文章目录
- CAAnimation.h
- CALayer.h
- CAMediaTiming.h
- CAMediaTimingFunction.h
- CATransaction.h
- CATransform3D.h
- CAValueFunction.h
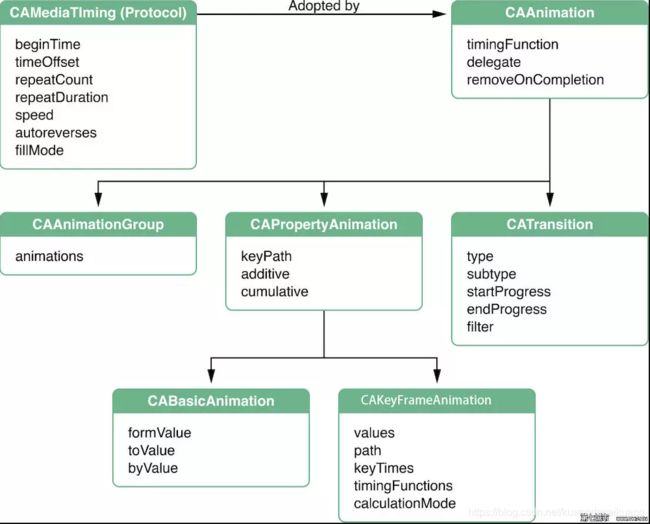
CAAnimation.h
#import
#import
typedef NSString * CAAnimationCalculationMode;
CAAnimationCalculationMode const kCAAnimationLinear;
CAAnimationCalculationMode const kCAAnimationDiscrete;
CAAnimationCalculationMode const kCAAnimationPaced;
CAAnimationCalculationMode const kCAAnimationCubic;
CAAnimationCalculationMode const kCAAnimationCubicPaced;
typedef NSString * CAAnimationRotationMode;
CAAnimationRotationMode const kCAAnimationRotateAuto;
CAAnimationRotationMode const kCAAnimationRotateAutoReverse;
typedef NSString * CATransitionType;
CATransitionType const kCATransitionFade;
CATransitionType const kCATransitionMoveIn;
CATransitionType const kCATransitionPush;
CATransitionType const kCATransitionReveal;
typedef NSString * CATransitionSubtype;
CATransitionSubtype const kCATransitionFromRight;
CATransitionSubtype const kCATransitionFromLeft;
CATransitionSubtype const kCATransitionFromTop;
CATransitionSubtype const kCATransitionFromBottom;
@interface CAAnimation : NSObject <NSSecureCoding, NSCopying, CAMediaTiming, CAAction>
+ (instancetype)animation;
+ (nullable id)defaultValueForKey:(NSString *)key;
- (BOOL)shouldArchiveValueForKey:(NSString *)key;
@property(nullable, strong) CAMediaTimingFunction *timingFunction;
@property(nullable, strong) id <CAAnimationDelegate> delegate;
@property(getter=isRemovedOnCompletion) BOOL removedOnCompletion;
@end
@protocol CAAnimationDelegate <NSObject>
@optional
- (void)animationDidStart:(CAAnimation *)anim;
- (void)animationDidStop:(CAAnimation *)anim finished:(BOOL)flag;
@end
@interface CAPropertyAnimation : CAAnimation
+ (instancetype)animationWithKeyPath:(nullable NSString *)path;
@property(nullable, copy) NSString *keyPath;
@property(getter=isAdditive) BOOL additive;
@property(getter=isCumulative) BOOL cumulative;
@property(nullable, strong) CAValueFunction *valueFunction;
@end
@interface CABasicAnimation : CAPropertyAnimation
@property(nullable, strong) id fromValue;
@property(nullable, strong) id toValue;
@property(nullable, strong) id byValue;
@end
@interface CAKeyframeAnimation : CAPropertyAnimation
@property(nullable, copy) NSArray *values;
@property(nullable) CGPathRef path;
@property(nullable, copy) NSArray<NSNumber *> *keyTimes;
@property(nullable, copy) NSArray<CAMediaTimingFunction *> *timingFunctions;
@property(copy) CAAnimationCalculationMode calculationMode;
@property(nullable, copy) NSArray<NSNumber *> *tensionValues;
@property(nullable, copy) NSArray<NSNumber *> *continuityValues;
@property(nullable, copy) NSArray<NSNumber *> *biasValues;
@property(nullable, copy) CAAnimationRotationMode rotationMode;
@end
@interface CASpringAnimation : CABasicAnimation
@property CGFloat mass;
@property CGFloat stiffness;
@property CGFloat damping;
@property CGFloat initialVelocity;
@property(readonly) CFTimeInterval settlingDuration;
@end
@interface CATransition : CAAnimation
@property(copy) CATransitionType type;
@property(nullable, copy) CATransitionSubtype subtype;
@property float startProgress;
@property float endProgress;
@end
@interface CAAnimationGroup : CAAnimation
@property(nullable, copy) NSArray<CAAnimation *> *animations;
@end
transform.rotation.x 围绕x轴翻转 参数:角度
transform.rotation.y 围绕y轴翻转 参数:同上
transform.rotation.z 围绕z轴翻转 参数:同上
transform.rotation 默认围绕z轴
transform.scale.x x方向缩放 参数:缩放比例 1.5
transform.scale.y y方向缩放 参数:同上
transform.scale.z z方向缩放 参数:同上
transform.scale 所有方向缩放 参数:同上
transform.translation.x x方向移动 参数:x轴上的坐标 100
transform.translation.y x方向移动 参数:y轴上的坐标
transform.translation.z x方向移动 参数:z轴上的坐标
transform.translation 移动 参数:移动到的点 (100,100)
position layer位置
position.x
position.y
transform CATransform3D 4*4矩阵
bounds layer大小
frame 不支持 frme 属性 computed from the bounds and position and is NOT animatable
anchorPoint 锚点位置
cornerRadius 圆角大小
zPosition z轴位置
CALayer.h
#import
#import
CAEmitterLayer 是一个粒子发射器系统,负责粒子的创建和发射源属性。
CAEAGLLayer 可以通过OpenGL ES来进行界面的绘制。
CAGradientLayer 可以创建出色彩渐变的图层效果
CAScrollLayer 可以支持其上管理的多个子层进行滑动,但是只能通过代码进行管理,不能进行用户点按触发
CAShapeLayer 可以让我们在layer层是直接绘制出自定义的形状。
CATextLayer 可以通过字符串进行文字的绘制
CATiledLayer 类似瓦片视图,可以将绘制分区域进行,常用于一张大的图片的分不分绘制。
CATransformLayer 用于构建一些3D效果的图层。
CAReplicatorLayer 用于对图层进行复制,包括图层的动画也能复制
typedef NSString * CALayerContentsGravity;
CALayerContentsGravity const kCAGravityCenter;
CALayerContentsGravity const kCAGravityTop;
CALayerContentsGravity const kCAGravityBottom;
CALayerContentsGravity const kCAGravityLeft;
CALayerContentsGravity const kCAGravityRight;
CALayerContentsGravity const kCAGravityTopLeft;
CALayerContentsGravity const kCAGravityTopRight;
CALayerContentsGravity const kCAGravityBottomLeft;
CALayerContentsGravity const kCAGravityBottomRight;
CALayerContentsGravity const kCAGravityResize;
CALayerContentsGravity const kCAGravityResizeAspect;
CALayerContentsGravity const kCAGravityResizeAspectFill;
typedef NSString * CALayerContentsFormat;
typedef NSString * CALayerContentsFilter;
CALayerContentsFilter const kCAFilterNearest;
CALayerContentsFilter const kCAFilterLinear;
CALayerContentsFilter const kCAFilterTrilinear;
typedef NSString * CALayerCornerCurve;
CALayerCornerCurve const kCACornerCurveCircular;
CALayerCornerCurve const kCACornerCurveContinuous;
NSString * const kCAOnOrderIn;
NSString * const kCAOnOrderOut;
NSString * const kCATransition
typedef NS_OPTIONS (unsigned int, CAEdgeAntialiasingMask) {
kCALayerLeftEdge = 1U << 0,
kCALayerRightEdge = 1U << 1,
kCALayerBottomEdge = 1U << 2,
kCALayerTopEdge = 1U << 3,
};
typedef NS_OPTIONS (NSUInteger, CACornerMask) {
kCALayerMinXMinYCorner = 1U << 0,
kCALayerMaxXMinYCorner = 1U << 1,
kCALayerMinXMaxYCorner = 1U << 2,
kCALayerMaxXMaxYCorner = 1U << 3,
};
@interface CALayer : NSObject <NSSecureCoding, CAMediaTiming>
+ (instancetype)layer;
- (instancetype)init;
- (instancetype)initWithLayer:(id)layer;
- (nullable instancetype)presentationLayer;
- (instancetype)modelLayer;
+ (nullable id)defaultValueForKey:(NSString *)key;
+ (BOOL)needsDisplayForKey:(NSString *)key;
- (BOOL)shouldArchiveValueForKey:(NSString *)key;
@property CGRect bounds;
@property CGPoint position;
@property CGFloat zPosition;
@property CGPoint anchorPoint;
@property CGFloat anchorPointZ;
@property CATransform3D transform;
- (CGAffineTransform)affineTransform;
- (void)setAffineTransform:(CGAffineTransform)m;
@property CGRect frame;
@property(getter=isHidden) BOOL hidden;
@property(getter=isDoubleSided) BOOL doubleSided;
@property(getter=isGeometryFlipped) BOOL geometryFlipped;
- (BOOL)contentsAreFlipped;
@property(nullable, readonly) CALayer *superlayer;
- (void)removeFromSuperlayer;
@property(nullable, copy) NSArray<__kindof CALayer *> *sublayers;
- (void)addSublayer:(CALayer *)layer;
- (void)insertSublayer:(CALayer *)layer atIndex:(unsigned)idx;
- (void)insertSublayer:(CALayer *)layer below:(nullable CALayer *)sibling;
- (void)insertSublayer:(CALayer *)layer above:(nullable CALayer *)sibling;
- (void)replaceSublayer:(CALayer *)oldLayer with:(CALayer *)newLayer;
@property CATransform3D sublayerTransform;
@property(nullable, strong) __kindof CALayer *mask;
@property BOOL masksToBounds;
- (CGPoint)convertPoint:(CGPoint)p fromLayer:(nullable CALayer *)l;
- (CGPoint)convertPoint:(CGPoint)p toLayer:(nullable CALayer *)l;
- (CGRect)convertRect:(CGRect)r fromLayer:(nullable CALayer *)l;
- (CGRect)convertRect:(CGRect)r toLayer:(nullable CALayer *)l;
- (CFTimeInterval)convertTime:(CFTimeInterval)t fromLayer:(nullable CALayer *)l;
- (CFTimeInterval)convertTime:(CFTimeInterval)t toLayer:(nullable CALayer *)l;
- (nullable __kindof CALayer *)hitTest:(CGPoint)p;
- (BOOL)containsPoint:(CGPoint)p;
@property(nullable, strong) id contents;
@property CGRect contentsRect;
@property(copy) CALayerContentsGravity contentsGravity;
@property CGFloat contentsScale;
@property CGRect contentsCenter;
@property(copy) CALayerContentsFormat contentsFormat
API_AVAILABLE(macos(10.12), ios(10.0), watchos(3.0), tvos(10.0));
@property(copy) CALayerContentsFilter minificationFilter;
@property(copy) CALayerContentsFilter magnificationFilter;
@property float minificationFilterBias;
@property(getter=isOpaque) BOOL opaque;
- (void)display;
- (void)setNeedsDisplay;
- (void)setNeedsDisplayInRect:(CGRect)r;
- (BOOL)needsDisplay;
- (void)displayIfNeeded;
@property BOOL needsDisplayOnBoundsChange;
@property BOOL drawsAsynchronously;
- (void)drawInContext:(CGContextRef)ctx;
- (void)renderInContext:(CGContextRef)ctx;
@property CAEdgeAntialiasingMask edgeAntialiasingMask;
@property BOOL allowsEdgeAntialiasing;
@property(nullable) CGColorRef backgroundColor;
@property CGFloat cornerRadius;
@property CGFloat borderWidth;
@property(nullable) CGColorRef borderColor;
@property float opacity;
@property BOOL allowsGroupOpacity;
@property(nullable, strong) id compositingFilter;
@property(nullable, copy) NSArray *filters;
@property(nullable, copy) NSArray *backgroundFilters;
@property BOOL shouldRasterize;
@property CGFloat rasterizationScale;
@property(nullable) CGColorRef shadowColor;
@property float shadowOpacity;
@property CGSize shadowOffset;
@property CGFloat shadowRadius;
@property(nullable) CGPathRef shadowPath;
- (CGSize)preferredFrameSize;
- (void)setNeedsLayout;
- (BOOL)needsLayout;
- (void)layoutIfNeeded;
- (void)layoutSublayers;
+ (nullable id<CAAction>)defaultActionForKey:(NSString *)event;
- (nullable id<CAAction>)actionForKey:(NSString *)event;
@property(nullable, copy) NSDictionary<NSString *, id<CAAction>> *actions;
- (void)addAnimation:(CAAnimation *)anim forKey:(nullable NSString *)key;
- (void)removeAllAnimations;
- (void)removeAnimationForKey:(NSString *)key;
- (nullable NSArray<NSString *> *)animationKeys;
- (nullable __kindof CAAnimation *)animationForKey:(NSString *)key;
@property(nullable, copy) NSString *name;
@property(nullable, weak) id <CALayerDelegate> delegate;
@property(nullable, copy) NSDictionary *style;
@end
@protocol CAAction
- (void)runActionForKey:(NSString *)event object:(id)anObject
arguments:(nullable NSDictionary *)dict;
@end
@protocol CALayerDelegate <NSObject>
@optional
- (void)displayLayer:(CALayer *)layer;
- (void)drawLayer:(CALayer *)layer inContext:(CGContextRef)ctx;
- (void)layoutSublayersOfLayer:(CALayer *)layer;
- (nullable id<CAAction>)actionForLayer:(CALayer *)layer forKey:(NSString *)event;
@end
CAMediaTiming.h
#import
#import
#import
typedef NSString * CAMediaTimingFillMode;
CAMediaTimingFillMode const kCAFillModeForwards;
CAMediaTimingFillMode const kCAFillModeBackwards;
CAMediaTimingFillMode const kCAFillModeBoth;
CAMediaTimingFillMode const kCAFillModeRemoved;
@protocol CAMediaTiming
@property CFTimeInterval beginTime;
@property CFTimeInterval duration;
@property float speed;
@property CFTimeInterval timeOffset;
@property float repeatCount;
@property CFTimeInterval repeatDuration;
@property BOOL autoreverses;
@property(copy) CAMediaTimingFillMode fillMode;
@end
CAMediaTimingFunction.h
#import
#import
typedef NSString * CAMediaTimingFunctionName;
CAMediaTimingFunctionName const kCAMediaTimingFunctionLinear;
CAMediaTimingFunctionName const kCAMediaTimingFunctionEaseIn;
CAMediaTimingFunctionName const kCAMediaTimingFunctionEaseOut;
CAMediaTimingFunctionName const kCAMediaTimingFunctionEaseInEaseOut;
CAMediaTimingFunctionName const kCAMediaTimingFunctionDefault;
@interface CAMediaTimingFunction : NSObject <NSSecureCoding>
+ (instancetype)functionWithName:(CAMediaTimingFunctionName)name;
+ (instancetype)functionWithControlPoints:(float)c1x :(float)c1y :(float)c2x :(float)c2y;
- (instancetype)initWithControlPoints:(float)c1x :(float)c1y :(float)c2x :(float)c2y;
- (void)getControlPointAtIndex:(size_t)idx values:(float[_Nonnull 2])ptr;
@end
CATransaction.h
#import
#import
@interface CATransaction : NSObject
+ (void)begin;
+ (void)commit;
+ (void)flush;
+ (void)lock;
+ (void)unlock;
+ (CFTimeInterval)animationDuration;
+ (void)setAnimationDuration:(CFTimeInterval)dur;
+ (nullable CAMediaTimingFunction *)animationTimingFunction;
+ (void)setAnimationTimingFunction:(nullable CAMediaTimingFunction *)function;
+ (BOOL)disableActions;
+ (void)setDisableActions:(BOOL)flag;
+ (nullable void (^)(void))completionBlock;
+ (void)setCompletionBlock:(nullable void (^)(void))block;
+ (nullable id)valueForKey:(NSString *)key;
+ (void)setValue:(nullable id)anObject forKey:(NSString *)key;
@end
NSString * const kCATransactionAnimationDuration;
NSString * const kCATransactionDisableActions;
NSString * const kCATransactionAnimationTimingFunction;
NSString * const kCATransactionCompletionBlock;
CATransform3D.h
struct CATransform3D
{
CGFloat m11, m12, m13, m14;
CGFloat m21, m22, m23, m24;
CGFloat m31, m32, m33, m34;
CGFloat m41, m42, m43, m44;
};
typedef struct CA_BOXABLE CATransform3D CATransform3D;
const CATransform3D CATransform3DIdentity;
bool CATransform3DIsIdentity (CATransform3D t);
bool CATransform3DEqualToTransform (CATransform3D a, CATransform3D b);
CATransform3D CATransform3DMakeTranslation (CGFloat tx, CGFloat ty, CGFloat tz);
CATransform3D CATransform3DTranslate (CATransform3D t, CGFloat tx, CGFloat ty, CGFloat tz);
CATransform3D CATransform3DMakeScale (CGFloat sx, CGFloat sy, CGFloat sz);
CATransform3D CATransform3DScale (CATransform3D t, CGFloat sx, CGFloat sy, CGFloat sz);
CATransform3D CATransform3DMakeRotation (CGFloat angle, CGFloat x, CGFloat y, CGFloat z);
CATransform3D CATransform3DRotate (CATransform3D t, CGFloat angle, CGFloat x, CGFloat y, CGFloat z);
CATransform3D CATransform3DConcat (CATransform3D a, CATransform3D b);
CATransform3D CATransform3DInvert (CATransform3D t);
CATransform3D CATransform3DMakeAffineTransform (CGAffineTransform m);
bool CATransform3DIsAffine (CATransform3D t);
CGAffineTransform CATransform3DGetAffineTransform (CATransform3D t);
@interface NSValue (CATransform3DAdditions)
+ (NSValue *)valueWithCATransform3D:(CATransform3D)t;
@property(readonly) CATransform3D CATransform3DValue;
@end
CAValueFunction.h
CAPropertyAnimation的属性, 插值计算方式
typedef NSString * CAValueFunctionName ;
@interface CAValueFunction : NSObject <NSSecureCoding>
+ (nullable instancetype)functionWithName:(CAValueFunctionName)name;
@property(readonly) CAValueFunctionName name;
@end
CAValueFunctionName const kCAValueFunctionRotateX;
CAValueFunctionName const kCAValueFunctionRotateY;
CAValueFunctionName const kCAValueFunctionRotateZ;
CAValueFunctionName const kCAValueFunctionScale;
CAValueFunctionName const kCAValueFunctionScaleX;
CAValueFunctionName const kCAValueFunctionScaleY;
CAValueFunctionName const kCAValueFunctionScaleZ;
CAValueFunctionName const kCAValueFunctionTranslate;
CAValueFunctionName const kCAValueFunctionTranslateX;
CAValueFunctionName const kCAValueFunctionTranslateY;
CAValueFunctionName const kCAValueFunctionTranslateZ;
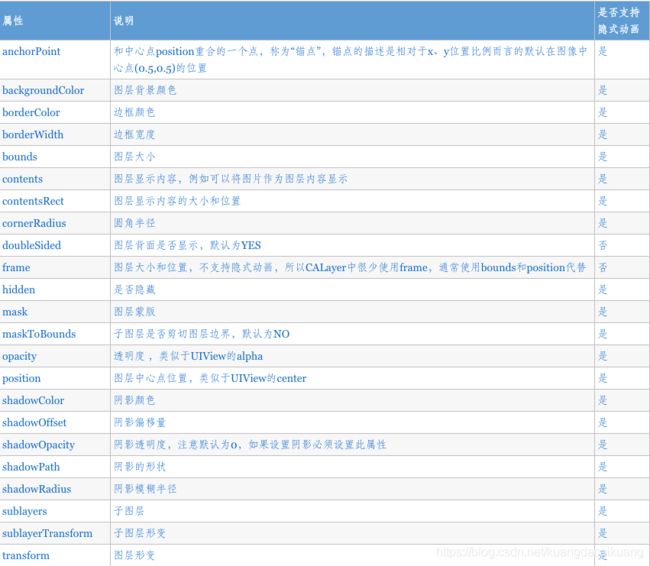