3.1简单模拟
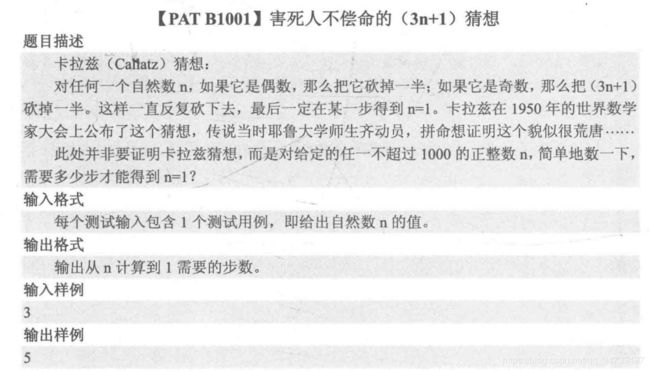
#include
#include
using namespace std;
int main()
{
int n, step = 0;
scanf("%d", &n);
while (1) {
if (n == 1) {
break;
}
if (n % 2 == 0) {
n /= 2;
}
else {
n = (3 * n + 1) / 2;
}
++step;
}
printf("%d\n", step);
system("pause");//vs里面防止控制台一闪而过。
return 0;
}
#include
#include
using namespace std;
int main()
{
int N,n,schoolcode,grade,maxschool=-1;
int stu[100001] = {0};
scanf("%d", &N);
n = N;
while (N--) {
scanf("%d%d", &schoolcode, &grade);
stu[schoolcode] += grade;
}
for (int i = 1; i <= n; ++i) {
if (stu[i] > stu[maxschool]) {
maxschool = i;
}
}
printf("%d %d\n", maxschool, stu[maxschool]);
system("pause");
return 0;
3.2查找元素
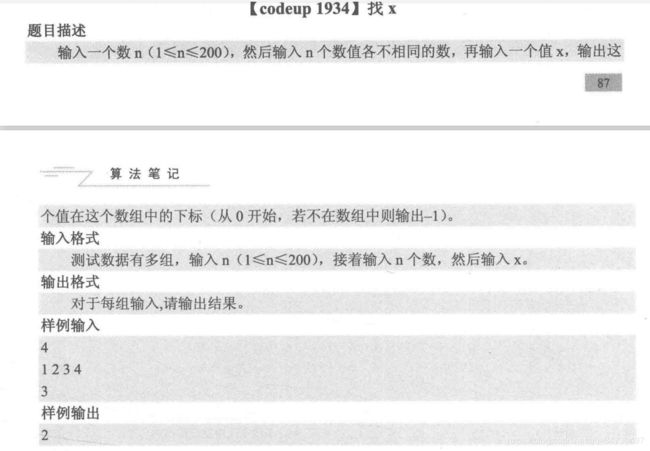
#include
#include
using namespace std;
int main()
{
int n, x,index=-1;
int num[1001];
scanf("%d", &n);
for (int i = 0; i < n; ++i) {
scanf("%d", &num[i]);//注意加&。
}
scanf("%d", &x);
for (int i = 0; i < n; ++i) {
if (num[i] == x) {
index = i;
break;
}
}
printf("%d\n", index);
system("pause");
return 0;
}
3.3图形输出
#include
using namespace std;
int main()
{
char C;
int N,row;
scanf("%d %c", &N, &C);
if (N % 2 == 0) {
row = N / 2;
}
else {
row = N / 2 + 1;
}
for (int i = 0; i < N; ++i) {
printf("%c", C);
}
printf("\n");
for (int i = 2; i < row ; ++i) {
printf("%c", C);
for (int j = 0; j < N-2; ++j) {
printf(" ");
}
printf("%c\n", C);
}
for (int i = 0; i < N; ++i) {
printf("%c", C);
}
system("pause");
return 0;
3.4日期差值
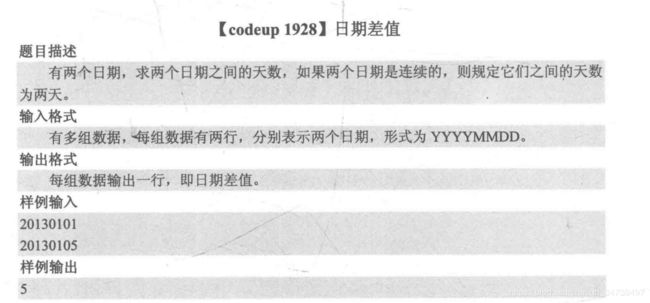
#include
#include
using namespace std;
int month[2][12] = {
31,28,31,30,31,30,31,31,30,31,30,31,
31,29,31,30,31,30,31,31,30,31,30,31
};
int leap(int year) {
return ((year % 4) && (year % 100 != 0)) || (year % 400 == 0);
}
int main()
{
int day ;
int time1,time2,startyear,endyear, startmonth,endmonth, startday,endday;
while (scanf("%d%d", &time1, &time2) != EOF) {
day = 1;
if (time1 > time2) {
swap(time1, time2);
}
startyear = time1 / 10000, startmonth = time1 % 10000 / 100, startday = time1 % 100;
endyear = time2 / 10000, endmonth = time2 % 10000 / 100, endday = time2 % 100;
while (startyear < endyear || startmonth < endmonth || startday < endday) {
startday++;
if (startday == month[leap(startyear)][startmonth] + 1) {
++startmonth;
startday = 1;
}
if (startmonth == 13) {
++startyear;
startmonth = 1;
}
++day;
}
printf("%d\n", day);
}
system("pause");
return 0;
}
3.5进制转换
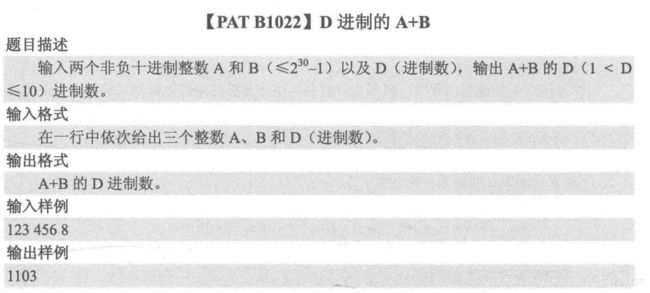
#include
#include
using namespace std;
int main()
{
int A, B,D,num,index=0;
int z[40] ;
scanf("%d%d%d", &A, &B,&D);
num = A + B;
do{
z[index++] = num%D;
num /= D;
} while (num);
for (int i = index-1; i>=0; --i) {
printf("%d", z[i]);
}
printf("\n");
system("pause");
return 0;
}
3.6字符串处理
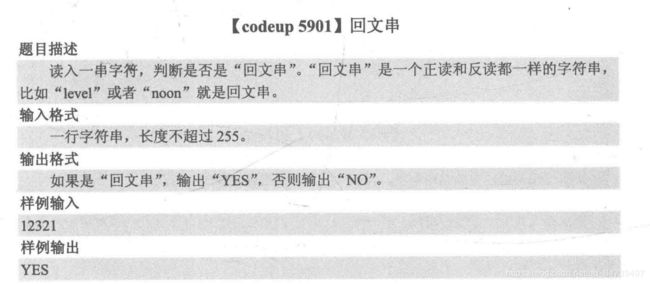
#include
#include
#include
using namespace std;
int main()
{
string s;
bool flag = true;
cin >> s;
for (int i = 0, j = s.length() - 1; i < j; ++i, --j) {
if (s[i] != s[j]) {
flag = false;
break;
}
}
if (flag) {
printf("YES\n");
}
else {
printf("NO\n");
}
system("pause");
return 0;
}
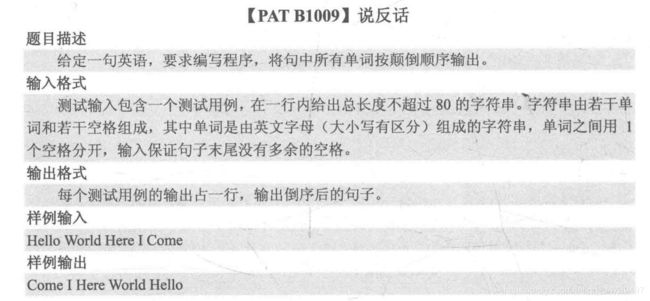
#include
#include
#include
using namespace std;
void Reverse(string &s,int left,int right) {
while (left < right) {
swap(s[left++], s[right--]);
}
}
int main()
{
string s;
int i, j, k=0;
getline(cin, s);
Reverse(s,0,s.length()-1);
for (i=0 ; i