import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from scipy.io import loadmat
from sklearn import svm
from sklearn.datasets import make_classification
data = loadmat('ex6data1.mat')
X = data['X']
Y = data['y']
# linear kernel
def plot_data_desicion(X, y):
pos = np.where(Y==1)[0] #表示行索引
neg = np.where(Y==0)[0]
plt.figure()
plt.scatter(X[pos,0], X[pos,1], marker='+', c='red')
plt.scatter(X[neg,0], X[neg,1], marker='o', c='blue')
# training
clf = svm.SVC(kernel='linear', C=100)
clf.fit(X, Y)
# accuracy
print('Test score: %.4f' % clf.score(X, Y))
# 方向向量
w = clf.coef_
b = clf.intercept_
x = np.linspace(np.min(X[:,0]),np.max(X[:,0]),100)
y = -(w[0,0]*x+b )/ w[0,1]
plt.plot(x, y)
plt.title('Decision Boundary')
plt.show()
data = loadmat('ex6data2.mat')
X = data['X']
Y = data['y']
# non-linear kernel
def plot_data_desicion(X, Y):
pos = np.where(Y==1)[0] #表示行索引
neg = np.where(Y==0)[0]
plt.figure()
plt.scatter(X[pos,0], X[pos,1], marker='+', c='red')
plt.scatter(X[neg,0], X[neg,1], marker='o', c='blue')
# training
clf = svm.SVC(kernel='rbf',gamma=100, C=100)
clf.fit(X, Y)
# accuracy
print('Test score: %.4f' % clf.score(X, Y))
a = np.transpose(np.linspace(np.min(X[:, 0]), np.max(X[:, 0]), 100).reshape(1, -1))
b = np.transpose(np.linspace(np.min(X[:, 1]), np.max(X[:, 1]), 100).reshape(1, -1))
X1, X2 = np.meshgrid(a, b)
boundary = np.zeros(X1.shape)
for i in range(X1.shape[1]):
this_X = np.hstack((X1[:, i].reshape(-1, 1), X2[:, i].reshape(-1, 1)))
boundary[:, i] = clf.predict(this_X)
plt.contour(X1, X2, boundary, [0, 1], color='blue')
plt.title('Decision Boundary')
plt.show()
plot_data_desicion(X,Y)
#
def plot_data_desicion(X, Y):
data = loadmat('ex6data3.mat')
X = data['X']
Y = data['y']
Xval = data['Xval']
Yval = data['yval']
pos = np.where(Y==1)[0] #表示行索引
neg = np.where(Y==0)[0]
plt.figure()
plt.scatter(X[pos,0], X[pos,1], marker='+', c='red')
plt.scatter(X[neg,0], X[neg,1], marker='o', c='blue')
# training
steps = [0.01, 0.03, 0.1, 0.3, 1, 3, 10, 30]
score, this_gamma, this_C = 0, 0, 0
for C in steps:
for gamma in steps:
clf = svm.SVC(kernel='rbf', C=C, gamma=gamma)
clf.fit(X, np.ravel(Y))
if clf.score(Xval, np.ravel(Yval)) > score:
score = clf.score(Xval, np.ravel(Yval))
this_C = C
this_gamma = gamma
# accuracy
# print('Test score: %.4f' % clf.score(X, Y))
model = svm.SVC(kernel='rbf', C=this_C, gamma=this_gamma)
model.fit(X, np.ravel(Y))
a = np.transpose(np.linspace(np.min(X[:, 0]), np.max(X[:, 0]), 100).reshape(1, -1))
b = np.transpose(np.linspace(np.min(X[:, 1]), np.max(X[:, 1]), 100).reshape(1, -1))
X1, X2 = np.meshgrid(a, b)
boundary = np.zeros(X1.shape)
for i in range(X1.shape[1]):
this_X = np.hstack((X1[:, i].reshape(-1, 1), X2[:, i].reshape(-1, 1)))
boundary[:, i] = model.predict(this_X)
plt.contour(X1, X2, boundary, [0, 1], color='blue')
plt.title('Decision Boundary')
plt.show()
plot_data_desicion(X,Y)
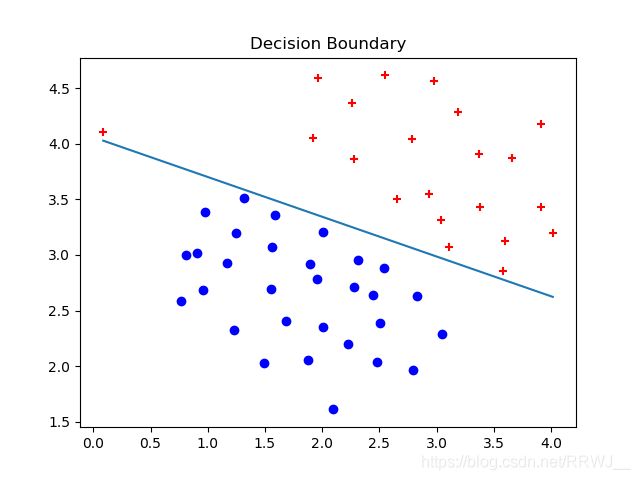
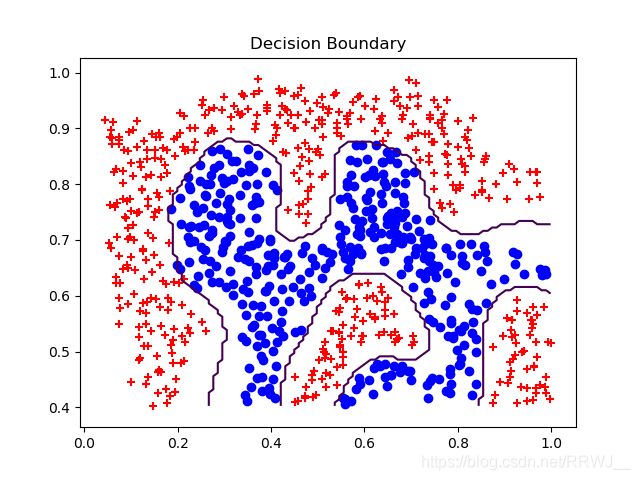
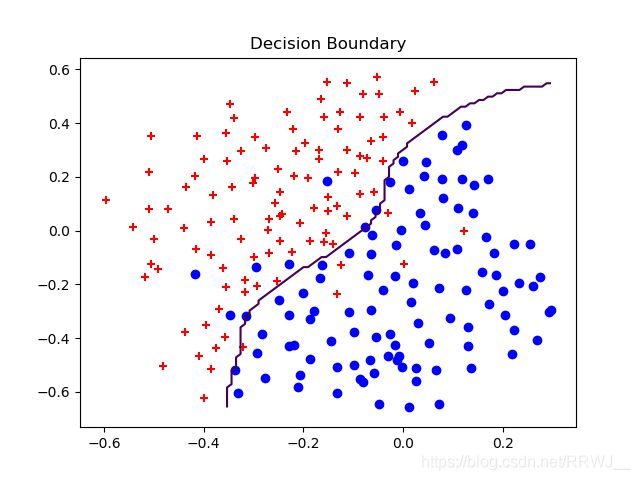