array([300, 330])
theta = math.pi / 50
angle = np.array([[math.cos(theta), math.sin(theta)], [-math.sin(theta), math.cos(theta)]])
point = np.array([300, 150])
step = 0
while True:
xx = point - cent
yy = angle.dot(xx)
yy = yy + cent
step = step + 1
Agent(yy[0] - 5, yy[1] - 5, yy[0] + 5, yy[1] + 5, colors[step % 7])
tk.update()
time.sleep(0.01)
point = yy
if step == 100:
break
point = eyel - 10
step = 0
theta = -theta * 2
angle = np.array([[math.cos(theta), math.sin(theta)], [-math.sin(theta), math.cos(theta)]])
while True:
xx = point - eyel
yy = angle.dot(xx)
yy = yy + eyel
step = step + 1
Agent(yy[0] - 5, yy[1] - 5, yy[0] + 5, yy[1] + 5, colors[step % 7])
tk.update()
time.sleep(0.01)
point = yy
if step == 50:
break
point = eyer - 10
step = 0
while True:
xx = point - eyer
yy = angle.dot(xx)
yy = yy + eyer
step = step + 1
Agent(yy[0] - 5, yy[1] - 5, yy[0] + 5, yy[1] + 5, colors[step % 7])
tk.update()
time.sleep(0.01)
point = yy
if step == 50:
break
theta = -theta
angle = np.array([[math.cos(theta), math.sin(theta)], [-math.sin(theta), math.cos(theta)]])
point = mouth + np.array([-50, 25])
step = 0
while True:
xx = point - mouth
yy = angle.dot(xx)
yy = yy + mouth
step = step + 1
Agent(yy[0] - 5, yy[1] - 5, yy[0] + 5, yy[1] + 5, colors[step % 7])
tk.update()
time.sleep(0.01)
point = yy
if step == 16:
break
label = Label(tk, text="end!", font=('heiti', 40), fg='red')
label.place(x=285, y=585)
tk.mainloop()
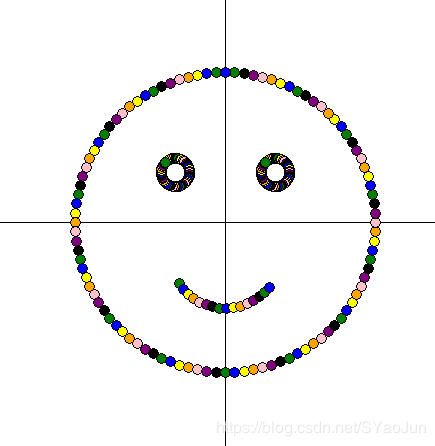