1 定义
- 概念:在BST(二叉搜索树)的基础上,任意一个结点的左右子树的高度差(平衡因子)的绝对值不超过1。
- AVL取自于创建它的两位前苏联的数学家(G.M.Adelse-Velskil和E.M.Landis)的名字。
2 基本操作
2.1 存储结构
struct node{
int data;
int height;
node *lchild, *rchild;
};
2.2 新建结点
node* newNode(int v){
node* Node = new node;
Node->data = v;
Node->height = 1;
Node->lchild = Node->rchild = NULL;
return Node;
}
2.3 获得以root为根结点的子树的当前height
int getHeight(node* root){
if(root == NULL) return 0;
return root->height;
}
2.4 计算root的平衡因子
int getBalandeFactor(node* root){
return getHeight(root->lchild) - getHeight(root->rchild);
}
2.5 更新root的height
void updateHeight(node* root){
root->height = max(getHeight(root->lchild), getHeight(root->rchild)) + 1;
}
2.6 插入结点
2.6.1 左单旋转
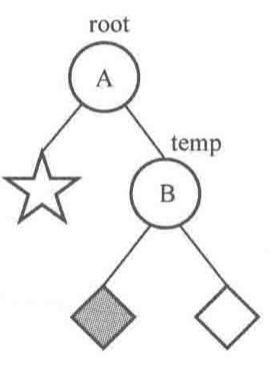
void L(node* &root){
node* temp = root->rchild;
root->rchild = temp->lchild;
temp->lchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
2.6.2 右单旋转
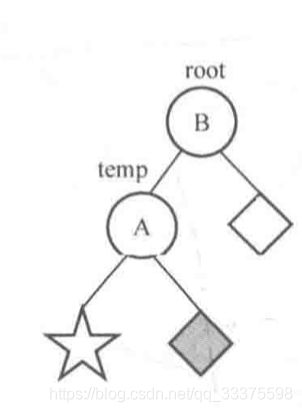
void R(node* &root){
node* temp = root->lchild;
root->lchild = temp->rchild;
temp->rchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
2.6.3 插入结点
void Insert(node* &root, int v){
if(root == NULL){
root = newNode(v);
return;
}
if(v < root->data){
Insert(root->lchild, v);
updateHeight(root);
if(getBalandeFactor(root) == 2){
if(getBalandeFactor(root->lchild) == 1){
R(root);
}else if(getBalandeFactor(root->lchild) == -1){
L(root->lchild);
R(root);
}
}
}else{
Insert(root->rchild, v);
updateHeight(root);
if(getBalandeFactor(root) == -2){
if(getBalandeFactor(root->rchild) == -1){
L(root);
}else if(getBalandeFactor(root->rchild) == 1){
R(root->rchild);
L(root);
}
}
}
2.7 创建AVL树
node* Create(int data[], int n){
node* root = NULL;
for (int i = 0; i < n; ++i)
{
Insert(root, data[i]);
}
return root;
}
2.8 查找结点值
void Search(node* root, int x){
if(root == NULL){
printf("Search fail!\n");
return;
}
if(x == root->data){
printf("%d\n", root->data);
}else if(x < root->data){
Search(root->lchild, x);
}else{
Search(root->rchild, x);
}
}
3.0 完整代码
#include
#include
using std::max;
struct node{
int data, height;
node *lchild, *rchild;
};
node* newNode(int v){
node* Node = new node;
Node->data = v;
Node->height = 1;
Node->lchild = Node->rchild = NULL;
return Node;
}
int getHeight(node* root){
if(root == NULL) return 0;
return root->height;
}
int getBalandeFactor(node* root){
return getHeight(root->lchild) - getHeight(root->rchild);
}
void updateHeight(node* root){
root->height = max(getHeight(root->lchild), getHeight(root->rchild)) + 1;
}
void Search(node* root, int x){
if(root == NULL){
printf("Search fail!\n");
return;
}
if(x == root->data){
printf("%d\n", root->data);
}else if(x < root->data){
Search(root->lchild, x);
}else{
Search(root->rchild, x);
}
}
void L(node* &root){
node* temp = root->rchild;
root->rchild = temp->lchild;
temp->lchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
void R(node* &root){
node* temp = root->lchild;
root->lchild = temp->rchild;
temp->rchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
void Insert(node* &root, int v){
if(root == NULL){
root = newNode(v);
return;
}
if(v < root->data){
Insert(root->lchild, v);
updateHeight(root);
if(getBalandeFactor(root) == 2){
if(getBalandeFactor(root->lchild) == 1){
R(root);
}else if(getBalandeFactor(root->lchild) == -1){
L(root->lchild);
R(root);
}
}
}else{
Insert(root->rchild, v);
updateHeight(root);
if(getBalandeFactor(root) == -2){
if(getBalandeFactor(root->rchild) == -1){
L(root);
}else if(getBalandeFactor(root->rchild) == 1){
R(root->rchild);
L(root);
}
}
}
}
node* Create(int data[], int n){
node* root = NULL;
for (int i = 0; i < n; ++i)
{
Insert(root, data[i]);
}
return root;
}
int main(int argc, char const *argv[])
{
int tree[7] = {100, 80, 60, 90, 120, 130, 110};
node* root = Create(tree, 7);
Search(root, 110);
Search(root,1);
return 0;
}