题目如下
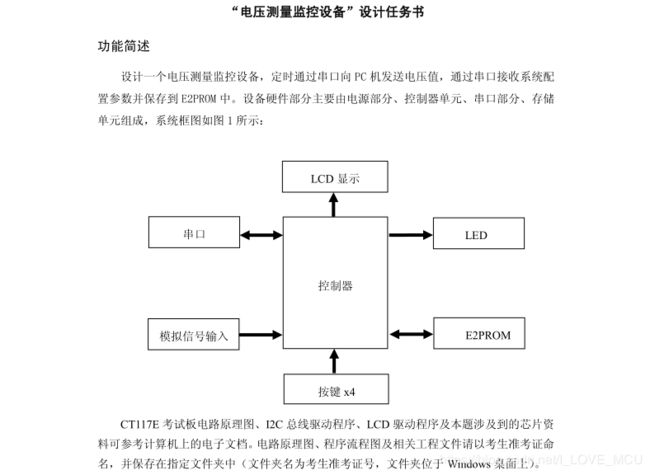
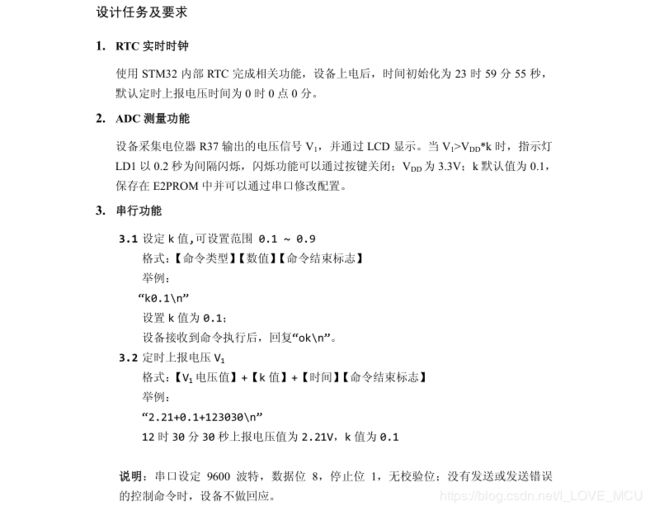
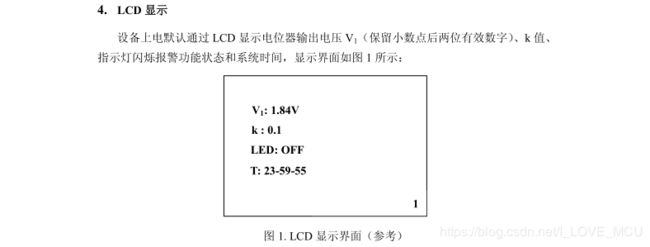
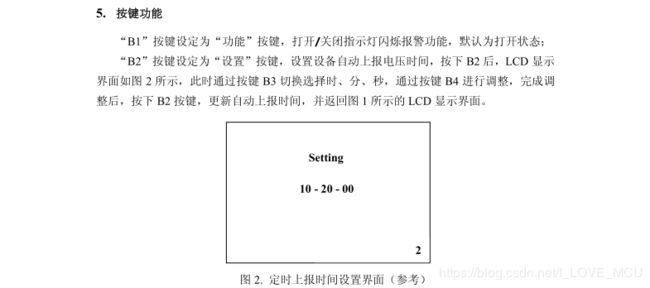
主要代码如下
#include "includeall.h"
vu32 TimingDelay = 0;
void Init(void)
{
Usart2_Init(9600);
SysTick_Config(SystemCoreClock/1000);
Delay_Ms(200);
LED_Init();
STM3210B_LCD_Init();
Beep_Init();
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
i2c_init();
Key_Init();
TIM2_Init(1999, 7199);
TIM3_Init(79, 7199);
ADC8_Init();
RTC_Init();
}
int main(void)
{
Init();
LCD_Clear(White);
LCD_SetBackColor(White);
LCD_SetTextColor(Black);
while(1)
{
LCD_Show();
KeyMonitor();
LED_Shine();
usartDataJudge();
}
}
void Delay_Ms(u32 nTime)
{
TimingDelay = nTime;
while(TimingDelay != 0);
}
#include "includeall.h"
#include "../Driver/USART/usart.h"
void Usart2_Init(u32 baud)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = baud;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx|USART_Mode_Tx;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_Init(USART2, &USART_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
USART_Cmd(USART2, ENABLE);
}
void USART2_SendByte(u8 data)
{
USART_ClearFlag(USART2, USART_FLAG_TC);
USART_SendData(USART2, data);
while(USART_GetFlagStatus(USART2, USART_FLAG_TC) == 0);
}
void USART2_SendString(u8 *str)
{
u8 i=0, num = 0;
while(str[i]!=0)
i++;
num = i;
for(i=0; i<num; i++)
{
USART2_SendByte(str[i]);
}
}
void USART2_IRQHandler(void)
{
u8 res = 0;
if( USART_GetITStatus(USART2, USART_IT_RXNE) )
{
TIM_SetCounter(TIM3, 0);
res = USART_ReceiveData(USART2);
if(g_stopreceive == 0)
{
g_usartrec[g_usartnum] = res;
g_usartnum++;
}
else
{
TIM_Cmd(TIM3, ENABLE);
g_usartnum = 0;
g_stopreceive = 0;
memset((void *)g_usartrec, 0, 50);
g_usartrec[g_usartnum] = res;
g_usartnum++;
}
}
}
int fputc(int ch, FILE *f){
USART_SendData(USART2, (uint8_t) ch);
while (USART_GetFlagStatus(USART2, USART_FLAG_TC) == RESET)
{}
return ch;
}
#include "includeall.h"
volatile double g_V = 0;
volatile u8 g_K = 1;
volatile u8 g_LS = 1;
volatile u8 g_LK = 1;
vu8 g_TimeDisplay = 0;
volatile Tim_str g_timtemp = {23, 59, 55};
volatile Tim_str g_settime = {0, 0, 0};
volatile u8 g_page = MAIN;
volatile u8 g_underline = 0;
volatile u8 g_usartrec[50];
volatile u8 g_stopreceive = 1;
volatile u8 g_usartnum = 0;
volatile u8 g_iicsr = 0;
void LCD_DisplayADC(void)
{
u8 strtem[10];
u8 i = 0, num = 0;
sprintf((char *)strtem, "%5.2lfV", ADC_Measure() );
while(strtem[i]!=0)i++;
num = i;
for(i=0; i<num; i++)
LCD_DisplayChar(Line5, 319-16*(4+i), strtem[i]);
}
void My_LCD_DisplayChar(u8 Line, u16 Add, u8 Ascii)
{
LCD_DisplayChar(Line, 319-16*Add, Ascii);
}
void My_LCD_DisplayStringLine(u8 Line, u16 Add, u8 *ptr)
{
u32 i = 0;
while ((*ptr != 0) && (i < 20))
{
My_LCD_DisplayChar(Line, Add+i, *ptr);
ptr++;
i++;
}
}
double ADC_Measure(void)
{
g_V = ADC_Get_Ave(8,5)/4096.0*3.3;
return g_V;
}
void LED_Shine(void)
{
if (g_LK == 1)
{
if(g_V > 0.33*g_K)
{
g_LS = 1;
}
else
{
g_LS = 0;
}
}
else
{
LEDOff(LED1);
g_LS = 0;
}
}
void LCD_Show(void)
{
u8 strtemp[20];
if(g_page == MAIN)
{
sprintf( (char *)strtemp, " V1:%4.2lfV", ADC_Measure());
LCD_DisplayStringLine(Line2, strtemp);
sprintf( (char *)strtemp, " k:0.%d", g_K);
LCD_DisplayStringLine(Line3, strtemp);
if(g_LK)
LCD_DisplayStringLine(Line4, (u8 *)" LED:ON ");
else
LCD_DisplayStringLine(Line4, (u8 *)" LED:OFF ");
Time_Show();
sprintf( (char *)strtemp, " T:%2d-%2d-%2d", g_timtemp.hh, g_timtemp.mm, g_timtemp.ss);
LCD_DisplayStringLine(Line5, strtemp);
}
else if(g_page == SETT)
{
LCD_DisplayStringLine(Line3, (u8 *)" Setting ");
sprintf( (char *)strtemp, " %2d-%2d-%2d", g_settime.hh, g_settime.mm, g_settime.ss);
LCD_DisplayStringLine(Line5, strtemp);
if(g_underline%3 == 2)
LCD_DisplayStringLine(Line6, (u8 *)" __ ");
else if(g_underline%3 == 1)
LCD_DisplayStringLine(Line6, (u8 *)" __ ");
else if(g_underline%3 == 0)
LCD_DisplayStringLine(Line6, (u8 *)" __ ");
}
}
void ReportVoltage(void)
{
u8 data[25];
sprintf((char*)data, "%.2lf+0.%d+%2d%2d%2d\n", g_V, g_K, g_timtemp.hh, g_timtemp.mm, g_timtemp.ss);
USART2_SendString(data);
}
void KeyMonitor(void)
{
if(g_page == MAIN)
{
switch(Key_Scan(0) ){
case K1_PRES:
{
g_LK = !g_LK;
}
break;
case K2_PRES:
{
LCD_Clear(White);
g_page = SETT;
}
}
}
else if(g_page == SETT)
{
switch(Key_Scan(0) ){
case K1_PRES:
{
g_LK = !g_LK;
}
break;
case K2_PRES:
{
RTC_SetAlarm(Time_Regulate(g_settime.hh, g_settime.mm, g_settime.ss) );
RTC_WaitForLastTask();
LCD_Clear(White);
g_page = MAIN;
}
break;
case K3_PRES:
{
g_underline++;
}
break;
case K4_PRES:
{
k4settime();
}
}
}
}
void k4settime(void)
{
if(g_underline%3 == SUL)
{
if(g_settime.ss != 59 )
{
g_settime.ss++;
}
else
{
g_settime.ss=0;
if(g_settime.mm != 59)
g_settime.mm++;
else
{
g_settime.mm = 0;
g_settime.hh++;
}
}
}
else if(g_underline%3 == MUL)
{
if(g_settime.mm != 59 )
{
g_settime.mm++;
}
else
{
g_settime.mm=0;
if(g_settime.hh!=23)
g_settime.hh++;
else
{
g_settime.mm=0;
g_settime.hh=0;
}
}
}
else if(g_underline%3 == HUL)
{
if(g_settime.hh != 23 )
{
g_settime.hh++;
}
else
{
g_settime.hh=0;
}
}
}
void usartDataJudge(void)
{
if(g_stopreceive && g_iicsr)
{
g_iicsr = 0;
if( (g_usartrec[0] == 'k') && (g_usartrec[1] == '0') && (g_usartrec[2] == '.')
&& (g_usartrec[3] >='1' && (g_usartrec[3] <='9') ))
{
USART2_SendString("ok\n");
IIC24_SendOneData(0, g_usartrec[3]-48);
Delay_Ms(10);
g_K = IIC24_RecOneData(0);
}
}
}
#include "includeall.h"
#include "../Driver/ADC/adc.h"
void ADC8_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
ADC_InitTypeDef ADC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOB, ENABLE);
RCC_ADCCLKConfig(RCC_PCLK2_Div6);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOB, &GPIO_InitStructure);
ADC_DeInit(ADC1);
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = DISABLE;
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfChannel = 1;
ADC_Init(ADC1, &ADC_InitStructure);
ADC_Cmd(ADC1, ENABLE);
ADC_ResetCalibration(ADC1);
while(ADC_GetResetCalibrationStatus(ADC1));
ADC_StartCalibration(ADC1);
while(ADC_GetCalibrationStatus(ADC1));
}
u16 ADC_Get(u8 ch)
{
ADC_RegularChannelConfig(ADC1, ch, 1, ADC_SampleTime_55Cycles5);
ADC_SoftwareStartConvCmd(ADC1, ENABLE);
while(ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == 0);
return ADC_GetConversionValue(ADC1);
}
u16 ADC_Get_Ave(u8 ch, u8 times)
{
u8 i = 0;
u16 value = 0;
for(i=0; i<times; i++)
{
value = value + ADC_Get(ch);
Delay_Ms(5);
}
return value/times;
}
#include "includeall.h"
#include "../Driver/RTC/rtc.h"
void RTC_Init(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
PWR_BackupAccessCmd(ENABLE);
RCC_LSICmd(ENABLE);
while (RCC_GetFlagStatus(RCC_FLAG_LSIRDY) == RESET)
{}
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSI);
RCC_RTCCLKCmd(ENABLE);
RTC_WaitForSynchro();
RTC_WaitForLastTask();
NVIC_InitStructure.NVIC_IRQChannel = RTC_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 2;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
if (BKP_ReadBackupRegister(BKP_DR1) != 0xA5A4)
{
RTC_ITConfig(RTC_IT_SEC|RTC_IT_ALR, ENABLE);
RTC_WaitForLastTask();
RTC_SetPrescaler(39999);
RTC_WaitForLastTask();
Time_Adjust(23, 59, 55);
BKP_WriteBackupRegister(BKP_DR1, 0xA5A5);
}
else
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_BKP|RCC_APB1Periph_PWR,ENABLE);
PWR_BackupAccessCmd(ENABLE);
RCC_RTCCLKCmd(ENABLE);
RTC_WaitForSynchro();
RTC_SetAlarm(Time_Regulate(0, 0, 0));
RTC_ITConfig(RTC_IT_SEC|RTC_IT_ALR, ENABLE);
RTC_WaitForLastTask();
}
}
uint32_t Time_Regulate(u8 hh, u8 mm, u8 ss)
{
uint32_t Tmp_HH = 0xFF, Tmp_MM = 0xFF, Tmp_SS = 0xFF;
Tmp_HH = hh;
Tmp_MM = mm;
Tmp_SS = ss;
return((Tmp_HH*3600 + Tmp_MM*60 + Tmp_SS));
}
void Time_Adjust(u8 hh, u8 mm, u8 ss)
{
RTC_WaitForLastTask();
RTC_SetCounter(Time_Regulate(hh, mm, ss));
RTC_WaitForLastTask();
}
void Time_Display(uint32_t TimeVar)
{
u8 Time[30];
if (RTC_GetCounter() == 0x00015180)
{
RTC_SetCounter(0x0);
RTC_WaitForLastTask();
}
g_timtemp.hh = (TimeVar / 3600)<24?(TimeVar / 3600):0;
g_timtemp.mm = (TimeVar % 3600) / 60;
g_timtemp.ss = (TimeVar % 3600) % 60;
sprintf((char*)Time, "Time: %2d:%2d:%2d\r\n", g_timtemp.hh, g_timtemp.mm, g_timtemp.ss);
}
void Time_Show(void)
{
if (g_TimeDisplay == 1)
{
Time_Display(RTC_GetCounter());
g_TimeDisplay = 0;
}
}
void RTC_IRQHandler(void)
{
if (RTC_GetITStatus(RTC_IT_SEC) != RESET)
{
RTC_ClearITPendingBit(RTC_IT_SEC);
g_TimeDisplay = 1;
RTC_WaitForLastTask();
}
else if(RTC_GetITStatus(RTC_IT_ALR) )
{
ReportVoltage();
RTC_ClearITPendingBit(RTC_IT_ALR);
}
}