文章目录
- Position
- Size
- Area
- CompArea
- UnitArea
Position
struct Position
{
PosType x;
PosType y;
Position() : x(0), y(0) { }
Position(const PosType _x, const PosType _y) : x(_x), y(_y) { }
bool operator!=(const Position &other) const { return x != other.x || y != other.y; }
bool operator==(const Position &other) const { return x == other.x && y == other.y; }
Position offset(const Position pos) const { return Position(x + pos.x, y + pos.y); }
Position offset(const PosType _x, const PosType _y) const { return Position(x + _x , y + _y ); }
void repositionTo(const Position newPos) { x = newPos.x; y = newPos.y; }
void relativeTo (const Position origin) { x -= origin.x; y -= origin.y; }
Position operator-( const Position &other ) const { return{ x - other.x, y - other.y }; }
};
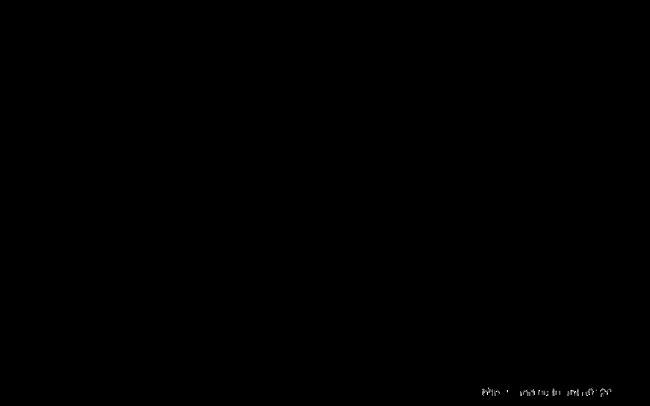
Size
struct Size
{
SizeType width;
SizeType height;
Size() : width(0), height(0) { }
Size(const SizeType _width, const SizeType _height) : width(_width), height(_height) { }
bool operator!=(const Size &other) const { return (width != other.width) || (height != other.height); }
bool operator==(const Size &other) const { return (width == other.width) && (height == other.height); }
uint32_t area() const { return (uint32_t) width * (uint32_t) height; }
void resizeTo(const Size newSize) { width = newSize.width; height = newSize.height; }
};
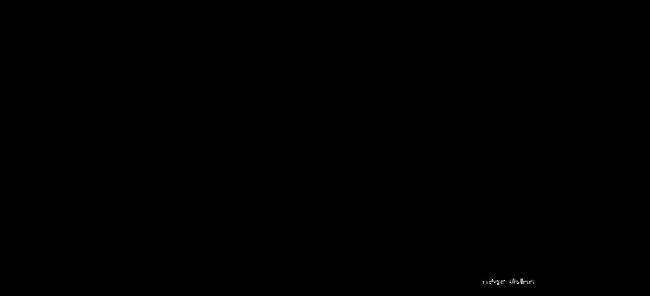
Area
struct Area : public Position, public Size
{
Area() : Position(), Size() { }
Area(const Position &_pos, const Size &_size) : Position(_pos), Size(_size) { }
Area(const PosType _x, const PosType _y, const SizeType _w, const SizeType _h) : Position(_x, _y), Size(_w, _h) { }
Position& pos() { return *this; }
const Position& pos() const { return *this; }
Size& size() { return *this; }
const Size& size() const { return *this; }
const Position& topLeft() const { return *this; }
Position topRight() const { return { (PosType) (x + width - 1), y }; }
Position bottomLeft() const { return { x , (PosType) (y + height - 1) }; }
Position bottomRight() const { return { (PosType) (x + width - 1), (PosType) (y + height - 1) }; }
Position center() const { return { (PosType) (x + width / 2), (PosType) (y + height / 2) }; }
bool contains(const Position &_pos) const { return (_pos.x >= x) && (_pos.x < (x + width)) && (_pos.y >= y) && (_pos.y < (y + height)); }
bool contains(const Area &_area) const { return contains(_area.pos()) && contains(_area.bottomRight()); }
bool operator!=(const Area &other) const { return (Size::operator!=(other)) || (Position::operator!=(other)); }
bool operator==(const Area &other) const { return (Size::operator==(other)) && (Position::operator==(other)); }
};
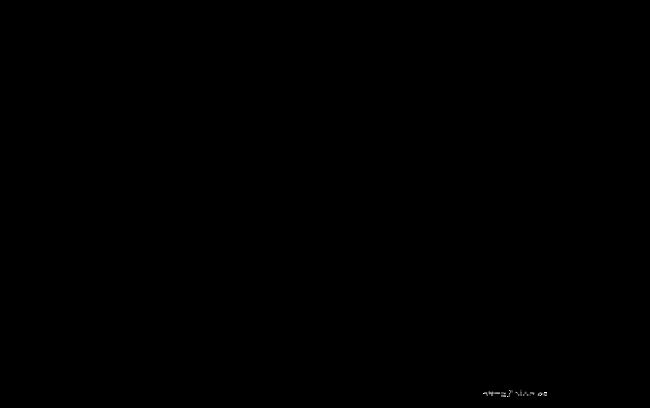
CompArea
struct CompArea : public Area
{
CompArea() : Area(), chromaFormat(NUM_CHROMA_FORMAT), compID(MAX_NUM_TBLOCKS) { }
CompArea(const ComponentID _compID, const ChromaFormat _cf, const Area &_area, const bool isLuma = false) : Area(_area), chromaFormat(_cf), compID(_compID) { if (isLuma) xRecalcLumaToChroma(); }
CompArea(const ComponentID _compID, const ChromaFormat _cf, const Position& _pos, const Size& _size, const bool isLuma = false) : Area(_pos, _size), chromaFormat(_cf), compID(_compID) { if (isLuma) xRecalcLumaToChroma(); }
CompArea(const ComponentID _compID, const ChromaFormat _cf, const uint32_t _x, const uint32_t _y, const uint32_t _w, const uint32_t _h, const bool isLuma = false) : Area(_x, _y, _w, _h), chromaFormat(_cf), compID(_compID) { if (isLuma) xRecalcLumaToChroma(); }
ChromaFormat chromaFormat;
ComponentID compID;
Position chromaPos() const;
Position lumaPos() const;
Size chromaSize() const;
Size lumaSize() const;
Position compPos( const ComponentID compID ) const;
Position chanPos( const ChannelType chType ) const;
Position topLeftComp (const ComponentID _compID) const { return recalcPosition(chromaFormat, compID, _compID, *this); }
Position topRightComp (const ComponentID _compID) const { return recalcPosition(chromaFormat, compID, _compID, { (PosType) (x + width - 1), y }); }
Position bottomLeftComp (const ComponentID _compID) const { return recalcPosition(chromaFormat, compID, _compID, { x , (PosType) (y + height - 1 )}); }
Position bottomRightComp(const ComponentID _compID) const { return recalcPosition(chromaFormat, compID, _compID, { (PosType) (x + width - 1), (PosType) (y + height - 1 )}); }
bool valid() const { return chromaFormat < NUM_CHROMA_FORMAT && compID < MAX_NUM_TBLOCKS && width != 0 && height != 0; }
const bool operator==(const CompArea &other) const
{
if (chromaFormat != other.chromaFormat) return false;
if (compID != other.compID) return false;
return Position::operator==(other) && Size::operator==(other);
}
const bool operator!=(const CompArea &other) const { return !(operator==(other)); }
#if REUSE_CU_RESULTS_WITH_MULTIPLE_TUS
void resizeTo (const Size& newSize) { Size::resizeTo(newSize); }
#endif
void repositionTo (const Position& newPos) { Position::repositionTo(newPos); }
void positionRelativeTo(const CompArea& origCompArea) { Position::relativeTo(origCompArea); }
private:
void xRecalcLumaToChroma();
};
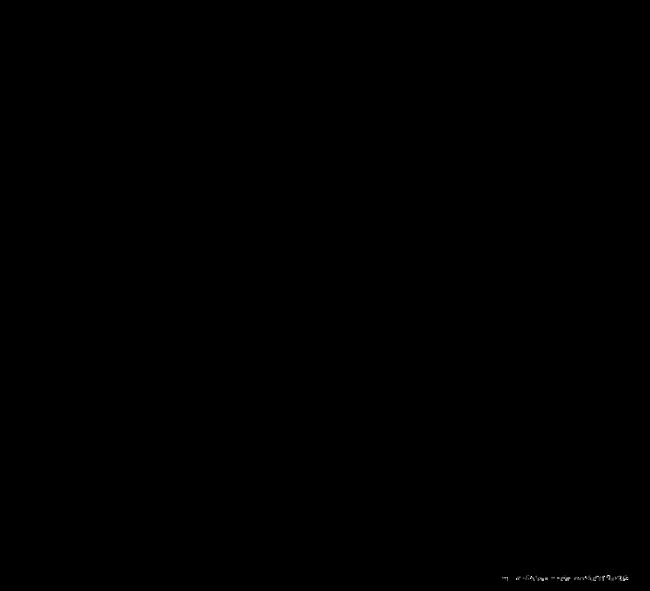
UnitArea
struct UnitArea
{
ChromaFormat chromaFormat;
UnitBlocksType blocks;
UnitArea() : chromaFormat(NUM_CHROMA_FORMAT) { }
UnitArea(const ChromaFormat _chromaFormat);
UnitArea(const ChromaFormat _chromaFormat, const Area &area);
UnitArea(const ChromaFormat _chromaFormat, const CompArea &blkY);
UnitArea(const ChromaFormat _chromaFormat, CompArea &&blkY);
UnitArea(const ChromaFormat _chromaFormat, const CompArea &blkY, const CompArea &blkCb, const CompArea &blkCr);
UnitArea(const ChromaFormat _chromaFormat, CompArea &&blkY, CompArea &&blkCb, CompArea &&blkCr);
CompArea& Y() { return blocks[COMPONENT_Y]; }
const CompArea& Y() const { return blocks[COMPONENT_Y]; }
CompArea& Cb() { return blocks[COMPONENT_Cb]; }
const CompArea& Cb() const { return blocks[COMPONENT_Cb]; }
CompArea& Cr() { return blocks[COMPONENT_Cr]; }
const CompArea& Cr() const { return blocks[COMPONENT_Cr]; }
CompArea& block(const ComponentID comp) { return blocks[comp]; }
const CompArea& block(const ComponentID comp) const { return blocks[comp]; }
bool contains(const UnitArea& other) const;
bool contains(const UnitArea& other, const ChannelType chType) const;
CompArea& operator[]( const int n ) { return blocks[n]; }
const CompArea& operator[]( const int n ) const { return blocks[n]; }
const bool operator==(const UnitArea &other) const
{
if (chromaFormat != other.chromaFormat) return false;
if (blocks.size() != other.blocks.size()) return false;
for (uint32_t i = 0; i < blocks.size(); i++)
{
if (blocks[i] != other.blocks[i]) return false;
}
return true;
}
#if REUSE_CU_RESULTS_WITH_MULTIPLE_TUS
void resizeTo (const UnitArea& unit);
#endif
void repositionTo(const UnitArea& unit);
const bool operator!=(const UnitArea &other) const { return !(*this == other); }
const Position& lumaPos () const { return Y(); }
const Size& lumaSize() const { return Y(); }
const Position& chromaPos () const { return Cb(); }
const Size& chromaSize() const { return Cb(); }
const UnitArea singleComp(const ComponentID compID) const;
const UnitArea singleChan(const ChannelType chType) const;
const SizeType lwidth() const { return Y().width; }
const SizeType lheight() const { return Y().height; }
const PosType lx() const { return Y().x; }
const PosType ly() const { return Y().y; }
bool valid() const { return chromaFormat != NUM_CHROMA_FORMAT && blocks.size() > 0; }
};
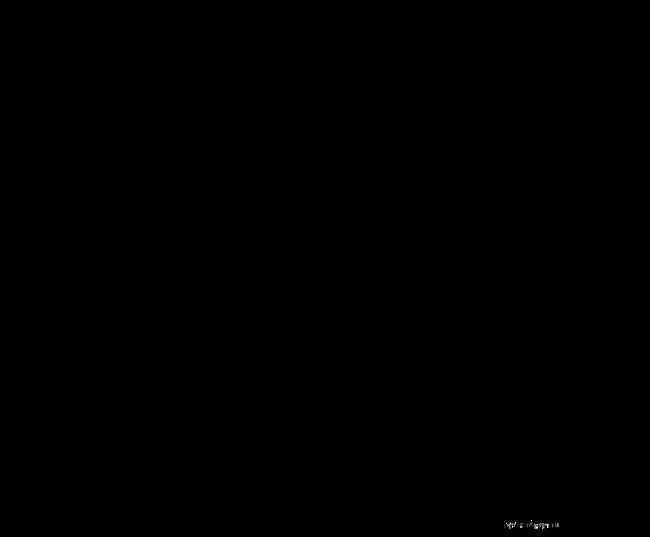