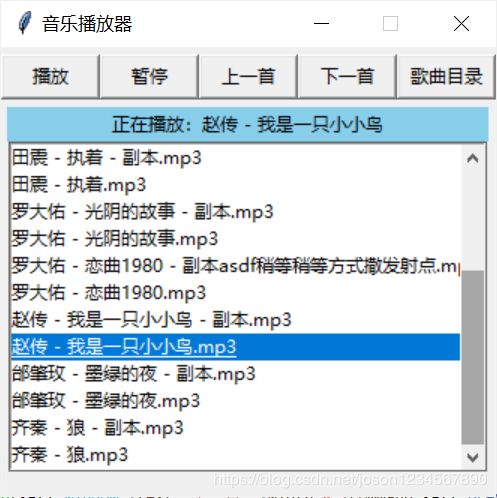
1.按钮功能区
触发功能按钮,播放,暂停,上/下一首,歌曲目录
def songpath(self):
"""选择歌曲路径"""
mixer.init()
self.songlist = dict()
directory = tkinter.filedialog.askdirectory()
try:
self.songlistbox.delete(0, 'end')
for files in os.listdir(directory):
if files.endswith('.mp3'):
realdir = os.path.realpath(files)
self.songlist[files] = directory + '/' + files
self.songlistbox.insert('end', files)
except:
self.songlistbox.delete(0, 'end')
def play(self):
"""播放"""
total = self.songlistbox.size()
if total != 0:
if self.songlistbox.curselection():
if self.stopbut['text'] == '继续':
self.stopbut['text'] = '暂停'
self.index = self.songlistbox.curselection()[0]
song = self.songlistbox.get(self.index)
mixer.init()
mixer.music.load(self.songlist[song])
mixer.music.play(-1)
self.playingsong.set('正在播放:' + song[:-4])
def previoussong(self):
"""上一首"""
total = self.songlistbox.size()
if total != 0 and self.index != None:
if self.stopbut['text'] == '继续':
self.stopbut['text'] = '暂停'
self.songlistbox.selection_clear(self.index)
self.index -= 1
if self.index < 0:
self.index = total - 1
self.songlistbox.selection_set(self.index)
self.index = self.songlistbox.curselection()[0]
song = self.songlistbox.get(self.index)
mixer.music.load(self.songlist[song])
mixer.music.play(-1)
self.playingsong.set('正在播放:' + song[:-4])
def nextsong(self):
"""下一首"""
total = self.songlistbox.size()
if total != 0 and self.index != None:
if self.stopbut['text'] == '继续':
self.stopbut['text'] = '暂停'
self.songlistbox.selection_clear(self.index)
self.index += 1
if self.index > total:
self.index = 0
self.songlistbox.selection_set(self.index)
self.index = self.songlistbox.curselection()[0]
song = self.songlistbox.get(self.index)
mixer.music.load(self.songlist[song])
mixer.music.play(-1)
self.playingsong.set('正在播放:' + song[:-4])
def stopsong(self):
"""暂停"""
total = self.songlistbox.size()
if total != 0 and self.index != None:
if self.stopbut['text'] == '暂停':
self.stopbut['text'] = '继续'
mixer.music.pause()
else:
self.stopbut['text'] = '暂停'
mixer.music.unpause()
2.动态Label标签
使用 textvariable 参数,set 设定动态标签内容
def label(self):
self.playingsong = StringVar()
self.playingsong.set('暂无播放歌曲')
self.playinglabel = tkinter.Label(self.window, width='45', bg='#87CEEB', textvariable=self.playingsong)
self.playinglabel.grid(row=1, column=0, columnspan=5)
3.歌单列表ListBox
根据选择的目录获取到具体歌曲信息,插入到listbox中
def listbox(self):
self.songlistbox = tkinter.Listbox(self.window, width=45, height=12, selectmode='single')
self.songlistbox.grid(row=2, column=0, columnspan=5)
yscrollbar = tkinter.Scrollbar(self.window, command=self.songlistbox.yview)
yscrollbar.place(x=306, y=65, height=217, anchor='nw')
self.songlistbox.config(yscrollcommand=yscrollbar.set)