"""
用tkinter内置标准库制作一个记事本程序
"""
import tkinter as tk
from tkinter import scrolledtext
import fileinput
from tkinter import filedialog
import os
from tkinter import messagebox
from tkinter import simpledialog
from tkinter import colorchooser
from tkinter import Scrollbar
from tkinter import Listbox
from tkinter import StringVar
from tkinter import LabelFrame
from tkinter import Label
from tkinter import Button
from tkinter import font
class editor():
def __init__(self,rt):
if rt == None:
self.t = tk.Tk()
self.t.title("文本编辑器")
self.frm_file=tk.Frame(self.t,bg='gray')
self.frm_file.grid(row =0,column =0,padx =0,sticky = 'w')
self.btn_open=tk.Button(self.frm_file,text="打开",relief='groove',command=self.openfile)
self.btn_open.pack(side='left',padx=5,fill='both',expand=1)
self.btn_new=tk.Button(self.frm_file,text="新建",relief='groove',command=self.neweditor)
self.btn_new.pack(side='left',padx=5,fill='both',expand=1)
self.btn_save=tk.Button(self.frm_file,text='保存',relief='groove',command=self.savefile)
self.btn_save.pack(side='left',padx=5,fill='both',expand=1)
self.btn_saveas=tk.Button(self.frm_file,text='另存为',relief='groove',command=self.saveasfile)
self.btn_saveas.pack(side='left',padx=5,fill='both',expand=1)
self.btn_exit=tk.Button(self.frm_file,text='退出',relief='groove',command=self.die)
self.btn_exit.pack(side='left',padx=5,fill='both',expand=1)
self.frm_edit = tk.Frame(self.t,bg='gray')
self.frm_edit.grid(row =0,column =1,padx =1,sticky = 'w')
self.btn_copy = tk.Button(self.frm_edit,text = "复制",command=self.copy)
self.btn_copy.pack(side='left',padx=5,fill='both',expand=1)
self.btn_cut = tk.Button(self.frm_edit,text = "剪切",command=self.cut)
self.btn_cut.pack(side='left',padx=5,fill='both',expand=1)
self.btn_paste = tk.Button(self.frm_edit,text = "粘贴",command=self.paste)
self.btn_paste.pack(side='left',padx=5,fill='both',expand=1)
self.btn_find = tk.Button(self.frm_edit,text = "查询(未实现)",command=self.find_char)
self.btn_find.pack(side='left',padx=5,fill='both',expand=1)
self.btn_allselect = tk.Button(self.frm_edit,text = "全选",command=self.select_char_all)
self.btn_allselect.pack(side='left',padx=5,fill='both',expand=1)
self.btn_font = tk.Button(self.frm_edit,text = "字体样式",command=self.font_it)
self.btn_font.pack(side='left',padx=5,fill='both',expand=1)
self.btn_color = tk.Button(self.frm_edit,text = "字体颜色",command=self.color_it)
self.btn_color.pack(side='left',padx=5,fill='both',expand=1)
self.bar=tk.Menu(self.t)
self.filem=tk.Menu(self.bar)
self.filem.add_separator()
self.filem.add_command(label="新建",command=self.neweditor,accelerator=" Ctr+N")
self.filem.bind_all("",self.neweditor)
self.filem.add_command(label="打开",command=self.openfile,accelerator=" Ctr+O")
self.filem.bind_all("",self.savefile)
self.filem.add_command(label="保存",command=self.savefile,accelerator=" Ctr+S")
self.filem.bind_all("",self.savefile)
self.filem.add_command(label = "另存为",command = self.saveasfile,accelerator = " Ctr + Shif + S ")
self.filem.bind_all("",self.saveasfile)
self.filem.add_command(label = "关闭",command = self.close,accelerator = " F4")
self.filem.bind_all("",self.close)
self.filem.add_separator()
self.filem.add_command(label="退出",command=self.die,accelerator=" ESC")
self.filem.bind_all("",self.die)
self.bar.add_cascade(label = "文件",menu = self.filem)
self.editm=tk.Menu(self.bar)
self.editm.add_separator()
self.editm.add_command(label = "复制",command = self.copy,accelerator = " "*10 + "Ctr + C")
self.editm.bind_all("",self.copy)
self.editm.add_separator()
self.editm.add_command(label = "黏贴",command = self.paste,accelerator = " "*10 + "Ctr + V")
self.editm.bind_all("",self.paste)
self.editm.add_separator()
self.editm.add_command(label = "剪切",command = self.cut,accelerator = " "*10 + "Ctr + X")
self.editm.bind_all("",self.cut)
self.editm.add_separator()
self.editm.add_command(label = "删除",command = self.delete_text,accelerator = " "*10 + "Delete")
self.editm.bind_all("",self.delete_text)
self.editm.add_separator()
self.editm.add_command(label = "查找(未实现)",command = self.find_char,accelerator = " "*10 + "Ctr +F")
self.editm.bind_all("",self.find_char)
self.editm.add_separator()
self.editm.add_command(label = "全选",command = self.select_char_all,accelerator = " "*10 + "Ctr + A")
self.editm.bind_all("",self.select_char_all)
self.bar.add_cascade(label = "编辑",menu = self.editm)
self.formm = tk.Menu(self.bar)
self.formm.add_command(label = "字体颜色",command = self.color_it,accelerator = " "*10 + "Alt + C")
self.formm.bind_all("",self.color_it)
self.formm.add_separator()
self.formm.add_command(label = "字体格式",command = self.font_it,accelerator = " "*10 + "Alt + F")
self.formm.bind_all("",self.font_it)
self.bar.add_cascade(label = "格式",menu = self.formm)
self.helpm = tk.Menu(self.bar)
self.helpm.add_command(label = "关于",command = self.about)
self.bar.add_cascade(label = "帮助",menu = self.helpm)
self.t.config(menu = self.bar)
self.st=scrolledtext.ScrolledText(self.t)
self.st.grid(row=1,column=0,columnspan=3,pady=3)
def openfile(self,event =None):
oname=tk.filedialog.askopenfilename(filetypes=[("打开文件","*.txt")])
print(oname)
if oname:
self.st.delete(1.0, "end")
for line in fileinput.input(oname):
self.st.insert("end",line)
self.t.title(oname)
def neweditor(self,event=None):
global root
win_list.append(editor(root))
def savefile(self,event=None):
if os.path.isfile(self.t.title()):
opf=open(self.t.title(),"w")
opf.write(self.st.get(1.0,"end"))
opf.flush()
opf.close()
messagebox.showinfo("保存消息框","保存成功!")
else:
sname=tk.filedialog.asksaveasfilename(title = '保存',initialdir=os.getcwd(),initialfile = '新建文本.txt',filetypes=[("打开文件","*.txt")])
if sname:
ofp=open(sname,"w")
ofp.write(self.st.get(1.0,"end"))
ofp.flush()
ofp.close()
self.t.title(sname)
messagebox.showinfo("保存消息框","保存成功!")
def saveasfile(self,event=None):
sname=tk.filedialog.asksaveasfilename(title="另存为",filetypes=[("保存文件","*.txt")],defaultextension = ".txt")
if sname:
ofp=open(sname,"w")
ofp.write(self.st.get(1.0,"end"))
ofp.flush()
ofp.close()
self.t.title(sname)
def close(self,event=None):
self.t.destroy()
def die(self,event=None):
self.t.destroy()
def copy(self,event=None):
text = self.st.get(tk.SEL_FIRST,tk.SEL_LAST)
self.st.clipboard_clear()
self.st.clipboard_append(text)
def paste(self,event=None):
try:
text = self.st.selection_get(selection = "CLIPBOARD")
self.st.insert(tk.INSERT,text)
except tk.TclError:
pass
def cut(self,event=None):
text=self.st.get(tk.SEL_FIRST,tk.SEL_LAST)
self.st.delete(tk.SEL_FIRST,tk.SEL_LAST)
self.st.clipboard_clear()
self.st.clipboard_append(text)
def delete_text(self,event=None):
try:
self.st.delete(tk.SEL_FIRST,tk.SEL_LAST)
except tk.TclError:
pass
def find_char(self,event=None):
target=simpledialog.askstring("Search Dialog","寻找字符串",initialvalue="请输入要查询的字符")
end = self.st.index(tk.END)
print(type(end))
print(end)
endindex = end.split(".")
print(endindex)
end_line = int(endindex[0])
end_column = int(endindex[1])
pos_line =1
pos_column=0
length =len(target)
pass
def select_char_all(self,event=None):
self.st.tag_add(tk.SEL,1.0,tk.END)
self.st.see(tk.INSERT)
self.st.focus()
def color_it(self,event=None):
color=colorchooser.askcolor(title = 'Python')
self.st["foreground"] = color[1]
def font_it(self,event=None):
self.t_font=tk.Toplevel()
self.t_font.title("字体选择面板")
self.label_size=tk.Label(self.t_font,text="字体大小")
self.label_size.grid(row = 0 ,column =0,padx =30)
self.label_font=tk.Label(self.t_font,text = "字体类型")
self.label_font.grid(row = 0,column =2,padx =30)
self.label_shape=tk.Label(self.t_font,text = "字体形状")
self.label_shape.grid(row = 0,column =4,padx =30)
self.label_weight=tk.Label(self.t_font,text = "字体粗细")
self.label_weight.grid(row =0,column = 6,padx =30)
self.scroll_size = Scrollbar(self.t_font)
self.scroll_size.grid(row=1,column=1,stick="ns")
self.label_font = Scrollbar(self.t_font)
self.label_font.grid(row=1,column=3,stick="ns")
self.label_shape = Scrollbar(self.t_font)
self.label_shape.grid(row=1,column=5,stick="ns")
self.label_weight = Scrollbar(self.t_font)
self.label_weight.grid(row=1,column=7,stick="ns")
list_var_font=StringVar()
list_var_size=StringVar()
list_var_shape=StringVar()
list_var_weight=StringVar()
self.list_font=Listbox(self.t_font,selectmode="browse",listvariable=list_var_font,exportselection=0)
self.list_font.grid(row=1,column=2,padx=4)
list_font_item=["\"Arial\"","\"Arial Baltic\"","\"Arial Black\"","\"Arial CE\"","\"Arial CYR\"","\"Arial Greek\"","\"Arial Narrow\"",
"\"Arial TUR\"","\"Baiduan Number\"","\"Batang,BatangChe\""]
for item in list_font_item:
self.list_font.insert(0,item)
self.list_font.bind("",self.change_font)
self.list_size=Listbox(self.t_font,selectmode="browse",listvariable=list_var_size,exportselection=0)
self.list_size.grid(row=1,column=0,padx=4)
list_size_item=[1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17]
for item in list_size_item:
self.list_size.insert(0,item)
self.list_size.bind("",self.change_size)
self.list_shape=Listbox(self.t_font,selectmode="browse",listvariable=list_var_shape,exportselection=0)
self.list_shape.grid(row=1,column=4,padx=4)
list_shape_item=["italic","roman"]
for item in list_shape_item:
self.list_shape.insert(0,item)
self.list_shape.bind("",self.change_shape)
self.list_weight=Listbox(self.t_font,selectmode="browse",listvariable=list_var_weight,exportselection=0)
self.list_weight.grid(row=1,column=6,padx=4)
list_weight_item=["bold","normal"]
for item in list_weight_item:
self.list_weight.insert(0,item)
self.list_weight.bind("",self.change_weight)
self.font_display=LabelFrame(self.t_font,text="字体样式演示区域")
self.font_display.grid(row=2,column=0,pady=4)
self.lab_display=Label(self.font_display,text = "我在这里")
self.lab_display.pack()
self.btn_ok=Button(self.t_font,text="确定",width=8,height=2,command=self.change)
self.btn_ok.grid(row=2,column=2,pady=4)
self.btn_cancel=Button(self.t_font,text="取消",width=8,height=2,command=self.exit_subwindow)
self.btn_cancel.grid(row=2,column=4,pady=4)
def change_font(self,event):
tk.customFont = font.Font(family = "Helvetica",size = 12,weight = "normal",slant = "roman",underline =0)
family = tk.customFont["family"]
tk.customFont.configure(family =self.list_font.get(self.list_font.curselection()))
self.st.config(font = tk.customFont)
self.font_count = 1
def change_size(self,event):
tk.customFont = font.Font(family = "Helvetica",size = 12,weight = "normal",slant = "roman",underline =0)
size = tk.customFont["size"]
tk.customFont.configure(size =self.list_size.get(self.list_size.curselection()))
self.st.config(font = tk.customFont)
self.size_count = 1
def change_shape(self,event):
tk.customFont = font.Font(family = "Helvetica",size = 12,weight = "normal",slant = "roman",underline =0)
slant = tk.customFont["slant"]
tk.customFont.configure(slant =self.list_shape.get(self.list_shape.curselection()))
self.st.config(font = tk.customFont)
self.shape_count =1
def change_weight(self,event):
tk.customFont = font.Font(family = "Helvetica",size = 12,weight = "normal",slant = "roman",underline =0)
weight = tk.customFont["weight"]
tk.customFont.configure(weight =self.list_weight.get(self.list_weight.curselection()))
self.st.config(font = tk.customFont)
self.shape_count =1
def change(self):
self.st["font"]=(self.list_size.get(self.list_size.curselection()))
self.st["font"]=(self.list_font.get(self.list_font.curselection()))
self.st["font"]=(self.list_shape.get(self.list_shape.curselection()))
def exit_subwindow(self):
self.t_font.destroy()
def about(self,event=None):
tk.messagebox.showinfo(title="当前版本为1.0",message="作者:大哥\n状态:继续努力ing")
if __name__=="__main__":
win_list=[]
root=None
editor(root).t.mainloop()
以上代码为tkinter库的综合练习涉及一部分文件IO操作,包括打开硬盘上的txt文件,修改,另存为等操作
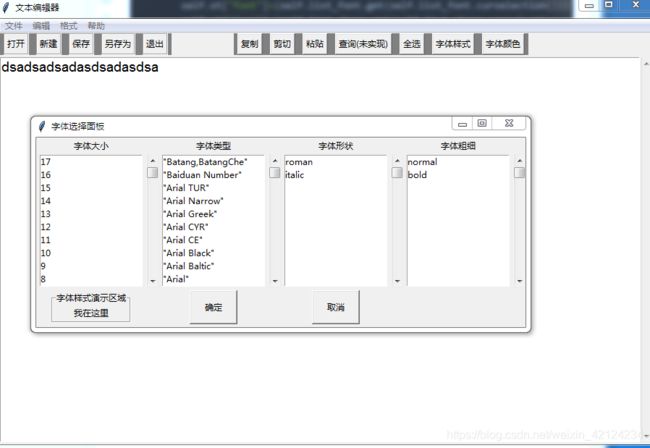