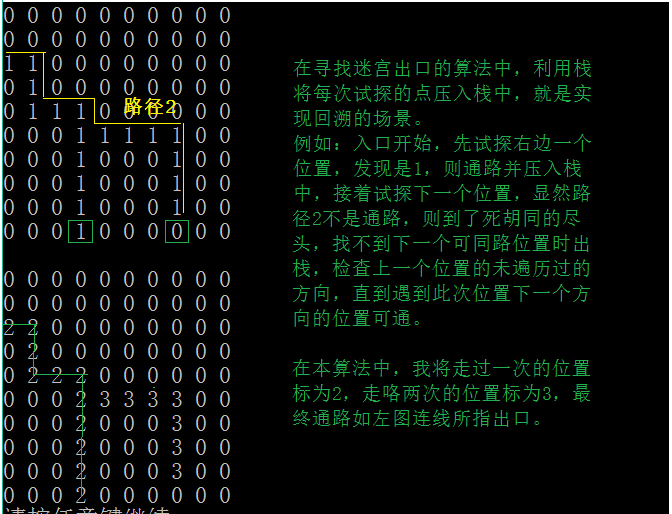
#include
using namespace std;
#include
//#include
#define N 10//矩阵最大行列数
template
class Mazestack//迷宫将要用到的栈
{
public:
Mazestack()
:_ptr(NULL)
,_size(0)
,_capacity()
{
}
~Mazestack()
{
if (_ptr!=NULL)
{
delete[]_ptr;
_ptr = NULL;
_size = 0;
_capacity = 0;
}
}
void Push(T& x)
{
if (_size==_capacity)
{
Expansion();
}
this->_ptr[_size++]=x;
}
void Pop()
{
if (_size!=0)
{
_size--;
}
}
T& Top()
{
return _ptr[_size-1];
}
bool Empty()
{
return _ptr==NULL;
}
int Size()
{
return _size;
}
private:
void Expansion()//扩容
{
if (_capacity==_size)
{
int newcapa=_capacity*2+2;
T* tmp=new T[newcapa];
assert(tmp);
swap(tmp, _ptr);
for(size_t i=0;i<_size;i++)
{
tmp[i]=_ptr[i];
}
delete[]_ptr;
_ptr=tmp;
_capacity=newcapa;
}
}
private:
T* _ptr;
size_t _size;
size_t _capacity;
};
struct Location//位置信息
{
Location(int row=0, int col=0)
:_col(col)
,_row(row)
{
}
bool operator==(Location l)
{
if ((l._row==_row)&&(l._col==_col))
{
return true;
}
return false;
}
// Location& operator=(Location& l)
// {
// _row=l._row;
// _col=l._col;
// return *this;
// }
int _row;
int _col;
};
bool Checkpass(int arr[][N],Location pos)//判断是否通路
{
if ((pos._row>=0)&&(pos._row<=N)&&
(pos._col>=0)&&(pos._col<=N)&&
(arr[pos._row][pos._col]==1))
{
return true;
}
return false;
}
void Initmaze(int (*arr)[N])//初始化迷宫
{
FILE* fp=fopen("Maze.txt","r");
assert(fp);
for (int i=0; i& s,Location& start)
{
Location cur=start;
s.Push(start);
maze[cur._row][cur._col]=2;
while (!s.Empty())
{
Location next=s.Top();
cur=next;
maze[next._row][next._col]=2;
if (cur==exp)
{
return true;
}
//上
cur._row--;
if(Checkpass(maze,cur))
{
s.Push(cur);
continue;
}
cur._row++;
//右
cur._col++;
if(Checkpass(maze,cur))
{
s.Push(cur);
continue;
}
cur._col--;
//下
cur._row++;
if(Checkpass(maze,cur))
{
s.Push(cur);
continue;
}
cur._row--;
//左
cur._col--;
if(Checkpass(maze,cur))
{
s.Push(cur);
continue;
}
cur._col++;
s.Pop();
maze[cur._row][cur._col]=3;
}
return false;
}
int main()
{
Location exp(9,3);//出口
Location start(2,0);//入口
Mazestack s; //栈
int maze[N][N]={0};
Initmaze(maze);
PrintMaze(maze);
Run(maze, exp, s, start);
cout<