1. 安装Vuex
2. Vuex新建示例模块
-
构建如图所示 store 文件夹其中包括以下几个模块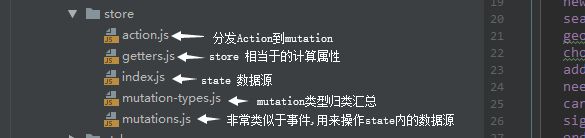
-
index.js 内容部分如下,实例中在 state 中初始化5条数据
import Vue from 'vue'
import Vuex from 'vuex'
import mutations from './mutations'
import actions from './action'
import getters from './getters'
Vue.use(Vuex)
const state = {
one: '',
two: '',
three:[],
four:'',
five:'',
}
export default new Vuex.Store({
state,
getters,
actions,
mutations,
})
import store from './store/index'
new Vue({
el: '#app',
router,
store,
components: { App },
template: ''
})
- 使用vuex
Vue.use(Vuex)
- 初始化数据状态
3. mutation事件定义
export const SET_ONE ='SET_ONE'
export const SET_TWO ='SET_TWO'
export const SET_THREE ='SET_THREE'
export const SET_FOUR ='GET_FOUR'
export const SET_FIVE ='GET_FIVE'
import {
SET_ONE,
SET_TWO,
SET_THREE,
SET_FOUR,
SET_FIVE
} from './mutation-types'
export default {
[SET_ONE](state, one) {
state.one = one
},
[SET_TWO](state, two) {
state.two = two
},
[SET_THREE](state, three) {
state.three = three
},
[SET_FOUR](state, four) {
state.four = four
},
[SET_FIVE](state, five) {
state.five = five
}
}
4. commit方法提交实战应用
- 通过 this.$store.commit() 把数据提交
- 计算属性更新数据 return this.$store.state.one
- 为了处理刷新state 数据源丢失问题可以通过本地存储数据 localStorage.setItem(),这样在其他组件或者页面的计算属性中调用 this.$store.state.one 实现数据同步更新
<template>
<div class="class">
<div>
<button @click="setStateOne">设置store.state.one为:一</button>
<p>store.state.one的值为:{{setOne}}</p>
</div>
</template>
<script>
export default {
name: "page",
data() {},
computed: {
setOne() {
return this.$store.state.one
}
},
mounted(){
let storageOne = localStorage.getItem('getOne')
console.log(storageOne)
if(storageOne){
this.$store.commit("SET_ONE", storageOne)
}
},
methods: {
setStateOne() {
let stateOne ='一'
localStorage.setItem('getOne',stateOne)
this.$store.commit("SET_ONE", stateOne)
}
}
}
</script>
5.mapState和mapMutation用法详解
<template>
<div class="class">
<button @click="setStateTwo">设置store.state.two为:二</button>
<p>store.state.two的值为:{{setTwo}}</p>
</div>
</template>
<script>
import {mapState,mapMutations} from 'vuex'
export default {
name: "page",
computed: {
...mapState({
setTwo:state=>state.two
})
},
methods: {
...mapMutations({
settwo:'SET_TWO',
setthree:'SET_THREE'
}),
setStateTwo(){
let stateTwo = '二'
this.settwo(stateTwo)
this.setthree(3)
}
}
}
</script>
6. 使用action和mapMutations 实现异步操作
import axios from '../axios'
export default {
setthree ({ commit }) {
return new Promise((resolve,reject)=>{
axios.ajax({
url:'www.baidu.com',
method:'get',
data:{
params:{
username:'myusername'
}
}
}).then((response)=>{
commit('SET_THREE',response)
resolve()
})
})
},
async getthree ({ commit }) {
let res = await getData();
commit('SET_THREE', res)
},
}
<template>
<div class="class">
<div>
<p>这里是服务器获取的list列表:{{listThree}}</p>
</div>
</div>
</template>
<script>
import {mapState,mapMutations} from 'vuex'
export default {
name: "page",
data() {
return {
listThree: [],
}
},
created(){
this.$store.dispatch("setthree").then(()=>{
this.listThree=this.three
})
this.setthree().then(()=>{
this.listThree=this.three
})
this.getMyThree()
},
computed:{
...mapState(['three'])
},
method:{
...mapActions([
"setthree"
])
...mapActions({
getMyThree:"getthree"
})
}
}
</script>
7. getters 和mapGetters
export default {
fourval (state) {
return state.four%2?'奇数':'偶数'
},
}
<script>
import {mapState,mapMutations} from 'vuex'
export default {
name: "page",
data() {
return {
dataFour:'' ,
}
},
created(){
this.dataFour=this.Objfour
},
computed:{
},
method:{
...mapGetters({
Objfour:'fourval'
})
}
}
</script>