Python Lambda & Functional Programming
函数式编程
匿名函数
纯函数
高阶函数
# higher-order functions
def apply_twice(func, arg):
return func(func(arg))
def add_five(x):
return x + 5
print(apply_twice(add_five, 10))
# Pure function
def pure_function(x, y):
temp = x + 2*y
return temp / (2*x + y)
# Impure function
some_list = []
def impure(arg):
some_list.append(arg)
#named function
def polynomial(x):
return x**2 + 5*x + 4
print(polynomial(-4))
#lambda
print((lambda x: x**2 + 5*x + 4) (-4))
double = lambda x: x * 2
print(double(7))
Decorators
装饰器
def decor(func):
def wrap():
print("============")
func()
print("============")
return wrap
def print_text():
print("Hello world!")
decorated = decor(print_text)
decorated()
print_text = decor(print_text)
print_text()
https://code.sololearn.com/391/#py
refs
https://www.sololearn.com/Play/Python/
https://repl.it/languages/python3
https://repl.it/languages/python
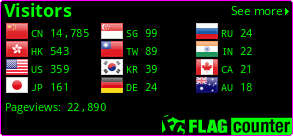
©xgqfrms 2012-2020
www.cnblogs.com 发布文章使用:只允许注册用户才可以访问!