最近一直使用数据库保存数据,想到了List集合保存对象更方便,便将方法已泛型的方式实现,便于通用
注 要使用到文件存储,所以在别忘记加文件权限
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
首先我们创建名为BookInfo 、PeopleInfo的javabean文件
public class BookInfo implements Serializable {
private String bookName ;
private String author ;
private float price ;
public BookInfo() {
}
public BookInfo(String bookName, String author, float price) {
this.bookName = bookName;
this.author = author;
this.price = price;
}
public String getBookName() { return bookName; }
public void setBookName(String bookName) {this.bookName = bookName; }
public String getAuthor() { return author; }
public void setAuthor(String author) {this.author = author;}
public float getPrice() { return price; }
public void setPrice(float price) { this.price = price; }
@Override
public String toString() {
return "{" + "bookName='" + bookName + '\'' + ", author='" + author + '\'' + ", price=" + price + '}';
}
}
public class PeopleInfo implements Serializable {
private String name ;
private int age ;
public PeopleInfo(String name, int age) { this.name = name; this.age = age; }
public String getName() {return name;}
public void setName(String name) {this.name = name;}
public int getAge() {return age;}
public void setAge(int age) {this.age = age;}
@Override
public String toString() {
return "PeopleInfo{" + "name='" + name + '\'' + ", age=" + age + '}';
}
}
创建DataUtils的工具类(主)
public class DataUtils {
public static void writeInfo(Context context , List info , String fileName) {
FileOutputStream fos = null ;
ObjectOutputStream oos = null ;
try {
fos=context.openFileOutput(fileName , Context.MODE_PRIVATE);
oos = new ObjectOutputStream(fos) ;
oos.writeObject(info);
} catch (IOException e) {
e.printStackTrace();
}
try {
if(oos != null) {
oos.close();
} else if(fos!=null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static List readInfo(Context context , String fileName) {
List info = new ArrayList<>() ;
FileInputStream fis = null ;
ObjectInputStream ois = null ;
try {
fis= context.openFileInput(fileName) ;
ois = new ObjectInputStream(fis) ;
info = (List) ois.readObject() ;
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
try {
if(ois != null) {
ois.close();
} else if(fis!=null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
return info;
}
public static void sortInfo(List list , final String methodName , final Integer state) {
if(methodName == null || state == null){
return ;
}
if(!methodExis(list.get(0) , methodName)) {
System.out.println("方法不存在") ;
return ;
}
Collections.sort(list, new Comparator() {
@Override
public int compare(T o1, T o2) {
Object ob1 = getData(o1 , methodName);;
Object ob2 = getData(o2 , methodName);
if(ob1 == null || ob2 == null) {
return 0 ;
}
if(ob1 instanceof Integer || ob1 instanceof Float || ob1 instanceof Double) {
if (Double.parseDouble(ob1+"") >= Double.parseDouble(ob2+"") ) {
return state==0? 1:state ==1? -1:0 ;
}
return state==0? -1:state ==1? 1:0 ;
} else if(ob1 instanceof String) {
Collator collator = Collator.getInstance(Locale.CHINA) ;
if ( collator.compare(((String)ob1) , (String)ob2) >0 ) {
return state==0 ? 1 : state ==1? -1:0 ;
}
return state==0? -1 : state ==1? 1:0 ;
}
return 0;
}
});
}
public static boolean methodExis(T info , String methodName) {
Class cls = (Class) info.getClass() ;
Method[] method = cls.getDeclaredMethods() ;
for(int i=0 ; iif(methodName.equals(method[i].getName())) {
return true ;
}
}
return false ;
}
public static Object getData(T info , String st) {
Class cls = (Class) info.getClass();
Method f = null;
Object obj = null;
try {
f = cls.getDeclaredMethod(st, new Class[0]);
obj = f.invoke(info);
} catch (Exception e) {
e.printStackTrace();
}
return obj ;
}
}
主方法调用
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
List infos = new ArrayList<>() ;
infos.add(new BookInfo("第一行代码" , "郭霖" ,40.2f)) ;
infos.add(new BookInfo("Android开发艺术" , "任玉刚" ,50.1f)) ;
infos.add(new BookInfo("疯狂Android讲义" , "李刚" ,45.4f)) ;
List listP = new ArrayList<>() ;
listP.add(new PeopleInfo("张三" , 21)) ;
listP.add(new PeopleInfo("李四" , 19)) ;
DataUtils.writeInfo(this , infos , "book");
DataUtils.writeInfo(this , listP , "people");
List copy = DataUtils.readInfo(this , "book") ;
List copy1 = DataUtils.readInfo(this , "people") ;
paint("默认输出" , copy , null , 0);
paint("价格升序排列打印" , copy , "getPrice" , 0);
paint("价格降序排列打印" , copy , "getPrice" , 1);
paint("人名升序排列打印" , copy , "getAuthor" , 0);
paint("人名升序排列打印" , copy1 , "getName" , 0);
}
public void paint( String logN, List list , String MethodName , int state){
Log.d("BookInfo" , logN) ;
DataUtils.sortInfo(list ,MethodName , state);
for(int i=0 ; i"BookInfo" , list.get(i).toString() ) ;
}
}
}
public void paint( String logN, List list , String MethodName , int state){
Log.d("BookInfo" , logN) ;
DataUtils.sortInfo(list ,MethodName , state);
for(int i=0 ; i"BookInfo" , list.get(i).toString() ) ;
}
}
}
打印信息 ,成功实现
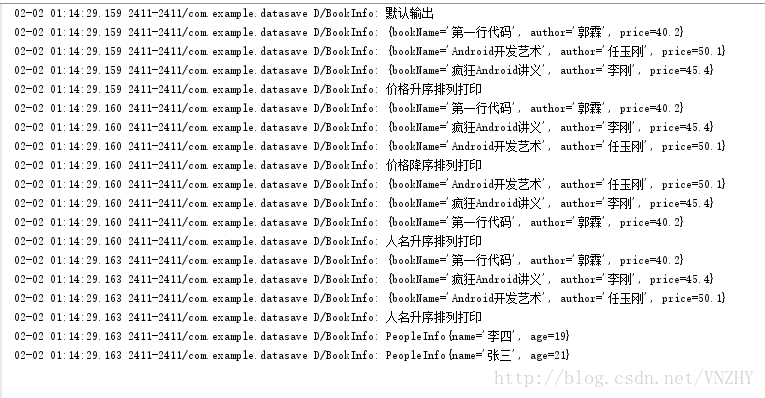
注:由于只做了list方法没优化,所以只能保存List< JavaBean序列化对象> 比如 List< List< info>> 、Map<> 等便无法保存 , 当然也可以自己去实现