最近网课非常多,所以想制作一个能自动刷课的程序。自己拿去使用的话代码只需要修改5处以内。主要方法是driver.find_element_by_xpath("********* ").click() 来完成模拟鼠标点击播放视频等一系列操作,绝对安全。关于xpath的获取可以自行搜索其他教程。
需要提前完成selenium的自动化环境搭建(浏览器驱动driver和python selenium的安装)
分成十个部分介绍:
from selenium import webdriver
import time
import threading
import re
import datetime
driver = webdriver.Chrome()
driver.get('自己学校的尔雅网址') #注意如果是通过学号登录,这个地址进入后一定是已经默认选择好学校的
由程序打开网页,提前设置好自己的账号密码,手动输入验证码,进入个人主页面。
def input_usename_and_password():
inp_captcha = input("请输入验证码:")
username = driver.find_element_by_id("unameId")
password = driver.find_element_by_id("passwordId")
verycode = driver.find_element_by_id("numcode")
username.send_keys(你的账号)
password.send_keys("你的密码")
verycode.send_keys(inp_Captcha)
sbm = driver.find_element_by_class_name("zl_btn_right")
time.sleep(1)
sbm.click()
def level_1st():
driver.switch_to.frame("frame_content")
time.sleep(1)
c_click = driver.find_element_by_xpath("这门课程的xpath")
c_click.click()
time.sleep(1)
driver.switch_to.window(driver.window_handles[-1])
def into_vedio_window():
time.sleep(1)
driver.find_element_by_xpath('从某一节课开始的xpath').click() #播放开始后,可以重新点击其他课程播放
time.sleep(1)
def play_vedio():
try:
time.sleep(2)
driver.switch_to.frame("iframe")
driver.switch_to.frame(0)
driver.find_element_by_xpath("//*[@id='video']/button").click()
#print("课程已经开始播放")
except:
pass
使用正则表达式,通过判断视频的进度width如果等于100%,则说明当前视频播放完成
def check_play(content):
time.sleep(1)
""""""
regex = r''
result = re.search(regex, content, re.S)
res = result.group(1) if result else None
print("\r当前播放到: {}".format(res)+'%',end=" ")
return res == '100'
七、播放下一个视频
这里要看具体情况,大部分网课有课后习题,所以需要点击两次下一页来进入下一个视频的播放页面
def next():
time.sleep(1)
try:
driver.switch_to.default_content()
driver.find_element_by_xpath('//*[@id="right2"]').click()
time.sleep(1.5)
driver.find_element_by_xpath('//*[@id="right2"]').click()
time.sleep(1.5)
except:
print("点击下一页失败,请检查页面情况")
def if_goal(): #有的网课每个章节的第一个视频有课程目标,需要跳过
if driver.find_element_by_xpath('//*[@id="right3"]'):
san = driver.find_element_by_xpath('//*[@id="right3"]')
san.click()
八、自动提交视频中选择题
通过判断每个选项的 value 值 ,如果为true即为正确答案。通过我的大量测试,发现"提交"这个按钮有三种xpath:ext-gen1043 ,ext-gen1044 ,ext-gen1045。使用多线程来运行。
class Test(threading.Thread):
def if_question1(self):
print('1045答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit1 = driver.find_element_by_xpath('//*[@id="ext-gen1045"]').click() # 提交
print('1045答题完成')
driver.switch_to.default_content()
except:
#print("1号没有检测到题目")
pass
def if_question2(self):
print('1044答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit2 = driver.find_element_by_xpath(' //*[@id="ext-gen1044"]').click() # 提交
print('1044答题完成')
driver.switch_to.default_content()
except:
#print("2号没有检测到题目")
pass
def if_question3(self):
print('1043答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit3 = driver.find_element_by_xpath(' //*[@id="ext-gen1043"]').click() # 提交
print('1043答题完成')
driver.switch_to.default_content()
except:
#print("3号没有检测到题目")
pass
九、展示当前课程序号以及系统时间
更好的了解课程进度
def print_chapter():
time.sleep(1)
span = driver.find_element_by_xpath('//h4[@class="currents"]/a/span/span')
print("课程"+span.text+"播放完毕")
print(datetime.datetime.now())
十、主方法
if __name__ == '__main__':
test = Test()
input_usename_and_password()
level_1st()
if_tongzhi()
into_vedio_window()
#into_vedio_vedio()
play_vedio()
t1 = threading.Thread(target=test.if_question1)
t2 = threading.Thread(target=test.if_question2)
t3 = threading.Thread(target=test.if_question3)
t1.start()
t2.start()
t3.start()
while True:
time.sleep(2)
if check_play( driver.page_source):
next()
print_chapter()
if_goal()
play_vedio()
else:
check_play(driver.page_source)
play_vedio()
运行展示
看起来有些乱,这是我之前运行的截图,在写这篇文章的过程中有一两处修改,比如展示系统时间。还需要继续做一些优化,出现的报错是因为网页在提交东西,不影响程序运行。
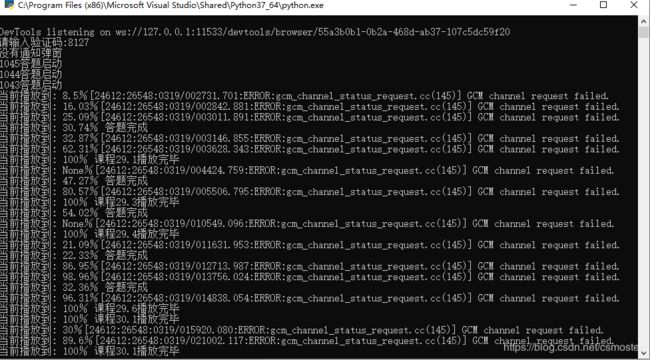
完整代码
from selenium import webdriver
import time
import threading
import re
import datetime
driver = webdriver.Chrome()
driver.get('自己学校的尔雅网址') #注意如果是通过学号登录,这个地址进入后一定是已经默认选择好学校的
def input_usename_and_password():
inp_captcha = input("请输入验证码:")
username = driver.find_element_by_id("unameId")
password = driver.find_element_by_id("passwordId")
verycode = driver.find_element_by_id("numcode")
username.send_keys(你的账号)
password.send_keys("你的密码")
verycode.send_keys(inp_Captcha)
sbm = driver.find_element_by_class_name("zl_btn_right")
time.sleep(1)
sbm.click()
def level_1st():
driver.switch_to.frame("frame_content")
time.sleep(1)
c_click = driver.find_element_by_xpath("这门课程的xpath")
c_click.click()
time.sleep(1)
driver.switch_to.window(driver.window_handles[-1])
def into_vedio_window():
time.sleep(1)
driver.find_element_by_xpath('从那节课开始的xpath').click()
time.sleep(1)
def into_vedio_vedio():
time.sleep(1)
driver.find_element_by_xpath"//*[@id='dct2']").click()
time.sleep(1)
def play_vedio():
try:
time.sleep(2)
driver.switch_to.frame("iframe")
driver.switch_to.frame(0)
driver.find_element_by_xpath("//*[@id='video']/button").click()
#print("课程已经开始播放")
except:
pass
def check_play(content):
time.sleep(1)
""""""
regex = r''
result = re.search(regex, content, re.S)
res = result.group(1) if result else None
print("\r当前播放到: {}".format(res)+'%',end=" ")
return res == '100'
def next():
time.sleep(3)
try:
driver.switch_to.default_content()
time.sleep(3)
driver.find_element_by_xpath('//*[@id="right2"]').click()
time.sleep(3)
driver.find_element_by_xpath('//*[@id="right2"]').click()
time.sleep(3)
except:
print("点击下一页失败,请检查页面情况")
class Test(threading.Thread):
def if_question1(self):
print('1045答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit1 = driver.find_element_by_xpath('//*[@id="ext-gen1045"]').click() # 提交
print('1045答题完成')
driver.switch_to.default_content()
except:
#print("1号没有检测到题目")
pass
def if_question2(self):
print('1044答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit2 = driver.find_element_by_xpath(' //*[@id="ext-gen1044"]').click() # 提交
print('1044答题完成')
driver.switch_to.default_content()
except:
#print("2号没有检测到题目")
pass
def if_question3(self):
print('1043答题启动')
while True:
time.sleep(5)
try:
innnn1 = driver.find_elements_by_xpath('//input')
input = driver.find_elements_by_xpath('//input[@value="true"]')[0]
input.click()
submit3 = driver.find_element_by_xpath(' //*[@id="ext-gen1043"]').click() # 提交
print('1043答题完成')
driver.switch_to.default_content()
except:
#print("3号没有检测到题目")
pass
def print_chapter():
time.sleep(1)
span = driver.find_element_by_xpath('//h4[@class="currents"]/a/span/span')
time1 = datetime.datetime.strptime(string,'%Y-%m-%d %H:%M:%S')
print("课程"+span.text+"播放完毕"+time1)
if __name__ == '__main__':
test = Test()
input_usename_and_password()
level_1st()
if_tongzhi()
into_vedio_window()
#into_vedio_vedio()
play_vedio()
t1 = threading.Thread(target=test.if_question1)
t2 = threading.Thread(target=test.if_question2)
t3 = threading.Thread(target=test.if_question3)
t1.start()
t2.start()
t3.start()
while True:
time.sleep(2)
if check_play( driver.page_source):
next()
print_chapter()
if_goal()
play_vedio()
else:
check_play(driver.page_source)
play_vedio()